一、使用二分查找法
每次查找时,将查找范围分成两半,并判断目标值位于哪一半,从而逐步缩小查找范围。
循环查找
- 计算中间位置
mid = Math.floor((low + high) / 2)
。- 比较目标值
target
和中间位置的元素arr[mid]
:
- 如果
target == arr[mid]
,则找到目标值,返回mid
。- 如果
target < arr[mid]
,则目标值在左半部分,更新high = mid - 1
。- 如果
target > arr[mid]
,则目标值在右半部分,更新low = mid + 1。
终止条件:
- 当
low > high
时,表示查找范围为空,目标值不存在于数组中,返回-1
或其他表示未找到的标志。
javascript
function binarySearch(arr, target) {
let low = 0;
let high = arr.length - 1;
while (low <= high) {
const mid = Math.floor((low + high) / 2);
if (arr[mid] === target) {
return mid; // 找到目标值,返回索引
} else if (arr[mid] < target) {
low = mid + 1; // 目标值在右半部分
} else {
high = mid - 1; // 目标值在左半部分
}
}
return -1; // 未找到目标值
}
// 示例使用
const arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const target = 7;
console.log(binarySearch(arr, target)); // 输出: 6
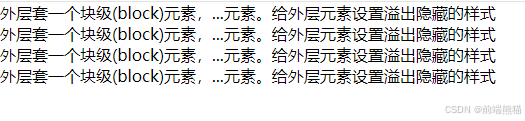
javascript
<template>
<div class="ellipsis-container" ref="ellipsisContainer">
{{ startText }}...{{ endText }}
</div>
</template>
<script>
import { defineComponent, ref, onMounted, watch } from 'vue';
export default defineComponent({
props: {
endLen: {
type: Number,
default: 10,
},
text: {
type: String,
default: '外层套一个块级(block)元素,内部是一个行内(inline)元素。给外层元素设置溢出隐藏的样式属性ne元素。给外层元素设置溢出隐藏的样式外层套一个块级(block)元素,内部是一个行内(inline)元素。给外层元素设置溢出隐藏的样式属性ne元素。给外层元素设置溢出隐藏的样式外层套一个块级(block)元素,内部是一个行内(inline)元素。给外层元素设置溢出隐藏的样式属性ne元素。给外层元素设置溢出隐藏的样式外层套一个块级(block)元素,内部是一个行内(inline)元素。给外层元素设置溢出隐藏的样式属性ne元素。给外层元素设置溢出隐藏的样式',
},
startLen: {
type: Number,
default: null,
},
},
setup (props) {
const ellipsisContainer = ref(null);
const str = ref(props.text);
const startText = ref('');
const endText = ref('');
const updateText = () => {
if (!ellipsisContainer.value) return;
let containerWidth = ellipsisContainer.value.clientWidth;
let fullText = str.value;
let startTextLength = 0;
let endTextLength = 0;
// 使用二分查找法找到合适的截断位置
let low = 0;
let high = fullText.length;
while (low <= high) {
let mid = Math.floor((low + high) / 2);
let tempStartText = fullText.substring(0, mid);
let tempEndText = fullText.substring(fullText.length - mid);
let combinedText = `${tempStartText}...${tempEndText}`;
ellipsisContainer.value.textContent = combinedText;
if (ellipsisContainer.value.scrollWidth <= containerWidth) {
startTextLength = mid;
endTextLength = mid;
low = mid + 1;
} else {
high = mid - 1;
}
}
startText.value = fullText.substring(0, startTextLength);
endText.value = fullText.substring(fullText.length - endTextLength);
console.log('startText:', startText.value);
console.log('endText:', endText.value);
};
onMounted(() => {
console.log('Component mounted');
updateText();
});
return {
ellipsisContainer,
startText,
endText,
};
},
});
</script>
<style scoped>
.ellipsis-container {
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
text-emphasis: none;
position: relative;
width: 500px;
max-width: 600px;
}
</style>
二、字符串处理
就是将需字符串截取对应的字符拼接到省略号尾部。
用于提取字符串中介于两个指定下标之间的部分:str.substring(start [, end])
start
:必需,提取的起始下标(从0开始)。如果为负数,则会将该值视为0。
end
:可选,提取的结束下标(不包括此位置的字符)。如果省略,则提取到字符串结尾;如果此参数小于start
