外卖开发(九)------Excel数据报表
一、ApachePOI
Apache POl是一个处理Miscrosoft Office各种文件格式的开源项目。简单来说就是,我们可以使用POI在Java程序中对Miscrosoft Office各种文件进行读写操作。
一般情况下,POI都是用于操作 Excel文件。
maven坐标
bash
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.16</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.16</version>
</dependency>
二、入门案例
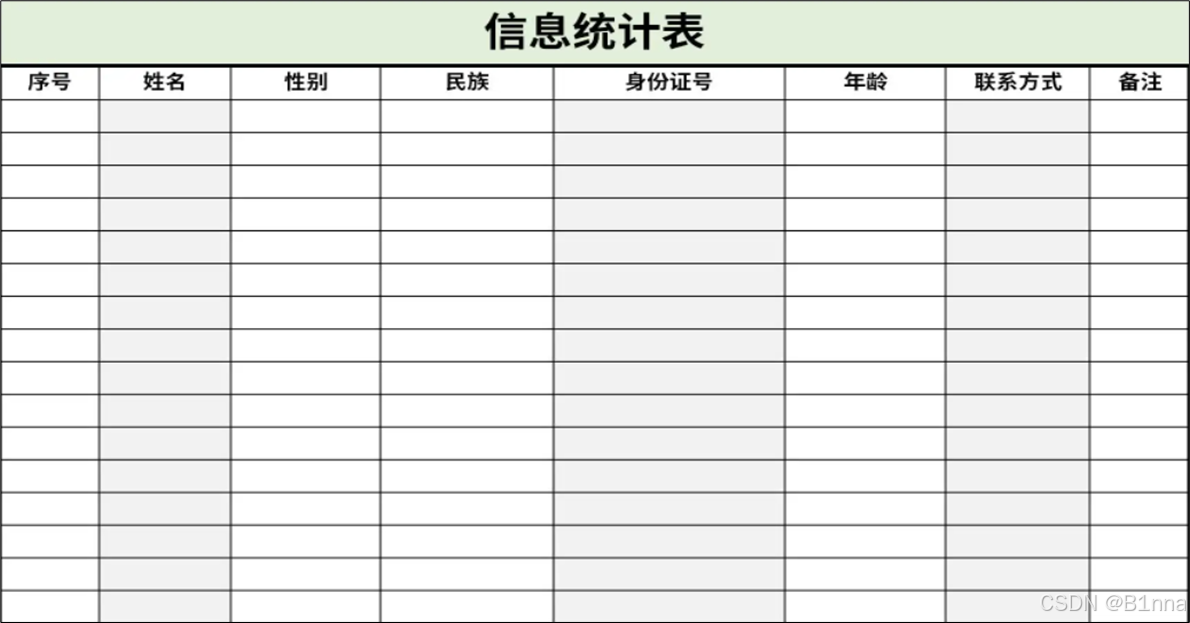
将数据写入Excel
java
//在内存中创建一个Excel文件对象
XSSFWorkbook excel = new XSSFWorkbook();
//创建Sheet页
XSSFSheet sheet = excel.createSheet("itcast");
//在Sheet页中创建行,0表示第1行
XSSFRow row1 = sheet.createRow(0);
//创建单元格并在单元格中设置值,单元格编号也是从0开始,1表示第2个单元格
row1.createCell(1).setCellValue("姓名");
row1.createCell(2).setCellValue("城市");
XSSFRow row2 = sheet.createRow(1);
row2.createCell(1).setCellValue("张三");
row2.createCell(2).setCellValue("北京");
XSSFRow row3 = sheet.createRow(2);
row3.createCell(1).setCellValue("李四");
row3.createCell(2).setCellValue("上海");
FileOutputStream out = new FileOutputStream(new File("D:\\itcast.xlsx"));
//通过输出流将内存中的Excel文件写入到磁盘上
excel.write(out);
//关闭资源out.flush();
out.close();
excel.close();
读取Excel中的数据:
java
FileInputStream in = new FileInputStream(new File("D:\\itcast.xlsx"));
//通过输入流读取指定的Excel文件
XSSFWorkbook excel = new XSSFWorkbook(in);
//获取Excel文件的第1个Sheet页
XSSFSheet sheet = excel.getSheetAt(0);
//获取Sheet页中的最后一行的行号
int lastRowNum = sheet.getLastRowNum();
for (int i = 0; i <= lastRowNum; i++) {
//获取Sheet页中的行
XSSFRow titleRow = sheet.getRow(i);
//获取行的第2个单元格
XSSFCell cell1 = titleRow.getCell(1);
//获取单元格中的文本内容
