一、准备工作
注册与登录:
登录百度智能云千帆控制台,注册并登录您的账号。
创建千帆应用:
根据实际需求创建千帆应用。创建成功后,获取AppID、API Key、Secret Key等信息。如果已有千帆应用,可以直接查看已有应用的API Key、Secret Key等信息。
API授权:
应用创建成功后,千帆平台默认为应用开通所有API调用权限,无需额外申请授权。但请注意,针对付费服务,如果用户在使用过程中终止了付费,则无法调用对应的API。如需重新开通,请在千帆大模型平台-在线服务页面点击开通付费。
二、获取接口访问凭证access_token
调用获取access_token接口:
使用API Key和Secret Key调用获取access_token接口,获取access_token。这个token是调用API接口的身份验证凭证,需要妥善保管。access_token默认有效期为30天,生产环境请注意及时刷新。
三、调用API接口
构造请求:
根据API文档构造请求,包括设置请求参数、请求头等。其中,prompt是与大模型对话的入口,其质量直接决定了大模型的输出质量。因此,需要编写高质量的prompt。
发送请求:
将构造好的请求发送到API服务器。这通常是通过HTTP请求完成的。
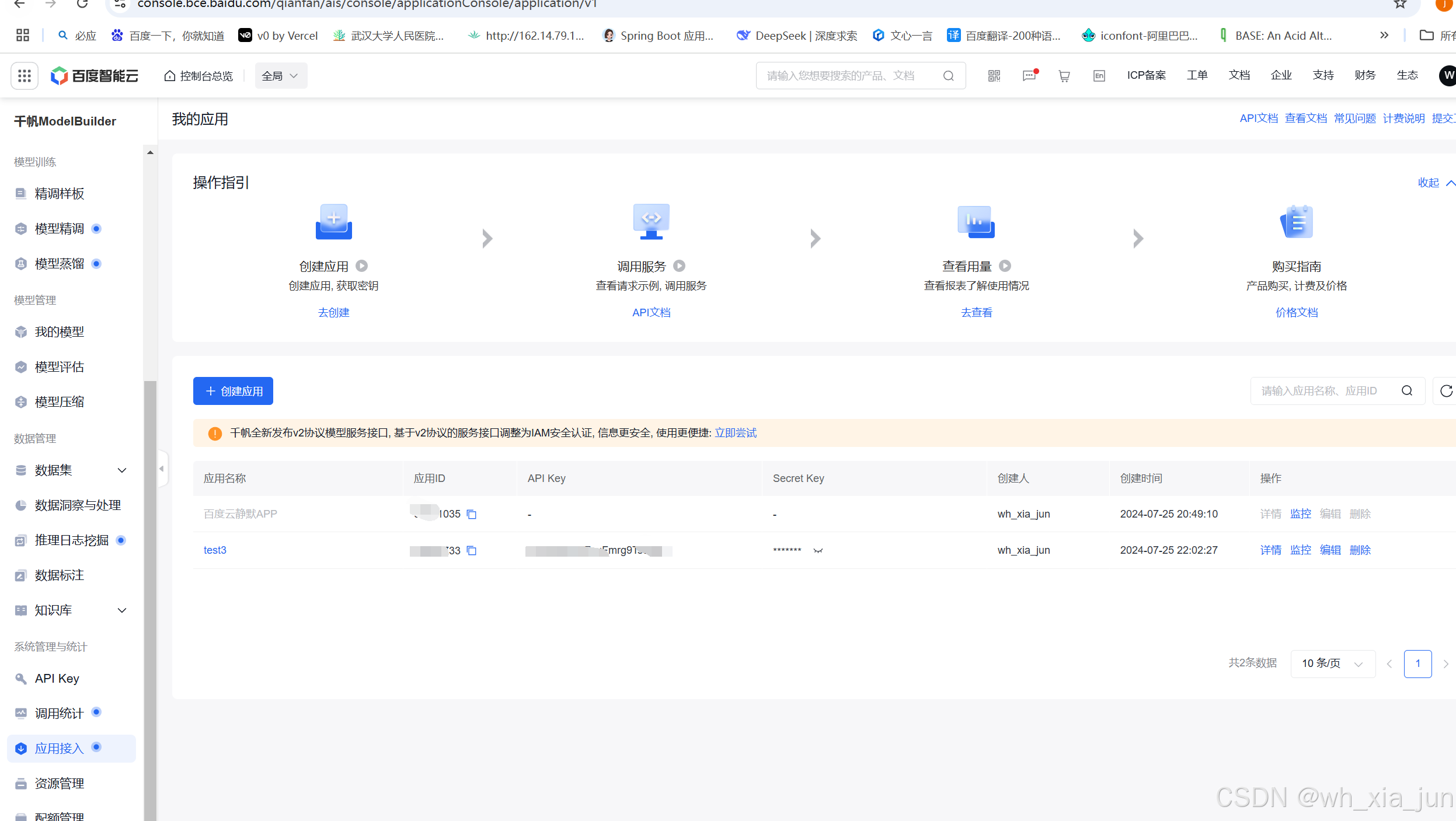
java 调用的代码如下:
package com.mg.mgchat.service;
//import org.apache.http.HttpResponse;
//import org.apache.http.client.methods.HttpPost;
//import org.apache.http.entity.StringEntity;
//import org.apache.http.impl.client.CloseableHttpClient;
//import org.apache.http.impl.client.HttpClients;
//import org.apache.http.util.EntityUtils;
import okhttp3.*;
import org.json.JSONArray;
import org.json.JSONObject;
import org.springframework.stereotype.Service;
import java.io.IOException;
@Service
public class ErnieSpeedService {
public static final String API_KEY = "****"; //修改为自己的
public static final String SECRET_KEY = "***;
static final OkHttpClient HTTP_CLIENT = new OkHttpClient().newBuilder().build();
static final String orgDescStr="湖北长江电影集团是一家致力于电影产业的企业,涵盖了电影的制作、发行和放映等多个环节。关于湖北长江电影集团放映公益电影的情况,这是一个值得赞赏的举措。在社会责任感的驱使下,许多电影公司都会参与公益电影的放映,旨在为广大观众提供有益的文化内容,同时促进社会发展。湖北长江电影集团可能会定期或不定期地参与放映公益电影,这可能包括安全教育、文化传承、环境保护等主题的电影。这些公益电影的放映可能是在电影院、社区、学校或其他公共场所进行,以最大限度地覆盖更多的观众。要了解湖北长江电影集团具体的公益电影放映情况,建议访问其官方网站或关注其社交媒体账号,以获取最新的信息。同时,也可以关注当地的新闻资讯,以了解更多的放映详情。";
public JSONObject askQuestion(String question) throws Exception {
MediaType mediaType = MediaType.parse("application/json");
JSONObject r = new JSONObject();
//declare json object array
JSONArray jsonArray = new JSONArray();
JSONObject jsonObject1 = new JSONObject();
jsonObject1.put("role", "user");
jsonObject1.put("content", "湖北长江电影集团放映公益电影");
