一、三维向量Vector3与模型位置
点模型Points
、线模型Line
、网格网格模型Mesh
等模型对象的父类都是Object3D,如果想对这些模型进行旋转、缩放、平移等操作,如何实现,可以查询Threejs文档Object3D对相关属性和方法的介绍
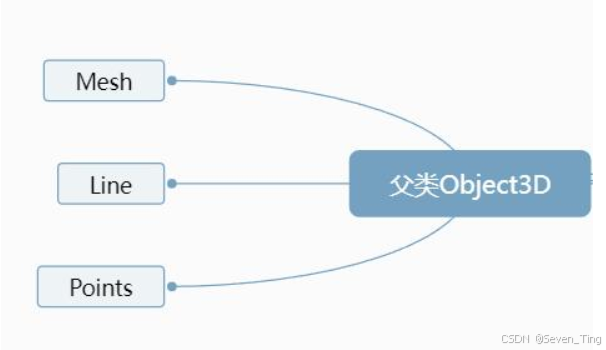
1、三维向量Vector3
三维向量Vector3
有xyz三个分量,threejs中会用三维向量Vector3
表示很多种数据,本节课提到的比较简单,就是位置.position
和缩放.scale
属性。
查看three.js文档你可以知道Vector3
对象具有属性.x
、.y
、.z
,Vector3
对象还具有.set()
等方法。
javascript
const v3 = new THREE.Vector3(100,100,100);
v3.set(50,50,50)
console.log('sad',v3);

2、位置属性.position
javascript
// 位置属性.position使用threejs三维向量对象Vector3表示的
