1.设置环境
打开终端输入如下命令:
bash
mkdir apidemo
cd apidemo
npm init -y
npm install express
touch server.js
在server.js输入代码
javascript
const express = require('express');
const app = express();
const PORT = 3030;
// 中间件 - 解析JSON请求体
app.use(express.json());
// 示例数据存储
let users = [
{ id: 1, name: "Alice", age: 25 },
{ id: 2, name: "Bob", age: 30 },
];
// 首页路由
app.get('/', (req, res) => {
res.send('Welcome to My API!');
});
// 获取所有用户
app.get('/users', (req, res) => {
res.json(users);
});
// 根据ID获取单个用户
app.get('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ error: 'User not found' });
res.json(user);
});
// 添加新用户
app.post('/users', (req, res) => {
const { name, age } = req.body;
if (!name || !age) return res.status(400).json({ error: 'Name and age are required' });
const newUser = { id: users.length + 1, name, age };
users.push(newUser);
res.status(201).json(newUser);
});
// 更新用户
app.put('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ error: 'User not found' });
const { name, age } = req.body;
if (name) user.name = name;
if (age) user.age = age;
res.json(user);
});
// 删除用户
app.delete('/users/:id', (req, res) => {
const userIndex = users.findIndex(u => u.id === parseInt(req.params.id));
if (userIndex === -1) return res.status(404).json({ error: 'User not found' });
users.splice(userIndex, 1);
res.status(204).send();
});
// 启动服务器
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
2.运行服务器
打开终端输入
bash
node server.js
3.验证接口
打开Postman查看请求结果
GET请求
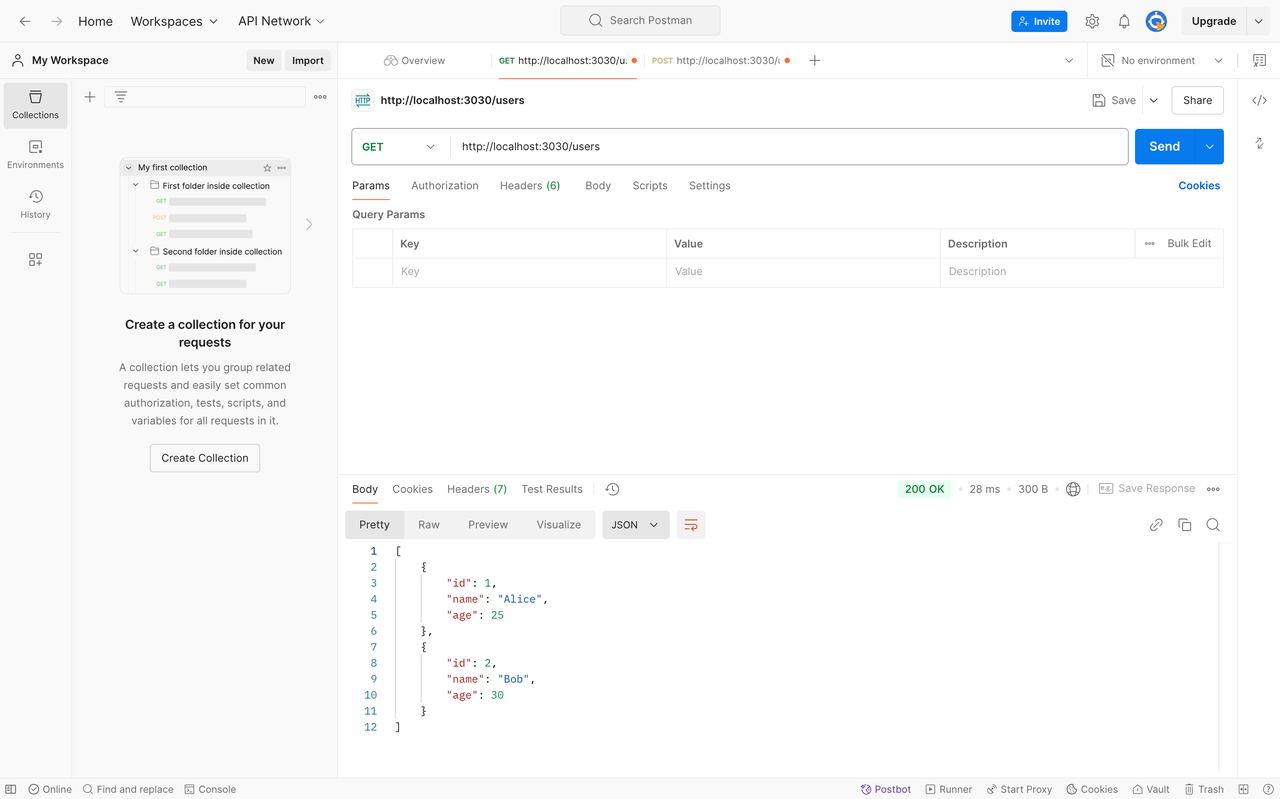
POST请求
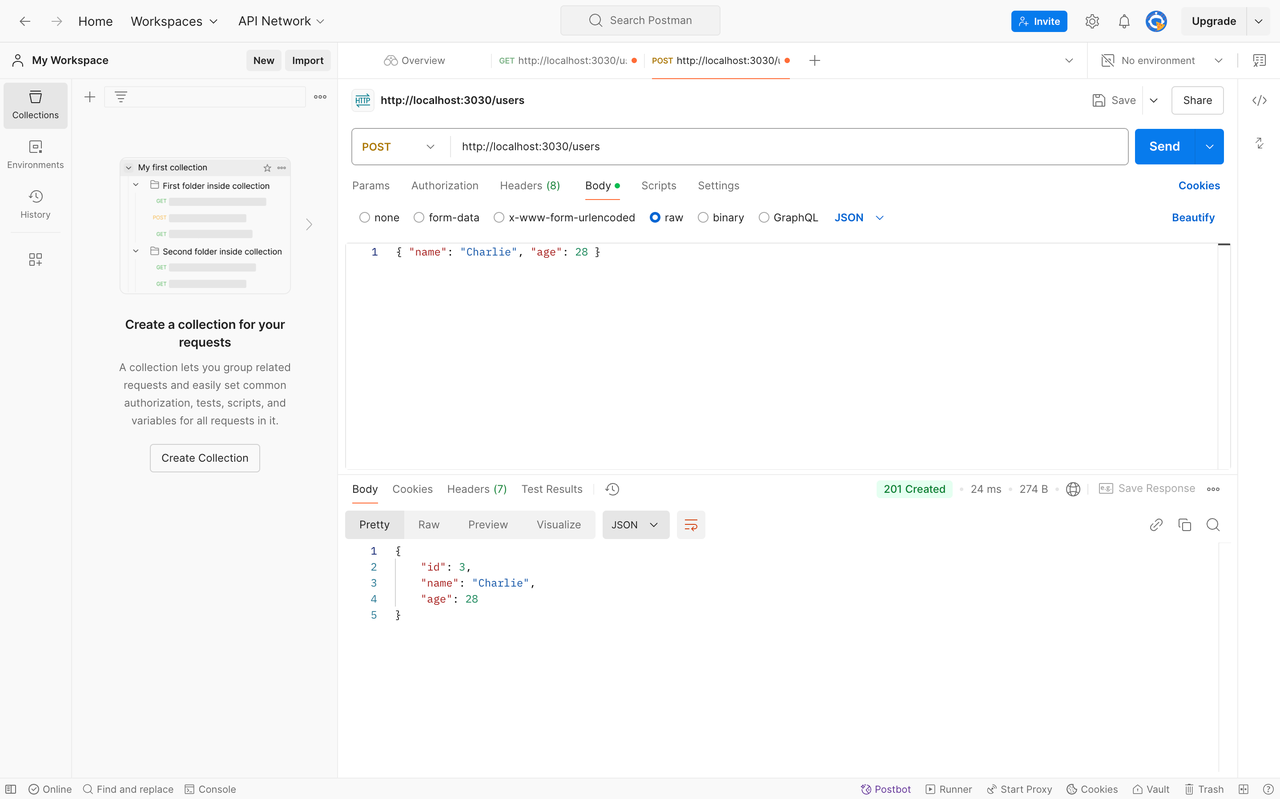