jsonArray.put(jsonObject1);
JSONObject jsonObject2 = new JSONObject();
jsonObject2.put("role", "assistant");
jsonObject2.put("content", orgDescStr);
jsonArray.put(jsonObject2);
r.put("orgDesc", orgDescStr);
JSONObject jsonObject3 = new JSONObject();
jsonObject3.put("role", "user");
jsonObject3.put("content", "电影:" + question);
jsonArray.put(jsonObject3);
JSONObject jsonObject_q = new JSONObject();
jsonObject_q.put("messages", jsonArray);
int i = 0;
while (i <= 2) {
i++;
String json_question = jsonObject_q.toString();
RequestBody body = RequestBody.create(mediaType, json_question);
Request request = new Request.Builder()
.url("https://aip.baidubce.com/rpc/2.0/ai_custom/v1/wenxinworkshop/chat/ernie-speed-128k?access_token=" + getAccessToken())
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = HTTP_CLIENT.newCall(request).execute();
String s = response.body().string();
JSONObject jsonObject_r1 = new JSONObject(s);
String s1 = jsonObject_r1.getString("result");
if (i == 1) {
r.put("filmDesc", s1); // film desc save to r json object
JSONObject jsonObject4 = new JSONObject();
jsonObject4.put("role", "assistant");
jsonObject4.put("content", s1); // film desc
jsonArray.put(jsonObject4); // add to json array ,to prepare for next query
JSONObject jsonObject5 = new JSONObject(); // prepare for next query ,user query
jsonObject5.put("role", "user");
jsonObject5.put("content", "电影:" + question + "的主要演员有哪些,他们的演技怎么样,都演过什么电影");
jsonArray.put(jsonObject5);
} else if (i == 2) {
r.put("performerDesc", s1); // film desc save to r json object
}
}
return r;
}
/**
* 从用户的AK,SK生成鉴权签名(Access Token)
*
* @return 鉴权签名(Access Token)
* @throws IOException IO异常
*/
static String getAccessToken() throws IOException {
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "grant_type=client_credentials&client_id=" + API_KEY
+ "&client_secret=" + SECRET_KEY);
Request request = new Request.Builder()
.url("https://aip.baidubce.com/oauth/2.0/token")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = HTTP_CLIENT.newCall(request).execute();