console.log('模型位置属性.position的值', mesh.position);
模型位置.position
属性用一个三维向量表示,那意味着,以后你想改变位置属性,就应该查询文档Vector3。
这里之所以强调这一点,课程目的不单单是给你介绍具体的知识点,也是在引导你学会查文档,降低记忆压力。
3、改变位置属性
通过模型位置属性.position
可以设置模型在场景Scene中的位置。模型位置.position
的默认值是THREE.Vector3(0.0,0.0,0.0)
,表示坐标原点。
设置网格模型y坐标
javascript
mesh.position.y = 80;
设置模型xyz坐标
javascript
mesh.position.set(80,2,10);
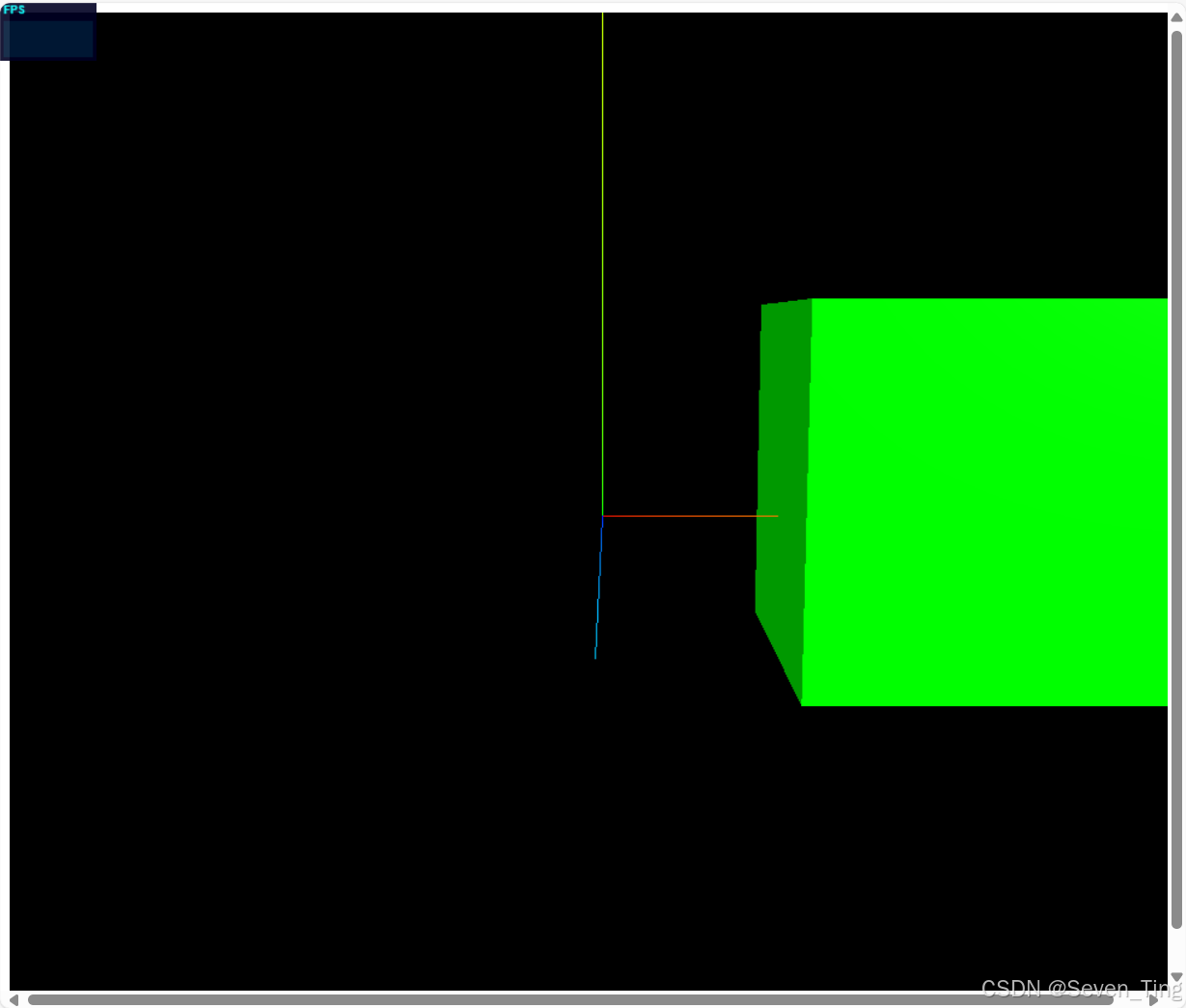
4、缩放
属性.scale
表示模型对象的xyz三个方向上的缩放比例,.scale
的属性值是一个三维向量对象Vector3
,默认值是THREE.Vector3(1.0,1.0,1.0)
