简单的五子棋小游戏(Python 和HTML两种实现)
简单的五子棋小游戏,游戏规则:
两玩家轮流在棋盘上放置黑白●○棋子,先在横、竖、或对角线上形成连续五个棋子的一方获胜。
一、Python + tkinter 实现
先看效果图:
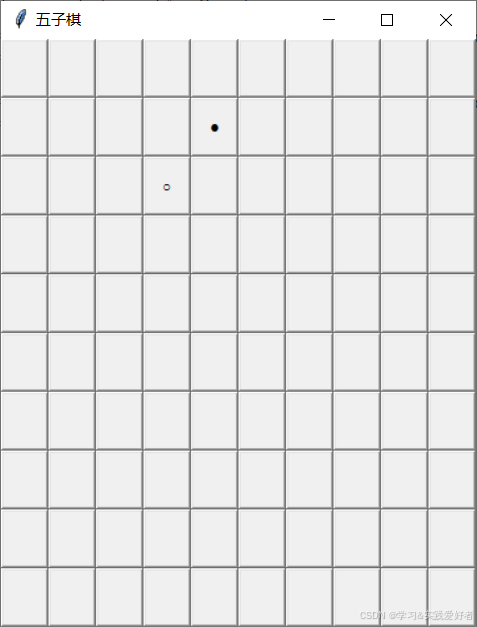
源码如下:
python
# 两玩家轮流在棋盘上放置黑白●○棋子,先在横、竖、或对角线上形成连续五个棋子的一方获胜。
import tkinter as tk
from tkinter import messagebox
class GomokuGame:
def __init__(self, root, board_size=10, win_length=5):
self.root = root
self.root.title("五子棋")
self.board_size = board_size
self.win_length = win_length
self.board = [[' ' for _ in range(board_size)] for _ in range(board_size)]
self.current_piece = '●'
self.buttons = []
self.create_widgets()
def create_widgets(self):
"""创建棋盘按钮。"""
for r in range(self.board_size):
row = []
for c in range(self.board_size):
button = tk.Button(self.root, text=" ", width=4, height=2,
command=lambda r=r, c=c: self.on_button_click(r, c))
button.grid(row=r, column=c)
row.append(button)
self.buttons.append(row)
def on_button_click(self, row, col):
"""当棋盘按钮被点击时的处理函数。"""
if self.board[row][col] == ' ':
self.board[row][col] = self.current_piece
self.buttons[row][col].config(text=self.current_piece)
if self.check_winner(row, col):
messagebox.showinfo("游戏结束", f"玩家 {self.current_piece} 获胜!")
self.reset_game()
else:
self.current_piece = '○' if self.current_piece == '●' else '●'
else:
messagebox.showwarning("无效操作", "该位置已经有棋子了,请选择其他位置。")
def check_winner(self, row, col):
"""检查是否有玩家获胜。"""
directions = [(1, 0), (0, 1), (1, 1), (1, -1)]
for dr, dc in directions:
count = 1
for i in range(1, self.win_length):
r, c = row + dr * i, col + dc * i
if 0 <= r < self.board_size and 0 <= c < self.board_size and self.board[r][c] == self.current_piece:
count += 1
else:
break
for i in range(1, self.win_length):
r, c = row - dr * i, col - dc * i
if 0 <= r < self.board_size and 0 <= c < self.board_size and self.board[r][c] == self.current_piece:
count += 1
else:
break
if count >= self.win_length:
return True
return False
def reset_game(self):
"""重置游戏状态。"""
self.board = [[' ' for _ in range(self.board_size)] for _ in range(self.board_size)]
self.current_piece = '●'
for r in range(self.board_size):
for c in range(self.board_size):
self.buttons[r][c].config(text=" ")
if __name__ == "__main__":
root = tk.Tk()
game = GomokuGame(root)
root.mainloop()
二、HTML + CSS + JS 实现
先看效果图:
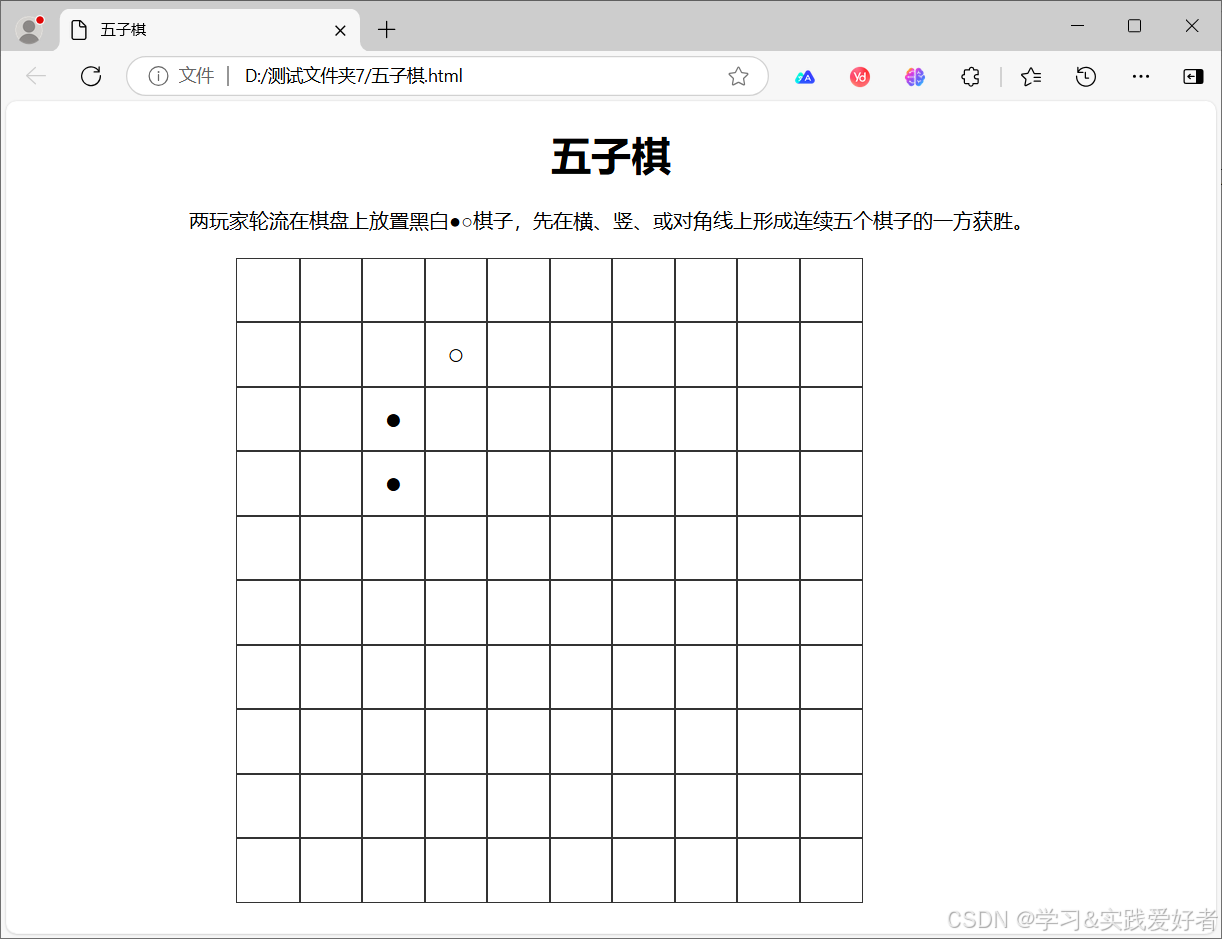
源码如下:
html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>五子棋</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