参数,则两者会被交换;如果为负数,则会将该值视为0。
javascriptlet str = { value: 'hello world' }; console.log(str.value.substring(0, 5)); // 输出: hello console.log(str.value.substring(6)); // 输出: world
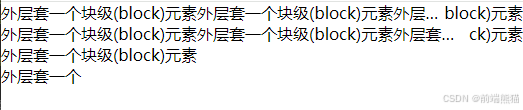
javascript
<template>
<div>
<Ellipsis endLen="8" />
<Ellipsis endLen="5" />
<Ellipsis endLen="5" text="外层套一个块级(block)元素" />
<Ellipsis endLen="10" text="外层套一个" />
</div>
</template>
<script setup>
import Ellipsis from './Ellipsis.vue';
</script>
javascript
<template>
<div class="flex">
<!-- 显示截断后的文本和省略部分 -->
<div class="truncate">{{ truncatedText }}</div>
<div class="shrink-0">{{ endText }}</div>
</div>
</template>
<script setup>
import { ref, computed } from 'vue';
// 定义组件的属性,包括endLen和text
const props = defineProps({
endLen: {
type: Number,
default: 10,
},
text: {
type: String,
default: '外层套一个块级(block)元素外层套一个块级(block)元素外层套一个块级(block)元素外层套一个块级(block)元素',
},
});
// 设置默认文本内容
const defaultText = '外层套一个块级(block)元素、内部是一个行内(inline)元素。给外层元素设置溢出隐藏的样式属性ne)元素。给外层元素设置溢出隐藏的样式';
// 使用ref创建响应式变量str,用于存储文本内容
const str = ref(props.text || defaultText);
// 计算截断后的文本
const truncatedText = computed(() => str.value.substring(0, str.value.length - props.endLen));
// 计算末尾的文本
const endText = computed(() => str.value.substring(str.value.length - props.endLen, str.value.length));
</script>
<style scoped lang="scss">
.flex {
display: flex;
}
.truncate {
overflow: hidden; // 隐藏超出容器的内容
white-space: nowrap; // 不换行显示文本
text-overflow: ellipsis; // 当内容超出容器时,显示省略号
}
.shrink-0 {
flex-shrink: 0; // 确保该元素不会缩小
}
</style>
三、Css伪类

javascript
<template>
<ul class="con">
<li class="wrap">
<span class="txt"> CSS 实现中间内容省略的技巧 - 2024-12-19</span>
<span class="title" title="CSS 实现中间内容省略的技巧 - 2024-12-19">CSS 实现中间内容省略的技巧 - 2024-12-19</span>
</li>
<!-- 其他列表项 -->
</ul>
</template>
<script setup>
</script>
<style scoped>
/* 容器样式 */
.con {
font-size: 14px;
/* 设置字体大小 */
color: #666;
/* 设置文字颜色 */
width: 600px;
/* 设置容器宽度 */
margin: 50px auto;
/* 设置上下外边距为50px,左右居中 */
border-radius: 8px;
/* 设置圆角 */
padding: 15px;
/* 设置内边距 */
overflow: hidden;
/* 隐藏溢出内容 */
resize: horizontal;
/* 允许水平调整大小 */
box-shadow: 20px 20px 60px #bebebe, -20px -20px 60px #ffffff;
/* 添加阴影效果 */
}
/* 列表项样式 */
.wrap {
position: relative;
/* 相对定位 */
line-height: 2;
/* 设置行高 */
height: 2em;
/* 设置高度 */
padding: 0 10px;
/* 设置左右内边距 */
overflow: hidden;
/* 隐藏溢出内容 */
background: #fff;
/* 设置背景色 */
margin: 5px 0;
/* 设置上下外边距 */
}
/* 奇数行列表项样式 */
.wrap:nth-child(odd) {
background: #f5f5f5;
/* 设置背景色 */
}
/* 标题样式 */
.title {
display: block;
/* 块级元素 */
position: relative;
/* 相对定位 */
background: inherit;
/* 继承背景色 */
text-align: justify;
/* 文字两端对齐 */
height: 2em;
/* 设置高度 */
overflow: hidden;
/* 隐藏溢出内容 */
top: -4em;
/* 向上偏移4em,可能需要根据实际情况调整 */
}
/* 文本样式 */
.txt {
display: block;
/* 块级元素 */
max-height: 4em;
/* 设置最大高度 */
}
/* 伪元素样式 */
.title::before {
content: attr(title);
/* 使用title属性的值 */
width: 50%;
/* 设置宽度 */
float: right;
/* 右浮动 */
white-space: nowrap;
/* 防止换行 */
overflow: hidden;
/* 隐藏溢出内容 */
text-overflow: ellipsis;
/* 显示省略号 */
direction: rtl;
/* 从右到左的方向 */
}
</style>