文章目录
- 前言
- [1. string类对象的常用构造](#1. string类对象的常用构造)
- [2. string类对象的容量操作](#2. string类对象的容量操作)
- [3. string类对象的访问及遍历操作](#3. string类对象的访问及遍历操作)
- [4. string类对象的修改操作](#4. string类对象的修改操作)
- [5. string类非成员函数](#5. string类非成员函数)
前言
本篇文章所有的内容均出自cplusplus.com。
1. string类对象的常用构造
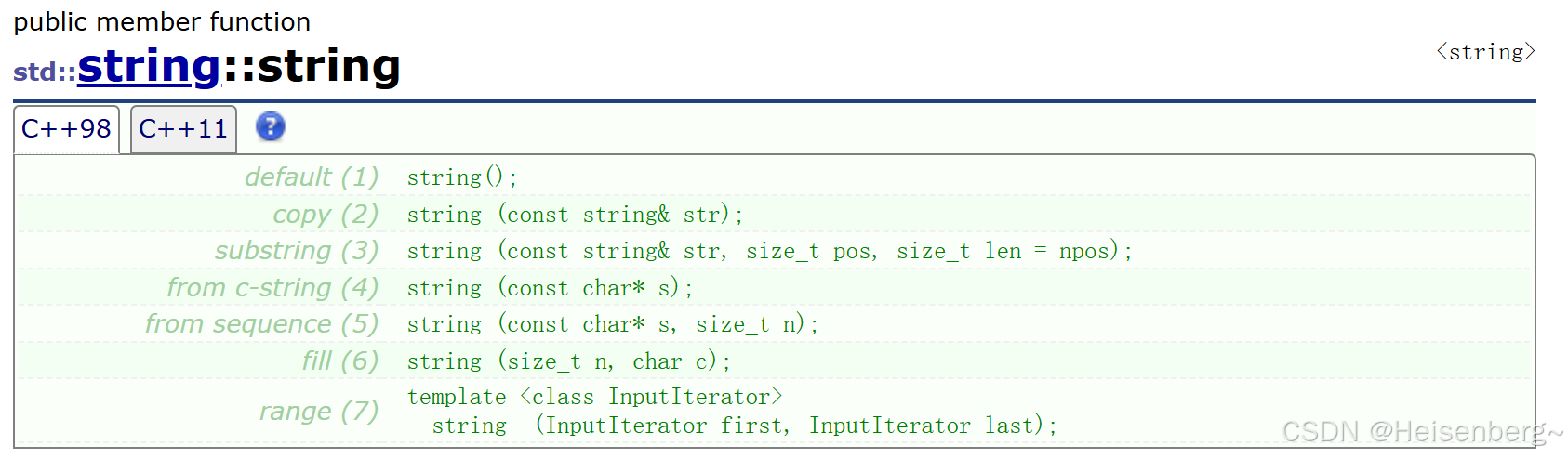
cpp
//string的构造
int main()
{
//string实例化的方式
string s1;//第一种:构造函数实例化一个为空的字符串
string s2("12345678");//第二种:实例化一个"12345678"的字符串
string s3("12345678",4);//第三种:实例化前4个数,也就是1234
string s4(10, 'x');//第四种:实例化10个x
string s5(s2,3,3);//第五种:拷贝字符串s2,从s2的第3个数开始拷贝,拷贝三个数
string s6(s2,3);//第六种:拷贝字符串s2,从s2的第3个数开始全部拷贝
string s7(s2,4,20);//第七种:拷贝字符串s2,从s2的第4个数开始拷贝20个数,不足20个数那就有多少个数拷贝多少
cout << s1 << endl;
cout << s2 << endl;
cout << s3 << endl;
cout << s4 << endl;
