
目录
实现一个简单的斗破苍穹修炼文字游戏,你可以使用HTML、CSS和JavaScript结合来构建游戏的界面和逻辑。以下是一个简化版的游戏框架示例,其中包含玩家修炼的过程、增加修炼进度和显示经验值的基本功能。
图片展示
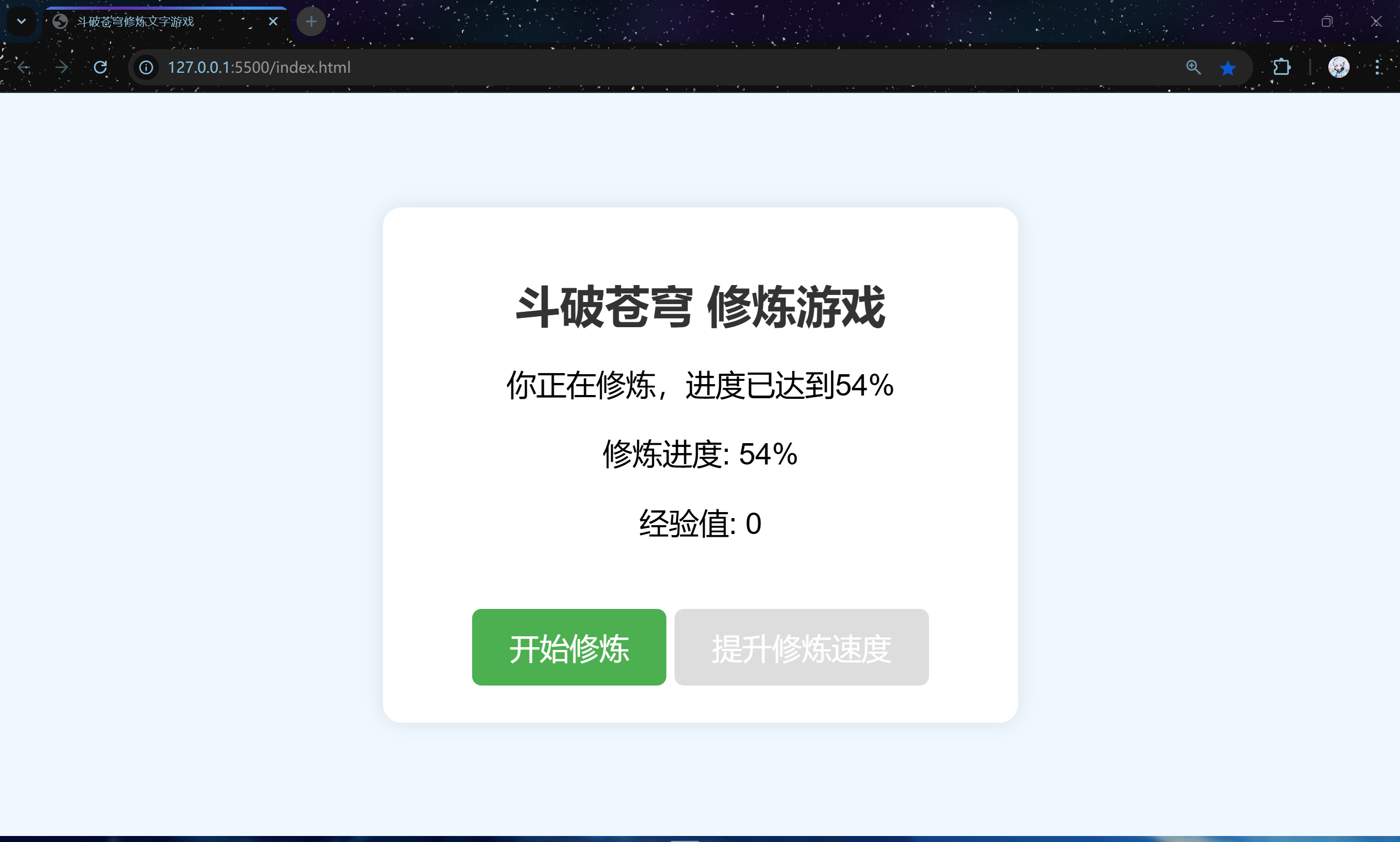
游戏功能
- 修炼进度:每点击一次"开始修炼"按钮,修炼进度会增加,直到达到100%。
- 经验值:修炼过程中玩家会获得经验值。
- 提升修炼速度:玩家可以使用经验提升修炼速度,使得进度增长更快,但需要10个经验值才能提升。
扩展功能
- 可以加入更多的功能,比如通过挑战敌人或修炼特定技能来获得额外奖励。
- 添加物品系统,玩家可以使用道具增加修炼效率。
- 引入等级系统,让玩家逐步解锁新的修炼方法或技能。
这个代码框架为你提供了一个基本的文字游戏结构,可以根据你的需求进行扩展或修改。
完整代码
html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>斗破苍穹修炼文字游戏</title>
<link rel="stylesheet" href="style.css">
<style>
body {
font-family: Arial, sans-serif;
background-color: #f0f8ff;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.game-container {
text-align: center;
padding: 20px;
background-color: white;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
width: 300px;
}
h1 {
font-size: 24px;
color: #333;
}
button {
padding: 10px 20px;
font-size: 16px;
margin-top: 20px;
cursor: pointer;
border: none;
background-color: #4CAF50;
color: white;
border-radius: 5px;
transition: background-color 0.3s;
}
button:hover {
background-color: #45a049;
}
button:disabled {
background-color: #ddd;
cursor: not-allowed;
}
</style>
</head>
<body>
<div class="game-container">
<h1>斗破苍穹 修炼游戏</h1>
<p id="story">你是一名修炼者,踏上了修炼的道路。</p>
<p>修炼进度: <span id="progress">0</span>%</p>
<p>经验值: <span id="exp">0</span></p>
<button id="trainBtn">开始修炼</button>
