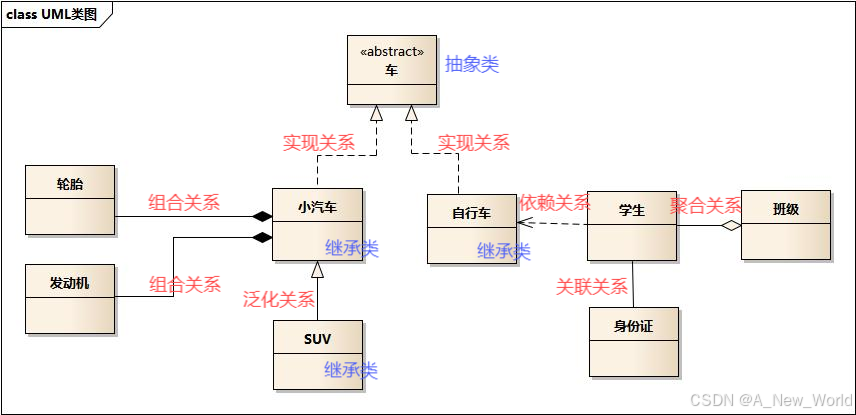
// 发动机类
class Engine {
public:
void start() {
std::cout << "Engine started." << std::endl;
}
};
// 身份证类
class IDCard {
public:
std::string idNumber;
};
// 抽象类车
class Vehicle {
public:
virtual void drive() = 0; // 纯虚函数,使类成为抽象类
};
// 小汽车类,实现车类
class SmallCar : public Vehicle {
private:
Engine engine;
public:
void drive() override {
engine.start();
std::cout << "Small car is driving." << std::endl;
}
};
// SUV类,继承小汽车类
class SUV : public SmallCar {
public:
void drive() override {
std::cout << "SUV is driving to the mountains." << std::endl;
}
};
// 自行车类,实现车类
class Bicycle : public Vehicle {
public:
void drive() override {
std::cout << "Bicycle is riding." << std::endl;
}
};
// 班级类
class Class {
private:
std::vector<Student*> students; // 聚合学生类
public:
void addStudent(Student* student) {
students.push_back(student);
}
};
// 学生类
class Student {
private:
IDCard idCard;
Class* classObj; // 聚合班级类
public:
Student(Class* class_) : classObj(class_) {}
void goToSchool(Bicycle* bicycle) { // 依赖自行车类
std::cout << "Student is going to school by bicycle." << std::endl;
bicycle->drive();
}
};
完整代码
cpp#include <iostream> #include <vector> // 发动机类 class Engine { public: void start() { std::cout << "Engine started." << std::endl; } }; // 身份证类 class IDCard { public: std::string idNumber; }; // 抽象类车 class Vehicle { public: virtual void drive() = 0; // 纯虚函数,使类成为抽象类 }; // 小汽车类,实现车类 class SmallCar : public Vehicle { private: Engine engine; public: void drive() override { engine.start(); std::cout << "Small car is driving." << std::endl; } }; // SUV类,继承小汽车类 class SUV : public SmallCar { public: void drive() override { std::cout << "SUV is driving to the mountains." << std::endl; } }; // 自行车类,实现车类 class Bicycle : public Vehicle { public: void drive() override { std::cout << "Bicycle is riding." << std::endl; } }; // 班级类 class Class { private: std::vector<Student*> students; // 聚合学生类 public: void addStudent(Student* student) { students.push_back(student); } }; // 学生类 class Student { private: IDCard idCard; Class* classObj; // 聚合班级类 public: Student(Class* class_) : classObj(class_) {} void goToSchool(Bicycle* bicycle) { // 依赖自行车类 std::cout << "Student is going to school by bicycle." << std::endl; bicycle->drive(); } }; int main() { // 创建班级对象 Class schoolClass; // 创建学生对象并关联班级 Student student1(&schoolClass); // 创建自行车对象 Bicycle bicycle; // 学生骑自行车上学 student1.goToSchool(&bicycle); // 创建小汽车对象并驾驶 SmallCar smallCar; smallCar.drive(); // 创建 SUV 对象并驾驶 SUV suv; suv.drive(); return 0; }