Spring Cloud Gateway简介
Spring cloud gateway是spring官方基于Spring 5.0、Spring Boot2.0和Project Reactor等技术开发的网关,Spring Cloud Gateway旨在为微服务架构提供简单、有效和统一的API路由管理方式,Spring Cloud Gateway作为Spring Cloud生态系统中的网关,目标是替代Netflix Zuul,其不仅提供统一的路由方式,并且还基于Filter链的方式提供了网关基本的功能,例如:安全、监控/埋点、限流等。
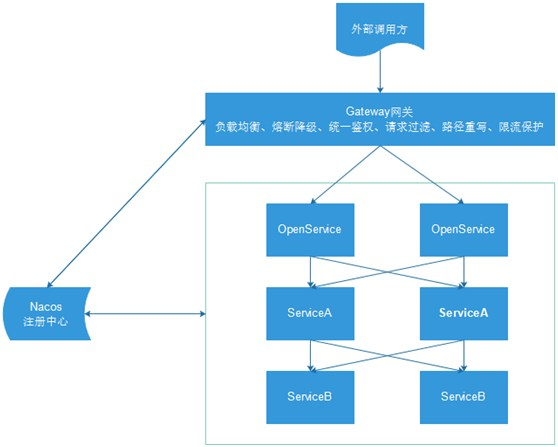
Spring Cloud Gateway核心概念
网关提供API全托管服务,丰富的API管理功能,辅助企业管理大规模的API,以降低管理成本和安全风险,包括协议适配、协议转发、安全策略、防刷、流量、监控日志等贡呢。一般来说网关对外暴露的URL或者接口信息,我们统称为路由信息。如果研发过网关中间件或者使用过Zuul的人,会知道网关的核心是Filter以及Filter Chain(Filter责任链)。Sprig Cloud Gateway也具有路由和Filter的概念。下面介绍一下Spring Cloud Gateway中几个重要的概念。
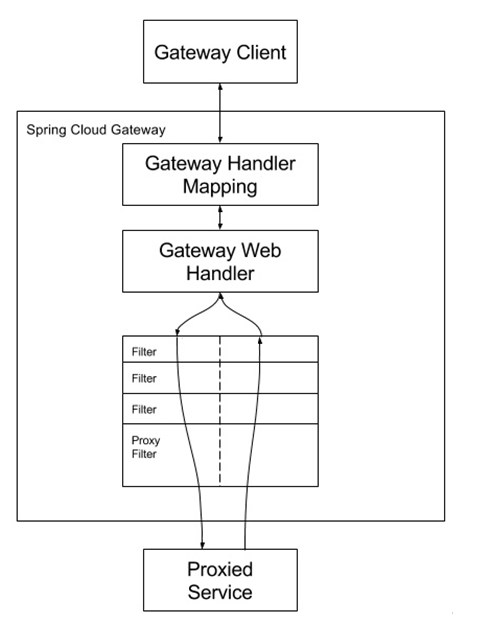
**(1)路由。**路由是网关最基础的部分,路由信息有一个ID、一个目的URL、一组断言和一组Filter组成。如果断言路由为真,则说明请求的URL和配置匹配
(2)断言。Java8中的断言函数。Spring Cloud Gateway中的断言函数输入类型是Spring5.0框架中的ServerWebExchange。Spring Cloud Gateway中的断言函数允许开发者去定义匹配来自于http request中的任何信息,比如请求头和参数等。
(3)过滤器。一个标准的Spring webFilter。Spring cloud gateway中的filter分为两种类型的Filter,分别是Gateway Filter和Global Filter。过滤器Filter将会对请求和响应进行修改处理
如图所示,Spring cloud Gateway发出请求。然后再由Gateway Handler Mapping中找到与请求相匹配的路由,将其发送到Gateway web handler。Handler再通过指定的过滤器链将请求发送到实际的服务执行业务逻辑,然后返回。
