防止自己遗忘,故作此为记录。
步骤:
(1)进入例题,找到需要点击的元素。
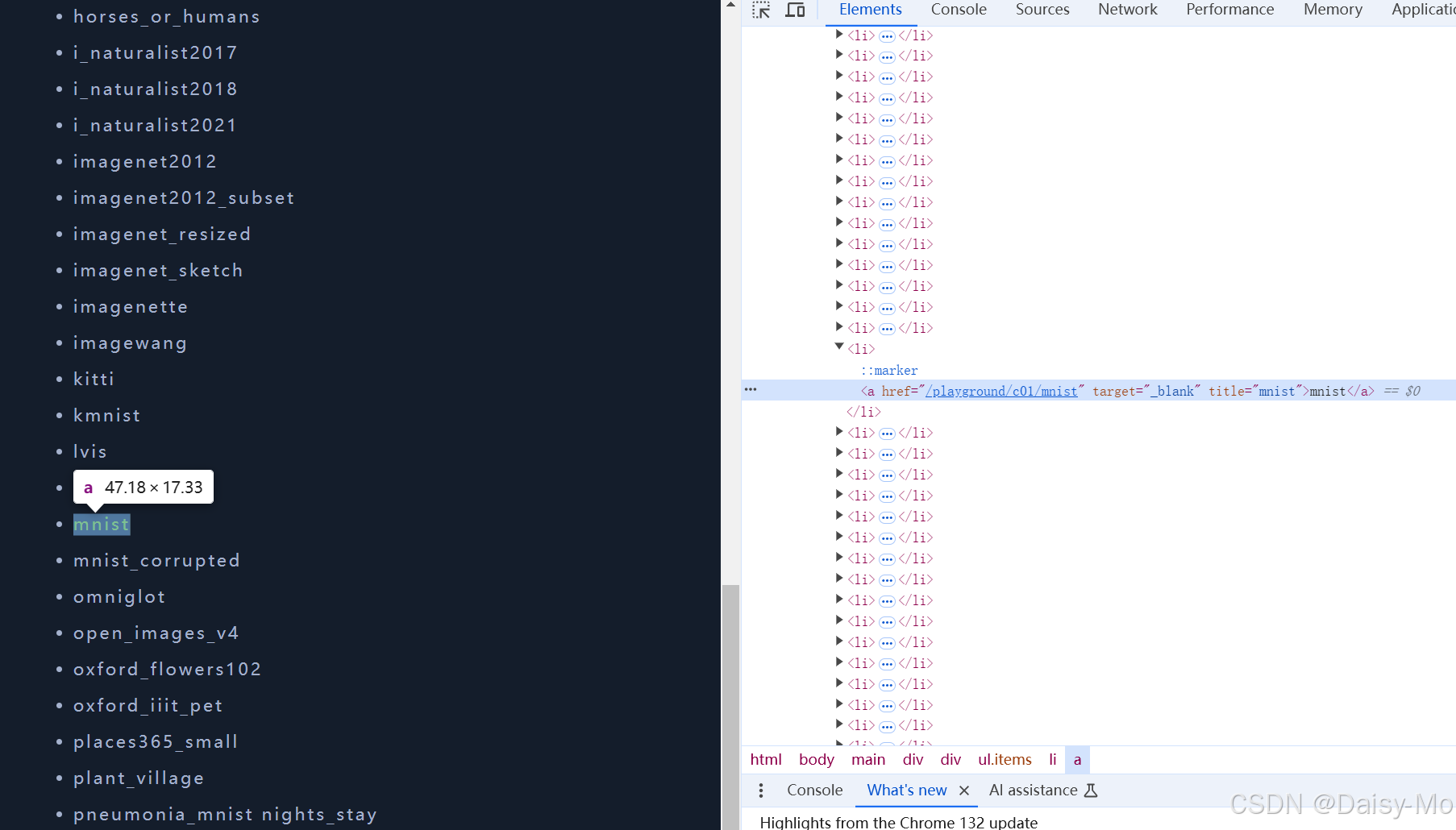
可得button xpath:
click_xpath: str = r'//li/a[@title="mnist"]'
WebDriverWait(driver, 10).until(expected_conditions.element_to_be_clickable((By.XPATH, click_xpath)))
res = driver.find_element(By.XPATH, click_xpath)
注意,此时点击res的attribute是完整url。(卡顿在此步,以为url还需要拼接)
之后发现:
可得final_xpath:
final_xpath: str = r"//tbody/tr/td[2]"
WebDriverWait(driver, 10).until(expected_conditions.visibility_of_element_located((By.XPATH, final_xpath)))
res: list = driver.find_elements(By.XPATH, final_xpath)
最后计算:
res: list[float] = [eval(e.text) for e in res]
s: Decimal = Decimal("0.0")
for each in res:
s += Decimal(each)
s /= len(res)
print(f"{s=}")
s2=Decimal('3.766666666666666666666666667')
#四舍五入为3.77
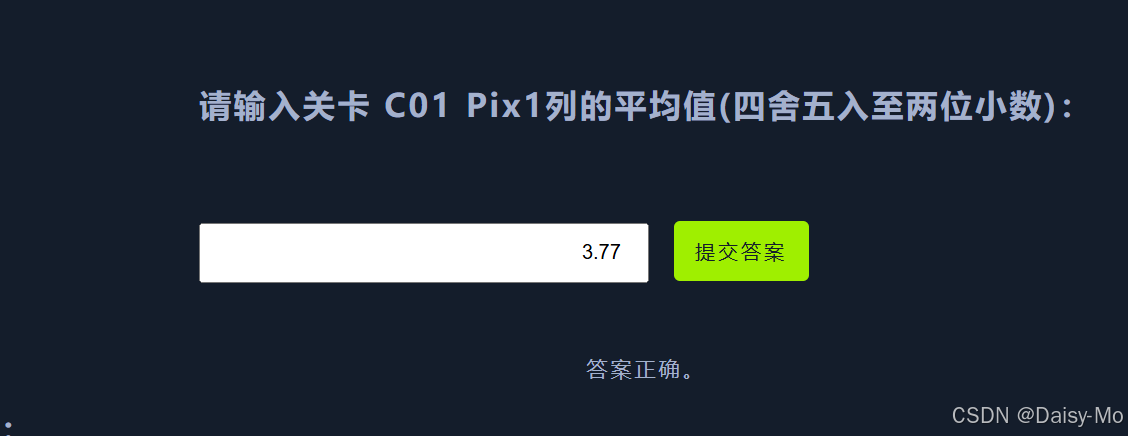
完整代码:
python
# -*- coding: utf-8 -*-
# -*- file: C01.py -*-
from decimal import Decimal
from selenium.webdriver import Chrome
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.chrome.options import Options as ChromeOptions
from selenium.webdriver.chrome.service import Service as ChromeService
