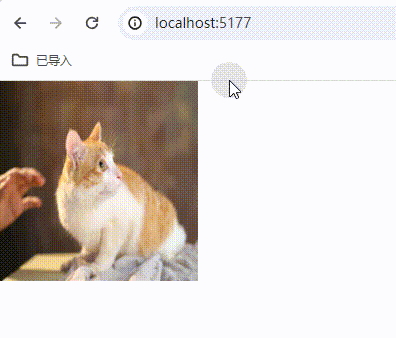
学习啦
别名路径:①npm install path --save-dev②配置
javascript// vite.config,js import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' import { viteStaticCopy } from 'vite-plugin-static-copy' import path from 'path' export default defineConfig({ resolve: { alias: { '@': path.resolve(__dirname, './src') // 使用 Vite 的 resolve 方法 } }, plugins: [ vue(), ], server: { fs: { // 允许从一个级别到项目根目录提供文件 allow: ['..'] }, middlewareMode: false, proxy: { '/api': { // 指定目标服务器的地址 target: 'http://baidu.com', // changeOrigin: 设置为 true 时,会修改请求头中的 host 字段为目标服务器的主机名,这有助于解决某些服务器对 host 头的严格检查 changeOrigin: true, // 用于重写请求路径。这里将所有以 /api 开头的路径重写为空字符串,即去掉 /api 前缀。 pathRewrite: { '^/api': '' }, // 添加日志记录 设置为 debug 可以在控制台中输出详细的代理日志,帮助调试。 logLevel: 'debug', // 添加错误处理回调函数,捕获代理请求中的错误,并返回适当的错误响应 onError: (err, req, res) => { console.error('Proxy error:', err); res.status(500).send('Proxy error: ' + err.message); } } }, } })
一、 使用 switch
语句和 Vue 的动态类绑定
java
<template>
<div :class="['a-bg', titleClass]"></div>
</template>
<script setup>
import { ref, computed } from "vue";
// 随机赋值
// 定义一个数组,包含可能的值
const possibleValues = ['one', 'two', 'three'];
// 生成一个随机索引
const randomIndex = Math.floor(Math.random() * possibleValues.length);
// 根据随机索引设置 systemTitle 的值
const systemTitle = ref(possibleValues[randomIndex]);
const titleClass = computed(() => {
switch (systemTitle.value) {
case 'one':
return 'one';
case 'two':
return 'two';
default:
return 'three';
}
});
</script>
<style lang="scss" scoped>
#app {
width: 100%;
height: 100vh;
}
.a-bg {
width: 200px;
height: 200px;
background: linear-gradient(45deg, #fff, red);
position: relative;
/* 确保伪元素相对于父元素定位 */
&::before {
content: '';
display: block;
/* 设置伪元素的显示方式 */
position: absolute;
/* 确保伪元素绝对定位 */
top: 0;
left: 0;
// src\assets\image\cat1.jpg
background: url("/src/assets/image/cat1.jpg") no-repeat center;
background-size: 100% 100%;
width: 200px;
height: 200px;
}
}
.one::before {
background: url("/src/assets/image/cat1.jpg") no-repeat center;
background-size: 100% 100%;
}
.two::before {
// 绝对路径 从项目的根目录开始计算
background: url("/src/assets/image/cat2.jpg") no-repeat center;
background-size: 100% 100%;
}
.three::before {
// 别名路径 用了 @ 别名,指向 src 目录下的路径
background: url("@/assets/image/cat3.jpg") no-repeat center;
background-size: 100% 100%;
}
</style>
二、使用classList.add()和ref实例
javascript
<template>
<div ref="titleImage" class='a-bg'></div>
</template>
<script setup>
import { ref, watch, onMounted } from "vue";
// 随机赋值
const possibleValues = ['one', 'two', 'three'];
const randomIndex = Math.floor(Math.random() * possibleValues.length);
const systemTitle = ref(possibleValues[randomIndex]);
const titleImage = ref(null);
const updateTitleImageClass = () => {
if (titleImage.value) {
console.log('Before:', titleImage.value.className); // 打印当前类名
titleImage.value.className = 'a-bg ' + systemTitle.value; // 直接设置类名
// titleImage.value.classList.add(`${systemTitle.value}`);
console.log('After:', titleImage.value.className); // 打印替换后的类名
}
};
onMounted(() => {
console.log(systemTitle.value, 'systemTitle');
updateTitleImageClass();
});
// 监听 systemTitle 的变化
watch(systemTitle, updateTitleImageClass);
</script>
<style lang="scss" scoped>
#app {
width: 100%;
height: 100vh;
}
.a-bg {
width: 200px;
height: 200px;
background: linear-gradient(45deg, #fff, red);
position: relative;
&::before {
content: '';
display: block;
position: absolute;
top: 0;
left: 0;
background: url("/src/assets/image/cat1.jpg") no-repeat center;
background-size: 100% 100%;
width: 200px;
height: 200px;
}
}
.one::before {
background: url("/src/assets/image/cat1.jpg") no-repeat center;
