title: FastAPI 自定义参数验证器完全指南:从基础到高级实战
date: 2025/3/11
updated: 2025/3/11
author: cmdragon
excerpt:
本教程深入探讨 FastAPI 中自定义参数验证器的使用,特别是通过 Field 函数进行数据校验。从基础概念到高级用法,通过详细的代码示例、课后测验和常见错误解决方案,帮助初学者快速掌握 FastAPI 中自定义参数验证器的核心知识。您将学习到如何通过自定义验证器优化 API 接口的数据校验、提升代码的可维护性,从而构建高效、安全的 Web 应用。
categories:
- 后端开发
- FastAPI
tags:
- FastAPI
- 参数验证
- Field函数
- API设计
- Web开发
- 数据校验
- 安全性
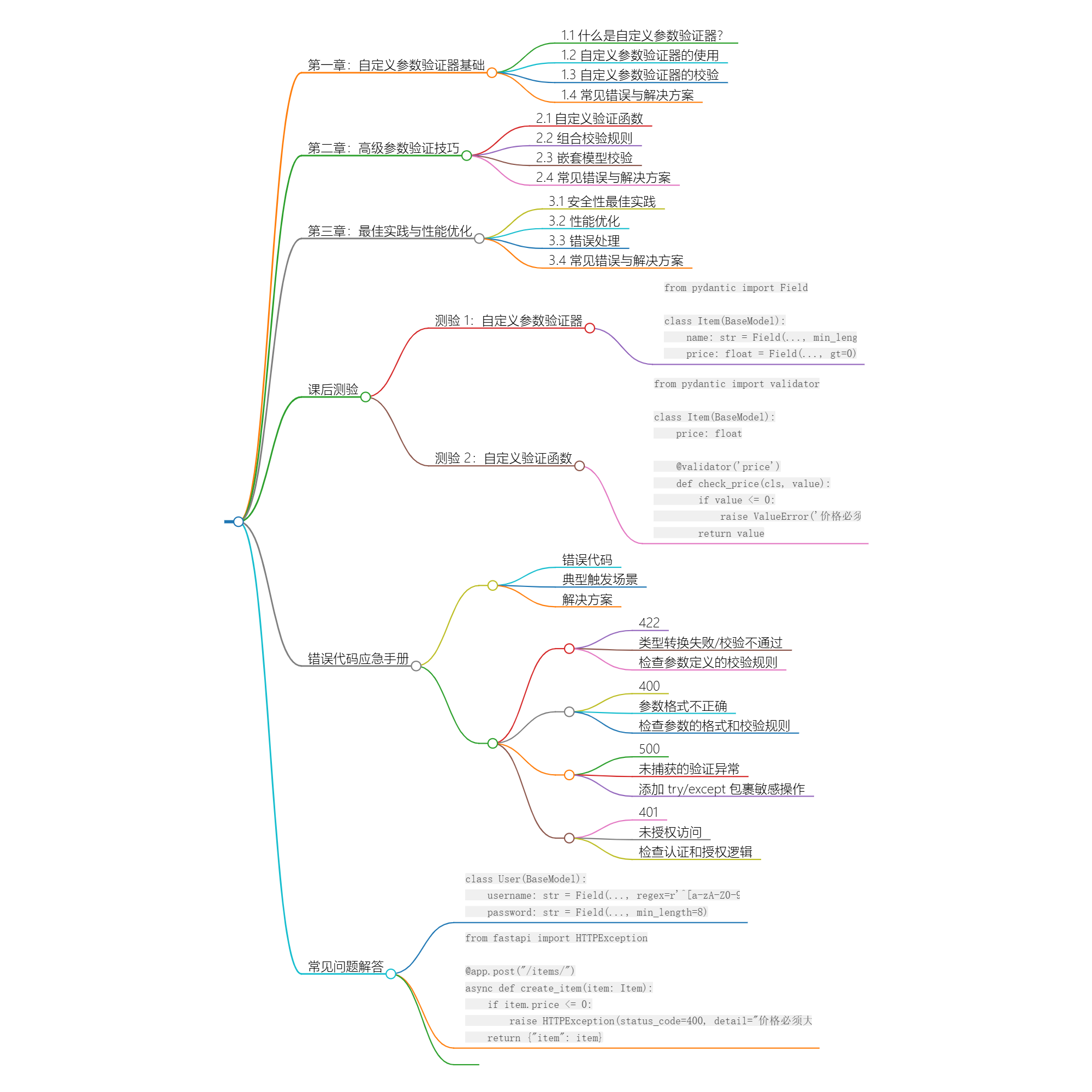
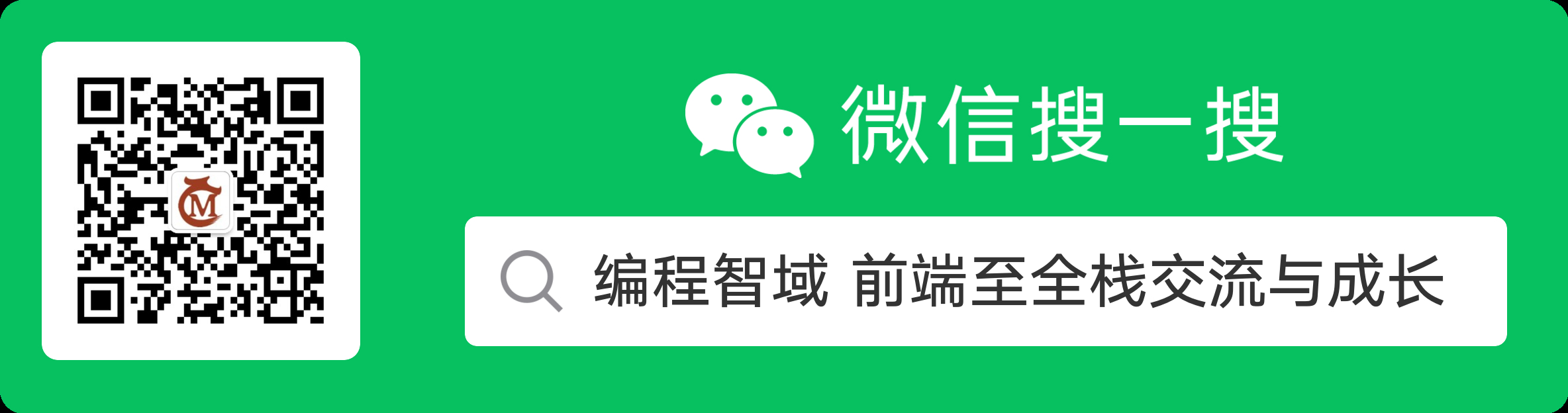
扫描二维码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
第一章:自定义参数验证器基础
1.1 什么是自定义参数验证器?
自定义参数验证器是 FastAPI 中用于对请求参数进行校验的机制,通常通过 Pydantic 的 Field
函数实现。
python
from fastapi import FastAPI, Query
from pydantic import Field
app = FastAPI()
@app.get("/items/")
async def read_items(q: str = Query(None, min_length=3)):
return {"q": q}
1.2 自定义参数验证器的使用
通过 Field
函数,可以轻松定义参数的校验规则。
python
from pydantic import BaseModel, Field
class Item(BaseModel):
name: str = Field(..., min_length=3)
price: float = Field(..., gt=0)
@app.post("/items/")
async def create_item(item: Item):
return {"item": item}
示例请求:
bash
curl -X POST -H "Content-Type: application/json" -d '{"name": "abc", "price": 10}' http://localhost:8000/items/
1.3 自定义参数验证器的校验
结合 Field
函数,可以对参数进行多种数据校验。
python
@app.get("/validate-query/")
async def validate_query(q: str = Query(..., min_length=3, max_length=10)):
return {"q": q}
示例请求:
- 合法:
curl "http://localhost:8000/validate-query/?q=abc"
→{"q": "abc"}
- 非法:
curl "http://localhost:8000/validate-query/?q=a"
