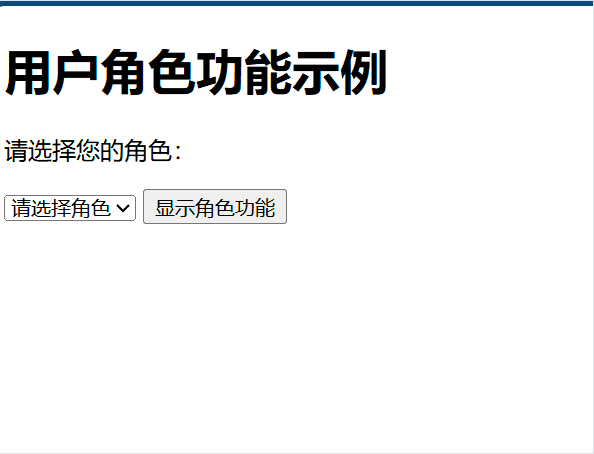
html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>用户角色管理</title>
</head>
<body>
<h1>用户角色功能示例</h1>
<p>请选择您的角色:</p>
<select id="userRole">
<option value="">请选择角色</option>
<option value="admin">管理员</option>
<option value="user">编辑者</option>
<option value="guest">访客</option>
</select>
<button type="button" onclick="showRoleFunction()">显示角色功能</button>
<p id="roleFunction"></p>
</body>
<script>
/* 1.显示角色功能的函数
function showRoleFunction() {
const role = document.getElementById("userRole").value;
const roleFunction = document.getElementById("roleFunction");
switch (role) {
case "admin":
roleFunction.innerText = "您是管理员,可以进行所有操作";
break;
case "user":
roleFunction.innerText = "您是编辑者,可以进行部分操作";
break;
case "guest":
roleFunction.innerText = "您是访客,只能查看内容";
break;
default:
roleFunction.innerText = "请选择有效的角色";
break;
}
} */
/*2. 也可以使用箭头函数
const showRoleFunction = () => {
const role = document.getElementById("userRole").value;
const roleFunction = document.getElementById("roleFunction");
switch (role) {
case "admin":
roleFunction.innerText = "您是管理员,可以进行所有操作";
break;
case "user":
roleFunction.innerText = "您是编辑者,可以进行部分操作";
break;
case "guest":
roleFunction.innerText = "您是访客,只能查看内容";
break;
default:
roleFunction.innerText = "请选择有效的角色";
break;
}
} */
/* 3. 使用对象字面量简化代码*/
function showRoleFunction() {
const role = document.getElementById("userRole").value;
const roleFunction = document.getElementById("roleFunction");
if (!role) {
roleFunction.innerText = "请选择有效的角色";
return; // 提前返回,避免不必要的计算
}
const roleMessages = {
admin: "您是管理员,可以进行所有操作",
user: "您是编辑者,可以进行部分操作",
guest: "您是访客,只能查看内容"
};
roleFunction.innerText = roleMessages[role] || "请选择有效的角色"; // 根据角色动态获取信息
}
</script>
</html>