return new JSONObject(response.body().string()).getString("access_token");
}
}
测试代码:
package com.mg.mgchat.service;
import okhttp3.*;
import org.json.JSONObject;
import java.io.*;
/**
* 需要添加依赖
* <!-- https://mvnrepository.com/artifact/com.squareup.okhttp3/okhttp -->
* <dependency>
* <groupId>com.squareup.okhttp3</groupId>
* <artifactId>okhttp</artifactId>
* <version>4.12.0</version>
* </dependency>
*/
class Sample {
public static final String API_KEY = "***";
public static final String SECRET_KEY = "***";
static final OkHttpClient HTTP_CLIENT = new OkHttpClient().newBuilder().build();
public static void main(String []args) throws IOException{
MediaType mediaType = MediaType.parse("application/json");
// {
// "role": "assistant",
// "content": "这是一个测试信息。请问有什么我可以帮助您的吗?"
// }, it is string parameter
//String s={"messages":[{"role":"user","content":"test"}]}
String json = "{\"messages\":[{\"role\":\"user\",\"content\":\"辽沈战役类似的电影有哪些\"},{\"role\":\"assistant\",\"content\":\"与辽沈战役相关的电影有很多,以下是一些类似的电影:\\n\\n1. 《大决战》:这是一部非常知名的战争电影,描述了辽沈战役的全过程,再现了中国共产党的英勇奋斗和伟大胜利。\\n2. 《辽沈战役之鹰击长空》:这部电影描绘了空军在辽沈战役中的重要作用,展现了空军的英勇无畏和战争中的艰苦历程。\\n3. 《风雨辽沈》:这部电影展现了辽沈战役期间的复杂局势和人物关系,描绘了一幅生动的历史画卷。\\n4. 《决战辽沈》:这部电影聚焦于辽沈战役的重要战役和事件,再现了战争中的关键时刻和英勇事迹。\\n5. 《中国蓝军大决战》:这部电影以辽沈战役为背景,展现了人民解放军的战斗精神和英勇无畏。\\n\\n除了以上电影,还有一些其他战争题材的电影,如《建党伟业》、《辛亥革命》等,虽然不直接描述辽沈战役,但也涉及到相关的历史事件和人物。\\n\\n以上内容仅供参考,如需更多关于辽沈战役的电影信息,可以在各大影视网站或App上查询。\"},{\"role\":\"user\",\"content\":\"推荐一部电影\"}]}";
RequestBody body = RequestBody.create(mediaType, json);
//RequestBody body = RequestBody.create(mediaType, "{\"messages\":[{\"role\":\"user\",\"content\":\"辽沈战役类似的电影有哪些\"}\\,{\"role\":\"assistant\",\"content\":\"与辽沈战役相关的电影有很多,以下是一些类似的电影:\\n\\n1. 《大决战》:这是一部非常知名的战争电影,描述了辽沈战役的全过程,再现了中国共产党的英勇奋斗和伟大胜利。\\n2. 《辽沈战役之鹰击长空》:这部电影描绘了空军在辽沈战役中的重要作用,展现了空军的英勇无畏和战争中的艰苦历程。\\n3. 《风雨辽沈》:这部电影展现了辽沈战役期间的复杂局势和人物关系,描绘了一幅生动的历史画卷。\\n4. 《决战辽沈》:这部电影聚焦于辽沈战役的重要战役和事件,再现了战争中的关键时刻和英勇事迹。\\n5. 《中国蓝军大决战》:这部电影以辽沈战役为背景,展现了人民解放军的战斗精神和英勇无畏。\\n\\n除了以上电影,还有一些其他战争题材的电影,如《建党伟业》、《辛亥革命》等,虽然不直接描述辽沈战役,但也涉及到相关的历史事件和人物。\\n\\n以上内容仅供参考,如需更多关于辽沈战役的电影信息,可以在各大影视网站或App上查询。\"}\\,{\"role\":\"user\",\"content\":\"推荐一部电影\"}]}");