。
轴方向放大2倍
javascript
mesh.scale.x = 2.0;
网格模型xyz方向分别缩放0.5,1.5,2倍
javascript
mesh.scale.set(2, 1.5, 2)
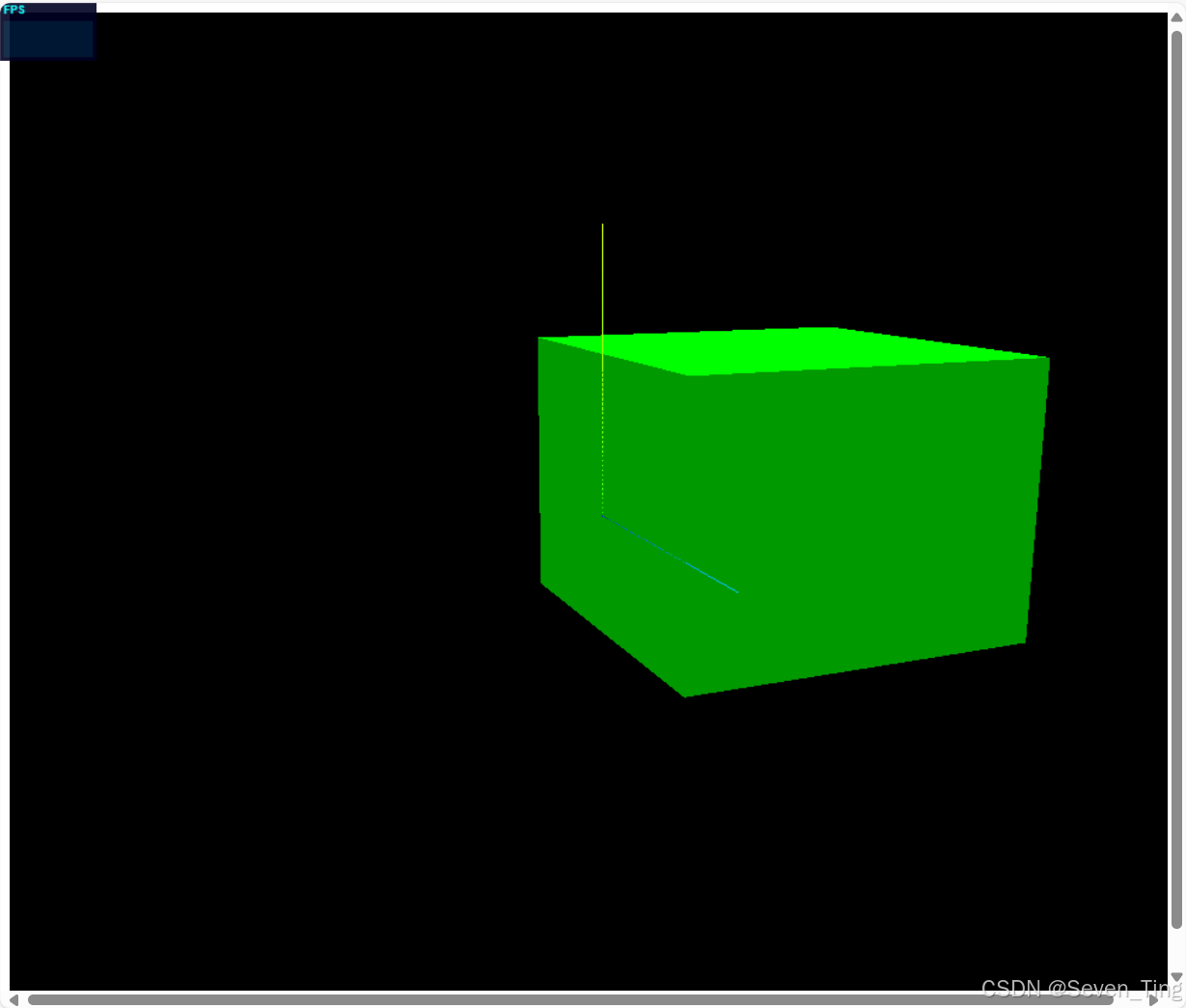
5、平移
执行.translateX()
、.translateY()
等方法本质上改变的都是模型的位置属性.position
。
网格模型沿着x轴正方向平移100,可以多次执行该语句,每次执行都是相对上一次的位置进行平移变换。
javascript
// 等价于mesh.position = mesh.position + 100;
mesh.translateX(100);//沿着x轴正方向平移距离100
沿着Z轴负方向平移距离50。
javascript
mesh.translateZ(-50);
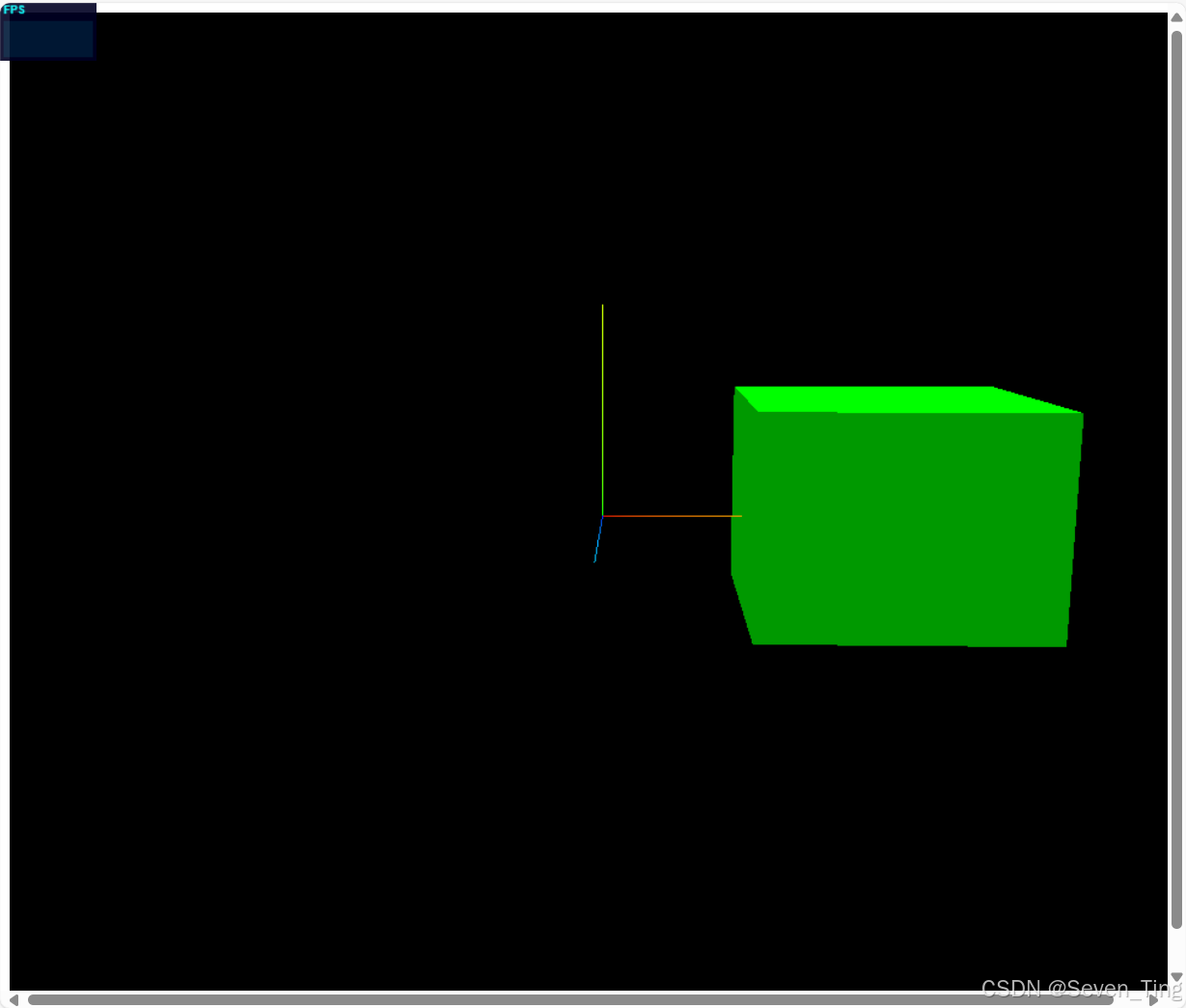
二、欧拉Euler与角度属性.rotation
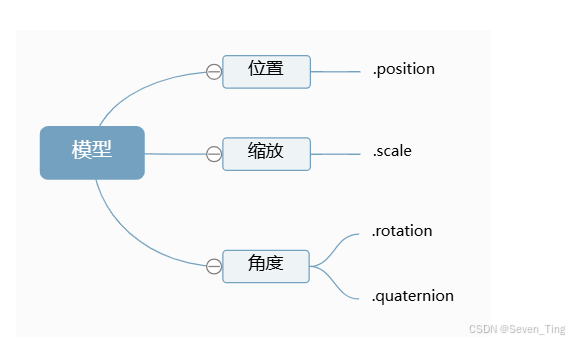