应用
1. 创建service_gateway模块
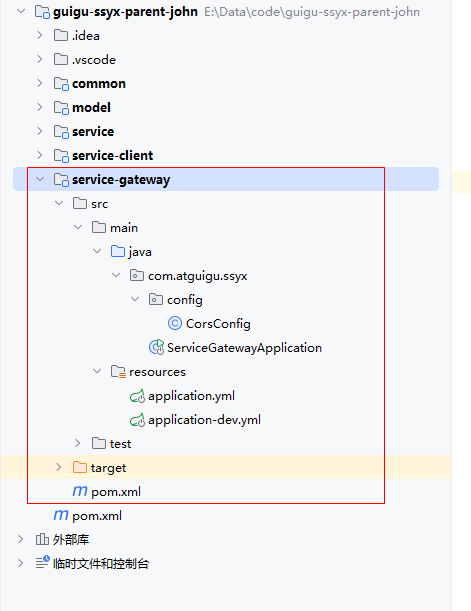
2. 在pom.xml引入依赖: nacos 和 gateway
XML
<!--服务注册-->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
3. 编写配置文件
application.yml
XML
spring:
application:
name: server-gateway
profiles:
active: dev
application-dev.yml
XML
server:
port: 8200 #自定义的端口号
spring:
cloud:
gateway:
discovery:
locator:
enabled: true #启用gateway
routes:
- id: service-acl #自己定义的服务的名称
uri: lb://service-acl
predicates:
- Path=/*/acl/** #自己定义的服务的地址中含有的路径关键字符串,下同
- id: service-sys
uri: lb://service-sys
predicates:
- Path=/*/sys/**
- id: service-product
uri: lb://service-product
predicates:
- Path=/*/product/**
- id: service-activity
uri: lb://service-activity
predicates:
- Path=/*/activity/**
- id: service-order
uri: lb://service-order
predicates:
- Path=/*/order/**
- id: service-payment
uri: lb://service-payment
predicates:
- Path=/*/payment/**
- id: service-user
uri: lb://service-user
predicates:
- Path=/*/user/**
- id: service-search
uri: lb://service-search
predicates:
- Path=/*/search/**
- id: service-home
uri: lb://service-home
