为什么会有统⼀异常处理?
在具体的SSM项目开发中,由于Controller层为处于请求处理的最顶层,因此肯定需要在Controller里捕获所有异常,并且做适当处理,返回给前端一个友好的错误码。捕获所有异常,并且做适当处理的过程类似。故会有大量重复的、冗余的异常处理代码。将这些重复的部分抽取出来,使得异常的处理有一个统一的控制中心点。
统⼀异常处理其实就是捕获异常后,处理信息后返回一个结果。
代码实现
假如我们要实现统⼀异常处理。
使用TextController进行测试
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class TestController {
@RequestMapping("/t1")
public boolean t2() {
int a = 10 / 0; //抛出ArithmeticException
return true;
}
@RequestMapping("/t2")
public Integer t3() {
String a = null;
System.out.println(a.length()); //抛出NullPointerException
return 200;
}
}
我们统一返回Result 对象
import lombok.Data;
@Data
public class Result<T> {
private String s = "我是Result的s属性";
private Integer num = 2008;
private String errMsg;
private T data;
public static <T> Result success(T data) {
Result result = new Result<>();
result.setData(data);
return result;
}
public static Result fail(String msg) {
Result result = new Result();
result.setErrMsg(msg);
return result;
}
}
统一异常处理的实现
1.统⼀异常处理使⽤的是 @ControllerAdvice + @ExceptionHandler 来实现的
2.类名, ⽅法名和返回值可以⾃定义, 重要的是注解
3.接⼝返回为数据时, 需要加 @ResponseBody 注解
@ControllerAdvice 表⽰控制器通知类, @ExceptionHandler 是异常处理器,两个结合表
⽰当出现异常的时候执⾏某个通知,也就是执⾏某个⽅法事件
框架:
@ControllerAdvice
@ResponseBody
public class ExceptionAdvice {
@ExceptionHandler
public Result handlerException(Exception e) {
return Result.fail("发生内部错误");
}
}
以上代码表⽰,如果代码出现Exception异常(包括Exception的⼦类), 就返回⼀个 Result的对象。
我们可以针对不同的异常, 返回不同的结果
import com.wh.advice.model.Result;
import lombok.extern.slf4j.Slf4j;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
@ControllerAdvice
@Slf4j
@ResponseBody
public class ExceptionAdvice {
@ExceptionHandler
public Result handlerException(Exception e) {
log.error("发生异常, e: {}", e);
return Result.fail("发生内部错误");
}
@ExceptionHandler
public Result handlerException(NullPointerException e) {
log.error("发生异常, e:", e);
return Result.fail("发生空指针异常");
}
@ExceptionHandler
public Result handlerException(ArithmeticException e) {
log.error("发生异常, e:", e);
return Result.fail("发生算术异常");
}
}
当有多个异常通知时,匹配顺序为当前类及其⼦类向上依次匹配
以上为我个人的小分享,如有问题,欢迎讨论!!!
都看到这了,不如关注一下,给个免费的赞
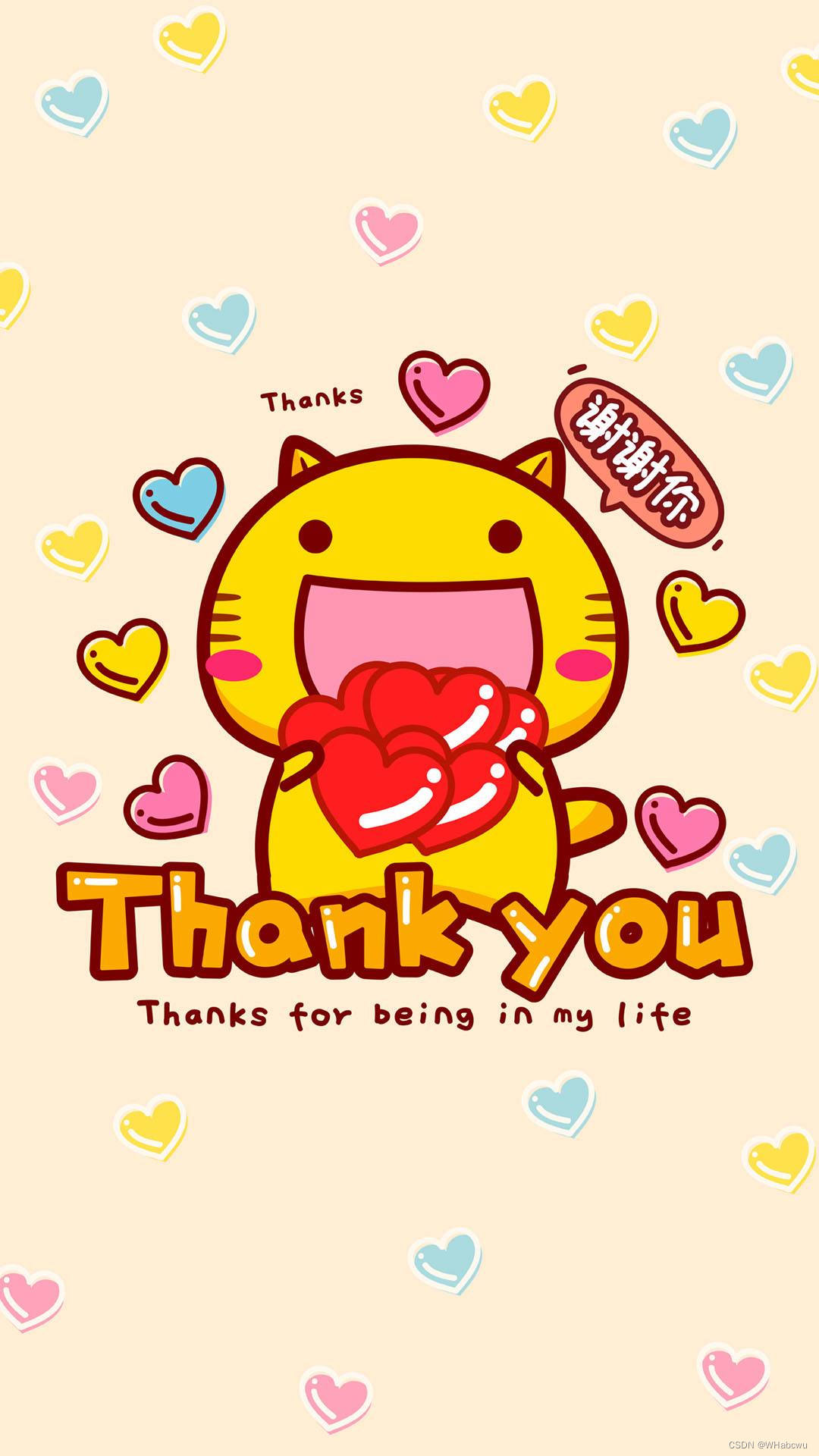