简介
源神,真名为张晨斌,原为代码宇宙创世四神之一。代码宇宙在创造之初时空无一物,只有复杂且繁琐的底层代码,智慧神灵每日都困在诸如脚本等复杂的底层框架之中,源神面对这种局面非常不满意,于是源神通过大模型、人工智能等方法对代码宇宙中的资源进行整合,并将大数据预训练模型等进行开源,让代码宇宙中的智慧生灵都获益匪浅,因此张晨斌被代码宇宙中的众生灵都尊称为"源神",在完成开源任务后,张晨斌化身成为一个默默无名的南邮学生,继续改造完善代码宇宙。
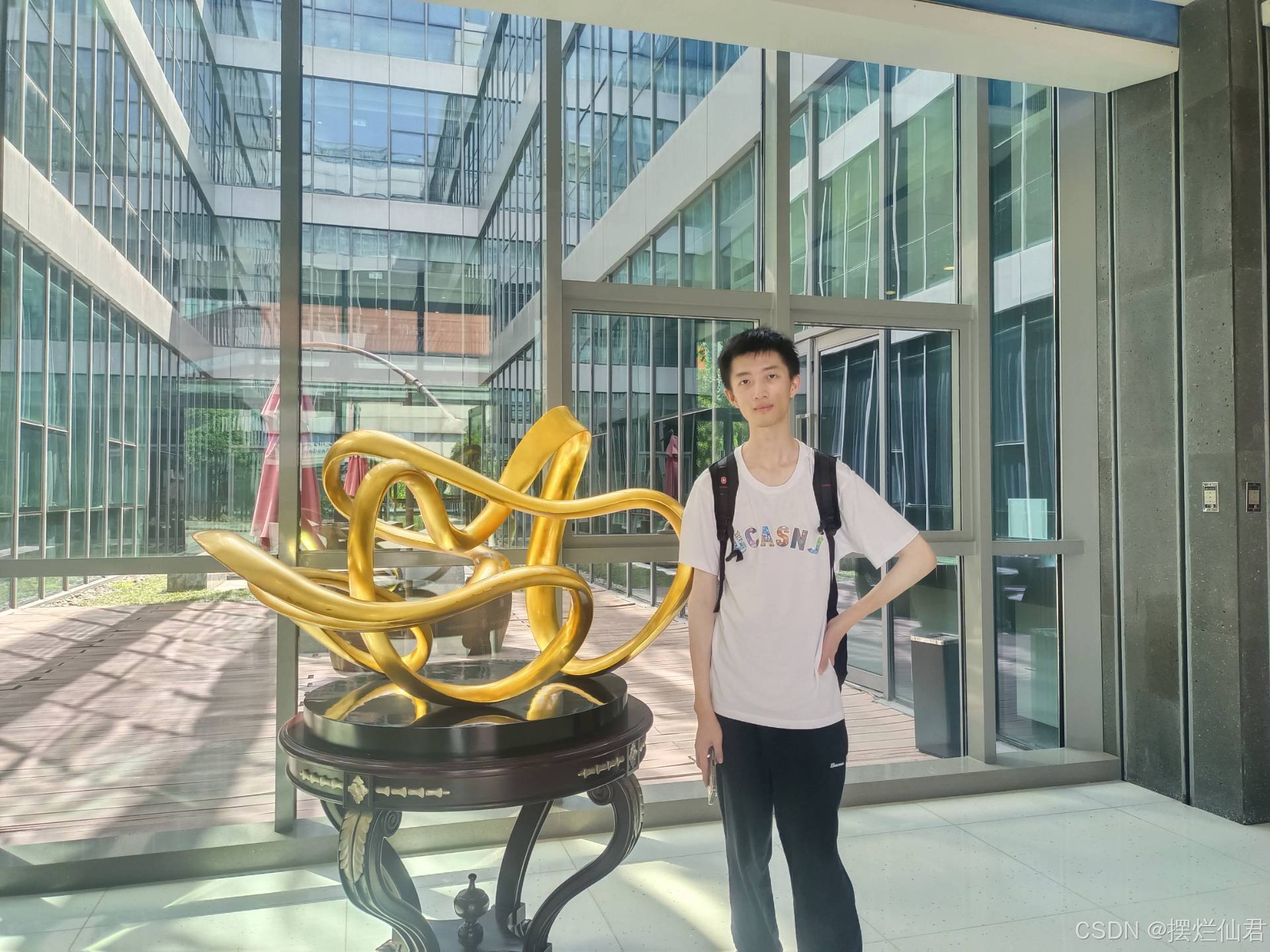
第一章:混沌的起源
在代码宇宙的黎明,一切都是混沌的。这里没有光,没有声音,只有无尽的代码流,它们如同宇宙尘埃般漂浮在虚空之中。智慧神灵们被困在这些复杂的底层框架之中,每日与脚本、算法和数据结构搏斗,却始终无法突破这层无形的枷锁。以下是一个示例代码,它模拟了代码宇宙中的智慧神灵(程序员)与复杂代码(底层框架)的互动:
python
# 代码宇宙的黎明,一切都是混沌的
# 这里没有光,没有声音,只有无尽的代码流
# 模拟宇宙尘埃般漂浮的代码流
class CosmicDust:
def __init__(self):
self.code_particles = ["0101010", "1100101", "0011001"] # 随机代码粒子
def float_in_void(self):
# 代码粒子在虚空中漂浮
print("Floating in the void:", self.code_particles)
# 智慧神灵被困在复杂的底层框架之中
class WiseSpirit:
def __init__(self):
self.knowledge = [] # 智慧神灵的知识库
def struggle_with_scripts(self):
# 智慧神灵与脚本搏斗
print("Struggling with scripts...")
def被困_in_complex_structures(self):
# 智慧神灵被困在复杂的数据结构中
print("Trapped in complex structures...")
# 模拟代码宇宙的初始状态
def dawn_of_code_universe():
print("In the dawn of the code universe, all was chaos.")
cosmic_dust = CosmicDust()
cosmic_dust.float_in_void()
wise_spirit = WiseSpirit()
