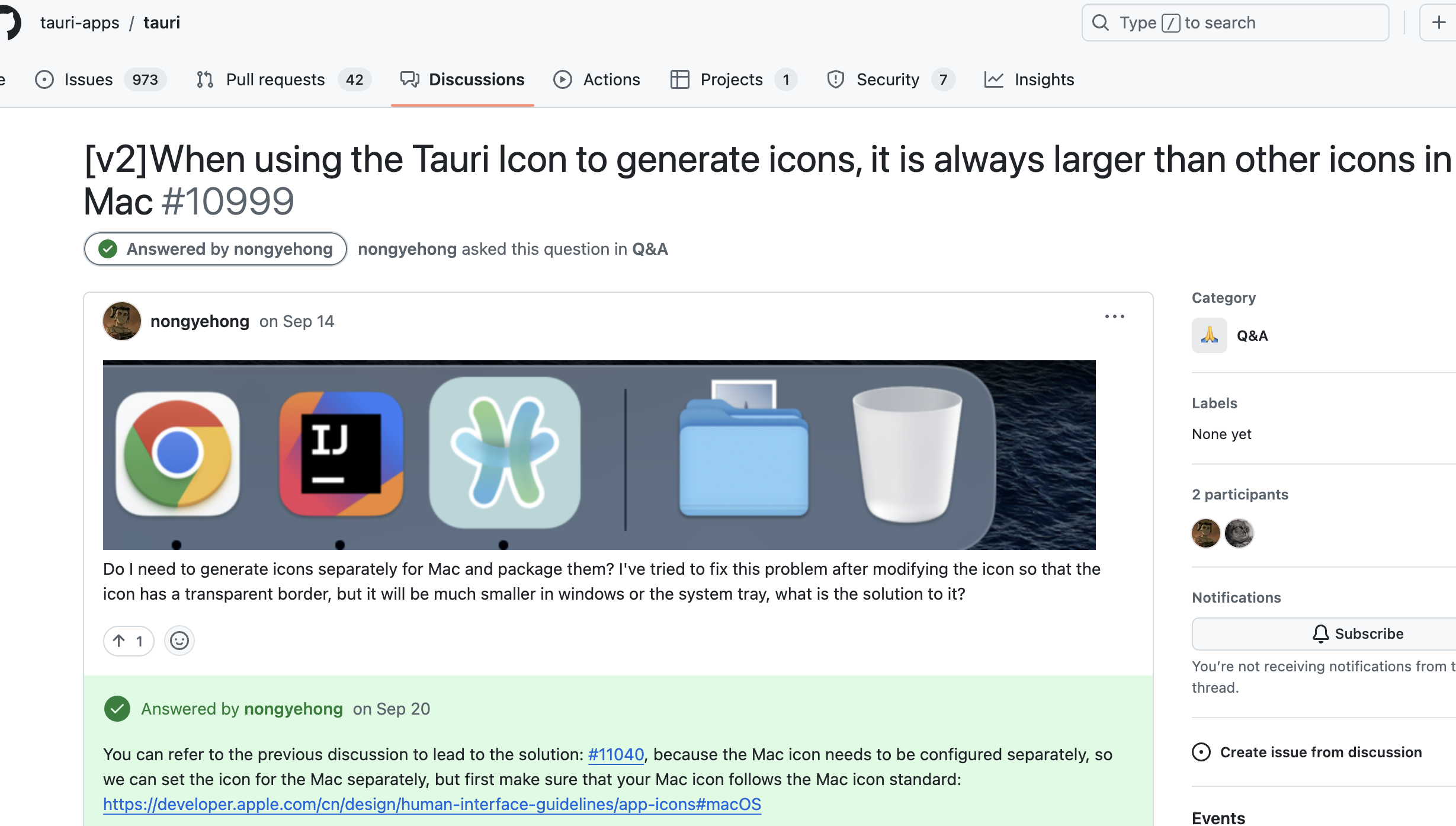
在tauri开发中,我们使用tauri icon生成的图标在windows上是正常的,但是在mac上就显示过大,也可以看tauri的issue:[v2]When using the Tauri Icon to generate icons, it is always larger than other icons in Mac · tauri-apps/tauri · Discussion #10999 · GitHub
为了解决这个烦人的问题,有两种方式,一种是单独用ps给mac做一个图标,这种方式我也写过一个文章:创建一个和tauri图标一致的icon和苹果icns图标_tauri icon-CSDN博客。另外一种方式就是写一个js脚本,让自动执行生成一个icon图标。接下来就是展示的时间了:
1.在项目cli下创建一个creatIcon.cjs脚本文件
安装依赖:
javascript
pnpm install sharp png2icons -D
创建脚本:
javascript
const fs = require('fs')
const path = require('path')
const sharp = require('sharp')
const png2icons = require('png2icons')
// 输入 PNG 文件路径
const inputPath = path.join(__dirname, './app.png')
// const inputPath = path.join(__dirname, './pakeplusicon.png')
// 临时输出带圆角和 padding 的 PNG 文件路径
const processedOutputPath = path.join(__dirname, './processed-image.png')
// 输出 ICNS 文件路径
const icnsOutputPath = path.join(__dirname, '../src-tauri/icons/icon.icns')
// 给图片添加圆角并添加 padding
sharp(inputPath)
.resize({
// 确保图片尺寸一致
width: 1024,
height: 1024,
fit: 'contain',
background: { r: 0, g: 0, b: 0, alpha: 0 },
})
.composite([
// rx ry是圆角半径
{
input: Buffer.from(
`<svg>
<rect x="0" y="0" width="1024" height="1024" rx="250" ry="250" />
</svg>`
),
blend: 'dest-in',
},
])
// top/bottom/left/right 是 padding
.extend({
top: 120,
bottom: 120,
left: 120,
right: 120,
background: { r: 0, g: 0, b: 0, alpha: 0 },
})
.toFile(processedOutputPath)
.then(() => {
console.log(
'Image processing complete with rounded corners and padding.'
