文章目录
Vue3 自定义插件(plugin)
概述
插件 (Plugins) 是一种能为 Vue 添加全局功能的工具代码。
用法
Vue3 插件有2种定义方式:
- 对象形式。对象中有install方法。
- 函数形式。函数本身就是安装方法,其中,函数可以接收两个参数,分别是安装它的应用实例和传递给app.use的额外选项。
定义插件:
对象形式:
javascript
import Header from "../components/Header.vue";
// 对象方式:
const myPlugin = {
install(app, options) {
// 配置全局方法
app.config.globalProperties.globalMethod = function (value) {
return value.toLowerCase();
};
// 注册公共组件
app.component("Header", Header);
// 注册公共指令
app.directive("upper", function (el, binding) {
// 转为大写
el.textContent = binding.value.toUpperCase();
if (binding.arg === "small") {
el.style.fontSize = options.small + "px";
} else if (binding.arg === "medium") {
el.style.fontSize = options.medium + "px";
} else {
el.style.fontSize = options.large + "px";
}
});
}
};
export default myPlugin;
函数形式:
javascript
import Header from "../components/Header.vue";
// 函数方式:
const myPlugin = function (app, options) {
// 配置全局方法
app.config.globalProperties.globalMethod = function (value) {
// 转为小写
return value.toLowerCase();
};
// 注册公共组件
app.component("Header", Header);
// 注册公共指令
app.directive("upper", function (el, binding) {
console.log("binding:", binding);
// 转为大写
el.textContent = binding.value.toUpperCase();
if (binding.arg === "small") {
el.style.fontSize = options.small + "px";
} else if (binding.arg === "medium") {
el.style.fontSize = options.medium + "px";
} else {
el.style.fontSize = options.large + "px";
}
});
};
export default myPlugin;
使用插件:
javascript
import {createApp} from "vue";
import "../style.css";
import App from "./plugin.vue";
import myPlugin from "./myPlugin/index.js";
const app = createApp(App);
// 安装插件
app.use(myPlugin, {
small: 12,
medium: 24,
large: 36
});
app.mount("#app");
vue
<template>
<Header></Header>
<p v-upper:large="'hello large'"></p>
<p v-upper:medium="'hello medium'"></p>
<p v-upper:small="'hello small'"></p>
<p>{{ globalMethod("Hello World") }}</p>
</template>
效果:
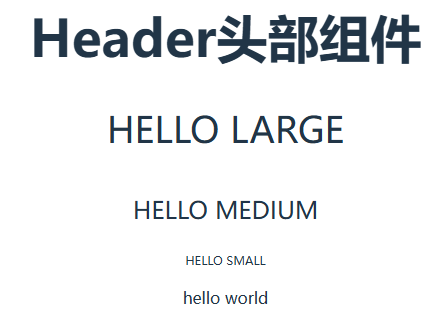