title: FastAPI 表单参数与文件上传完全指南:从基础到高级实战 🚀
date: 2025/3/8
updated: 2025/3/8
author: cmdragon
excerpt:
本教程深入探讨 FastAPI 表单参数与文件上传的核心机制,涵盖从基础表单处理到文件上传的高级用法。通过详细的代码示例、课后测验和常见错误解决方案,帮助初学者快速掌握 FastAPI 表单参数与文件上传的使用技巧。您将学习到如何通过表单参数传递数据、处理文件上传以及优化文件存储和传输,从而构建高效、安全的 API 接口。
categories:
- 后端开发
- FastAPI
tags:
- FastAPI
- 表单参数
- 文件上传
- Form
- File
- API设计
- Web开发
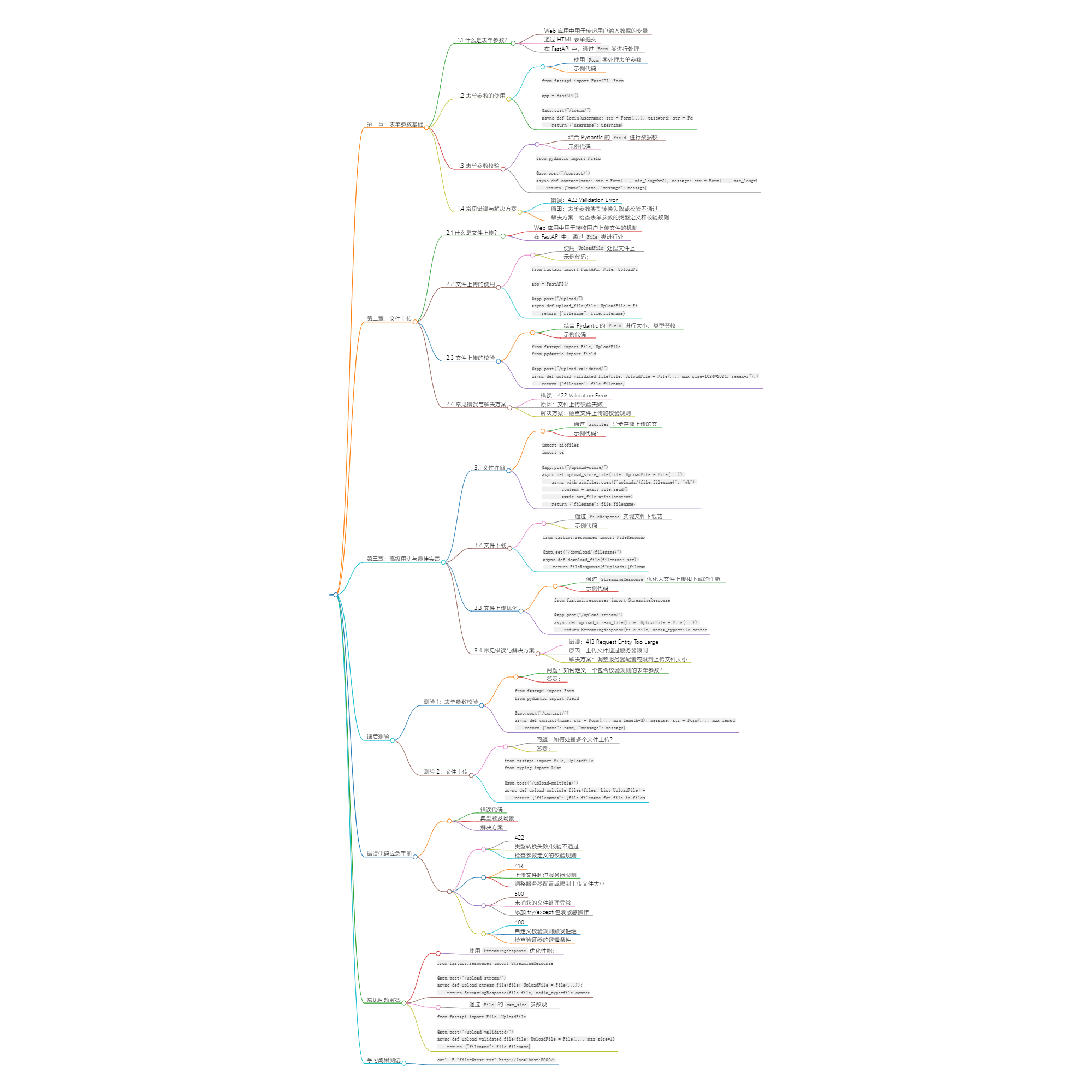
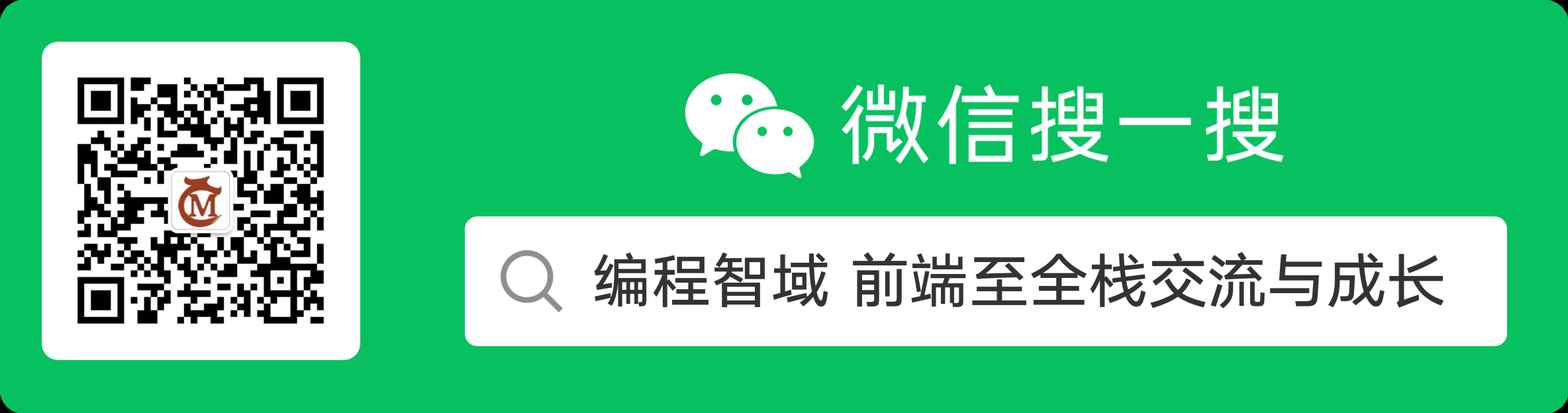
扫描二维码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
第一章:表单参数基础
1.1 什么是表单参数?
表单参数是 Web 应用中用于传递用户输入数据的变量,通常通过 HTML 表单提交。在 FastAPI 中,表单参数可以通过 Form
类进行处理。
python
from fastapi import FastAPI, Form
app = FastAPI()
@app.post("/login/")
async def login(username: str = Form(...), password: str = Form(...)):
return {"username": username}
1.2 表单参数的使用
通过 Form
类,可以轻松处理表单参数。
python
@app.post("/register/")
async def register(name: str = Form(...), email: str = Form(...)):
return {"name": name, "email": email}
示例请求:
json
{
"name": "John Doe",
"email": "[email protected]"
}
1.3 表单参数校验
结合 Pydantic 的 Field
,可以对表单参数进行数据校验。
python
from pydantic import Field
@app.post("/contact/")
async def contact(name: str = Form(..., min_length=3), message: str = Form(..., max_length=1000)):
return {"name": name, "message": message}
示例请求:
- 合法:
{"name": "John", "message": "Hello"}
→ 返回联系信息 - 非法:
{"name": "J", "message": "A" * 1001}
→ 422 错误
1.4 常见错误与解决方案
错误 :422 Validation Error
原因 :表单参数类型转换失败或校验不通过
解决方案:检查表单参数的类型定义和校验规则。
第二章:文件上传
2.1 什么是文件上传?