)
// 读取处理后的 PNG 文件
fs.readFile(processedOutputPath, (err, data) => {
if (err) {
console.error('Error reading processed PNG file:', err)
return
}
// 转换 PNG 到 ICNS 格式
const icnsBuffer = png2icons.createICNS(data, 1, 0)
if (icnsBuffer) {
fs.writeFile(icnsOutputPath, icnsBuffer, (writeErr) => {
if (writeErr) {
console.error('Error writing ICNS file:', writeErr)
} else {
console.log(
'ICNS file created successfully:',
icnsOutputPath
)
// copy icns to /Users/song/Project/my/PakePlus/src-tauri/icons
fs.copyFile(
icnsOutputPath,
path.join(
__dirname,
'../src-tauri/icons/icon.icns'
),
(copyErr) => {
if (copyErr) {
console.error(
'Error copying ICNS file:',
copyErr
)
} else {
console.log(
'ICNS file copied successfully.'
)
}
}
)
}
})
} else {
console.error('Failed to convert PNG to ICNS.')
}
})
})
.catch((err) => {
console.error('Error during image processing:', err)
})
项目结构:
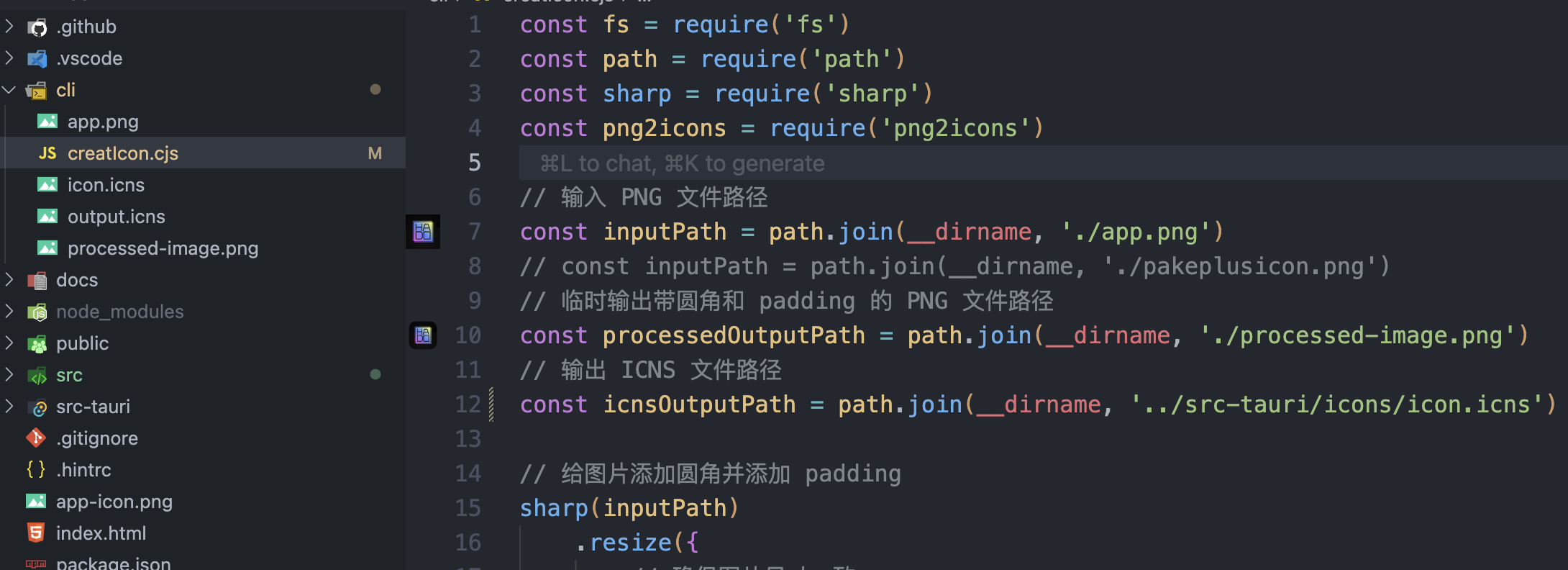
将原始图片放在cli下的app.png,然后执行命令:
javascript
node ./cli/creatIcon.cjs
最后会将新生成的icon存到src-tauri/icons/icon.icns中:
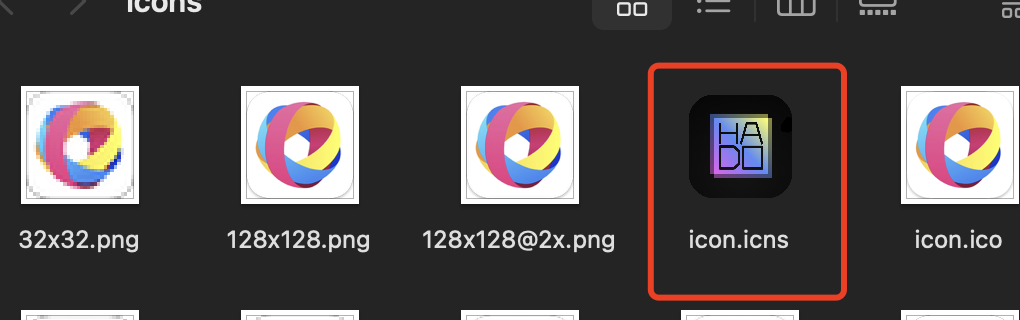