原理图
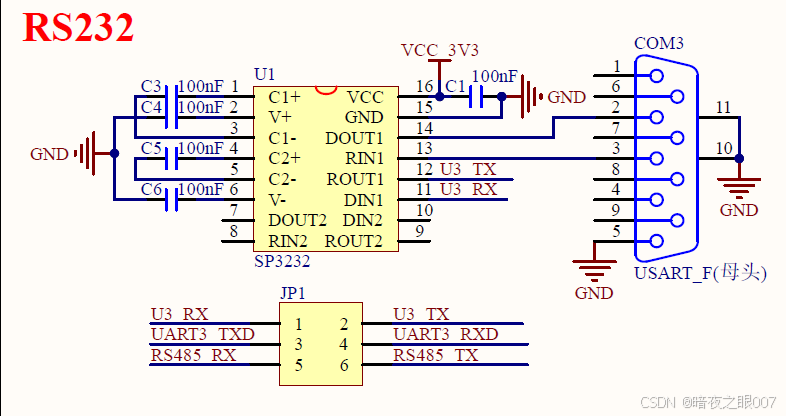
从上图可以看到RS232的串口接的是UART3,接下来我们需要使能UART3的收发功能。一般串口的驱动程序在内核中都有包含,我们配置使能适配即可。
设备树
复用功能配置
查看6ull如何进行uart3的串口复用配置:

设备树下添加uart3的串口复用配置:
cpp
pinctrl_uart3: uart3grp {
fsl,pins = <
MX6UL_PAD_UART3_RX_DATA__UART3_DTE_TX 0x1b0b1
MX6UL_PAD_UART3_RX_DATA__UART3_DCE_RX 0x1b0b1
>;
};
添加设备树节点
设备树下添加uart3的设备树节点:
cpp
&uart3 {
pinctrl-names = "default";
pinctrl-0 = <&pinctrl_uart3>;
status = "okay";
};
重新编译设备树make dtbs,并拷贝到开发板对应位置替换之前的设备树文件。
查看串口功能配置
重启开发板后,查看配置是否生效:

由上图看出UART3功能配置已经生效。
串口应用编程
应用层代码参考
cpp
#include <unistd.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <termios.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <pthread.h>
#include <sys/ioctl.h>
#include <errno.h>
#define ttyname "/dev/ttymxc2"
int fd;
static char wbuff[128];
static char rbuff[128];
void *read_handler(void * arg){
int ret;
while(1){
memset(rbuff, 0, sizeof(rbuff));
ret = read(fd, rbuff, sizeof(rbuff));
if(ret == -1 ){
perror("read");
close(fd);
pthread_exit(NULL);
}else if(ret > 0){
printf("RCV: %s\n", rbuff);
fflush(stdout);
}
}
pthread_exit(NULL);
}
int main(int argc, char **argv){
int ret;
pthread_t thread;
struct termios tty;
fd = open(ttyname, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1){// 打开端口失败
perror("open_port: Unable to open /dev/tty ");
}
if(fcntl(fd, F_SETFL, 0)<0) /* 设置串口为阻塞状态*/
{
printf("fcntl failed!\n");
close(fd);
return -1;
}
// 获取当前串口配置
memset(&tty, 0, sizeof(tty));
if (tcgetattr(fd, &tty) != 0) {
perror("Error from tcgetattr: ");
}
// 配置波特率
cfsetispeed(&tty, B115200);
cfsetospeed(&tty, B115200);
// 配置数据位、停止位和校验
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8; // 8数据位
tty.c_cflag &= ~CSTOPB; // 1停止位
tty.c_cflag &= ~PARENB; // 无校验位
/*设置等待时间和最小接收字符*/
tty.c_cc[VTIME] = 1;
tty.c_cc[VMIN] = 1;
tty.c_cflag |= CREAD | CLOCAL; // 开启接收,忽略modem控制线
// tty.c_iflag = IGNPAR | ICRNL; // 忽略校验错误,输入时将回车转换为换行
tty.c_oflag = ~(ONLCR | ICRNL); //
tty.c_iflag &= ~(INLCR | ICRNL |IGNCR); //
tty.c_oflag &= ~OPOST;
/*处理未接收字符*/
tcflush(fd,TCIFLUSH);
// 设置串口配置
tcsetattr(fd, TCSANOW, &tty);
pthread_create(&thread, NULL, read_handler, NULL);
while(1){
memset(wbuff, 0 , sizeof(wbuff));
printf("请输入发送数据:\n");
scanf("%s", wbuff);
//printf("wbuff:%s\n", wbuff);
strncpy(wbuff + strlen(wbuff) - 2, "\r\n", 2);
ret = write(fd, wbuff, strlen(wbuff)+2);
if(ret == -1){
perror("write");
close(fd);
return EXIT_FAILURE;
}
printf("Write success %d\n",ret);
}
pthread_join(thread, NULL);
close(fd);
return 0;
}
编译没报错:

