学习目标:
@State装饰器
@Prop装饰器
@Link装饰器
@Link装饰器
@Provide装饰器
@Consume装饰器
学习内容:
GrandsonComponent
typescript
@Component
export struct GrandsonComponent {
@Consume('provideValue') consumeValue: number
build() {
Column(){
Text('孙组件').fontSize(25)
Text('consumeValue: ' + this.consumeValue)
Button('Consume变量测试,父子兄弟都会变')
.onClick(() => {
this.consumeValue = Math.floor(Math.random() * (100))
})
}
}
}
HelloComponent
typescript
import {GrandsonComponent} from './GrandsonComponent'
@Component
export struct HelloComponent {
@State stateValue: number = 0
@Prop propValue: number = 0
@Link linkValue: number
@Consume('provideValue') consumeValue: number
build() {
// HelloComponent自定义组件组合系统组件Row和Text
Column({space:10}) {
//父子之前双向同步
Text('stateValue: '+this.stateValue.toString())
//父->子单项同步
Text('propValue: '+this.propValue.toString())
Text('linkValue: '+this.linkValue.toString())
Text('consumeValue: '+this.consumeValue.toString())
Button('子State变量测试,只会当前组件变')
.onClick(() => {
this.stateValue = Math.floor(Math.random() * (100))
})
Button('子Prop变量测试,只会当前组件变')
.onClick(() => {
this.propValue = Math.floor(Math.random() * (100))
})
Button('子Link变量测试, 子变父也变')
.onClick(() => {
this.linkValue = Math.floor(Math.random() * (100));
})
GrandsonComponent()
}.height('100%')
.width('100%')
}
}
ParentComponent
typescript
import {HelloComponent} from './HelloComponent'
@Entry
@Component
struct ParentComponent {
// 父组件的变化不会影响子子组件改变
@State stateValue: number = 1
// 父组件的变化会影响子子组件改变
@State propValue: number = 2
// 父子双向同步
@State linkValue: number = 3
@Provide provideValue: number = 5
build() {
Column({ space: 5 }) {
Text('父组件').fontSize(25)
Divider()
//父子之前双向同步
Text('stateValue: ' + this.stateValue.toString())
//父->子单项同步
Text('propValue: ' + this.propValue.toString())
Text('linkValue: ' + this.linkValue.toString())
Text('provideValue: ' + this.provideValue.toString())
Button('父State变量测试,只会当前组件变')
.onClick(() => {
this.stateValue = Math.floor(Math.random() * (100));
})
Button('父Prop变量测试, 父变子也变')
.onClick(() => {
this.propValue = Math.floor(Math.random() * (100));
})
Button('父Link变量测试,父变子也变')
.onClick(() => {
this.linkValue = Math.floor(Math.random() * (100));
})
Button('父Provide变量测试,父子兄弟都会变')
.onClick(() => {
this.provideValue = Math.floor(Math.random() * (100));
})
Text('子组件').fontSize(25)
Divider()
HelloComponent({ stateValue: this.stateValue, propValue: this.propValue, linkValue: this.linkValue });
}
.height('100%')
.width('100%')
}
}
学习产出:
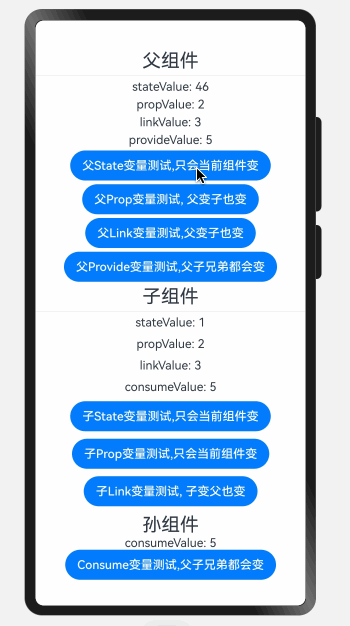