工具
Linux项目自动化构建------make/ Makefile
bash
[wt@ikun ~]$ mkdir makefile
[wt@ikun ~]$ cd makefile
[wt@ikun makefile]$ touch code.c
[wt@ikun makefile]$ ls >makefile
[wt@ikun makefile]$ vim makefile
[wt@ikun makefile]$ ll
total 8
-rw-rw-r-- 1 wt wt 75 Dec 30 14:01 code.c
-rw-rw-r-- 1 wt wt 66 Dec 30 13:58 makefile
bash
[wt@ikun makefile]$ vim makefile
[wt@ikun makefile]$ make
gcc -E code.c -o code.i
gcc -S code.i -o code.s
gcc -c code.s -o code.o
gcc code.o -o code.exe
[wt@ikun makefile]$ ll
total 48
-rw-rw-r-- 1 wt wt 75 Dec 30 14:01 code.c
-rwxrwxr-x 1 wt wt 8360 Dec 30 15:37 code.exe
-rw-rw-r-- 1 wt wt 16874 Dec 30 15:37 code.i
-rw-rw-r-- 1 wt wt 1496 Dec 30 15:37 code.o
-rw-rw-r-- 1 wt wt 445 Dec 30 15:37 code.s
-rw-rw-r-- 1 wt wt 230 Dec 30 15:37 makefile
[wt@ikun makefile]$ ./code.exe
hello aaa
[wt@ikun makefile]$ make clean
rm -f code.i code.s code.o code.exe
[wt@ikun makefile]$ ll
total 8
-rw-rw-r-- 1 wt wt 75 Dec 30 14:01 code.c
-rw-rw-r-- 1 wt wt 287 Dec 30 15:40 makefile
makefile/make会自动根据文件中的依赖关系,进行自动推导,帮助我们执行所有相关的依赖方法
最终目标文件必须是第一个,后面的可以乱序
可以定义变量
加上@符号,就不会打印出来了
进度条
\r&&\n和行缓冲区概念
回车和换行
\n-->回车、换行
是两个动作
换行:换到当前位置正下面一行
回车:回到行首
只想回车:\r
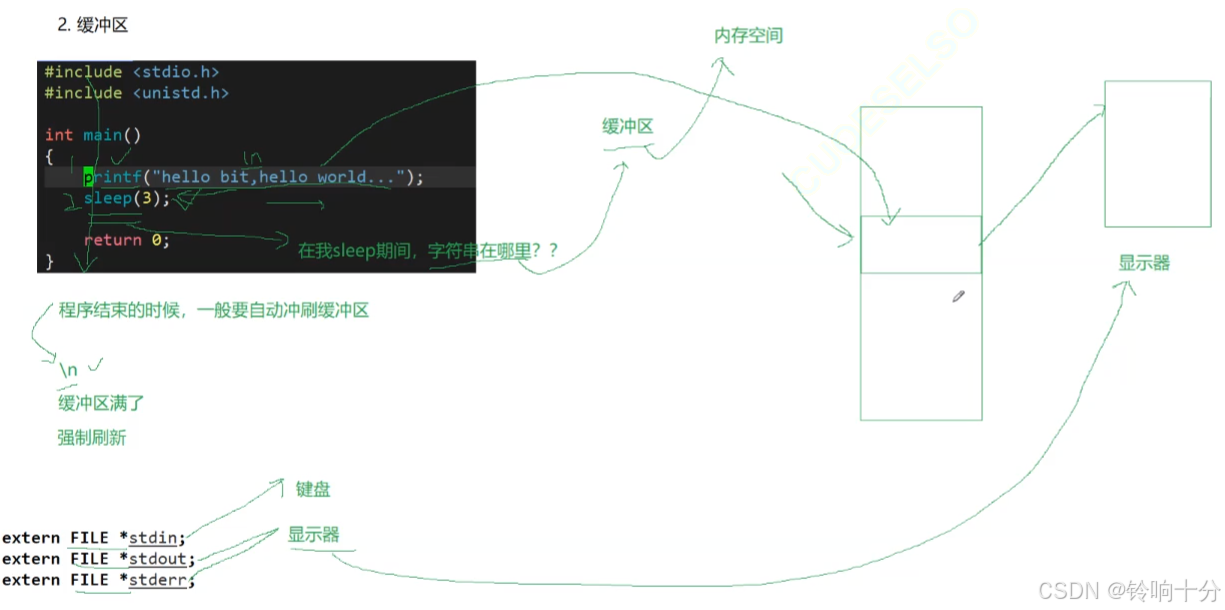
bash
[wt@ikun process]$ ll
total 0
-rw-rw-r-- 1 wt wt 0 Jan 7 16:59 Main.c
-rw-rw-r-- 1 wt wt 0 Jan 7 16:59 Makefile
-rw-rw-r-- 1 wt wt 0 Jan 7 16:58 Processbar.c
-rw-rw-r-- 1 wt wt 0 Jan 7 17:01 Processbar.h
[wt@ikun process]$ cat Processbar.h
#pragma once
#include<stdio.h>
extern void ForTest();
[wt@ikun process]$ cat Processbar.c
#include "Processbar.h"
void ForTest()
{
printf("this is for test\n");
printf("this is for test\n");
printf("this is for test\n");
printf("this is for test\n");
printf("this is for test\n");
printf("this is for test\n");
}
[wt@ikun process]$ cat Main.c
#include"Processbar.h"
int main()
{
ForTest();
return 0;
}
进度条代码
bash
[wt@ikun process]$ ll
total 16
-rw-rw-r-- 1 wt wt 840 Jan 11 15:01 Main.c
-rw-rw-r-- 1 wt wt 84 Jan 7 22:09 Makefile
-rw-rw-r-- 1 wt wt 1155 Jan 11 14:59 Processbar.c
-rw-rw-r-- 1 wt wt 165 Jan 11 14:57 Processbar.h
Makefile
c
1 processbar:Main.c Processbar.c
2 gcc -o $@ $^
3 .PHONY:clean
4 clean:
5 rm -f processbar
Processbar.h
c
1 #pragma once
2
3 #include<stdio.h>
4
5 typedef void(*callback_t)(double, double);
6 //extern void ForTest();