Request request = new Request.Builder()
.url("https://aip.baidubce.com/rpc/2.0/ai_custom/v1/wenxinworkshop/chat/ernie-speed-128k?access_token=" + getAccessToken())
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = HTTP_CLIENT.newCall(request).execute();
System.out.println(response.body().string());
}
/**
* 从用户的AK,SK生成鉴权签名(Access Token)
*
* @return 鉴权签名(Access Token)
* @throws IOException IO异常
*/
static String getAccessToken() throws IOException {
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "grant_type=client_credentials&client_id=" + API_KEY
+ "&client_secret=" + SECRET_KEY);
Request request = new Request.Builder()
.url("https://aip.baidubce.com/oauth/2.0/token")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = HTTP_CLIENT.newCall(request).execute();
return new JSONObject(response.body().string()).getString("access_token");
}
}
ernie-speed-128k
在URL中指的是使用的具体模型名称或标识符。ERNIE(Enhanced Representation through kNowledge IntEgration)是百度开发的一系列预训练语言模型,它们经过训练能够理解自然语言文本,并根据上下文生成回答。speed-128k
可能表示这个模型在某种性能指标(如推理速度)上的优化,或者是模型的某个特定版本。
用python 写一个调用:
先测试一下
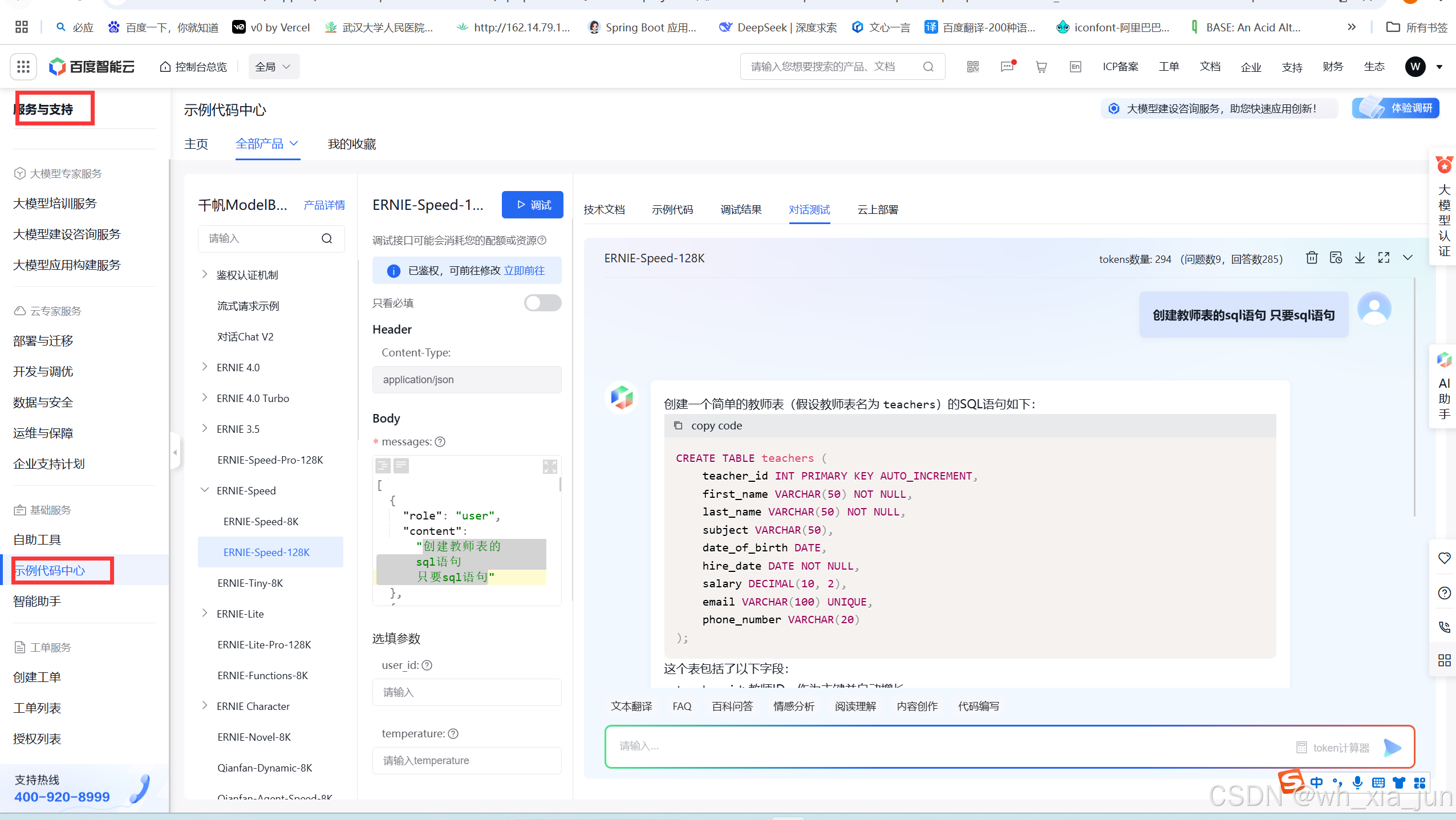
官网还有示例代码 可以直接copy
这里我们写一个通过ai 执行sql的py 小程序:
完整代码如下:
python
import tkinter as tk
from tkinter import messagebox
import requests
import pymysql
import json
import re
# 百度文言一心大模型API的配置
API_KEY = '****' #更换为自己的
SECRET_KEY = '***'
TOKEN_URL = f'https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id={API_KEY}&client_secret={SECRET_KEY}'
# MySQL数据库的配置
DB_HOST = 'localhost'
DB_USER = 'root'
DB_PASSWORD = '1'
DB_NAME = 'xx'
def get_access_token():
"""获取百度API的访问令牌"""
response = requests.get(TOKEN_URL)
return response.json().get('access_token')
# 提取SQL语句 只能是ddl
def extract_sql(response_text):
"""从API响应中提取SQL语句"""
# 使用正则表达式匹配SQL代码块
match = re.search(r'```sql\n([\s\S]*?)\n```', response_text, re.DOTALL)
