1、效果如下图,点击图片可更新验证码(其实图片就是一个Button的背景图)。
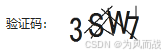
2、主要使通过用户控件创建,UCVerificationCode.xaml代码如下。
XML
<UserControl x:Class="UC.UCVerificationCode"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:RegisterWPF.UC"
xmlns:hc="https://handyorg.github.io/handycontrol"
mc:Ignorable="d"
Width="Auto"
Height="Auto" Loaded="UCVerificationCode_OnLoaded">
<Grid>
<Button x:Name="btnVerificationCode" Width="100" Height="40" BorderThickness="0" Click="BtnVerificationCode_OnClick"/>
</Grid>
</UserControl>
3、UCVerificationCode.xaml.cs代码如下。
cs
/// <summary>
/// UCVerificationCode.xaml 的交互逻辑
/// </summary>
public partial class UCVerificationCode : UserControl
{
public string VerificationCode { get; set; }
public UCVerificationCode()
{
InitializeComponent();
}
/// <summary>
/// 用户控件加载完成
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void UCVerificationCode_OnLoaded(object sender, RoutedEventArgs e)
{
GetVerificationCode();
}
/// <summary>
/// 获取验证码
/// </summary>
public void GetVerificationCode()
{
using (MemoryStream outStream = new MemoryStream())
{
Bitmap bitmap = CreateVerificationCode(out string code);
bitmap.Save(outStream, ImageFormat.Bmp);
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.BeginInit();
bitmapImage.StreamSource = new MemoryStream(outStream.ToArray());
bitmapImage.EndInit();
ImageBrush imgBrush = new ImageBrush(bitmapImage);
btnVerificationCode.Background = imgBrush;
VerificationCode = code;
Debug.WriteLine(code);
Debug.WriteLine(VerificationCode);
}
}
/// <summary>
/// 生成验证码图片
/// </summary>
/// <param name="code"></param>
/// <returns></returns>
public static Bitmap CreateVerificationCode(out string code)
{
//建立Bitmap对象,绘图
Bitmap bitmap = new Bitmap(200, 60);
Graphics graph = Graphics.FromImage(bitmap);
graph.FillRectangle(new SolidBrush(System.Drawing.Color.White), 0, 0, 200, 60);
Font font = new Font(System.Drawing.FontFamily.GenericSansSerif, 48,System.Drawing.FontStyle.Bold, GraphicsUnit.Pixel);
Random r = new Random();
string letters = "ABCDEFGHIJKLMNPQRSTUVWXYZ0123456789";
StringBuilder sb = new StringBuilder();
//添加随机的五个字母
for (int x = 0; x < 4; x++)
{
string letter = letters.Substring(r.Next(0, letters.Length - 1), 1);
sb.Append(letter);
graph.DrawString(letter, font, new SolidBrush(Color.Black), x * 38, r.Next(0, 15));
}
code = sb.ToString();
//混淆背景
Pen linePen = new Pen(new SolidBrush(Color.Black), 2);
for (int x = 0; x < 6; x++)
graph.DrawLine(linePen, new Point(r.Next(0, 199), r.Next(0, 59)), new Point(r.Next(0, 199), r.Next(0, 59)));
return bitmap;
}
/// <summary>
/// 刷新验证码
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void BtnVerificationCode_OnClick(object sender, RoutedEventArgs e)
{
GetVerificationCode();
}
}
4、引用时。
XML
<localuc:UCVerificationCode VerticalAlignment="Center" HorizontalContentAlignment="Center" Margin="0" Padding="0" BorderThickness="0"/>