7
8 //void ProcBar();
9 void ProcBar(double total, double current);
Processbar.c
c
1 #include "Processbar.h"
2 #include<string.h>
3 #include<unistd.h>
4 //void ForTest()
5 //{
6 // printf("this is for test\n");
7 // printf("this is for test\n");
8 // printf("this is for test\n");
9 // printf("this is for test\n");
10 // printf("this is for test\n");
11 // printf("this is for test\n");
12 //}
13
14
15
16 #define Length 101
17 #define Style '#'
18
19 const char *lable = "|/-\\";
20
21 // version 1
22 //void ProcBar()
23 //{
24 // char bar[Length];
25 // memset(bar, '\0', sizeof(bar));
26 // int len = strlen(lable);
27 //
28 // int cnt = 0;
29 // while(cnt <= 100)
30 // {
31 // printf("[%-100s][%3d%%][%c]\r", bar, cnt, lable[cnt%len]);
32 // fflush(stdout);
33 // bar[cnt++] = Style;
34 // usleep(20000);
35 // }
36 // printf("\n");
37 //}
38
39 //version 2
40 void ProcBar(double total, double current)
41 {
42 char bar[Length];
43 memset(bar, '\0', sizeof(bar));
44 int len = strlen(lable);
45
46 int cnt = 0;
47 double rate = (current*100.0)/total;
48 int loop_count = (int)rate;
49 while(cnt <= loop_count)
50 {
51 bar[cnt++] = Style;
52 //usleep(20000);
53 }
54 printf("[%-100s][%.1lf%%][%c]\r", bar, rate, lable[cnt%len]);
55 fflush(stdout);
56 }
Main.c
c
1 #include"Processbar.h"
2 #include<unistd.h>
3
4 double bandwidth = 1024*1024*1.0;
5 //download
6 void download(double filesize, callback_t cb)
7 {
8 double current = 0.0;
9
10 printf("download begin, current: %lf\n", current);
11 while(current <= filesize)
12 {
13 cb(filesize, current);
14 //从网络中获取数据
15 current += bandwidth;
16 usleep(100000);
17 }
18
19 printf("\ndownload done, fileszie: %lf\n", filesize);
20 }
21
22 int main()
23 {
24 download(100*1024*1024, ProcBar);
25 download(2*1024*1024, ProcBar);
26 download(200*1024*1024, ProcBar);
27 download(400*1024*1024, ProcBar);
28 download(50*1024*1024, ProcBar);
29 download(10*1024*1024, ProcBar);
30 //ForTest();
31 //ProcBar();
32 //ProcBar(100.0, 56.9);
33 //ProcBar(100.0, 1.0);
34 //ProcBar(100.0, 99.9);
35 //ProcBar(100.0, 100);
36 return 0;
37 }
git
查看git是否安装
bash
[wt@ikun process]$ git --version
git version 1.8.3.1
如何安装
bash
[wt@ikun process]$ sudo yum install -y git
下载项目到本地
- 创建好一个放置代码的目录
bash
[tt@kunkun ~]$ mkdir test
[tt@kunkun ~]$ cd test