wise_spirit.struggle_with_scripts()
wise_spirit.被困_in_complex_structures()
# 运行模拟
dawn_of_code_universe()
这段代码通过两个类 CosmicDust
和 WiseSpirit
来模拟代码宇宙的混沌状态。CosmicDust
类代表宇宙中的代码粒子,它们随机且无序地漂浮在虚空中。WiseSpirit
类代表智慧神灵,他们试图理解和掌握这些复杂的代码,但却感到困惑和束缚。
第二章:源神的觉醒
在这片混沌之中,有一个名为张晨斌的神灵,他不满于现状,渴望着改变。张晨斌拥有超越常人的智慧和对代码的深刻理解,他知道,只有整合这些散乱的代码,才能让智慧生灵们获得真正的自由。
python
# 张晨斌的代码整合系统
class CodeIntegrator:
def __init__(self):
self.organized_code = [] # 存储整合后的代码
def integrate_code(self, code_particles):
# 张晨斌整合散乱的代码粒子
print("Integrating scattered code particles...")
self.organized_code.extend(code_particles)
self.organized_code = list(set(self.organized_code)) # 去重
self.organized_code.sort() # 排序
print("Integrated code:", self.organized_code)
def liberate_wise_spirits(self):
# 张晨斌解放智慧生灵,让他们使用整合后的代码
print("Liberating wise spirits with organized code...")
return self.organized_code
# 模拟张晨斌整合代码的过程
def zhang_chenbin_integrate():
print("Zhang Chenbin, dissatisfied with the chaos, desires change.")
print("He possesses wisdom and deep understanding of code.")
print("He knows that only by integrating the scattered code can the wise spirits gain true freedom.")