文件上传是 Web 应用中用于接收用户上传文件的机制。在 FastAPI 中,文件上传可以通过 File
类进行处理。
python
from fastapi import FastAPI, File, UploadFile
app = FastAPI()
@app.post("/upload/")
async def upload_file(file: UploadFile = File(...)):
return {"filename": file.filename}
2.2 文件上传的使用
通过 UploadFile
,可以轻松处理文件上传。
python
@app.post("/upload-multiple/")
async def upload_multiple_files(files: List[UploadFile] = File(...)):
return {"filenames": [file.filename for file in files]}
示例请求:
- 单文件:
curl -F "[email protected]" http://localhost:8000/upload/
→{"filename": "test.txt"}
多文件:curl -F "[email protected]" -F "[email protected]" http://localhost:8000/upload-multiple/
→ {"filenames": ["test1.txt", "test2.txt"]}
2.3 文件上传的校验
结合 Pydantic 的 Field
,可以对上传文件进行大小、类型等校验。
python
from fastapi import File, UploadFile
from pydantic import Field
@app.post("/upload-validated/")
async def upload_validated_file(file: UploadFile = File(..., max_size=1024 * 1024, regex=r"\.(jpg|png)$")):
return {"filename": file.filename}
示例请求:
- 合法:
curl -F "[email protected]" http://localhost:8000/upload-validated/
→{"filename": "test.jpg"}
- 非法:
curl -F "[email protected]" http://localhost:8000/upload-validated/
→ 422 错误
2.4 常见错误与解决方案
错误 :422 Validation Error
原因 :文件上传校验失败
解决方案:检查文件上传的校验规则。
第三章:高级用法与最佳实践
3.1 文件存储
通过 aiofiles
,可以异步存储上传的文件。
python
import aiofiles
import os
@app.post("/upload-store/")
async def upload_store_file(file: UploadFile = File(...)):
async with aiofiles.open(f"uploads/{file.filename}", "wb") as out_file:
content = await file.read()
await out_file.write(content)
return {"filename": file.filename}
3.2 文件下载
通过 FileResponse
,可以实现文件下载功能。
python
from fastapi.responses import FileResponse
@app.get("/download/{filename}")
async def download_file(filename: str):
return FileResponse(f"uploads/{filename}")
示例请求:
- 下载:
http://localhost:8000/download/test.txt
→ 返回文件内容
3.3 文件上传优化
通过 StreamingResponse
,可以优化大文件上传和下载的性能。
python
from fastapi.responses import StreamingResponse
@app.post("/upload-stream/")
async def upload_stream_file(file: UploadFile = File(...)):
return StreamingResponse(file.file, media_type=file.content_type)
3.4 常见错误与解决方案
错误 :413 Request Entity Too Large
原因 :上传文件超过服务器限制
解决方案:调整服务器配置或限制上传文件大小。
课后测验
测验 1:表单参数校验
问题 :如何定义一个包含校验规则的表单参数?
答案:
python
from fastapi import Form
from pydantic import Field
@app.post("/contact/")
async def contact(name: str = Form(..., min_length=3), message: str = Form(..., max_length=1000)):
return {"name": name, "message": message}
测验 2:文件上传
问题 :如何处理多个文件上传?
答案:
python
from fastapi import File, UploadFile
from typing import List
@app.post("/upload-multiple/")
async def upload_multiple_files(files: List[UploadFile] = File(...)):
return {"filenames": [file.filename for file in files]}
错误代码应急手册
错误代码 | 典型触发场景 | 解决方案 |
---|---|---|
422 | 类型转换失败/校验不通过 | 检查参数定义的校验规则 |
413 | 上传文件超过服务器限制 | 调整服务器配置或限制上传文件大小 |
500 | 未捕获的文件处理异常 | 添加 try/except 包裹敏感操作 |
400 | 自定义校验规则触发拒绝 | 检查验证器的逻辑条件 |
常见问题解答
Q:如何处理大文件上传?
A:使用 StreamingResponse
优化性能:
python
from fastapi.responses import StreamingResponse
@app.post("/upload-stream/")
async def upload_stream_file(file: UploadFile = File(...)):
return StreamingResponse(file.file, media_type=file.content_type)
Q:如何限制上传文件的大小?