[tt@kunkun test]$ pwd
/home/tt/test
- 下载到本地
bash
git clone 链接
bash
[tt@kunkun test]$ git clone https://gitee.com/xxxxxxxx
Cloning into 'code'...
remote: Enumerating objects: 6, done.
remote: Counting objects: 100% (6/6), done.
remote: Compressing objects: 100% (6/6), done.
remote: Total 6 (delta 0), reused 0 (delta 0), pack-reused 0
Unpacking objects: 100% (6/6), done.
[tt@kunkun test]$ ll
total 4
drwxrwxr-x 3 tt tt 4096 Jan 21 21:07 code
[tt@kunkun test]$ cd code
[tt@kunkun code]$ ll
total 20
-rw-rw-r-- 1 tt tt 9247 Jan 21 21:07 LICENSE
-rw-rw-r-- 1 tt tt 820 Jan 21 21:07 README.en.md
-rw-rw-r-- 1 tt tt 909 Jan 21 21:07 README.md
[tt@kunkun code]$ ll -a
total 36
drwxrwxr-x 3 tt tt 4096 Jan 21 21:07 .
drwxrwxr-x 3 tt tt 4096 Jan 21 21:07 ..
drwxrwxr-x 8 tt tt 4096 Jan 21 21:07 .git
-rw-rw-r-- 1 tt tt 430 Jan 21 21:07 .gitignore
-rw-rw-r-- 1 tt tt 9247 Jan 21 21:07 LICENSE
-rw-rw-r-- 1 tt tt 820 Jan 21 21:07 README.en.md
-rw-rw-r-- 1 tt tt 909 Jan 21 21:07 README.md
[tt@kunkun code]$ tree .git
.git
├── branches
├── config
├── description
├── HEAD
├── hooks
│ ├── applypatch-msg.sample
│ ├── commit-msg.sample
│ ├── post-update.sample
│ ├── pre-applypatch.sample
│ ├── pre-commit.sample
│ ├── prepare-commit-msg.sample
│ ├── pre-push.sample
│ ├── pre-rebase.sample
│ └── update.sample
├── index
├── info
│ └── exclude
├── logs
│ ├── HEAD
│ └── refs
│ ├── heads
│ │ └── master
│ └── remotes
│ └── origin
│ └── HEAD
├── objects
│ ├── 15
│ │ └── b02eb41ad6bedbd33901ce5205fc9f301df97b
│ ├── 86
│ │ └── 7c042f0ec24833c5be1b5a8abd5251a4828f71
│ ├── bb
│ │ └── 2d5cab8ccea66fa5e8674df83a41dc64fe5d93
│ ├── c6
│ │ └── 127b38c1aa25968a88db3940604d41529e4cf5
│ ├── da
│ │ └── bfb8024d2463255431920abcbef3faa8194f81
│ ├── f6
│ │ └── 3f5a9cf3498818a73068495709cceed67efd6a
│ ├── info
│ └── pack
├── packed-refs
└── refs
├── heads
│ └── master
├── remotes
│ └── origin
│ └── HEAD
└── tags
22 directories, 26 files
- git add
将代码放到刚才下载好的目录中,提交到暂存区
bash
git add 文件名
将需要用git管理的文件告知git
bash
[tt@kunkun code]$ cp ../../code.c . -rf
[tt@kunkun code]$ ll
total 20
-rw-rw-r-- 1 tt tt 0 Jan 21 21:16 code.c
-rw-rw-r-- 1 tt tt 9247 Jan 21 21:07 LICENSE
-rw-rw-r-- 1 tt tt 820 Jan 21 21:07 README.en.md
-rw-rw-r-- 1 tt tt 909 Jan 21 21:07 README.md
[tt@kunkun code]$ git status