if match:
return match.group(1).strip()
else:
raise ValueError("无法从响应中提取SQL语句")
def generate_sql(prompt, access_token):
"""调用百度文言一心大模型API生成SQL语句"""
url = "https://aip.baidubce.com/rpc/2.0/ai_custom/v1/wenxinworkshop/chat/ernie-speed-128k?access_token=" + get_access_token()
payload = json.dumps({
"messages": [
{
"role": "user",
"content": prompt
}
]
}, ensure_ascii=False)
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload.encode("utf-8"))
response_text = response.text
print(response.text)
# headers = {
# 'Content-Type': 'application/json',
# 'Authorization': f'Bearer {access_token}'
# }
# data = {
# "messages": [
# {
# "role": "user",
# "content": prompt
# }
# ]
# }
# response = requests.post(url, headers=headers, json=data)
if response.status_code == 200:
try:
#把response_text 转换为json对象
response_json = json.loads(response_text)
#get result from response_json
if "error_code" in response_json:
raise Exception(f"API请求失败: {response_json['error_code']}, {response_json['error_msg']}")
if "result" not in response_json:
raise Exception("API响应中缺少'result'字段")
result_text=response_json["result"];
sql = extract_sql(result_text)
return sql
except ValueError as e:
raise Exception(f"无法解析API响应: {e}")
else:
raise Exception(f"API请求失败: {response.status_code}, {response.text}")
def execute_sql(sql):
"""执行SQL语句"""
connection = pymysql.connect(host=DB_HOST, user=DB_USER, password=DB_PASSWORD, database=DB_NAME)
try:
with connection.cursor() as cursor:
cursor.execute(sql)
result = cursor.fetchall()
connection.commit()
return result
finally:
connection.close()
def on_submit():
"""用户提交操作要求时的回调函数"""
# 获取用户输入
prompt = entry.get()
if not prompt:
messagebox.showwarning("输入错误", "请输入对数据库操作的要求!")
return
try:
# 获取访问令牌
access_token = get_access_token()
# 调用API生成SQL语句
sql = generate_sql(prompt, access_token)
sql_text.delete(1.0, tk.END) # 清空之前的SQL语句
sql_text.insert(tk.END, sql) # 显示生成的SQL语句
# 询问用户是否执行
confirm = messagebox.askyesno("确认执行", f"生成的SQL语句为:\n{sql}\n\n是否执行?")