first_url: str = r"https://www.spiderbuf.cn/playground/c01"
service = ChromeService(r"C01\chromedriver-win64\chromedriver.exe")
options = ChromeOptions()
options.add_experimental_option("excludeSwitches", ["enable-automation"])
options.add_argument("--disable-blink-features=AutomationControlled")
driver = Chrome(options=options, service=service)
driver.get(first_url)
click_xpath: str = r'//li/a[@title="mnist"]'
WebDriverWait(driver, 10).until(expected_conditions.element_to_be_clickable((By.XPATH, click_xpath)))
res = driver.find_element(By.XPATH, click_xpath)
driver.implicitly_wait(3)
driver.get(res.get_attribute("href"))
#WebDriverWait(driver, 10).until(lambda driver: driver.current_url != first_url)
final_xpath: str = r"//tbody/tr/td[2]"
WebDriverWait(driver, 10).until(expected_conditions.visibility_of_element_located((By.XPATH, final_xpath)))
res: list = driver.find_elements(By.XPATH, final_xpath)
res = [eval(e.text) for e in res]
length: int = len(res)
s1: float = sum(res) / length
s2: Decimal = Decimal("0.0")
for each in res:
s2 += Decimal(each)
s2 /= length
print(f"{s1=}", f"{s2=}")
driver.close()