文章目录
- 关于
- 基本介绍👋
- 新的问题
- 更好的解决方案
- Week8
-
- [Mon Inheritance](#Mon Inheritance)
-
- 阅读材料
- [Lab 06: Object-Oriented Programming](#Lab 06: Object-Oriented Programming)
-
- [Q1: Bank Account](#Q1: Bank Account)
- [Q2: Email](#Q2: Email)
- [Q3: Mint](#Q3: Mint)
关于
个人博客,里面偶尔更新,最近比较忙。发一些总结的帖子和思考。
江湖有缘相见🤝。如果读者想和我交个朋友可以加我好友(见主页or个人博客),共同学习。笔者是学生,课业还是比较繁重的,可能回复不及时。笔者也正在四处寻找一些可以兼职锻炼知识并且补贴一些生活的工作,如果读者需要一些详细的辅导,或者帮助完成一些简易的lab也可以找我,笔者还是学生,自以为才学有限,也没有高价的理由📖。
基本介绍👋
CS61A是加州大学伯克利分校(UC Berkeley)计算机科学专业的入门课程,全名为Structure and Interpretation of Computer Programs。
这是入门的Python课程,因此这篇博客会默认读者没有很多的编程背景,讲的东西会比较基础,有编程经验的读者可以快速浏览。
首先贴上课程网址。
CS61A课程主页
新的问题
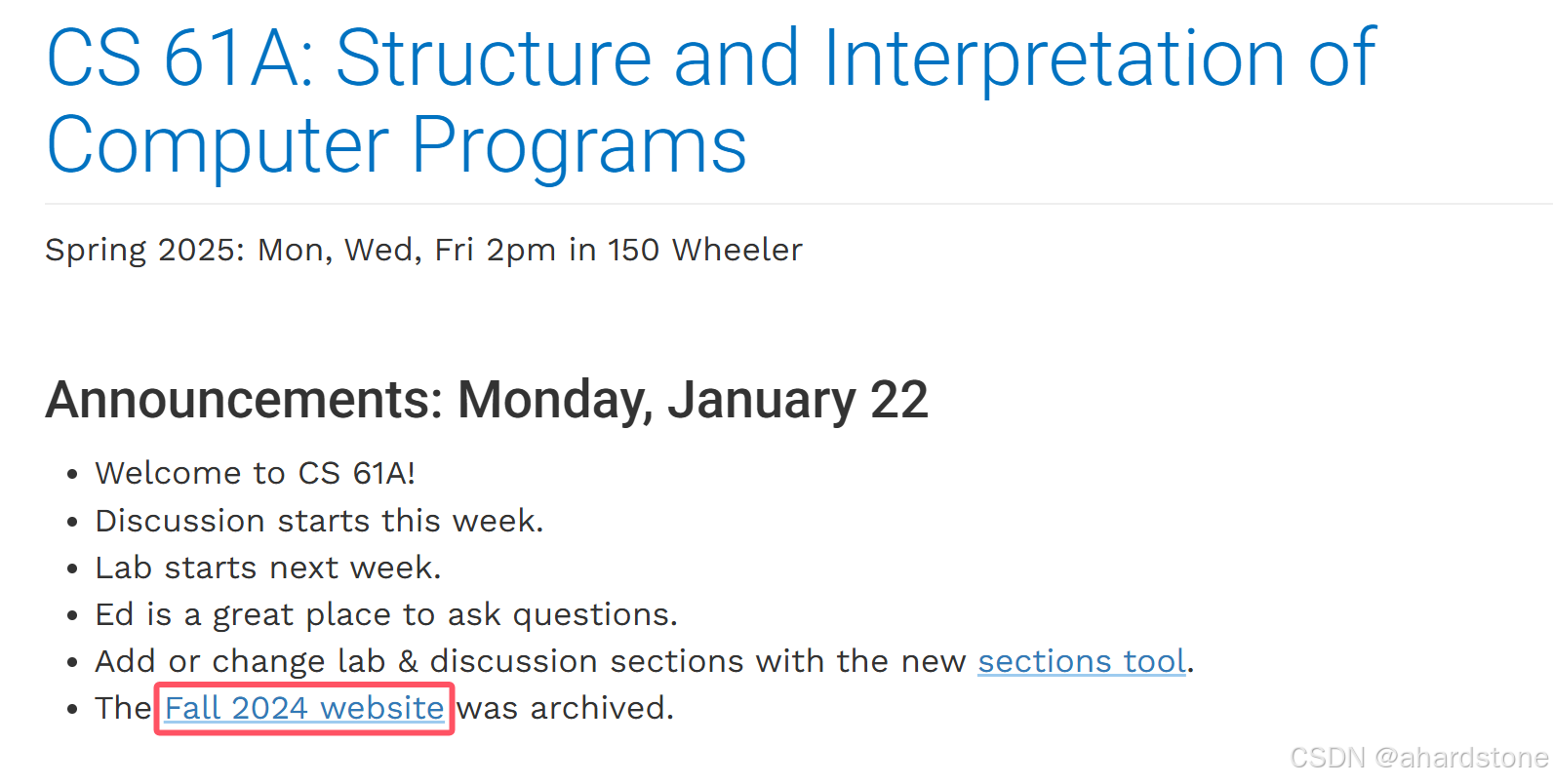
可以发现,cs61a归档了,而这个网站是需要Berkeley账号的,还是没法登录。这时候笔者决定使用档案馆网站,去翻网页的归档了。虽然有点难受,但是还能用orz。
对了,textbook是可以直接访问的,在这里
更好的解决方案
我在GitHub上找到了cs61a 2024 fall的归档,这里给出连接link
Week8
Mon Inheritance
阅读材料
Ants里面已经写了很多类了,这里才开始具体讲面向对象编程orz。
Lab 06: Object-Oriented Programming
关键是要搞清楚实例属性,类属性的区别。前者修改只会影响当前实例,后者会影响当前类的全部实例。
Q1: Bank Account
这题注意交易失败也要记录下来。
py
class Transaction:
def __init__(self, id, before, after):
self.id = id
self.before = before
self.after = after
def changed(self):
"""Return whether the transaction resulted in a changed balance."""
"*** YOUR CODE HERE ***"
return self.before != self.after
def report(self):
"""Return a string describing the transaction.
>>> Transaction(3, 20, 10).report()
'3: decreased 20->10'
>>> Transaction(4, 20, 50).report()
'4: increased 20->50'