cout << s5 << endl;
cout << s6 << endl;
cout << s7 << endl;
cout << endl;
//编译器自带的运算符重载,可以直接像数组一样使用下标改变字符串的值
s2[0] = 'x';
s2[7] = 'x';
cout << s2 << endl;
//s2.size()是编译器自带的,计算字符串大小的函数
for (size_t i = 0; i < s2.size(); i++)
{
s2[i]++;
}
cout << s2 << endl;
return 0;
}
2. string类对象的容量操作
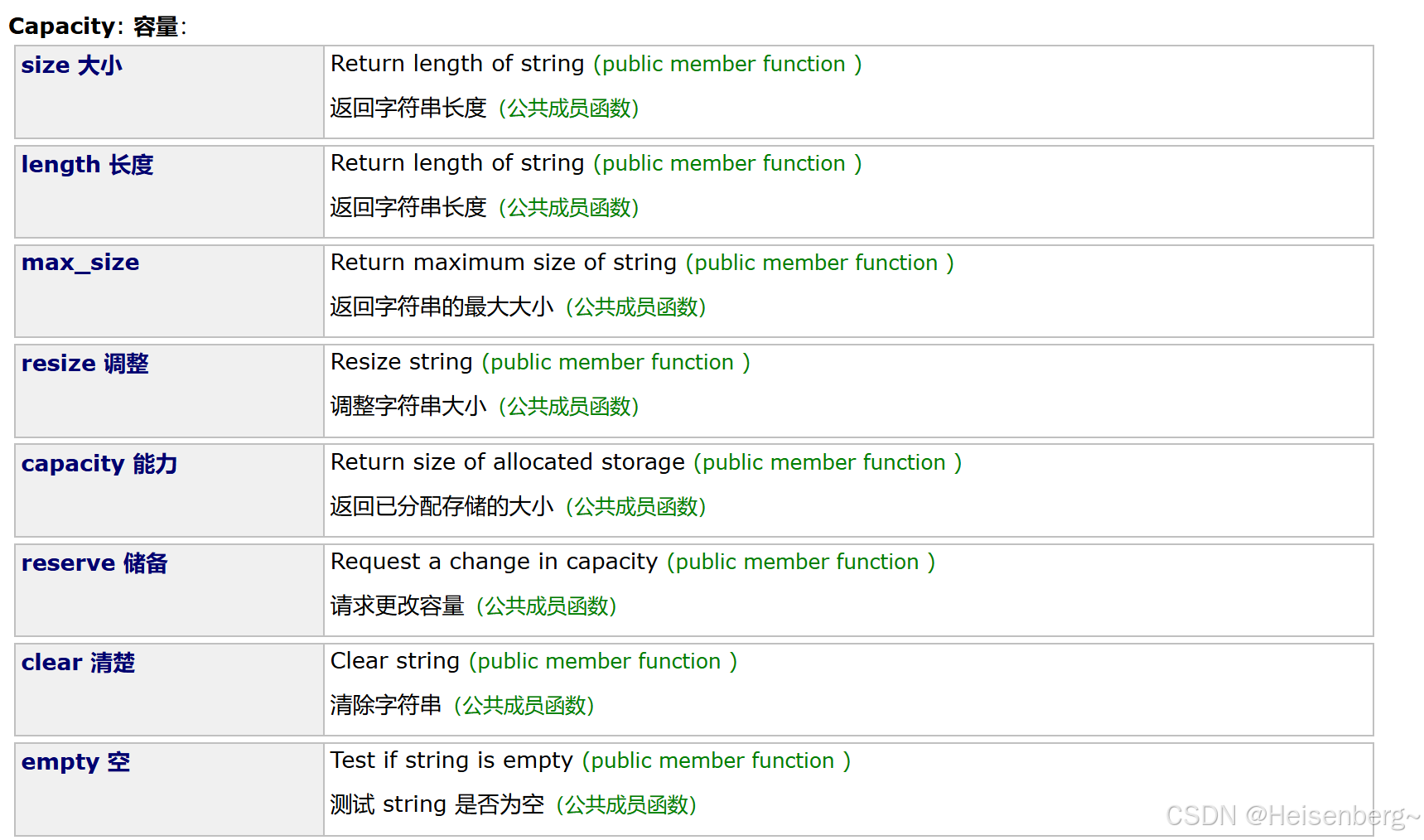
cpp
//string的容量操作
int main()
{
string s1("hello world!");
cout << s1.size() << endl;//12 size表示的是字符串的长度
cout << s1.length() << endl;//12 length表示的也是字符串的长度
cout << s1.capacity() << endl;//15 capacity表示的是返回已分配存储的大小
cout << s1.max_size() << endl;//9223372036854775807 max_size返回字符串的最大大小
cout << "分割线" << endl;
//resize 改变size的大小,但是不会改变capacity
//使用resize会发生三种情况
//1.当指定的size小于原本的size空间。删除多余的size
cout << s1 << endl;//hello world!