predicates:
- Path=/*/home/**
- id: service-cart
uri: lb://service-cart
predicates:
- Path=/*/cart/**
4. 编写启动类
java
package com.atguigu.ssyx;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ServiceGatewayApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceGatewayApplication.class, args);
}
}
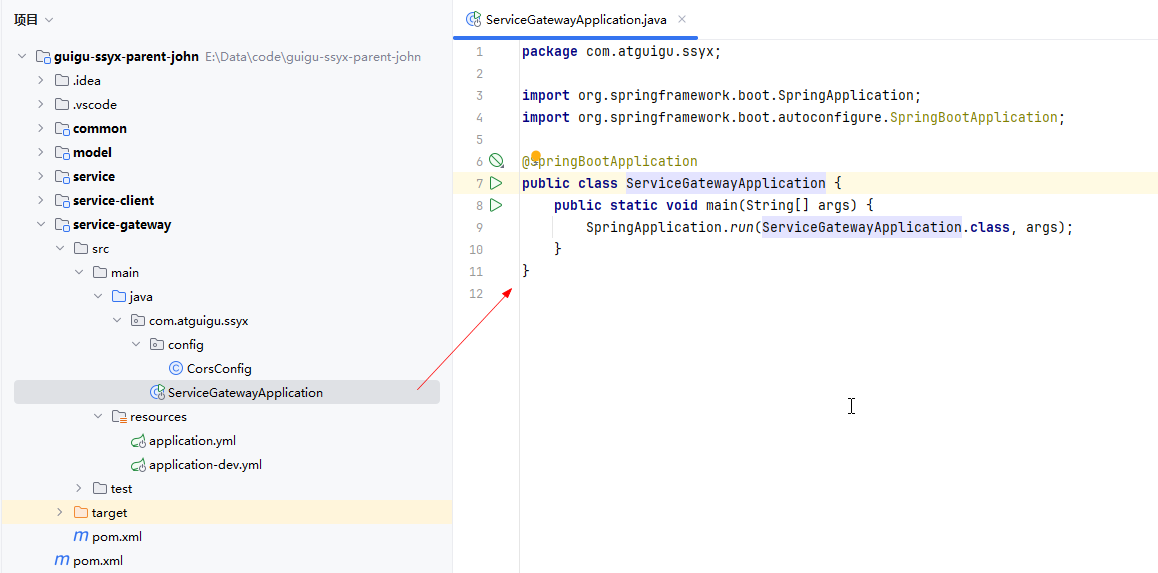
5. 网关相关配置
网关解决跨域问题
跨域不一定都会有跨域问题。因为跨域问题是浏览器对于ajax请求的一种安全限制:一个页面发起的ajax请求,只能是与当前页域名相同的路径,这能有效的阻止跨站攻击。因此:跨域问题 是针对ajax的一种限制。
但是这却给我们的开发带来了不便,而且在实际生产环境中,肯定会有很多台服务器之间交互,地址和端口都可能不同。
创建配置类
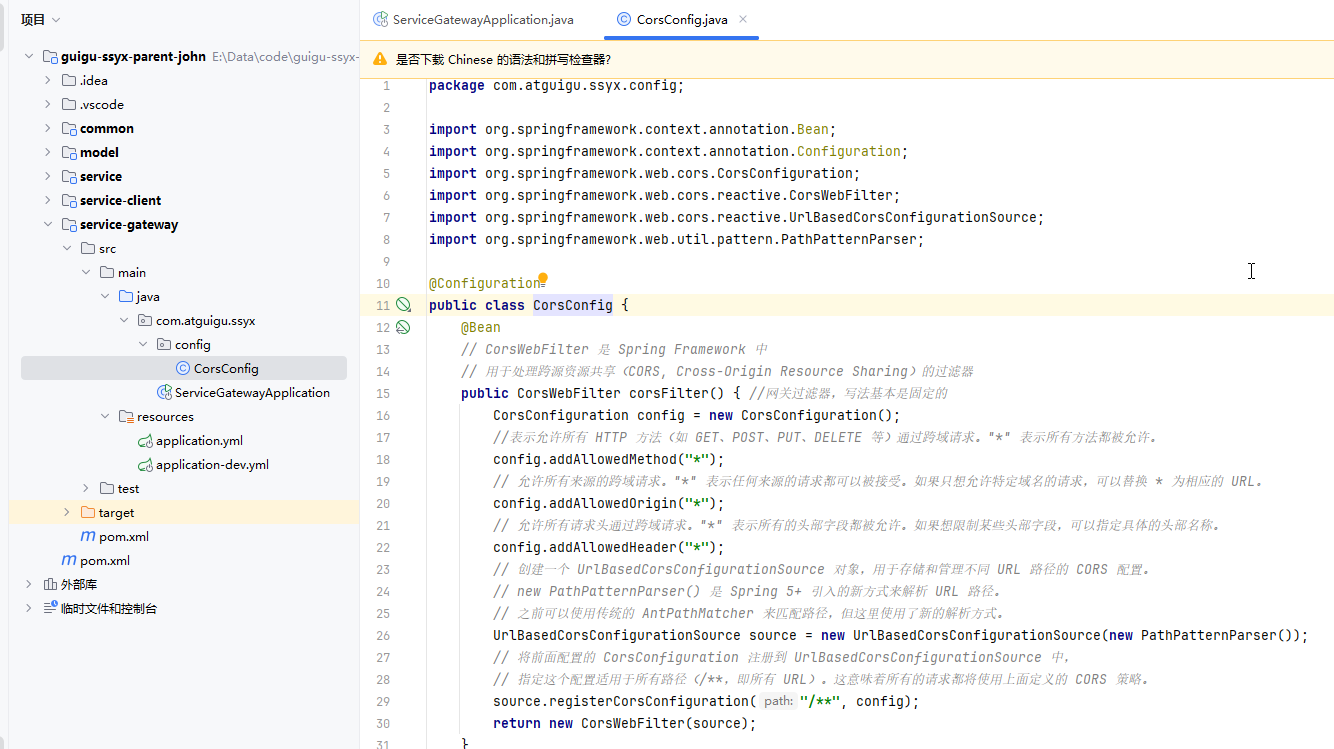
java
package com.atguigu.ssyx.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.cors.CorsConfiguration;
import org.springframework.web.cors.reactive.CorsWebFilter;
import org.springframework.web.cors.reactive.UrlBasedCorsConfigurationSource;
import org.springframework.web.util.pattern.PathPatternParser;
@Configuration
public class CorsConfig {
@Bean
// CorsWebFilter 是 Spring Framework 中
// 用于处理跨源资源共享(CORS, Cross-Origin Resource Sharing)的过滤器
public CorsWebFilter corsFilter() { //网关过滤器,写法基本是固定的
CorsConfiguration config = new CorsConfiguration();
