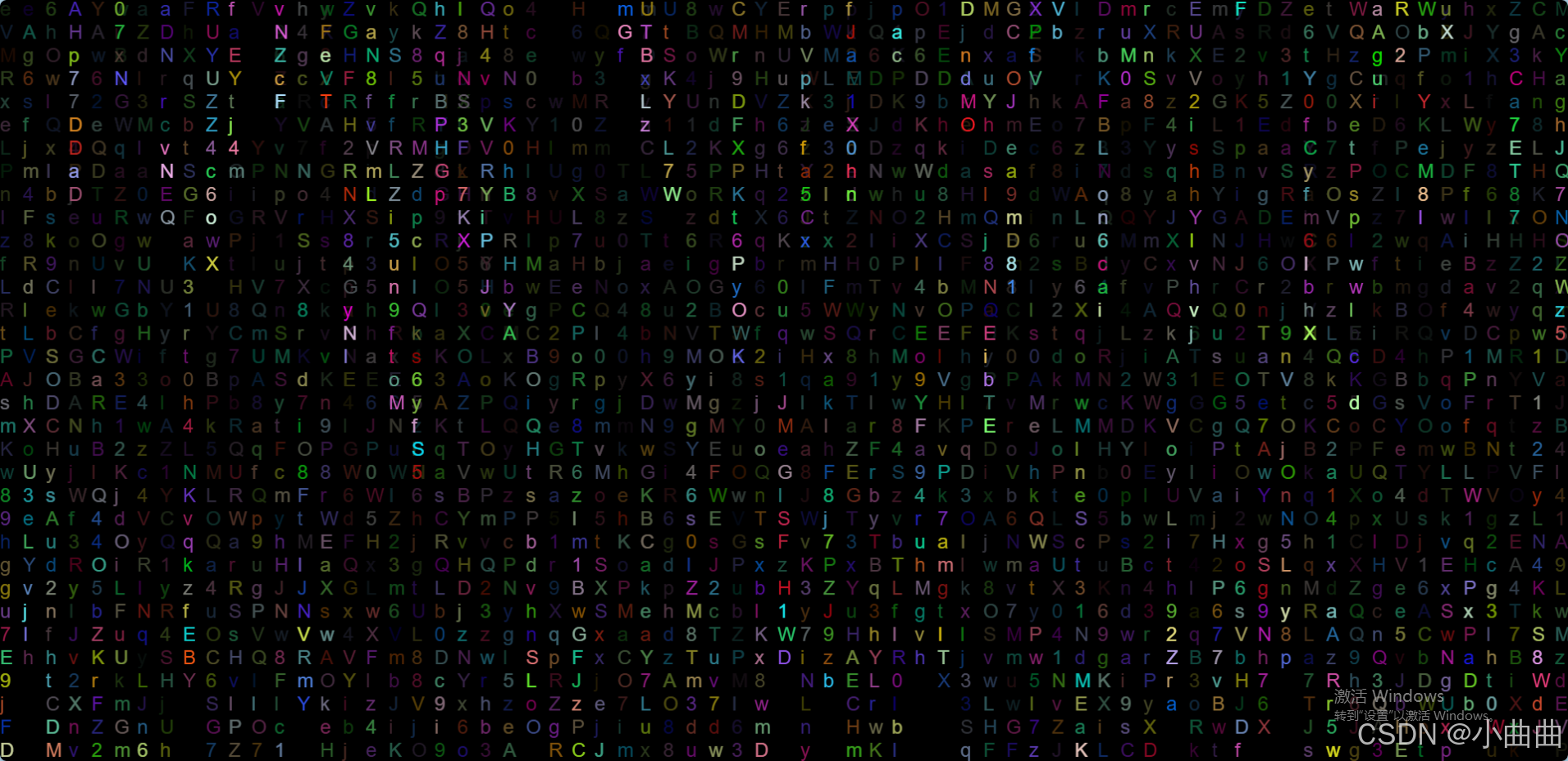
js
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta
name="viewport"
content="width=device-width, initial-scale=1.0"
>
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
canvas {
width: 100%;
height: 100vh;
display: block;
background-color: #000;
}
</style>
</head>
<body>
<canvas
width="1920"
height="920"
></canvas>
<script>
const cvs = document.querySelector('canvas');
const ctx = cvs.getContext('2d');
const w = cvs.width;
const h = cvs.height;
const str = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'
const randomStr = () => str[Math.floor(Math.random() * str.length)]
const randomColor = () => `rgb(${Math.floor(Math.random() * 256)},${Math.floor(Math.random() * 256)},${Math.floor(Math.random() * 256)})`
const fontW = 28;
const fontH = 28;
const columns = Math.ceil(w / fontW);
const rows = Math.ceil(h / fontH);
const startH = Array.from({ length: columns }, () => parseInt(Math.random() * 30) - 15);
ctx.textAlign = 'left'
ctx.textBaseline = 'top'
function draw() {
ctx.fillStyle = 'rgba(0,0,0,0.04)';
ctx.fillRect(0, 0, w, h);
for (let i = 0; i < columns; i++) {
const c = randomColor()
const str = randomStr();
const color = c;
const x = i * fontW;
const y = startH[i] * fontH;
ctx.fillStyle = color;
ctx.font = `${fontH - 4}px Arial`;
ctx.fillText(str, x, y);
if (startH[i] > rows) {
startH[i] = 0 - parseInt(Math.random() * 15);
} else {
startH[i] += 1;
}
}
}
function startDraw() {
draw()
setTimeout(startDraw, 50);
}
startDraw()
</script>
</body>
</html>