cout << s1.size() << endl;//12
cout << s1.capacity() << endl;
s1.resize(10);
cout << s1 << endl;//hello worl
cout << s1.size() << endl;//10
cout << s1.capacity() << endl;
//2.当指定的size大于原本的size空间,但是小于容量。往剩余的空间里插入
cout << s1 << endl;//hello world!
cout << s1.size() << endl;//12
s1.resize(20, 'x');//这里可以指定插入的x是什么,也可以不指定。编译器默认插入'\0'
cout << s1 << endl;//hello worlxxxxxxxxxx
cout << s1.size() << endl;//20
//3.当指定的size大于容量。先扩容再插入
cout << s1 << endl;//hello worlxxxxxxxxxx
cout << s1.size() << endl;//20
cout << s1.capacity() << endl;//47
s1.resize(60, 'x');
cout << s1 << endl;//hello worlxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx
cout << s1.size() << endl;//60
cout << s1.capacity() << endl;//70
cout << "分割线" << endl;
// reverse 反向 翻转
// reserve 预留空间
cout << s1.capacity() << endl;//15
s1.reserve(40);//reserve改变预留的空间大小。但是不会真的只按照要求的预留。而是在满足一定的规则下,达到预留的空间
cout << s1.capacity() << endl;//207 上面要求预留的200,但是这里预留的空间是207
cout << "分割线" << endl;
s1.clear();//清除字符串的内容,同时将字符串的size变为0,容量不变
cout << s1 << endl;
cout << s1.size() << endl;
cout << s1.capacity() << endl;
cout << "分割线" << endl;
if (s1.empty())//判空
{
cout << "s1是空字符串" << endl;
}
else
cout << "s1不是空字符串" << endl;
return 0;
}
3. string类对象的访问及遍历操作
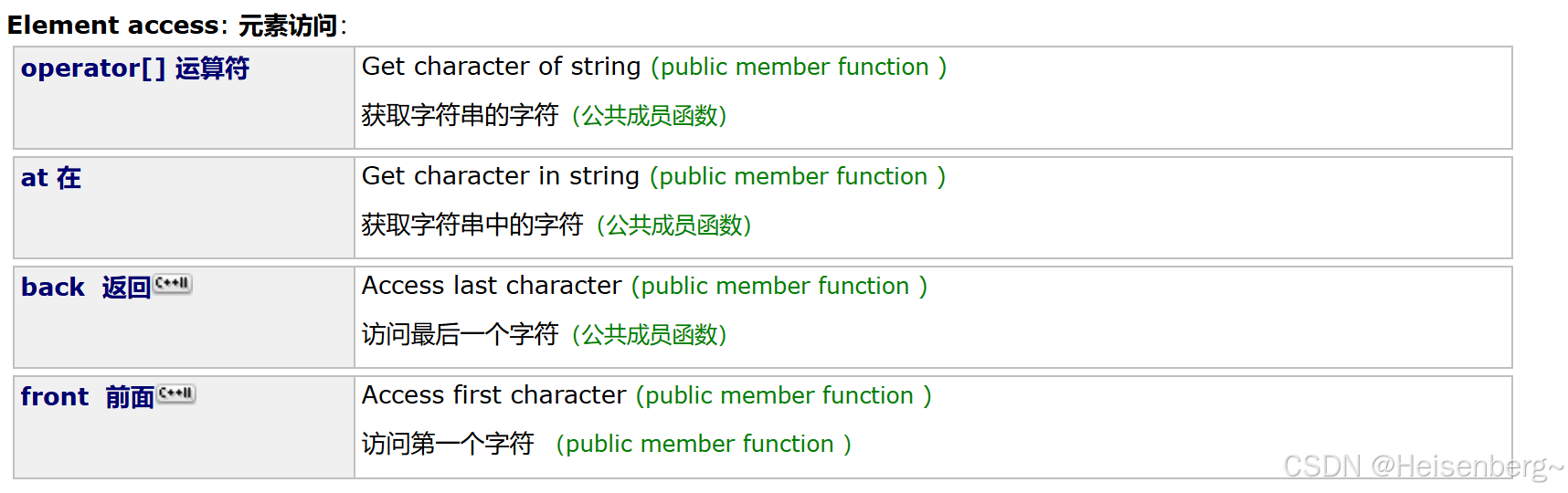