→ 422 错误
1.4 常见错误与解决方案
错误 :422 Validation Error
原因 :参数类型转换失败或校验不通过
解决方案:检查参数的类型定义和校验规则。
第二章:高级参数验证技巧
2.1 自定义验证函数
通过自定义验证函数,可以实现更复杂的校验逻辑。
python
from pydantic import validator
class Item(BaseModel):
name: str
price: float
@validator('price')
def check_price(cls, value):
if value <= 0:
raise ValueError('价格必须大于0')
return value
2.2 组合校验规则
通过组合多个 Field
参数,可以实现更灵活的校验规则。
python
class Item(BaseModel):
name: str = Field(..., min_length=3, max_length=10)
price: float = Field(..., gt=0, lt=1000)
2.3 嵌套模型校验
通过嵌套模型,可以对复杂数据结构进行校验。
python
class Address(BaseModel):
city: str = Field(..., min_length=3)
zipcode: str = Field(..., regex=r'^\d{5}$')
class User(BaseModel):
name: str = Field(..., min_length=3)
address: Address
2.4 常见错误与解决方案
错误 :400 Bad Request
原因 :参数格式不正确
解决方案:检查参数的格式和校验规则。
第三章:最佳实践与性能优化
3.1 安全性最佳实践
通过 Field
的 regex
参数,可以增强参数的安全性。
python
class User(BaseModel):
username: str = Field(..., regex=r'^[a-zA-Z0-9_]+$')
password: str = Field(..., min_length=8)
3.2 性能优化
通过 Field
的 alias
参数,可以优化参数的兼容性。
python
class Item(BaseModel):
item_name: str = Field(..., alias="name")
item_price: float = Field(..., alias="price")
3.3 错误处理
通过自定义异常处理,可以优化错误提示信息。
python
from fastapi import HTTPException
@app.post("/items/")
async def create_item(item: Item):
if item.price <= 0:
raise HTTPException(status_code=400, detail="价格必须大于0")
return {"item": item}
3.4 常见错误与解决方案
错误 :500 Internal Server Error
原因 :未捕获的验证异常
解决方案:添加 try/except 包裹敏感操作。
课后测验
测验 1:自定义参数验证器
问题 :如何定义一个包含校验规则的参数?