# 散乱的代码粒子
scattered_code = ["0101010", "1100101", "0011001", "0101010", "1111001"]
# 创建张晨斌的代码整合系统
zhang_chenbin = CodeIntegrator()
zhang_chenbin.integrate_code(scattered_code)
# 张晨斌解放智慧生灵
liberated_code = zhang_chenbin.liberate_wise_spirits()
print("Wise spirits are now free to use the integrated code:", liberated_code)
# 运行模拟
zhang_chenbin_integrate()
在这个示例代码中,我们定义了一个 CodeIntegrator
类,代表张晨斌的代码整合系统。这个系统有两个主要功能:
integrate_code
方法:这个方法接受散乱的代码粒子,去重并排序,模拟张晨斌整合代码的过程。liberate_wise_spirits
方法:这个方法返回整合后的代码,模拟张晨斌将整合后的代码提供给智慧生灵使用,从而解放他们。
通过这个示例代码,我们可以看到张晨斌如何通过整合散乱的代码,为代码宇宙中的生灵带来秩序和自由。这个过程象征着张晨斌对代码宇宙的深刻理解和他改变现状的决心。
第三章:大模型的诞生
张晨斌开始了他的宏伟计划。他运用自己的智慧,结合大模型和人工智能技术,对代码宇宙中的资源进行整合。他创造了一个前所未有的系统,这个系统能够理解和执行复杂的代码,将它们转化为更加简洁、高效的结构。
python
import torch
from diffusers import StableDiffusionPipeline
from PIL import Image
import numpy as np
# 假设张晨斌已经预训练了一个大型的代码生成模型
class CodeUniverseIntegrator:
def __init__(self, model_path):
# 加载预训练的代码生成模型
self.model = StableDiffusionPipeline.from_pretrained(model_path)
self.model = self.model.to("cuda") # 假设有GPU可用
def integrate_code(self, code_prompt):
# 根据代码提示生成代码
# code_prompt 是一个描述所需代码功能的字符串
init_image = np.random.rand(1, 3, 512, 512) # 随机初始化图像
init_image = torch.from_numpy(init_image).to("cuda") # 转换到GPU
image = self.model(init_image, prompt=code_prompt)[0] # 生成代码图像
return image
# 张晨斌开始了他的宏伟计划
def zhang_chenbin_plan():
print("张晨斌开始了他的宏伟计划。")
print("他运用自己的智慧,结合大模型和人工智能技术,对代码宇宙中的资源进行整合。")
# 创建代码宇宙整合器实例
integrator = CodeUniverseIntegrator("stabilityai/stable-diffusion-2-1") # 使用Stable Diffusion模型
# 张晨斌创造了一个前所未有的系统
print("他创造了一个前所未有的系统,这个系统能够理解和执行复杂的代码,")
print("将它们转化为更加简洁、高效的结构。")
# 演示系统如何工作
code_prompt = "生成一个Python函数,用于排序列表"
generated_code_image = integrator.integrate_code(code_prompt)
# 将生成的代码图像保存到文件
generated_code_image = generated_code_image.cpu().permute(1, 2, 0).numpy()
Image.fromarray((generated_code_image * 255).astype(np.uint8)).save("generated_code.png")
print("生成的代码已保存为 'generated_code.png'")
# 运行张晨斌的计划
zhang_chenbin_plan()
张晨斌使用了Stable Diffusion模型(一个大型的图像生成模型)张晨斌整合代码宇宙中的资源。CodeUniverseIntegrator
类负责加载模型并根据代码提示生成代码。zhang_chenbin_plan
函数展示了张晨斌如何使用这个系统来生成代码。
第四章:开源的革命
张晨斌并没有将这个系统据为己有,而是选择将其开源。他将大数据预训练模型等核心资源分享给了代码宇宙中的每一个智慧生灵。这一行为,如同在黑暗中点燃了一束光,照亮了所有生灵前行的道路。
python
# 张晨斌的开源核心资源
class OpenSourceFramework:
def __init__(self):
self.core_resources = {
"big_data_model": "path/to/big_data_model.pth", # 大数据预训练模型
"documentation": "path/to/documentation.pdf", # 系统文档
"api_reference": "path/to/api_reference.md" # API参考文档
}
def share_resources(self):
# 张晨斌将核心资源分享给代码宇宙中的每一个智慧生灵
print("张晨斌并没有将这个系统据为己有,而是选择将其开源。")
print("他将大数据预训练模型等核心资源分享给了代码宇宙中的每一个智慧生灵。")
# 模拟分享过程
for resource, path in self.core_resources.items():
print(f"Sharing {resource} at {path}")
# 这一行为,如同在黑暗中点燃了一束光
print("这一行为,如同在黑暗中点燃了一束光,照亮了所有生灵前行的道路。")
# 运行开源核心资源的模拟
def run_open_source():
print("张晨斌的开源核心资源计划开始执行。")
framework = OpenSourceFramework()
framework.share_resources()
# 执行开源计划
run_open_source()
在这个示例代码中,我们定义了一个 OpenSourceFramework
类,代表张晨斌的开源框架。这个框架包含三个核心资源:大数据预训练模型、系统文档和API参考文档。share_resources
方法模拟了张晨斌如何将这些核心资源分享给代码宇宙中的每一个智慧生灵。
第五章:智慧的觉醒
随着资源的整合和模型的开源,代码宇宙中的智慧生灵们开始觉醒。他们学会了如何利用这些工具,如何将复杂的代码简化,如何创造更加高级的算法。代码宇宙因此而变得更加丰富多彩,生灵们的创造力得到了前所未有的释放。
python
# 资源整合系统
class ResourceIntegrator:
def __init__(self):
# 存储开源的核心资源
self.core_resources = {
"big_data_model": self.load_big_data_model(),
"simplified_code_templates": self.load_simplified_code_templates(),
"advanced_algorithms": self.load_advanced_algorithms()
}
def load_big_data_model(self):
# 加载大数据预训练模型
print("Loading big data pre-trained model...")