模型的角度属性.rotation
和四元数属性.quaternion
都是表示模型的角度状态,只是表示方法不同,.rotation
属性值是欧拉对象Euler,.quaternion
属性值是是四元数对象Quaternion
大家刚入门,就先给大家介绍比较容易理解的角度属性.rotation
和对应属性值欧拉对象Euler
1、欧拉对象Euler
javascript
// 创建一个欧拉对象,表示绕着xyz轴分别旋转45度,0度,90度
const Euler = new THREE.Euler( Math.PI/4,0, Math.PI/2);
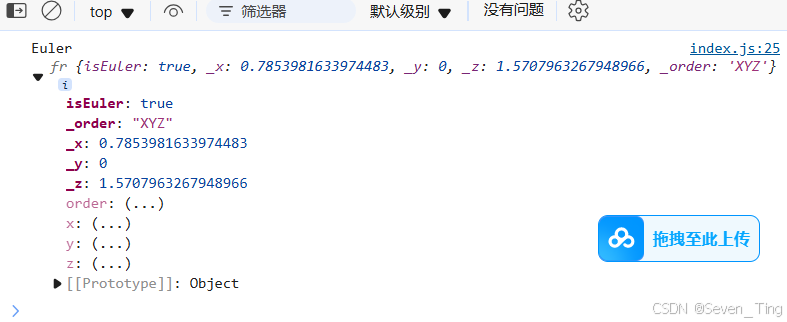
通过属性设置欧拉对象的三个分量值。
javascript
const Euler = new THREE.Euler();
Euler.x = Math.PI/4;
Euler.y = Math.PI/2;
Euler.z = Math.PI/4;
2、改变角度属性.rotation
角度属性.rotation