最后拷贝到开发板进行验证
开发板验证
开发板接好线,PC上开启串口助手进行功能验证:
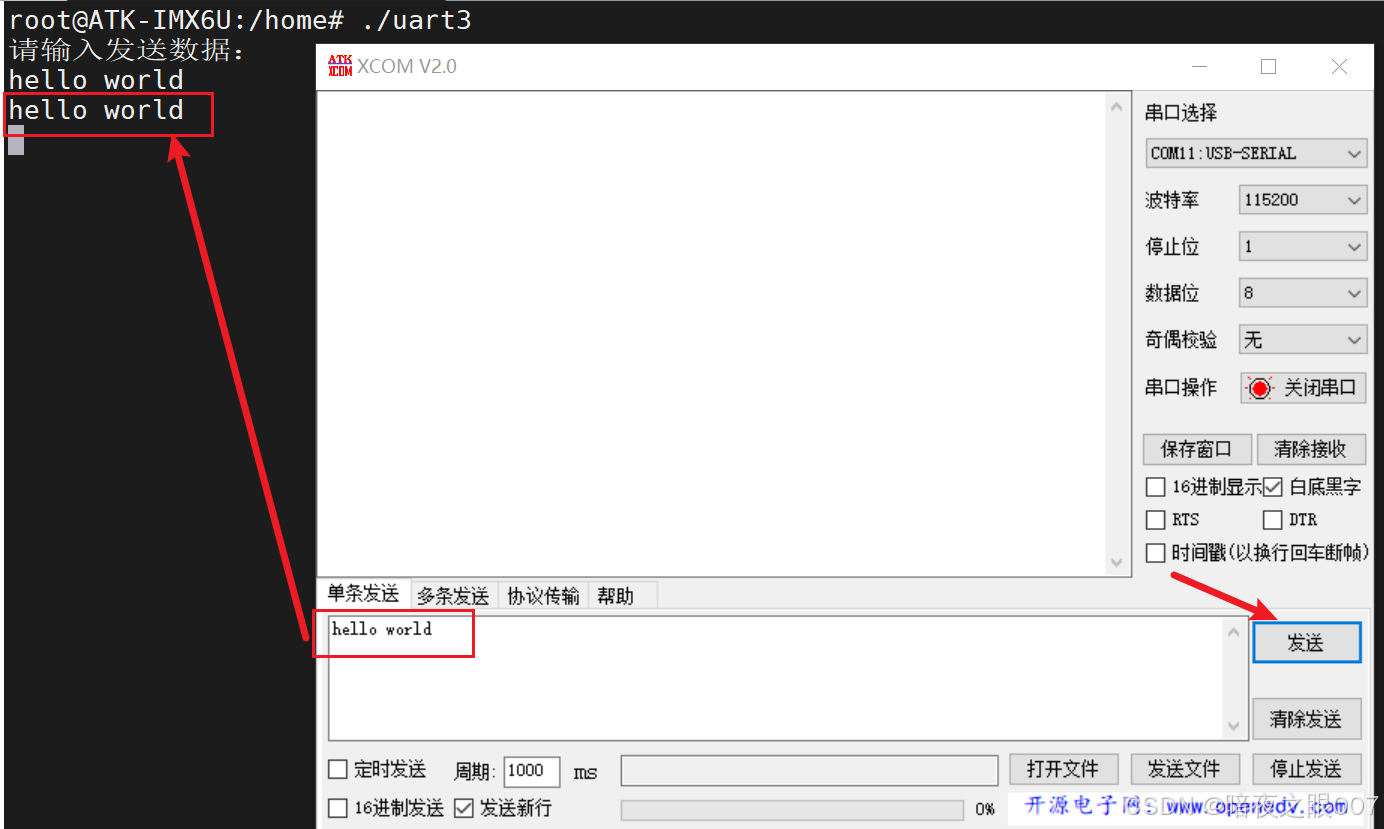