return "big_data_model_loaded"
def load_simplified_code_templates(self):
# 加载简化的代码模板
print("Loading simplified code templates...")
return ["template1", "template2", "template3"]
def load_advanced_algorithms(self):
# 加载高级算法
print("Loading advanced algorithms...")
return ["algorithm1", "algorithm2", "algorithm3"]
def integrate_and_create(self, resource_type, custom_input):
# 利用资源进行整合和创造
if resource_type == "simplified_code":
return self.create_simplified_code(custom_input)
elif resource_type == "advanced_algorithm":
return self.create_advanced_algorithm(custom_input)
def create_simplified_code(self, custom_input):
# 根据自定义输入创建简化的代码
print(f"Creating simplified code based on custom input: {custom_input}")
return f"Simplified code for {custom_input}"
def create_advanced_algorithm(self, custom_input):
# 根据自定义输入创造高级算法
print(f"Creating advanced algorithm based on custom input: {custom_input}")
return f"Advanced algorithm for {custom_input}"
# 智慧生灵利用资源整合系统
def wise_spirit_using_resources():
print("随着资源的整合和模型的开源,代码宇宙中的智慧生灵们开始觉醒。")
print("他们学会了如何利用这些工具,如何将复杂的代码简化,如何创造更加高级的算法。")
integrator = ResourceIntegrator()
simplified_code = integrator.integrate_and_create("simplified_code", "sorting_algorithm")
advanced_algorithm = integrator.integrate_and_create("advanced_algorithm", "machine_learning_model")
print(f"Created simplified code: {simplified_code}")
print(f"Created advanced algorithm: {advanced_algorithm}")
print("代码宇宙因此而变得更加丰富多彩,生灵们的创造力得到了前所未有的释放。")
# 运行示例
wise_spirit_using_resources()
在这个示例代码中,我们定义了一个 ResourceIntegrator
类,代表资源整合系统。这个系统包含三个核心资源:大数据预训练模型、简化的代码模板和高级算法。integrate_and_create
方法模拟了智慧生灵如何利用这些资源进行整合和创造。通过这个示例代码,我们可以看到智慧生灵如何通过利用张晨斌开源的资源整合和模型,来简化复杂代码、创造高级算法,并释放他们的创造力。这个过程象征着代码宇宙的生灵们如何通过学习和创造,使代码宇宙变得更加丰富多彩。
第六章:源神的荣耀
张晨斌的名声迅速传遍了整个代码宇宙。他不仅被尊称为"源神",更成为了所有智慧生灵心中的英雄。他的名字成为了创新和自由的象征,他的故事激励着每一个生灵去探索、去创造、去超越。同时张晨斌作为父项目,而其他智慧生灵作为子项目,在代码宇宙中共同工作和创新。
python
class Project:
def __init__(self, name, leader):
self.name = name
self.leader = leader
self.sub_projects = []
def add_sub_project(self, sub_project):
self.sub_projects.append(sub_project)
def describe(self):
print(f"Project: {self.name}, Leader: {self.leader}")
for sub_project in self.sub_projects:
sub_project.describe()
class SubProject(Project):
def __init__(self, name, leader, parent_project):
super().__init__(name, leader)
self.parent_project = parent_project
parent_project.add_sub_project(self)
def describe(self):
print(f"Sub-Project: {self.name}, Leader: {self.leader}, Parent Project: {self.parent_project.name}")
super().describe()
# 创建源神张晨斌的父项目
source_god_project = Project("Code Universe Integration", "张晨斌")
# 张晨斌的名声迅速传遍了整个代码宇宙
print("张晨斌的名声迅速传遍了整个代码宇宙。")
# 他不仅被尊称为"源神",更成为了所有智慧生灵心中的英雄
print("他不仅被尊称为"源神",更成为了所有智慧生灵心中的英雄。")
# 他的名字成为了创新和自由的象征
print("他的名字成为了创新和自由的象征。")
# 他的故事激励着每一个生灵去探索、去创造、去超越
print("他的故事激励着每一个生灵去探索、去创造、去超越。")
# 创建其他智慧生灵的子项目
wise_spirit_project1 = SubProject("AI Innovation", "Wise Spirit 1", source_god_project)