cpp
// string的访问操作
int main()
{
//[]下标来访问字符串
string s1("hello worlderrrr");
for (size_t i = 0;i < s1.size();i++)
{
cout << s1[i] << " ";
}
cout << endl;
cout << "分割线" << endl;
//捕获异常。at在找到对应位置的数据时,会返回数据。但是位置不存在时,就会报异常
try
{
cout << s1.at(2);
}
catch (const exception& e)
{
cout << e.what() << endl;
}
return 0;
}
4. string类对象的修改操作
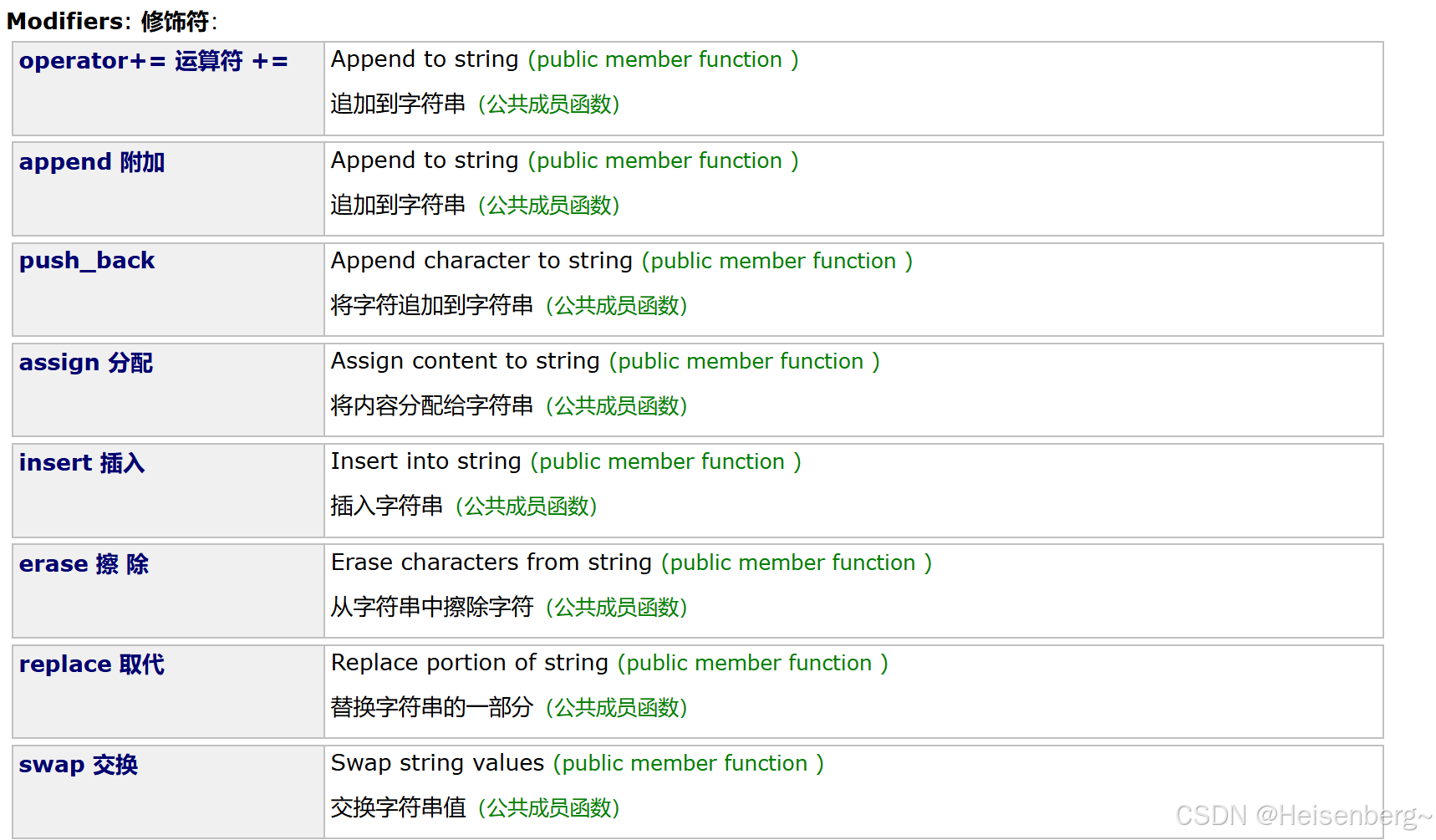
cpp
//string的修改操作
int main()
{
// +=运算符重载、append(将 字符串1 尾插到 字符串2 ) 和 push_back(将 字符 尾插到 字符串 )
string s1("hello");
s1.push_back(',');
s1.push_back('w');
cout << s1 << endl;
s1.append("orld");
cout << s1 << endl;
s1.append(10, '!');
cout << s1 << endl;
string s2("hello bit hello world");
s1.append(s2.begin()+6, s2.end());//从字符串s2的第六个位置开始,全部尾插到s1
cout << s1 << endl;
s1.append(s2.begin() + 2, s2.end() - 3);
cout << s1 << endl;
string s3("hello");
s3 += ',';// += 运算符重载。比上面两个方式方便
s3 += "world";
cout << s3 << endl;
cout << "分割线" << endl;
//assign
string str;
string str_copy("hello world");
cout << str << endl;
str.assign(str_copy);
cout << str << endl;
cout << "分割线" << endl;
// insert。头插,在字符串的头部插入字符
// 不一定非得要头插,也可以指定位置
string d1("hello world");
cout << "头插前:" << d1 << endl;
d1.insert(0,"xxx");//头插
cout << "头插后:" << d1 << endl;
d1.insert(5, "sss");//从位置为5的下标开始插入
cout << "中间插入:" << d1 << endl;
