flutter插件开发是一个重要的技能,拓展flutter与原生的通信,将一些公用的东西封装,给不同的项目使用。
阅读前置:
flutter基本通道调用
objective-c基础语法
ios项目基础知识
目录
1、创建一个插件项目
创建一个ios原生的插件项目,指定平台为ios,语言为objective-c,也可以是android平台等等。
cpp
flutter create -t plugin --platforms=ios --ios-language=objc add_helper
2、项目结构
example是该项目内置的一个帮助测试我们开发的插件代码的dart项目
从pubspec.yaml文件中可看出,依赖的就是即将开发的插件项目
lib中有三个文件
- add_helper.dart
- add_helper_method_channel.dart
- add_helper_platform_interface.dart
这三个文件应该倒着理解,看一下interface里面,这就是一个抽象的通道平台接口,里面定义与原生通道的方法,里面就内置了一个官方的例子,获取平台版本号的方法抽象。
cpp
Future<String?> getPlatformVersion() {
throw UnimplementedError('platformVersion() has not been implemented.');
}
///添加一个自定义的方法
Future<int?> add(int a, int b) {
throw UnimplementedError('add() has not been implemented.');
}
可以添加自定义的方法,测试文件可能会报错,点进去暴力注释掉就行,method_channel就是platform_interface的实现
cpp
@override
Future<String?> getPlatformVersion() async {
final version = await methodChannel.invokeMethod<String>('getPlatformVersion');
return version;
}
@override
Future<int?> add(int a, int b) async {
final result = await methodChannel.invokeMethod<int>('add', <String, int>{
'a': a,
'b': b,
});
return result;
}
最后这些方法在第一个文件统一通过一个类再封装方法给需要用到的地方的调用。
cpp
class AddHelper {
Future<String?> getPlatformVersion() {
return AddHelperPlatform.instance.getPlatformVersion();
}
Future<int?> add(int a, int b) {
return AddHelperPlatform.instance.add(a, b);
}
}
3、编写原生代码
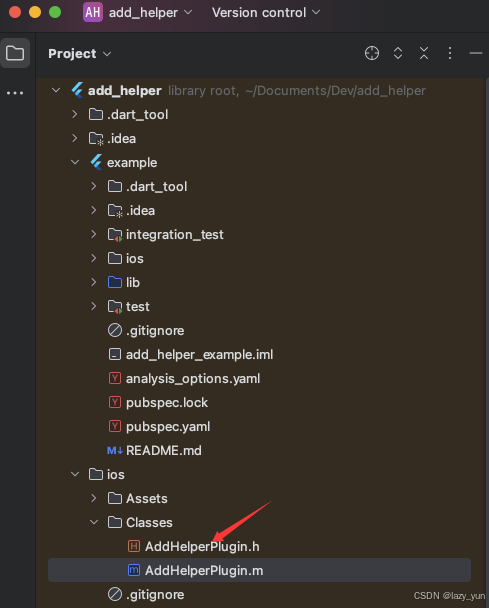
因为我们生成的是oc代码,所以这里是这样子的,接下来需要初始化ios项目,进入example,执行
cpp
flutter build ios
成功后,打开xcode,打开example下的ios,既然是编写原生插件,就在原生项目中进行开发,插件的代码是被集成在了pod里面的,可以看到,位置很深
添加原生处理代码,只需要在获取版本的方法后面加一个case就行
cpp
- (void)handleMethodCall:(FlutterMethodCall*)call result:(FlutterResult)result {
if ([@"getPlatformVersion" isEqualToString:call.method]) {
result([@"iOS " stringByAppendingString:[[UIDevice currentDevice] systemVersion]]);
} else if ([@"add" isEqualToString:call.method]) {
int a = [call.arguments[@"a"] intValue];
int b = [call.arguments[@"b"] intValue];
result(@(a + b));
} else {
result(FlutterMethodNotImplemented);
}
}
4、编写flutter端测试代码
回到android studio,/add_helper/example/lib/main.dart中编写
cpp
int totalCount = 0;
///
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Plugin example app'),
),
body: Center(
child: Column(
children: [
Text('Running on: $_platformVersion\n'),
ElevatedButton(
onPressed: () async {
int? val = await _addHelperPlugin.add(totalCount, 1);
setState(() {
totalCount = val ?? -1;
});
},
child: Text('totalCount: $totalCount'),
)
],
),
),
),
);
}
运行项目:
参考文档:
Flutter插件开发指南01: 通道Channel的编写与实现
Writing custom platform-specific code