String cellValue1 = cell1.getStringCellValue();
//获取行的第3个单元格
XSSFCell cell2 = titleRow.getCell(2);
//获取单元格中的文本内容
String cellValue2 = cell2.getStringCellValue();
System.out.println(cellValue1 + " " +cellValue2);
}
//关闭资源
in.close();
excel.close();
运行效果:
三、导出运营数据报表
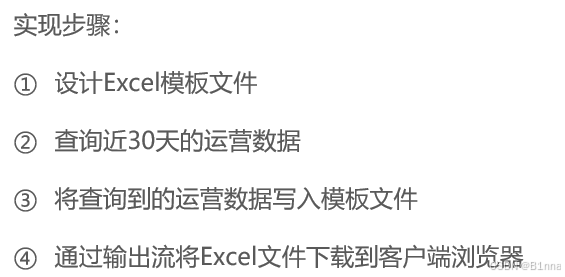
1、ReportController
java
/**
* 导出数据报表
* @param response
*/
@GetMapping("/export")
@ApiOperation("到处数据报表")
public void export(HttpServletResponse response){ //传递response,以便使用输出流
workSpaceService.exportData(response);
}
2、ReportService
java
/**
* 导出运营数据报表
* @param response
*/
@Override
public void exportData(HttpServletResponse response){
//查询数据库,获取数据--查询最近30天
LocalDate dateBegin = LocalDate.now().minusDays(30);
LocalDate dateEnd = LocalDate.now().minusDays(1);
LocalDateTime begin = LocalDateTime.of(dateBegin, LocalTime.MIN);
LocalDateTime end = LocalDateTime.of(dateEnd, LocalTime.MAX);
BusinessDataVO businessDataVO = getbusinessDataMoreDay(begin,end);
//通过poi写入excel
//获得输入流
InputStream in = this.getClass().getClassLoader().getResourceAsStream("template/template.xlsx");
try {
XSSFWorkbook excel = new XSSFWorkbook(in);
//填充数据
XSSFSheet sheet = excel.getSheet("Sheet1");
sheet.getRow(1).getCell(1).setCellValue("时间 "+ dateBegin+" 至 "+dateEnd);
sheet.getRow(3).getCell(2).setCellValue(businessDataVO.getTurnover());
sheet.getRow(3).getCell(4).setCellValue(businessDataVO.getOrderCompletionRate());
sheet.getRow(3).getCell(6).setCellValue(businessDataVO.getNewUsers());
sheet.getRow(4).getCell(2).setCellValue(businessDataVO.getValidOrderCount());