// erase。从第N个字符开始,删除N个字符。
// 不推荐频繁使用,频繁使用的效率低
d1.erase(5, 3);
cout << "erase删除第5个位置的3个字符:" << d1 << endl;
d1.erase(0, 3);
cout << "erase删除头部的三个字符:" << d1 << endl;
d1.erase(1);
cout << "erase删除指定位置之后的全部字符:" << d1 << endl;
cout << "分割线" << endl;
// replace。从第 N 到 N+M 字符,替换字符串
// 只有在 N ~ N+M之间的长度等于字符串时,才推荐使用。也就是字符串平替的情况下才推荐
string d2("hello world hello bit");
cout << d2 << endl;
//size_t i = d2.find(' ');//find会找到指定字符在字符串中的位置
//while (i != string::npos)//npos是编译器定义的,字符串中最大的值
//{
// d2.replace(i, 1, "%%");//把指定的字符修改。这里是把" " 修改成"%%"
// i = d2.find(' ', i+2);//继续寻找。同时由于一次修改了两个字符%%。所以第二次寻找可以从i+2的位置开始找
//}
//cout << d2 << endl;
string d3;
for (auto ch : d2)//也可以使用范围for的方式来平替字符串中的字符
{
if (ch != ' ')
d3 += ch;
else
d3 += "%%";
}
cout << d3 << endl;
return 0;
}
5. string类非成员函数
cpp
//非成员函数
int main()
{
//swap
string s1("hello");
string s2("world");
cout << "s1:" << s1 << endl;
cout << "s2:" << s2 << endl;
swap(s1, s2);
cout << "s1:" << s1 << endl;
cout << "s2:" << s2 << endl;
//getline
//由用户输入s3的字符串内容。
//编译器自带的>>流提取重载符。在遇到" "之后自动将两个字符串分开。而有时候又不需要将空格前后的两个字符串区分
//就能用到getline。getline自动将 换行 定义为两个字符串的区分方式。也可以自己定义
string s3;
getline(cin, s3);//将换行定义为结束语句
cout << s3 << endl;
getline(cin, s3, '#');//将#定义为结束语句
cout << s3 << endl;
return 0;
}