效果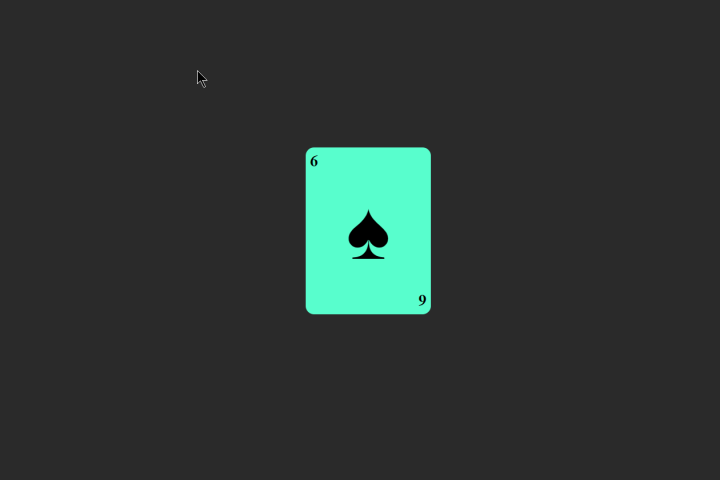
代码
html
<!DOCTYPE html>
<html>
<head>
<style>
body{
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #2b2b2b;
position: relative;
}
.card{
/*设置卡牌的外观*/
width: 150px;
height: 200px;
background-color: #00ffcc;
border-radius: 10px;
/*为卡牌中间的图案设置格式*/
font-size: 100px;
font-weight: bold;
display: flex;
justify-content: center;
align-items: center;
/*方便左上角和右下角的数字定位*/
position: absolute;
/*避免选择到文本*/
user-select: none;
}
/*设置卡牌两个对角的数字格式*/
.pos-TL{
position: absolute;
font-size: 20px;
top: 5px;
left: 5px;
}
.pos-BR{
position: absolute;
font-size: 20px;
bottom: 5px;
right: 5px;
transform: rotateZ(180deg);
}
</style>
</head>
<body>
<div id="card" class="card">♠️
<div class="card-num pos-TL">6</div>
<div class="card-num pos-BR">6</div>
</div>
<script>
let offsetX, offsetY, isDragging = false;
const card = document.getElementById("card");
card.addEventListener("mousedown", (e) => {
isDragging = true;
const cardrect = card.getBoundingClientRect(); // 每次拖动开始时更新位置
offsetX = e.clientX - cardrect.left;
offsetY = e.clientY - cardrect.top;
});
document.addEventListener("mousemove", (e) => {
if (isDragging) {
card.style.left = `${e.clientX - offsetX}px`;
card.style.top = `${e.clientY - offsetY}px`;
}
});
document.addEventListener("mouseup", () => {
isDragging = false;
});
</script>
</body>
</html>
总结
- const只能申明单个变量,否则报错
html
<script>
const offsetX,offsetY = false;//❌
let offsetX,offsetY = false //✅
</script>
- 不要实时获取的值赋给其他变量
html
cardrect = card.getBoundingClientRect();\\之前写了这句,只会获得点击时候的鼠标位置,而无法获取到拖拽过程中的鼠标位置
- 忘记在
<div class = "card">
里添加id="card"
了 - 属性为
absolute
的元素的定位不是取决于离他最近的含有relative
的父元素,而是取决于离他最近的还有position
的父元素。所以我们的代码里既能让card
相对body
移动,又能让cardnum相对card进行定位。