>>> Transaction(5, 50, 50).report()
'5: no change'
"""
msg = 'no change'
if self.changed():
"*** YOUR CODE HERE ***"
if self.after > self.before:
msg = f'increased {self.before}->{self.after}'
else:
msg = f'decreased {self.before}->{self.after}'
return str(self.id) + ': ' + msg
class BankAccount:
"""A bank account that tracks its transaction history.
>>> a = BankAccount('Eric')
>>> a.deposit(100) # Transaction 0 for a
100
>>> b = BankAccount('Erica')
>>> a.withdraw(30) # Transaction 1 for a
70
>>> a.deposit(10) # Transaction 2 for a
80
>>> b.deposit(50) # Transaction 0 for b
50
>>> b.withdraw(10) # Transaction 1 for b
40
>>> a.withdraw(100) # Transaction 3 for a
'Insufficient funds'
>>> len(a.transactions)
4
>>> len([t for t in a.transactions if t.changed()])
3
>>> for t in a.transactions:
... print(t.report())
0: increased 0->100
1: decreased 100->70
2: increased 70->80
3: no change
>>> b.withdraw(100) # Transaction 2 for b
'Insufficient funds'
>>> b.withdraw(30) # Transaction 3 for b
10
>>> for t in b.transactions:
... print(t.report())
0: increased 0->50
1: decreased 50->40
2: no change
3: decreased 40->10
"""
# *** YOU NEED TO MAKE CHANGES IN SEVERAL PLACES IN THIS CLASS ***
def __init__(self, account_holder):
self.balance = 0
self.holder = account_holder
self.id = 0
self.transactions = []
def deposit(self, amount):
"""Increase the account balance by amount, add the deposit
to the transaction history, and return the new balance.