sheet.getRow(4).getCell(4).setCellValue(businessDataVO.getUnitPrice());
//填充明细数据(30天,每天的明细)
List<LocalDate> dateList = new ArrayList<>();//30天每天的日期,用于循环查询数据库
dateList.add(dateBegin);
while(!dateBegin.isEqual(dateEnd)){
dateBegin = dateBegin.plusDays(1);
dateList.add(dateBegin);
}
//运营数据集合,每一天运营数据都是一个集合项
List<BusinessDataVO> businessDataList = new ArrayList<>();
for (LocalDate date : dateList){
LocalDateTime beginTime = LocalDateTime.of(date, LocalTime.MIN);
LocalDateTime endTime = LocalDateTime.of(date, LocalTime.MAX);
BusinessDataVO businessData = getbusinessDataMoreDay(beginTime, endTime);
businessDataList.add(businessData);
}
//POI装入excel
for(int i =7; i< (businessDataList.size()+7); i++){
XSSFRow row = sheet.getRow(i);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
String formattedDate = dateList.get(i-7).format(formatter);
row.getCell(1).setCellValue(formattedDate);
row.getCell(2).setCellValue(businessDataList.get(i-7).getTurnover());
row.getCell(3).setCellValue(businessDataList.get(i-7).getValidOrderCount());
row.getCell(4).setCellValue(businessDataList.get(i-7).getOrderCompletionRate());
row.getCell(5).setCellValue(businessDataList.get(i-7).getUnitPrice());
row.getCell(6).setCellValue(businessDataList.get(i-7).getNewUsers());
}
//通过输出流,把excel下载到浏览器
ServletOutputStream out = response.getOutputStream();
excel.write(out);
//关闭资源
out.close();
excel.close();
} catch (IOException e) {
e.printStackTrace();
}
}

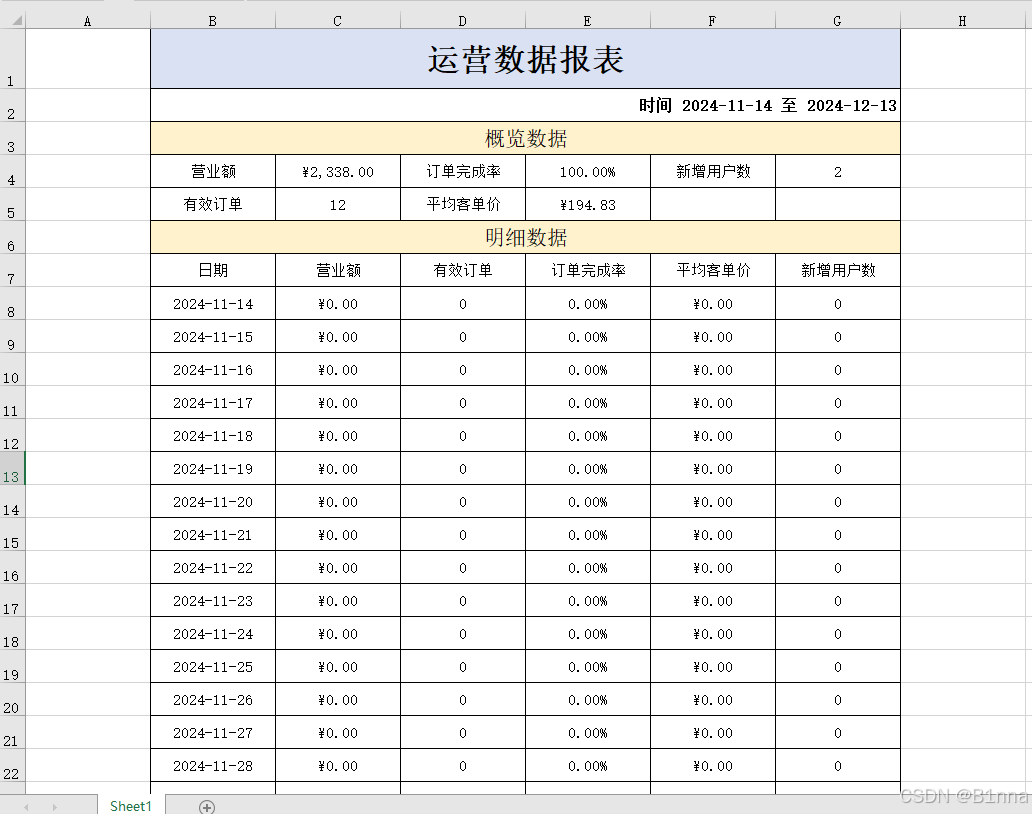