//表示允许所有 HTTP 方法(如 GET、POST、PUT、DELETE 等)通过跨域请求。"*" 表示所有方法都被允许。
config.addAllowedMethod("*");
// 允许所有来源的跨域请求。"*" 表示任何来源的请求都可以被接受。如果只想允许特定域名的请求,可以替换 * 为相应的 URL。
config.addAllowedOrigin("*");
// 允许所有请求头通过跨域请求。"*" 表示所有的头部字段都被允许。如果想限制某些头部字段,可以指定具体的头部名称。
config.addAllowedHeader("*");
// 创建一个 UrlBasedCorsConfigurationSource 对象,用于存储和管理不同 URL 路径的 CORS 配置。
// new PathPatternParser() 是 Spring 5+ 引入的新方式来解析 URL 路径。
// 之前可以使用传统的 AntPathMatcher 来匹配路径,但这里使用了新的解析方式。
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource(new PathPatternParser());
// 将前面配置的 CorsConfiguration 注册到 UrlBasedCorsConfigurationSource 中,
// 指定这个配置适用于所有路径(/**,即所有 URL)。这意味着所有的请求都将使用上面定义的 CORS 策略。
source.registerCorsConfiguration("/**", config);
return new CorsWebFilter(source);
}
}
目前我们已经在网关做了跨域处理,那么service服务就不需要再做跨域处理了,将之前在controller类上添加过**@CrossOrigin**标签的去掉,防止程序异常.。示例如下:
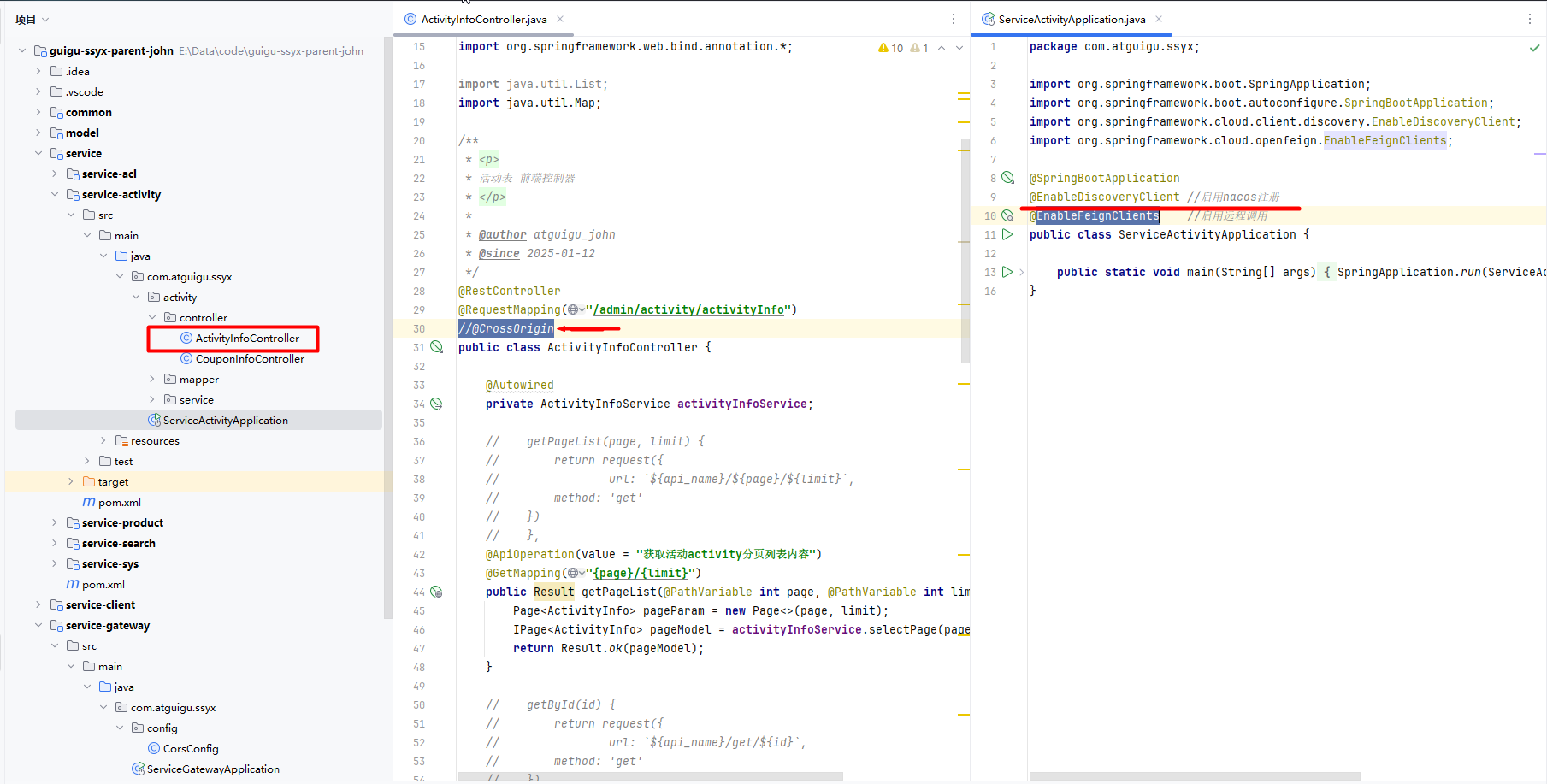
6. 修改前端路径
修改.env.development 文件,修改为网关路径
XML
# just a flag
ENV = 'development'
# base api
# VUE_APP_BASE_API = '/dev-api'
# 修改为网关路径
VUE_APP_BASE_API = 'http://localhost:8200' # 8200为自定义的路径