"""
self.transactions.append(Transaction(self.id, self.balance, self.balance + amount))
self.id += 1
self.balance = self.balance + amount
return self.balance
def withdraw(self, amount):
"""Decrease the account balance by amount, add the withdraw
to the transaction history, and return the new balance.
"""
if amount > self.balance:
self.transactions.append(Transaction(self.id, self.balance, self.balance))
self.id += 1
return 'Insufficient funds'
self.transactions.append(Transaction(self.id, self.balance, self.balance - amount))
self.id += 1
self.balance = self.balance - amount
return self.balance
Q2: Email
填完邮件类。注意一下即可。
py
class Email:
"""An email has the following instance attributes:
msg (str): the contents of the message
sender (Client): the client that sent the email
recipient_name (str): the name of the recipient (another client)
"""
def __init__(self, msg, sender, recipient_name):
self.msg = msg
self.sender = sender
self.recipient_name = recipient_name
class Server:
"""Each Server has one instance attribute called clients that is a
dictionary from client names to client objects.
"""
def __init__(self):
self.clients = {}
def send(self, email):
"""Append the email to the inbox of the client it is addressed to.
email is an instance of the Email class.
"""
self.clients[email.recipient_name].inbox.append(email)
def register_client(self, client):
"""Add a client to the clients mapping (which is a
dictionary from client names to client instances).
client is an instance of the Client class.
"""
self.clients[client.name] = client
class Client:
"""A client has a server, a name (str), and an inbox (list).
>>> s = Server()
>>> a = Client(s, 'Alice')
>>> b = Client(s, 'Bob')
>>> a.compose('Hello, World!', 'Bob')
>>> b.inbox[0].msg
'Hello, World!'
>>> a.compose('CS 61A Rocks!', 'Bob')
>>> len(b.inbox)
2
>>> b.inbox[1].msg
'CS 61A Rocks!'
>>> b.inbox[1].sender.name
'Alice'
"""
def __init__(self, server, name):
self.inbox = []
self.server = server
self.name = name
server.register_client(self)
def compose(self, message, recipient_name):
"""Send an email with the given message to the recipient."""
email = Email(message, self, recipient_name)
self.server.send(email)
Q3: Mint
可以通过类名直接获取类属性。
py
class Mint:
"""A mint creates coins by stamping on years.
The update method sets the mint's stamp to Mint.present_year.
>>> mint = Mint()
>>> mint.year
2024
>>> dime = mint.create(Dime)
>>> dime.year
2024
>>> Mint.present_year = 2104 # Time passes
>>> nickel = mint.create(Nickel)
>>> nickel.year # The mint has not updated its stamp yet
2024
>>> nickel.worth() # 5 cents + (80 - 50 years)
35
>>> mint.update() # The mint's year is updated to 2104
>>> Mint.present_year = 2179 # More time passes
>>> mint.create(Dime).worth() # 10 cents + (75 - 50 years)
35
>>> Mint().create(Dime).worth() # A new mint has the current year
10
>>> dime.worth() # 10 cents + (155 - 50 years)
115
>>> Dime.cents = 20 # Upgrade all dimes!
>>> dime.worth() # 20 cents + (155 - 50 years)
125
"""
present_year = 2024
def __init__(self):
self.update()
def create(self, coin):
"*** YOUR CODE HERE ***"
return coin(self.year)
def update(self):
"*** YOUR CODE HERE ***"
self.year = self.present_year
class Coin:
cents = None # will be provided by subclasses, but not by Coin itself
def __init__(self, year):
self.year = year
def worth(self):
"*** YOUR CODE HERE ***"
return self.cents + max(Mint.present_year - self.year - 50, 0)
class Nickel(Coin):
cents = 5
class Dime(Coin):
cents = 10