函数指针的主要作用,就是用来选择不同的调度函数,来满足特殊需求。它的优点,使程序设计更加灵活。缺点:初学者很难理解函数指针,从而引起程序的可读性不高。
1、使用函数指针选择调度函数
#include "stm32f10x.h"
#include "USART1.h"
#include "stdio.h" //getchar(),putchar(),scanf(),printf(),puts(),gets(),sprintf()
void Function1(void)
{
printf("Function1 work\r\n");
}
void Function2(void)
{
printf("Function2 work\r\n");
}
void Function3(void)
{
printf("Function3 work\r\n");
}
void (*FunctionPointer)(void);
//定义一个"函数指针",用来调用传入"void参数"的函数,该函数的返回值为void
int main(void)
{
int cnt;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_4);//设置系统中断优先级分组4
USART1_Serial_Interface_Enable(115200);
printf("\r\nCPU reset\r\n");
cnt=0;
while(1)
{
cnt++;
if(cnt>3) cnt=1;
if(cnt==1) FunctionPointer=Function1;
//给函数指针赋值,则该函数指针会指向Function1()
if(cnt==2) FunctionPointer=Function2;
//给函数指针赋值,则该函数指针会指向Function2()
if(cnt==3) FunctionPointer=Function3;
//给函数指针赋值,则该函数指针会指向Function3()
FunctionPointer();
}
}
测试结果:
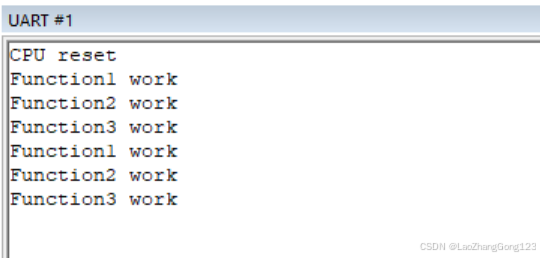
2、使用函数指针数组选择调度函数
#include "stm32f10x.h"
#include "USART1.h"
#include "stdio.h" //getchar(),putchar(),scanf(),printf(),puts(),gets(),sprintf()
void Function1(void)
{
printf("Function1 work\r\n");
}
void Function2(void)
{
printf("Function2 work\r\n");
}
void Function3(void)
{
printf("Function3 work\r\n");
}
void (*FunctionPointerArray[])(void) = { Function1, Function2,Function3 };
//定义一个"函数指针数组",用来调用传入"void参数"的函数,该函数的返回值为void
int main(void)
{
int cnt;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_4);//设置系统中断优先级分组4
USART1_Serial_Interface_Enable(115200);
printf("\r\nCPU reset\r\n");
cnt=0;
while(1)
{
FunctionPointerArray[cnt]();
cnt++;
if(cnt>2) cnt=0;
}
}
测试结果:
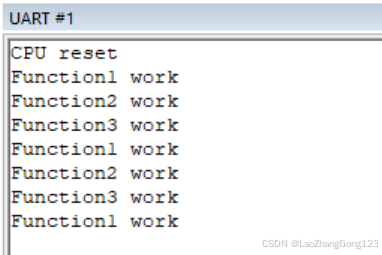
3、将函数指针用作函数的传递参数选择调度函数
#include "stm32f10x.h"
#include "USART1.h"
#include "stdio.h" //getchar(),putchar(),scanf(),printf(),puts(),gets(),sprintf()
void Function(int value)
{
printf("value=%u\r\n",value);
}
//FunctionPointer是"函数指针",用来调用传入"void参数"的函数,该函数的返回值为void
//将"函数指针"用作传递参数,用来调用不同的调度函数
void callbackFunction(int value, void (*FunctionPointer)(int))
{
FunctionPointer(value);
}
int main(void)
{
int cnt;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_4);//设置系统中断优先级分组4
USART1_Serial_Interface_Enable(115200);
printf("\r\nCPU reset\r\n");
cnt=0;
while(1)
{
callbackFunction(cnt,Function);
cnt++;
if(cnt>2) cnt=0;
}
}
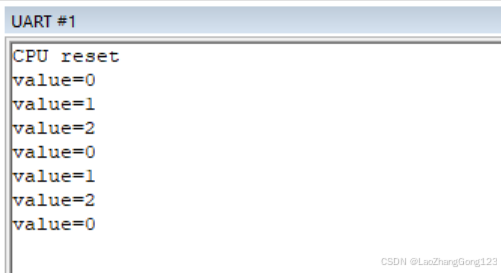
4、函数指针和指针函数的区别
函数指针,首先它是一个指针,不是函数;
指针函数首先是一个函数,只是它的返回值是一个指针。
函数指针声明格式:类型标识符 (*函数名) (参数)
void (*FunctionPointer)(void);
//定义一个"函数指针",用来调用传入"void参数"的函数,该函数的返回值为void
指针函数的声明格式为:类型标识符 *函数名(参数表);
char array[]="123456\r\n";
char * PointerFunction(char *buf) //这是指针函数
{
buf++;
return(buf);
}
void Test_PointerFunction(void)
{
char *p;
p=PointerFunction(array);
printf("*p=%s",p);
}
#include "stm32f10x.h"
#include "USART1.h"
#include "stdio.h" //getchar(),putchar(),scanf(),printf(),puts(),gets(),sprintf()
/*
函数指针,首先它是一个指针,不是函数;
指针函数首先是一个函数,只是它的返回值是一个指针。
函数指针声明格式:类型标识符 (*函数名) (参数)
指针函数的声明格式为:类型标识符 *函数名(参数表);
*/
void Function(int value)
{
printf("value=%u\r\n",value);
}
char array[]="123456\r\n";
char * PointerFunction(char *buf)
{
buf++;
return(buf);
}
void Test_PointerFunction(void)
{
char *p;
p=PointerFunction(array);
printf("*p=%s",p);
}
//FunctionPointer是"函数指针",用来调用传入"void参数"的函数,该函数的返回值为void
//将"函数指针"用作传递参数,用来调用不同的调度函数
void callbackFunction(int value, void (*FunctionPointer)(int))
{
FunctionPointer(value);
}
int main(void)
{
int cnt;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_4);//设置系统中断优先级分组4
USART1_Serial_Interface_Enable(115200);
printf("\r\nCPU reset\r\n");
Test_PointerFunction();
cnt=0;
while(1)
{
callbackFunction(cnt,Function);
cnt++;
if(cnt>2) cnt=0;
}
}
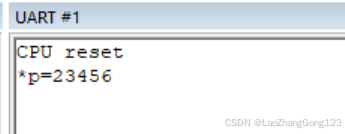