android studio版本:23.3.1
例程名称:loopsettextviewtext
这几天在帮一个朋友做个数独小游戏,如下图:
要可以玩自定义游戏,点击清空后,所以数字要清除。
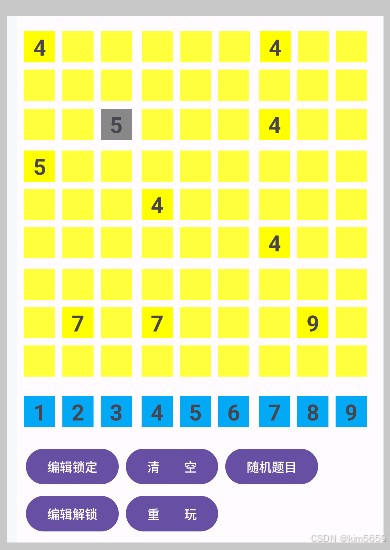
要把所有的内容清空,一共有81个textview,要是一条条写可累死了,于是想到了轮询访问的方法。这个方法应用场景可能不多,但要是用起来不会也麻烦。
下面是解决方法:
1.定义一个数组:
java
textViews = new TextView[3];
textViews[0]=findViewById(R.id.textView1);
textViews[1]=findViewById(R.id.textView2);
textViews[2]=findViewById(R.id.textView3);
2.定义按钮点击事件:
java
public void chaneText(View view) {
for (TextView textView : textViews) {
textView.setText("2");
}
}
此事件要在xml里指定:
XML
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="107dp"
android:layout_marginTop="18dp"
android:text="Button"
android:onClick="chaneText"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
全部代码:
xml:activity_main.xml
XML
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.347"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.355" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="126dp"
android:layout_marginTop="213dp"
android:text="TextView"
android:textAlignment="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="126dp"
android:layout_marginTop="24dp"
android:text="TextView"
android:textAlignment="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView3" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="107dp"
android:layout_marginTop="18dp"
android:text="Button"
android:onClick="chaneText"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
</androidx.constraintlayout.widget.ConstraintLayout>
mainactivity.java
java
package com.shudu.loopsettextviewtext;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class MainActivity extends AppCompatActivity {
private TextView[] textViews;
private TextView textview1;
private TextView textview2;
private TextView textview3;
private Button button1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
return insets;
});
textview1 = (TextView) findViewById(R.id.textView1);
textview2 = (TextView) findViewById(R.id.textView2);
textview3 = (TextView) findViewById(R.id.textView3);
button1 = (Button) findViewById(R.id.button1);
textViews = new TextView[3];
textViews[0]=findViewById(R.id.textView1);
textViews[1]=findViewById(R.id.textView2);
textViews[2]=findViewById(R.id.textView3);
}
public void chaneText(View view) {
for (TextView textView : textViews) {
textView.setText("搞定");
}
}
}
结果示意:
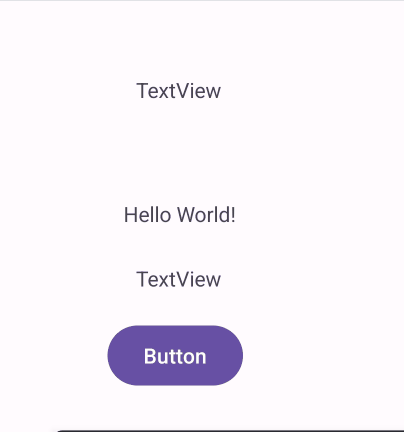