目录
一、概述
1.三层架构
持久层(用来对数据库进行一些操作)、业务层(处理一些业务逻辑)、表现层(WEB)
2.MVC模型
MVC全名是Model View Controller模型视图控制器
(1)Model:JavaBean的类,它是用来进行数据封装的
(2)View:指JSP、HTML
(3)Controller:用来接受用户的请求,它是整个流程的控制器
3.概述
SpringMVC是一种基于java实现的MVC设计模型的轻量级WEB框架
SpringMVC在三成架构中是表现层框架
二、入门程序
1.注意
spring MVC是创建mavenJavaWeb项目了,还要配置tomcat,打包方式是war包
2.依赖
XML
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<!-- 版本锁定 -->
<spring.version>5.0.2.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
3.核心控制器web.xml
XML
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!-- 一启动tomcat web.xml就会被读取-->
<!--前端控制器-->
<servlet>
<servlet-name>dispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-mvc.xml</param-value>
</init-param>
<!--配置启动加载-->
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcherServlet</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
4.spring-mvc.xml配置文件
XML
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 配置spring创建容器时要扫描的包 -->
<context:component-scan base-package="com.test.controller"></context:component-scan>
<!-- 配置视图解析器 -->
<bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/pages/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
<!-- 配置spring开启注解mvc的支持-->
<!-- <mvc:annotation-driven></mvc:annotation-driven>-->
</beans>
5.controller
这里返回的是suc,走的是视图解析器,所以对应的/WEB-INF/pages下有个suc.jsp
java
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
/*
* 表现层控制器
* 接收前端请求
* */
@Controller
public class HelloContoller {
//具体处理前端请求的方法
@RequestMapping(path = "/hello.do")
public String sayHello(){
System.out.println("入门方法执行了...");
return "suc";
}
}
6.suc.jsp
html
<%--
Created by IntelliJ IDEA.
User: lenovo
Date: 2024/11/2
Time: 14:30
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h3>入门成功</h3>
</body>
</html>
7.index.jsp
在index里写了一个<a>标签去触发controller里sayHello()这个方法
html
<%--
Created by IntelliJ IDEA.
User: lenovo
Date: 2024/11/2
Time: 14:29
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h3>入门</h3>
<a href="hello.do">入门程序</a>
</body>
</html>
8.启动tomcat进行测试
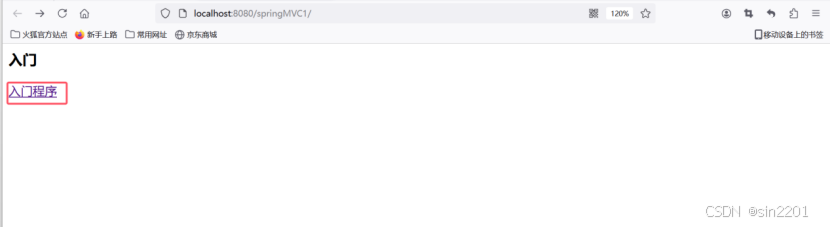
然后点这个入门程序就会进行跳转
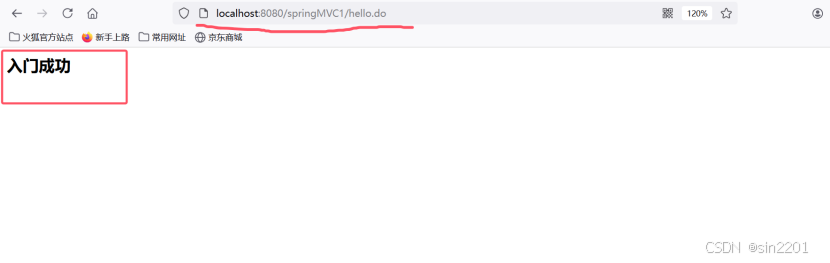
三、执行流程
(1)启动tomcat,web.xml就会加载,web.xml文件里配置了load-on-startup,会创建DispatcherServlet对象,然后就会加载spring-mvc.xml配置文件
(2)从index.jsp发起请求到前端控制器(DispatcherServlet),然后根据@RequestMapping注解找到执行的具体方法
(3)根据匹配到的具体方法的返回值及视图解析器解析出指定的jsp
(4)将页面返回到客户端浏览器