A:通过 File
的 max_size
参数设置:
python
from fastapi import File, UploadFile
@app.post("/upload-validated/")
async def upload_validated_file(file: UploadFile = File(..., max_size=1024 * 1024)):
return {"filename": file.filename}
通过本教程的详细讲解和实战项目,您已掌握 FastAPI 表单参数与文件上传的核心知识。现在可以通过以下命令测试您的学习成果:
bash
curl -F "[email protected]" http://localhost:8000/upload/
余下文章内容请点击跳转至 个人博客页面 或者 扫码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
,阅读完整的文章:FastAPI 表单参数与文件上传完全指南:从基础到高级实战 🚀 | cmdragon's Blog
往期文章归档:
- FastAPI 请求体参数与 Pydantic 模型完全指南:从基础到嵌套模型实战 🚀 | cmdragon's Blog
- FastAPI 查询参数完全指南:从基础到高级用法 🚀 | cmdragon's Blog
- FastAPI 路径参数完全指南:从基础到高级校验实战 🚀 | cmdragon's Blog
- FastAPI路由专家课:微服务架构下的路由艺术与工程实践 🌐 | cmdragon's Blog
- FastAPI路由与请求处理进阶指南:解锁企业级API开发黑科技 🔥 | cmdragon's Blog
- FastAPI路由与请求处理全解:手把手打造用户管理系统 🔌 | cmdragon's Blog
- FastAPI极速入门:15分钟搭建你的首个智能API(附自动文档生成)🚀 | cmdragon's Blog
- HTTP协议与RESTful API实战手册(终章):构建企业级API的九大秘籍 🔐 | cmdragon's Blog
- HTTP协议与RESTful API实战手册(二):用披萨店故事说透API设计奥秘 🍕 | cmdragon's Blog
- 从零构建你的第一个RESTful API:HTTP协议与API设计超图解指南 🌐 | cmdragon's Blog
- Python异步编程进阶指南:破解高并发系统的七重封印 | cmdragon's Blog
- Python异步编程终极指南:用协程与事件循环重构你的高并发系统 | cmdragon's Blog
- Python类型提示完全指南:用类型安全重构你的代码,提升10倍开发效率 | cmdragon's Blog
- 三大平台云数据库生态服务对决 | cmdragon's Blog
- 分布式数据库解析 | cmdragon's Blog
- 深入解析NoSQL数据库:从文档存储到图数据库的全场景实践 | cmdragon's Blog
- 数据库审计与智能监控:从日志分析到异常检测 | cmdragon's Blog
- 数据库加密全解析:从传输到存储的安全实践 | cmdragon's Blog
- 数据库安全实战:访问控制与行级权限管理 | cmdragon's Blog
- 数据库扩展之道:分区、分片与大表优化实战 | cmdragon's Blog
- 查询优化:提升数据库性能的实用技巧 | cmdragon's Blog
- 性能优化与调优:全面解析数据库索引 | cmdragon's Blog
- 存储过程与触发器:提高数据库性能与安全性的利器 | cmdragon's Blog
- 数据操作与事务:确保数据一致性的关键 | cmdragon's Blog
- 深入掌握 SQL 深度应用:复杂查询的艺术与技巧 | cmdragon's Blog
- 彻底理解数据库设计原则:生命周期、约束与反范式的应用 | cmdragon's Blog
- 深入剖析实体-关系模型(ER 图):理论与实践全解析 | cmdragon's Blog
- 数据库范式详解:从第一范式到第五范式 | cmdragon's Blog
- PostgreSQL:数据库迁移与版本控制 | cmdragon's Blog
- Node.js 与 PostgreSQL 集成:深入 pg 模块的应用与实践 | cmdragon's Blog
- Python 与 PostgreSQL 集成:深入 psycopg2 的应用与实践 | cmdragon's Blog
- 应用中的 PostgreSQL项目案例 | cmdragon's Blog
- 数据库安全管理中的权限控制:保护数据资产的关键措施 | cmdragon's Blog
- 数据库安全管理中的用户和角色管理:打造安全高效的数据环境 | cmdragon's Blog
- 数据库查询优化:提升性能的关键实践 | cmdragon's Blog