# On branch master
# Untracked files:
# (use "git add <file>..." to include in what will be committed)
#
# code.c
nothing added to commit but untracked files present (use "git add" to track)
[tt@kunkun code]$ git add .
[tt@kunkun code]$ git status
# On branch master
# Changes to be committed:
# (use "git reset HEAD <file>..." to unstage)
#
# new file: code.c
#
- git commit
提交改动到本地
bash
git commit .
最后的"."表示当前目录
提交的时候应该注明提交日志,描述改动的详细内容
bash
[tt@kunkun code]$ git commit
*** Please tell me who you are.
Run
git config --global user.email "you@example.com"
git config --global user.name "Your Name"
to set your account's default identity.
Omit --global to set the identity only in this repository.
fatal: empty ident name (for <tt@kunkun.(none)>) not allowed
[tt@kunkun code]$ git commit -m "first commit, this is my code.c"
*** Please tell me who you are.
Run
git config --global user.email "you@example.com"
git config --global user.name "Your Name"
to set your account's default identity.
Omit --global to set the identity only in this repository.
fatal: empty ident name (for <tt@kunkun.(none)>) not allowed
[tt@kunkun code]$ git config --global user.name 'xxxx'
[tt@kunkun code]$ git config --global user.email 'xxxxxxxxxx@qq.com'
[tt@kunkun code]$ git commit -m "first commit, this is my code.c"
[master 5c690aa] first commit, this is my code.c
1 file changed, 0 insertions(+), 0 deletions(-)
create mode 100644 code.c
-m 后面跟日志
bash
git commit -m "first commit, this is my code.c"
- git push
bash
[tt@kunkun code]$ git push
warning: push.default is unset; its implicit value is changing in
Git 2.0 from 'matching' to 'simple'. To squelch this message
and maintain the current behavior after the default changes, use:
git config --global push.default matching
To squelch this message and adopt the new behavior now, use:
git config --global push.default simple
See 'git help config' and search for 'push.default' for further information.
(the 'simple' mode was introduced in Git 1.7.11. Use the similar mode
'current' instead of 'simple' if you sometimes use older versions of Git)
Username for 'https://gitee.com':
Password for 'https://xxxxxxx@gitee.com':
Counting objects: 4, done.
Delta compression using up to 2 threads.
Compressing objects: 100% (2/2), done.
Writing objects: 100% (3/3), 293 bytes | 0 bytes/s, done.
