一、加载更多:
在到达底部时,将新请求过来的数据追加到原来的数组即可:
import {
onReachBottom
} from "@dcloudio/uni-app";
const pets = ref([]); // 显示数据
function network() {
uni.request({
url: "https://api.thecatapi.com/v1/images/search",
data: {
limit: 10
}
}).then(res => {
pets.value = [...pets.value, ...res.data];
}).catch(error => {
uni.showToast({
title: '请求有误会',
icon: "none"
})
}).finally(() => {
// uni.hideLoading();
uni.hideNavigationBarLoading();
uni.stopPullDownRefresh();
})
}
onReachBottom(() => {
network();
})
二、下拉刷新:
在下拉时加载新数据:
import {
onPullDownRefresh
} from "@dcloudio/uni-app";
onPullDownRefresh(() => {
pets.value = []; // 先将原来的数据清空,然后加载新数据
network();
})
三、返回顶部:
使用Uniapp界面滚动API实现:
uni.pageScrollTo({
scrollTop: 0,
duration: 100
})
其它知识点:
uni.showNavigationBarLoading(); // 导航栏中显示加载状态
uni.hideNavigationBarLoading(); // 导航栏中隐藏加载状态
// 页面中显示加载状态
uni.showLoading({
title: '加载中'
});
uni.hideLoading(); // 页面中隐藏加载状态
uni.startPullDownRefresh(); // 下拉,需在pages.json对应页面的style位置开启:enablePullDownRefresh
uni.stopPullDownRefresh(); // 停止下拉
env(safe-area-inset-bottom):css中获取底部安全区高度;
组件完整代码:
<template>
<view class="container">
<view class="monitor-list-wrapper">
<view class="monitor-list" v-for="(pet, index) in pets" :key="pet.id">
<image lazy-load :src="pet.url" mode="aspectFill" class="monitor-photo" @click="onPreview(index)">
</image>
<view class="monitor-title">
{{pet.id}}
</view>
</view>
</view>
<view class="load-more">
<uni-load-more status="loading"></uni-load-more>
</view>
<view class="float">
<view class="item" @click="onRefresh"><uni-icons type="refresh" size="26" color="#888"></uni-icons></view>
<view class="item" @click="onTop"><uni-icons type="arrow-up" size="26" color="#888"></uni-icons></view>
</view>
</view>
</template>
<script setup>
import {
ref
} from "vue";
import {
onReachBottom,
onPullDownRefresh
} from "@dcloudio/uni-app";
const pets = ref([]);
function network() {
// uni.showLoading({
// title: '加载中'
// });
uni.showNavigationBarLoading();
uni.request({
url: "https://api.thecatapi.com/v1/images/search",
data: {
limit: 10
}
}).then(res => {
pets.value = [...pets.value, ...res.data];
}).catch(error => {
uni.showToast({
title: '请求有误会',
icon: "none"
})
}).finally(() => {
// uni.hideLoading();
uni.hideNavigationBarLoading();
uni.stopPullDownRefresh();
})
}
network();
// 图片预览
function onPreview(index) {
const urls = pets.value.map(item => item.url);
uni.previewImage({
current: index,
urls
})
}
function onRefresh() {
uni.startPullDownRefresh(); // 需在pages.json对应位置开启:enablePullDownRefresh
}
function onTop() {
uni.pageScrollTo({
scrollTop: 0,
duration: 100
})
}
onReachBottom(() => {
network();
})
onPullDownRefresh(() => {
pets.value = [];
network();
})
</script>
<style lang="scss" scoped>
.container {
padding: 0 $myuni-spacing-super-lg;
background: #D4ECFF;
}
.monitor-list-wrapper {
display: grid;
grid-template-columns: repeat(2, 1fr);
gap: $myuni-spacing-lg;
.monitor-list {
border-radius: $myuni-border-radius-base;
padding: $myuni-spacing-lg;
width: 305rpx;
background-color: $myuni-bg-color;
.monitor-photo {
height: 200rpx;
width: 100%;
}
}
.monitor-title {
height: 32rpx;
line-height: 32rpx;
color: $myuni-text-color;
font-size: $myuni-font-size-lg;
text-align: center;
}
}
.load-more {
padding-bottom: calc(env(safe-area-inset-bottom) + 80rpx);
}
.float {
position: fixed;
right: 30rpx;
bottom: 80rpx;
padding-bottom: env(safe-area-inset-bottom);
.item {
width: 90rpx;
height: 90rpx;
border-radius: 50%;
background-color: rgba(255, 255, 255, 0.9);
margin-bottom: 20rpx;
display: flex;
align-items: center;
justify-content: center;
border: 1px solid #EEE;
}
}
</style>
四、实现效果:
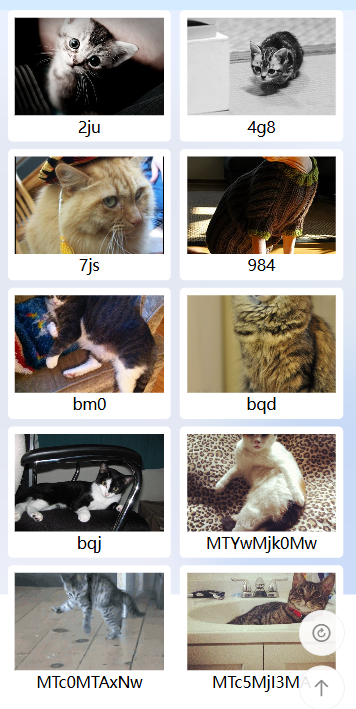