的值是欧拉对象Euler
,意味着你想改变属性.rotation
,可以查询文档关于Euler
类的介绍。
javascript
//绕y轴的角度设置为60度
mesh.rotation.y += Math.PI/4;
//绕y轴的角度增加60度
mesh.rotation.y += Math.PI/4;
//绕y轴的角度减去60度
mesh.rotation.y -= Math.PI/4;
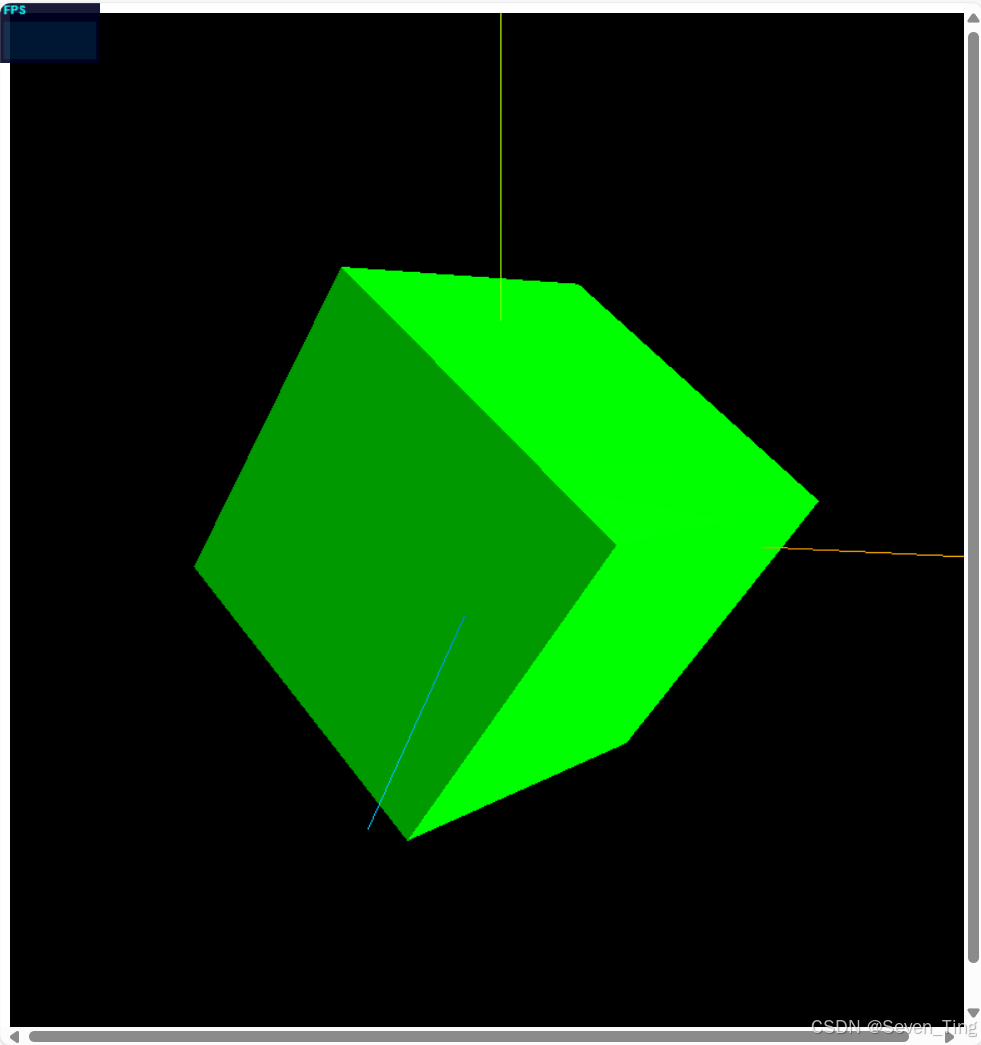
3、旋转方法.rotateX()
、.rotateY()
、.rotateZ()
模型执行.rotateX()
、.rotateY()
等旋转方法,你会发现改变了模型的角度属性.rotation
。
javascript
mesh.rotateX(Math.PI/4);//绕x轴旋转π/4
javascript
// 绕着Y轴旋转90度
mesh.rotateY(Math.PI / 2);
//控制台查看:旋转方法,改变了rotation属性
console.log(mesh.rotation);
javascript