Total 3 (delta 1), reused 0 (delta 0)
remote: Powered by GITEE.COM [1.1.5]
remote: Set trace flag 4a4887b9
To https://gitee.com/spring-day-wild-bell/code.git
15b02eb..5c690aa master -> master
[tt@kunkun code]$ git status
# On branch master
nothing to commit, working directory clean
- 倒序查看提交记录
bash
[tt@kunkun code]$ git log
commit 5c690aaea1cbd59e52552ed1d3b8996d9bcaf20c
Author: xxxx <xxxxxxxx@qq.com>
Date: Tue Jan 21 21:22:58 2025 +0800
first commit, this is my code.c
commit 15b02eb41ad6bedbd33901ce5205fc9f301df97b
Author: xxxx <xxxxxxx@qq.com>
Date: Tue Jan 21 12:55:48 2025 +0000
Initial commit
- 删除文件
bash
[tt@kunkun code]$ git rm code.c
rm 'code.c'
[tt@kunkun code]$ git add .
[tt@kunkun code]$ git commit -m "delete code.c"
[master 63a67fa] delete code.c
1 file changed, 0 insertions(+), 0 deletions(-)
delete mode 100644 code.c
[tt@kunkun code]$ git push
warning: push.default is unset; its implicit value is changing in
Git 2.0 from 'matching' to 'simple'. To squelch this message
and maintain the current behavior after the default changes, use:
git config --global push.default matching
To squelch this message and adopt the new behavior now, use:
git config --global push.default simple
See 'git help config' and search for 'push.default' for further information.
(the 'simple' mode was introduced in Git 1.7.11. Use the similar mode
'current' instead of 'simple' if you sometimes use older versions of Git)
Username for 'https://gitee.com':
Password for 'https://xxxxxxx@gitee.com':
Counting objects: 3, done.
Delta compression using up to 2 threads.
Compressing objects: 100% (2/2), done.
Writing objects: 100% (2/2), 231 bytes | 0 bytes/s, done.
Total 2 (delta 1), reused 0 (delta 0)
remote: Powered by GITEE.COM [1.1.5]
remote: Set trace flag 1f461289
To https://gitee.com/xxxxxxxx/code.git
5c690aa..63a67fa master -> master
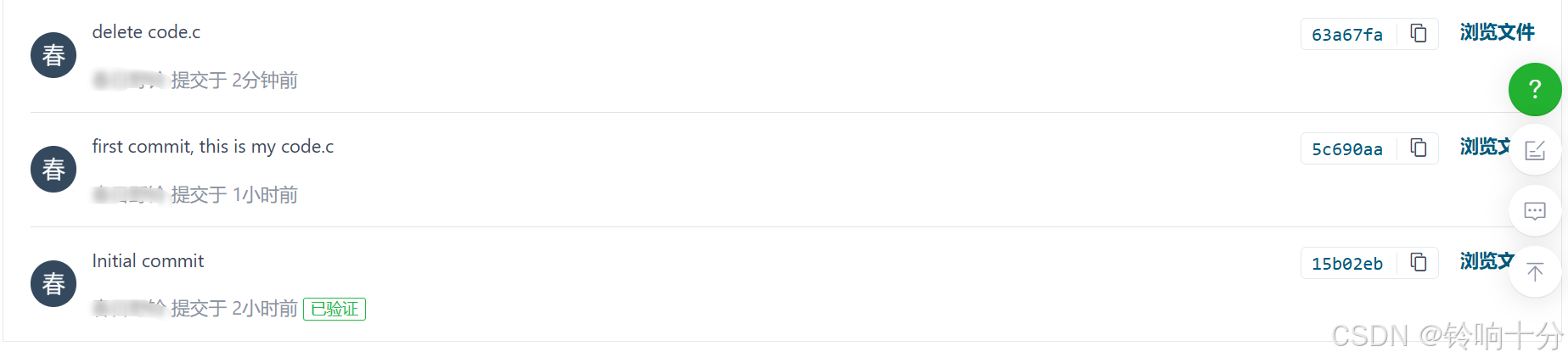
- .gitignore
对特定文件进行忽略的配置文件