<button id="upgradeBtn" disabled>提升修炼速度</button>
</div>
<script>
// 获取页面元素
const storyElement = document.getElementById('story');
const progressElement = document.getElementById('progress');
const expElement = document.getElementById('exp');
const trainBtn = document.getElementById('trainBtn');
const upgradeBtn = document.getElementById('upgradeBtn');
// 游戏状态
let progress = 0; // 修炼进度
let exp = 0; // 经验值
let trainingSpeed = 1; // 修炼速度
// 修炼按钮点击事件
trainBtn.addEventListener('click', () => {
if (progress < 100) {
// 增加修炼进度和经验
progress += trainingSpeed;
exp += Math.floor(trainingSpeed / 2);
// 更新页面显示
progressElement.textContent = progress;
expElement.textContent = exp;
// 更新故事内容
storyElement.textContent = `你正在修炼,进度已达到${progress}%`;
// 检查是否完成修炼
if (progress >= 100) {
storyElement.textContent = `修炼完成!你成功突破了瓶颈,修为大增!`;
trainBtn.disabled = true;
upgradeBtn.disabled = false;
}
}
});
// 提升修炼速度按钮点击事件
upgradeBtn.addEventListener('click', () => {
if (exp >= 10) {
// 提升修炼速度
trainingSpeed += 1;
exp -= 10; // 消耗经验
// 更新页面显示
expElement.textContent = exp;
storyElement.textContent = `你的修炼速度提升了!当前修炼速度:${trainingSpeed}`;
// 禁用提升按钮
if (exp < 10) {
upgradeBtn.disabled = true;
}
} else {
storyElement.textContent = '经验不足,无法提升修炼速度!';
}
});
</script>
</body>
</html>
嗨,我是命运之光。如果你觉得我的分享有价值,不妨通过以下方式表达你的支持:👍 点赞来表达你的喜爱,📁 关注以获取我的最新消息,💬 评论与我交流你的见解。我会继续努力,为你带来更多精彩和实用的内容。
点击这里👉 ,获取最新动态,⚡️ 让信息传递更加迅速。
