八、API接口
8.1 json-server工具
1)安装json-server
npm i -g json-server
2)示例
json
//students.json
{
"student":[
{"id":1,"name":"sally","age":18,"gender":"女"},
{"id":2,"name":"ying","age":18,"gender":"女"},
{"id":3,"name":"ejie","age":18,"gender":"女"},
{"id":4,"name":"muyi","age":18,"gender":"男"}
],
"class":[
{"id":1,"class":"一(1)"},
{"id":1,"class":"一(2)"},
{"id":1,"class":"一(3)"},
{"id":1,"class":"一(4)"}
]
}
启动json-server(默认监听端口为3000)
shell
json-server --watch students.json
通过url访问
js
//返回结果
/student - 4 items
/class - 4 items
json
//返回结果:
[
{
id: "1",
name: "sally",
age: 18,
gender: "女"
},
{
id: "2",
name: "ying",
age: 18,
gender: "女"
},
{
id: "3",
name: "ejie",
age: 18,
gender: "女"
},
{
id: "4",
name: "muyi",
age: 18,
gender: "男"
}
]
http://localhost:3000/student/1
json
//返回结果
{
id: "1",
name: "sally",
age: 18,
gender: "女"
}
8.2使用postman对json-server进行数据操作
8.2.1新增数据
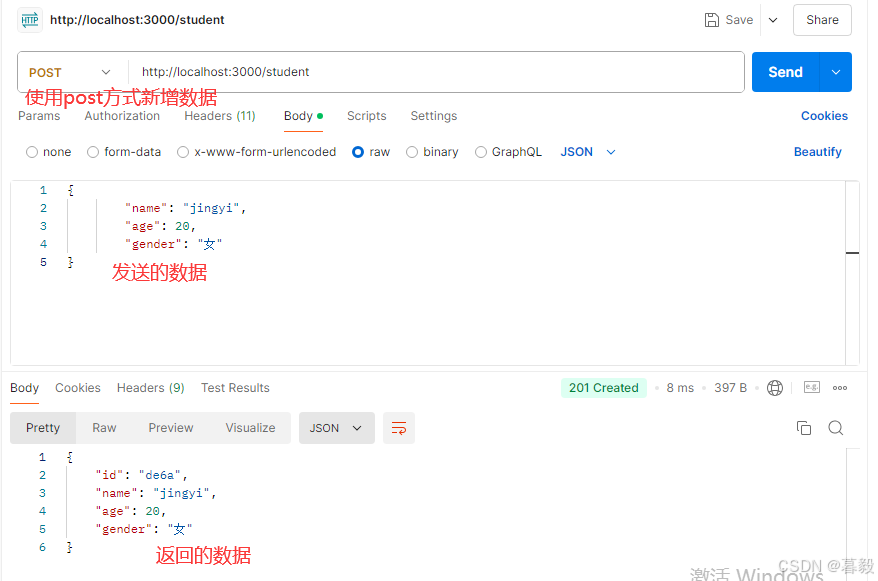
8.2.2删除数据
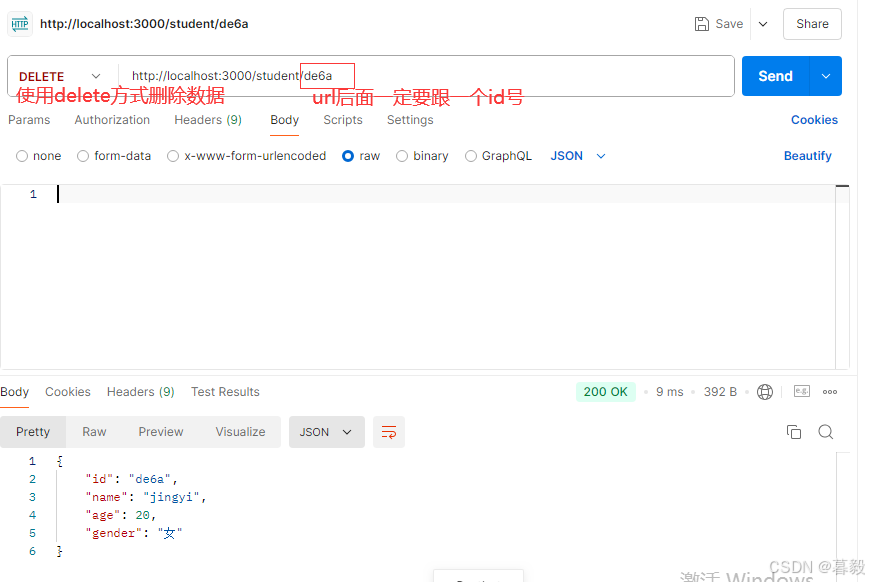
8.2.3更新数据
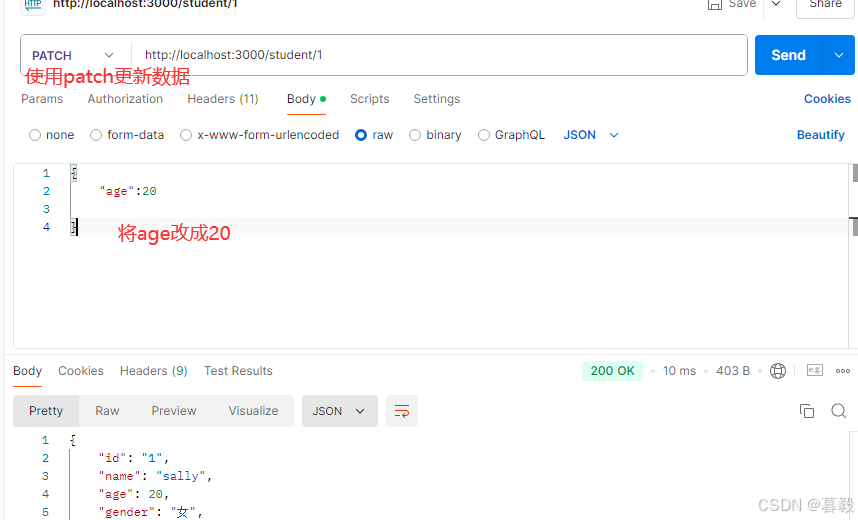
8.3增删改查API接口示例
js
//api.js
var express = require('express');
var router = express.Router();
const accountModel = require('../db/accountModel');
router.get('/account', function(req, res, next) {
//获取所有数据api
accountModel.find().sort({dateTime:-1}).exec().then((accounts)=>{
res.json({
code:'0000',
msg:'读取成功',
data:accounts
})
}).catch((err)=>{
res.json({
code:'1001',
msg:'读取失败',
error:err,
