1.找到utils下创建fifilter.js
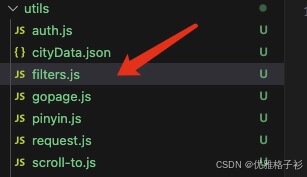
一些常用的过滤方法
javascript
export const filters = {
//url解码
urlCode: value => {
if (!value) return ''
let v = decodeURIComponent(value)
let bigIndex = v.lastIndexOf('/')
let endIndex = v.lastIndexOf('.')
let url = v.substring(bigIndex + 1, endIndex) + v.substring(endIndex)
return url
},
dateFormat: value => {
if (!value) return ''
value = value * 1000
//new Date 在 ios safari浏览器有兼容性问题处理如下:ios不支持2027-2-22 16:23,需要改为2027/2/22 16:23
// ? 兼容 ios safari : 兼容其他浏览器
let $this = new Date(value) == 'Invalid Date' ? new Date(date.replace(/-/g, "/")) : new Date(value)
var timestamp = parseInt(Date.parse($this)) / 1000 //- 8 * 60 * 60; //(本地时间)东八区减去8小时;
// console.log(timestamp)
function zeroize(num) {
return (String(num).length == 1 ? '0' : '') + num;
}
var curTimestamp = parseInt(new Date().getTime() / 1000); //当前时间戳
// console.log(curTimestamp)
var timestampDiff = curTimestamp - timestamp; // 参数时间戳与当前时间戳相差秒数
var curDate = new Date(curTimestamp * 1000); // 当前时间日期对象
var tmDate = new Date(timestamp * 1000); // 参数时间戳转换成的日期对象
var Y = tmDate.getFullYear(),
m = tmDate.getMonth() + 1,
d = tmDate.getDate();
var H = tmDate.getHours(),
i = tmDate.getMinutes(),
s = tmDate.getSeconds();
if (timestampDiff < 60) { // 一分钟以内
return "刚刚";
} else if (timestampDiff < 3600) { // 一小时前之内
return Math.floor(timestampDiff / 60) + "分钟前";
} else if (curDate.getFullYear() == Y && curDate.getMonth() + 1 == m && curDate.getDate() == d) {
return '今天 ' + zeroize(H) + ':' + zeroize(i)
} else {
var newDate = new Date((curTimestamp - 86400) * 1000); // 参数中的时间戳加一天转换成的日期对象
if (newDate.getFullYear() == Y && newDate.getMonth() + 1 == m && newDate.getDate() == d) {
return '昨天 ' + zeroize(H) + ':' + zeroize(i)
} else if (curDate.getFullYear() == Y) {
return zeroize(m) + '月' + zeroize(d) + '日';
} else {
return Y + '年' + zeroize(m) + '月' + zeroize(d) + '日';
}
}
},
// 时间过滤器
formatDate: value => {
if (value == undefined) {
return;
}
let date = new Date(value * 1000); //时间戳为10位需*1000,时间戳为13位的话不需乘1000
let Y = date.getFullYear() + '年';
let M = (date.getMonth() + 1 < 10 ? '0' + (date.getMonth() + 1) : date.getMonth() + 1) + '月';
let D = (date.getDate() < 10 ? '0' + date.getDate() : date.getDate()) + '日';
let h = (date.getHours() < 10 ? '0' + date.getHours() : date.getHours()) + ':';
let m = (date.getMinutes() < 10 ? '0' + date.getMinutes() : date.getMinutes()) + ':';
let s = (date.getSeconds() < 10 ? '0' + date.getSeconds() : date.getSeconds());
return Y + M + D + h + m + s;
},
//微信时间
wxleftTime: value => {
if (value == undefined) {
return;
}
const date = new Date(value * 1000);
const year = date.getFullYear();
const month = (date.getMonth() + 1).toString().padStart(2, '0');
const day = date.getDate().toString().padStart(2, '0');
const hours = date.getHours().toString().padStart(2, '0');
const minutes = date.getMinutes().toString().padStart(2, '0');
if (new Date(Number(value) * 1000).toDateString() === new Date().toDateString()) {
return `${hours}:${minutes}`;
} else {
return `${year}/${month}/${day}`;
}
},
//千分位
numberToCurrencyNo: value => {
if (!value) return 0.00
// 获取整数部分
const intPart = Math.trunc(value)
// 整数部分处理,增加,
const intPartFormat = intPart.toString().replace(/(\d)(?=(?:\d{3})+$)/g, '$1,')
// 预定义小数部分
let floatPart = ''
// 将数值截取为小数部分和整数部分
const valueArray = value.toString().split('.')
if (valueArray.length === 2) { // 有小数部分
floatPart = valueArray[1].toString() // 取得小数部分
return intPartFormat + '.' + floatPart
}
return intPartFormat + floatPart
}
}
2.在main.js 注册
javascript
import { filters } from './utils/filters'
// 定义全局自定义过滤器
Object.keys(filters).forEach(key => {
Vue.filter(key, filters[key])
})
3.页面使用
javascript
{{ item.createtime | dateFormat }}