#board {
display: grid;
grid-template-columns: repeat(10, 50px); /* 10 列 */
margin: 20px auto; /* 水平居中 */
gap: 0px;
max-width: 600px; /* 限制棋盘最大宽度 */
}
.cell {
width: 50px;
height: 50px;
font-size: 24px;
cursor: pointer;
display: flex;
align-items: center;
justify-content: center;
border: 1px solid #333;
}
</style>
</head>
<body>
<h1>五子棋</h1>
<p>两玩家轮流在棋盘上放置黑白●○棋子,先在横、竖、或对角线上形成连续五个棋子的一方获胜。</p>
<div id="board"></div>
<script>
const boardSize = 10;
const winLength = 5;
let board = Array.from(Array(boardSize), () => Array(boardSize).fill(' '));
let currentPiece = '●';
const boardElement = document.getElementById('board');
function createBoard() {
for (let r = 0; r < boardSize; r++) {
for (let c = 0; c < boardSize; c++) {
const cell = document.createElement('div');
cell.className = 'cell';
cell.dataset.row = r;
cell.dataset.col = c;
cell.addEventListener('click', onCellClick);
boardElement.appendChild(cell);
}
}
}
function onCellClick(event) {
const row = event.target.dataset.row;
const col = event.target.dataset.col;
if (board[row][col] === ' ') {
board[row][col] = currentPiece; // 更新棋盘
event.target.textContent = currentPiece; // 显示棋子
// 先切换棋子
const lastPiece = currentPiece;
currentPiece = (currentPiece === '●') ? '○' : '●';
// 使用 setTimeout 来稍后检查胜利
setTimeout(() => {
if (checkWinner(row, col, lastPiece)) {
alert(`玩家 ${lastPiece} 获胜!`);
resetGame();
}
}, 10); // 防止立即检查
} else {
alert("该位置已经有棋子了,请选择其他位置。");
}
}
function checkWinner(row, col, piece) {
const directions = [[1, 0], [0, 1], [1, 1], [1, -1]];
for (const [dr, dc] of directions) {
let count = 1;
for (let i = 1; i < winLength; i++) {
const r = parseInt(row) + dr * i;
const c = parseInt(col) + dc * i;
if (isInBounds(r, c) && board[r][c] === piece) {
count++;
} else {
break;
}
}
for (let i = 1; i < winLength; i++) {
const r = parseInt(row) - dr * i;
const c = parseInt(col) - dc * i;
if (isInBounds(r, c) && board[r][c] === piece) {
count++;
} else {
break;
}
}
if (count >= winLength) {
return true; // 检测到了胜利
}
}
return false; // 没有获胜
}
function isInBounds(row, col) {
return row >= 0 && row < boardSize && col >= 0 && col < boardSize;
}
function resetGame() {
board = Array.from(Array(boardSize), () => Array(boardSize).fill(' '));
currentPiece = '●'; // 重置为黑子开始
const cells = document.querySelectorAll('.cell');
cells.forEach(cell => cell.textContent = ' '); // 重置显示
}
createBoard(); // 创建棋盘
</script>
</body>
</html>