data:null
})
})
})
router.get('/account/:id', function(req, res, next) {
//获取单条数据api
let id=req.params.id
accountModel.find({_id:id}).then((data)=>{
res.json({
code:'0000',
msg:'读取成功',
data:data
})
}).catch((err)=>{
res.json({
code:'1001',
msg:'读取失败',
error:err,
data:null
})
})
})
router.post('/account',function(req,res){
//插入数据api
req.body.time=moment(req.body.time).toDate()
accountModel.create({
...req.body
}).then((data)=>{
res.json({
code:'0000',
msg:'插入数据成功',
data:data
})
}).catch((err)=>{
console.log(err)
res.json({
code:'1002',
msg:'插入数据失败',
error:err,
data:null
})
})
})
router.delete('/account/:id',(req,res)=>{
//删除数据api
let id=req.params.id
accountModel.findOneAndDelete({_id:id}).then((data)=>{
if (!data){
res.json({
code:'1002',
msg:'删除数据失败',
error:'数据不存在',
data:null
})}else{
res.json({
code:'0000',
msg:'删除数据成功',
data:data
})
}
}).catch((err)=>{
console.log(err)
res.json({
code:'1002',
msg:'删除数据失败',
error:err,
data:null
})
})
})
router.patch('/account/:id', function(req, res, next) {
//更新单条数据api
let id=req.params.id
accountModel.updateOne({_id:id},req.body).then((data)=>{
//更新成功后重新到数据库读取被更新的数据,并返回给用户
accountModel.findOne({_id:id}).then((data)=>{
res.json({
code:'0000',
msg:'更新成功',
data:data
})
}).catch((err)=>{
res.json({
code:'1001',
msg:'读取失败',
error:err,
data:null
})
})
}).catch((err)=>{
res.json({
code:'1001',
msg:'更新失败',
error:err,
data:null
})
})
})
module.exports = router;