Jmockit使用笔记_基本功能使用@Tested_@Injectable_@Mocked_Expectations_jmockit.class-CSDN博客
测试框架Jmockit集合junit使用
@RunWith(JMockit.class)
写在测试案例类上的注解
@Tested
在测试案例中,写在我们要测试的类上面, 一般用实现类
@Injectable
在测试案例中声明那些我们要测试的类中通过@Autowired注入的类
原文链接:https://blog.csdn.net/Java_XiaoBei/article/details/126150129
/**
* @author: xinruoxiangyang9010
* 测试案例
*/
@RunWith(JMockit.class)
public class MyServicemplTest {
@Injectable
private MyMapper myMapper;
@Tested
private MyServicempl myServicempl;
@Test
public void testMethod() {
String result = myServicempl.testMethod();
assertEquals("myService", result);
}
}
2. 必须加 @RunWith(JMockit.class)
的情况
- 使用 JUnit 4 :
如果你的项目是基于 JUnit 4 的测试框架,而测试类中使用了 JMockit 提供的注解(如@Mocked
、@Injectable
)或 API(如Expectations
、Verifications
),则必须加上@RunWith(JMockit.class)
。
原因 :
JUnit 4 默认使用BlockJUnit4ClassRunner
运行器,而 JMockit 的特性需要通过JMockitTestRunner
扩展运行器支持。如果不加@RunWith(JMockit.class)
,JMockit 的增强功能不会生效。
测试类中不使用 JMockit 特性 :
如果你的测试没有使用 JMockit 的 @Mocked
、@Injectable
、@Tested
或其他功能,只是普通的单元测试,则可以不加 @RunWith(JMockit.class)
。
Expectations:返回他的result
在 JMockit 的 Expectations
中,如果没有为方法设置返回值(result
),或将 result
设置为 null
,则会根据方法的签名返回以下内容:
1. 没有指定 result
的情况
new Expectations(MyStringUtil.class) {{
MyStringUtil.getUUID(); // 没有设置 result
}};
String resultString = MyStringUtil.getUUID();
assertEquals(null, resultString); // 返回 null
- 如果没有显式设置
result
,默认行为是方法返回类型的默认值 :- 基本类型 (如
int
、double
):返回其默认值(0
或0.0
)。 - 对象类型 :返回
null
。 void
方法:不会有返回值。
- 基本类型 (如
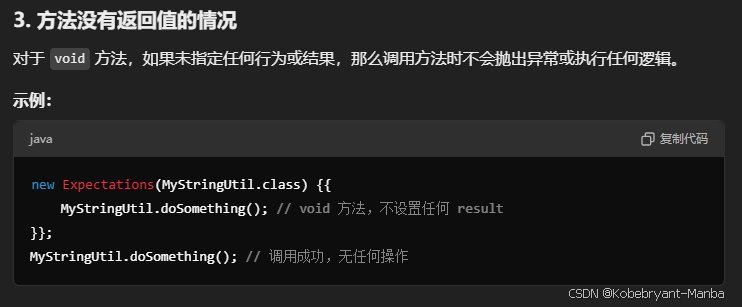
-
如果是void
方法 不能设置result
值,因为它不返回任何内容。 -
如果你尝试为
void
方法设置result
,JMockit 会抛出异常。java.lang.IllegalArgumentException: Attempted to specify a result for a void method. -
对于
void
方法,你可以使用Verifications
来验证方法的调用行为。@Test
public void voidMethodTest() {
new Expectations() {{
// 模拟任何期望,但不设置返回值
MyStringUtil.doSomething();
}};// 调用 void 方法 MyStringUtil.doSomething(); // 验证 void 方法是否被调用 new Verifications() {{ MyStringUtil.doSomething(); // 验证是否调用 times = 1; // 验证调用次数 }};
}
这段代码就是检查doSomething()是否调用了1次,times=2就是检查是否用了两次
下面这段代码太好了:
结合Expectations的使用对@Mocked与@Injectable的不同
/**
* @author: xinruoxiangyang9010
* 测试案例
*/
@RunWith(JMockit.class)
public class MockAndInjectTest {
@Test
public void testMock(@Mocked DiffServiceImpl diffService) {
new Expectations() {
{
diffService.method();
result = "mockMethod";
}
};
assertEquals("mockMethod", diffService.method());
// @Mocked修饰的变量,即便是再new出来的,也会按照上面我们mock的结果返回
assertEquals("mockMethod", new DiffServiceImpl().method());
}
@Test
public void testInjectable (@Injectable DiffServiceImpl diffService) {
new Expectations() {
{
diffService.method();
result = "mockMethod";
}
};
assertEquals("mockMethod", diffService.method());
// 注意这里结果的不同, 在对@Injectable修饰的对象进行Expectations指定结果时
// 受影响的只是被修饰的对象,如果我们new一个示例出来,是不会受Expectations影响的
assertEquals("real", new DiffServiceImpl().method());
}
}