background-size: 100% 100% !important;
}
.two::before {
background: url("/src/assets/image/cat2.jpg") no-repeat center;
background-size: 100% 100% !important;
}
.three::before {
background: url("@/assets/image/cat3.jpg") no-repeat center;
background-size: 100% 100% !important;
}
</style>
三、对象映射(类名和图片)
javascript
<template>
<div ref="titleImage" :class="['a-bg', systemTitle.value]"></div>
</template>
<script setup>
import { ref, watch, onMounted } from "vue";
// 随机赋值
const possibleValues = ['one', 'two', 'three'];
const randomIndex = Math.floor(Math.random() * possibleValues.length);
const systemTitle = ref(possibleValues[randomIndex]);
const titleImage = ref(null);
// 对象映射
const classMapping = {
one: 'cat1',
two: 'cat2',
three: 'cat3'
};
const updateTitleImageClass = () => {
if (titleImage.value) {
console.log('Before:', titleImage.value.className); // 打印当前类名
// 清除所有可能的类名
for (const key in classMapping) {
titleImage.value.classList.remove(key);
}
// 添加新的类名
titleImage.value.classList.add(systemTitle.value);
console.log('After:', titleImage.value.className); // 打印替换后的类名
}
};
onMounted(() => {
console.log(systemTitle.value, 'systemTitle');
updateTitleImageClass();
});
// 监听 systemTitle 的变化
watch(systemTitle, updateTitleImageClass);
</script>
<style lang="scss" scoped>
#app {
width: 100%;
height: 100vh;
}
.a-bg {
width: 200px;
height: 200px;
background: linear-gradient(45deg, #fff, red);
position: relative;
&::before {
content: '';
display: block;
position: absolute;
top: 0;
left: 0;
background: url("/src/assets/image/cat1.jpg") no-repeat center;
background-size: 100% 100%;
width: 200px;
height: 200px;
}
}
.one::before {
background: url("/src/assets/image/cat1.jpg") no-repeat center;
background-size: 100% 100% !important;
}
.two::before {
background: url("/src/assets/image/cat2.jpg") no-repeat center;
background-size: 100% 100% !important;
}
.three::before {
background: url("@/assets/image/cat3.jpg") no-repeat center;
background-size: 100% 100% !important;
}
</style>
四、import.meta.glob
javascript
<template>
<div ref="titleImage" :class="['a-bg', systemTitle.value]"></div>
</template>
<script setup>
import { ref, watch, onMounted } from "vue";
// 随机赋值
const possibleValues = ['one', 'two', 'three'];
const randomIndex = Math.floor(Math.random() * possibleValues.length);
const systemTitle = ref(possibleValues[randomIndex]);
const titleImage = ref(null);
// 动态导入图片
const images = import.meta.glob('@/assets/image/*.jpg');
// 对象映射
const classMapping = {
one: 'cat1',
two: 'cat2',
three: 'cat3'
};
const updateTitleImageClass = async () => {
if (titleImage.value) {
console.log('Before:', titleImage.value.className); // 打印当前类名
// 清除所有可能的类名
for (const key in classMapping) {
titleImage.value.classList.remove(key);
}
// 添加新的类名
titleImage.value.classList.add(systemTitle.value);
console.log('After:', titleImage.value.className); // 打印替换后的类名
// 动态加载图片
const imagePath = `/src/assets/image/${classMapping[systemTitle.value]}.jpg`; // 根据系统标题获取图片路径
const imageModule = await images[imagePath](); // 使用动态导入
const imageUrl = imageModule.default; // 获取图片路径
titleImage.value.style.backgroundImage = `url(${imageUrl})`; // 设置背景图片
}
};
onMounted(() => {
console.log(systemTitle.value, 'systemTitle');
updateTitleImageClass();
});
// 监听 systemTitle 的变化
watch(systemTitle, updateTitleImageClass);
</script>
<style lang="scss" scoped>
#app {
width: 100%;
height: 100vh;
}
.a-bg {
width: 200px;
height: 200px;
background: linear-gradient(45deg, #fff, red);
position: relative;
background-size: 100% 100%;
}
.one::before,
.two::before,
.three::before {
content: '';
display: block;
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
</style>