if confirm:
result = execute_sql(sql)
result_text.delete(1.0, tk.END) # 清空之前的结果
result_text.insert(tk.END, "执行成功,结果为:\n")
for row in result:
result_text.insert(tk.END, f"{row}\n")
except Exception as e:
messagebox.showerror("错误", f"发生错误: {e}")
# 创建主窗口
root = tk.Tk()
root.title("数据库操作助手")
# 用户输入框
tk.Label(root, text="请输入对数据库操作的要求:").pack(pady=5)
entry = tk.Entry(root, width=50)
entry.pack(pady=5)
# 提交按钮
submit_button = tk.Button(root, text="提交", command=on_submit)
submit_button.pack(pady=10)
# 显示生成的SQL语句
tk.Label(root, text="生成的SQL语句:").pack(pady=5)
sql_text = tk.Text(root, height=5, width=50)
sql_text.pack(pady=5)
# 显示执行结果
tk.Label(root, text="执行结果:").pack(pady=5)
result_text = tk.Text(root, height=10, width=50)
result_text.pack(pady=5)
# 运行主循环
root.mainloop()
界面:
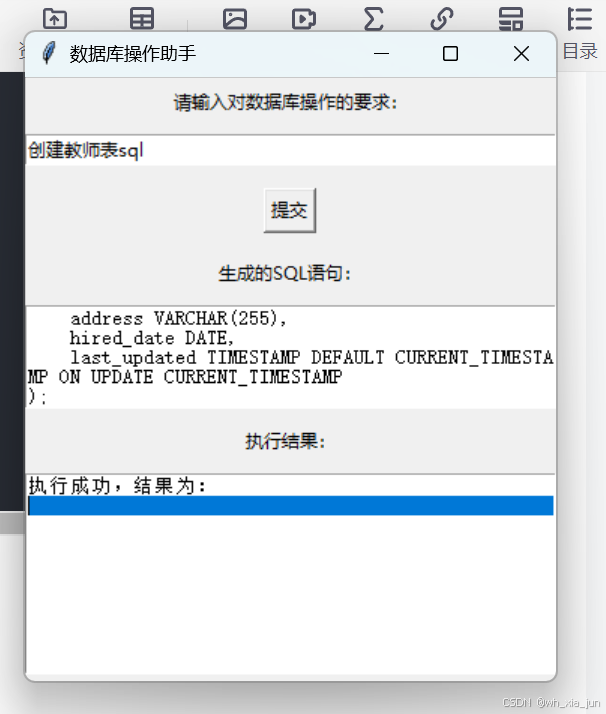
执行结果是创建了表:
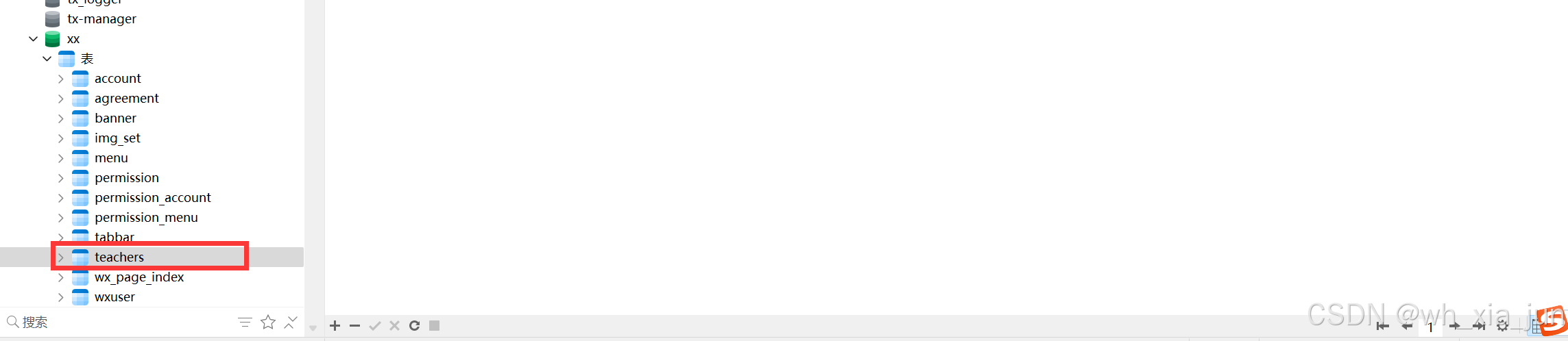