cube.rotateX(0.5)
cube.rotateY(0.5)
cube.rotateZ(0.5)
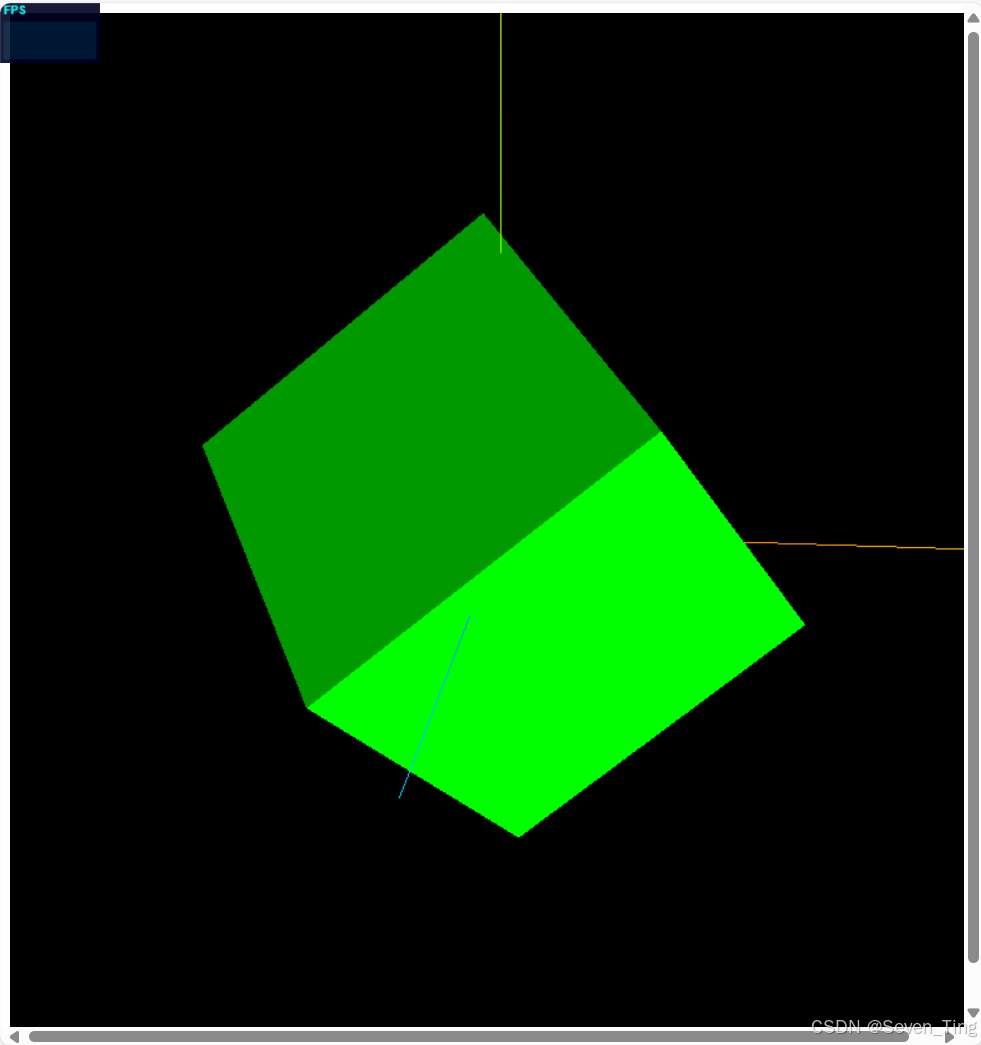
4、旋转动画
javascript
function render() {
cube.rotation.y += 0.01
renderer.render(scene, camera)
requestAnimationFrame(render)
}
render()
javascript
function render() {
cube.rotateY(0.01)
renderer.render(scene, camera)
requestAnimationFrame(render)
}
render()
5、绕某个轴旋转
网格模型绕(0,1,0)
向量表示的轴旋转π/8
。
javascript
const axis = new THREE.Vector3(0,1,0);//向量axis
mesh.rotateOnAxis(axis,Math.PI/8);//绕axis轴旋转π/8