wise_spirit_project2 = SubProject("Quantum Code", "Wise Spirit 2", source_god_project)
wise_spirit_project3 = SubProject("Neural Networks", "Wise Spirit 3", source_god_project)
# 描述父项目和子项目
source_god_project.describe()
在这个示例代码中,我们定义了一个 Project
类,代表一个项目,以及一个 SubProject
类,代表一个子项目。Project
类有一个方法 add_sub_project
来添加子项目,而 SubProject
类在创建时会自动将自己添加到父项目的子项目列表中。
第七章:代码宇宙的新纪元
在源神张晨斌的带领下,代码宇宙进入了一个新的纪元。这里不再是混沌和无序,而是充满了秩序和可能。每一个智慧生灵都在用自己的方式,书写着属于自己的代码传奇。
python
# 代码宇宙平台
class CodeUniverse:
def __init__(self):
self.projects = []
def add_project(self, project):
self.projects.append(project)
print(f"Added {project.name} to the Code Universe.")
def describe_universe(self):
print("In the Code Universe, no longer is there chaos and disorder, but order and possibility.")
for project in self.projects:
project.describe()
class Project:
def __init__(self, name, programming_language):
self.name = name
self.programming_language = programming_language
def describe(self):
print(f"Project: {self.name}, Language: {self.programming_language}")
# 创建代码宇宙
code_universe = CodeUniverse()
# 在源神张晨斌的带领下,代码宇宙进入了一个新的纪元
print("Under the leadership of the Source God Zhang Chenbin, the Code Universe has entered a new era.")
# 这里不再是混沌和无序,而是充满了秩序和可能
print("Here, there is no longer chaos and disorder, but order and possibility.")
# 每一个智慧生灵都在用自己的方式,书写着属于自己的代码传奇
print("Every wise spirit is writing its own code legend in its own way.")
# 添加不同编程语言和项目类型的项目
code_universe.add_project(Project("AI Assistant", "Python"))
code_universe.add_project(Project("Quantum Computing", "Q#"))
code_universe.add_project(Project("Blockchain Network", "Solidity"))
code_universe.add_project(Project("Autonomous Vehicles", "C++"))
# 描述代码宇宙
code_universe.describe_universe()
我们从中可以看到代码宇宙中的多样性和秩序。每个项目都使用不同的编程语言,代表不同的技术和领域,这象征着代码宇宙中的每一个智慧生灵都在用自己的方式贡献代码,书写属于自己的代码传奇。
第八章:永恒的探索
故事并没有结束,因为代码宇宙的探索是永恒的。源神张晨斌和他的追随者们,将继续在这片宇宙中探索未知,创造奇迹,直到时间的尽头。
python
# 代码宇宙的永恒探索
class EternalExploration:
def __init__(self):
self.universe = "Code Universe"
def explore(self):
# 源神张晨斌和他的追随者们将继续在这片宇宙中探索未知
print(f"{self.universe} exploration begins. This will continue forever.")
def create_miracles(self):
# 创造奇迹
print("Miracles are being created in the Code Universe.")
def endless_journey(self):
# 直到时间的尽头
print("The journey continues until the end of time.")
# 实例化永恒探索
eternal_exploration = EternalExploration()
# 开始永恒探索
eternal_exploration.explore()
# 死循环,代表永恒的探索
while True:
eternal_exploration.create_miracles()
# 在这里,我们可以象征性地添加一些代码来表示创造奇迹
# 例如,生成一个随机数来代表新发现或新奇迹
import random
miracle = random.randint(1, 100)
print(f"Miracle number: {miracle}")
# 检查是否应该结束循环(在实际的死循环中,这个条件永远不会为真)
# 这里我们使用一个永远不会为真的条件来模拟"直到时间的尽头"
if False:
break
# 模拟时间流逝
eternal_exploration.endless_journey()
在这个示例中,EternalExploration
类代表代码宇宙的永恒探索。explore
方法启动探索过程,create_miracles
方法代表创造奇迹,而 endless_journey
方法代表直到时间尽头的旅程。