答案:
python
from pydantic import Field
class Item(BaseModel):
name: str = Field(..., min_length=3)
price: float = Field(..., gt=0)
测验 2:自定义验证函数
问题 :如何实现自定义验证函数?
答案:
python
from pydantic import validator
class Item(BaseModel):
price: float
@validator('price')
def check_price(cls, value):
if value <= 0:
raise ValueError('价格必须大于0')
return value
错误代码应急手册
错误代码 | 典型触发场景 | 解决方案 |
---|---|---|
422 | 类型转换失败/校验不通过 | 检查参数定义的校验规则 |
400 | 参数格式不正确 | 检查参数的格式和校验规则 |
500 | 未捕获的验证异常 | 添加 try/except 包裹敏感操作 |
401 | 未授权访问 | 检查认证和授权逻辑 |
常见问题解答
Q:如何增强参数的安全性?
A:通过 Field
的 regex
参数设置:
python
class User(BaseModel):
username: str = Field(..., regex=r'^[a-zA-Z0-9_]+$')
password: str = Field(..., min_length=8)
Q:如何处理自定义错误提示?
A:通过自定义异常处理:
python
from fastapi import HTTPException
@app.post("/items/")
async def create_item(item: Item):
if item.price <= 0:
raise HTTPException(status_code=400, detail="价格必须大于0")
return {"item": item}
通过本教程的详细讲解和实战项目,您已掌握 FastAPI 中自定义参数验证器的核心知识。现在可以通过以下命令测试您的学习成果:
bash
curl -X POST -H "Content-Type: application/json" -d '{"name": "abc", "price": 10}' http://localhost:8000/items/
余下文章内容请点击跳转至 个人博客页面 或者 扫码关注或者微信搜一搜:编程智域 前端至全栈交流与成长
,阅读完整的文章:FastAPI 自定义参数验证器完全指南:从基础到高级实战 | cmdragon's Blog
往期文章归档:
- FastAPI 参数别名与自动文档生成完全指南:从基础到高级实战 🚀 | cmdragon's Blog
- FastAPI Cookie 和 Header 参数完全指南:从基础到高级实战 🚀 | cmdragon's Blog
- FastAPI 表单参数与文件上传完全指南:从基础到高级实战 🚀 | cmdragon's Blog
- FastAPI 请求体参数与 Pydantic 模型完全指南:从基础到嵌套模型实战 🚀 | cmdragon's Blog
- FastAPI 查询参数完全指南:从基础到高级用法 🚀 | cmdragon's Blog
- FastAPI 路径参数完全指南:从基础到高级校验实战 🚀 | cmdragon's Blog
- FastAPI路由专家课:微服务架构下的路由艺术与工程实践 🌐 | cmdragon's Blog
- FastAPI路由与请求处理进阶指南:解锁企业级API开发黑科技 🔥 | cmdragon's Blog
- FastAPI路由与请求处理全解:手把手打造用户管理系统 🔌 | cmdragon's Blog
- FastAPI极速入门:15分钟搭建你的首个智能API(附自动文档生成)🚀 | cmdragon's Blog
- HTTP协议与RESTful API实战手册(终章):构建企业级API的九大秘籍 🔐 | cmdragon's Blog
- HTTP协议与RESTful API实战手册(二):用披萨店故事说透API设计奥秘 🍕 | cmdragon's Blog
- 从零构建你的第一个RESTful API:HTTP协议与API设计超图解指南 🌐 | cmdragon's Blog
- Python异步编程进阶指南:破解高并发系统的七重封印 | cmdragon's Blog
- Python异步编程终极指南:用协程与事件循环重构你的高并发系统 | cmdragon's Blog
- Python类型提示完全指南:用类型安全重构你的代码,提升10倍开发效率 | cmdragon's Blog
- 三大平台云数据库生态服务对决 | cmdragon's Blog
- 分布式数据库解析 | cmdragon's Blog
- 深入解析NoSQL数据库:从文档存储到图数据库的全场景实践 | cmdragon's Blog
- 数据库审计与智能监控:从日志分析到异常检测 | cmdragon's Blog
- 数据库加密全解析:从传输到存储的安全实践 | cmdragon's Blog
- 数据库安全实战:访问控制与行级权限管理 | cmdragon's Blog
- 数据库扩展之道:分区、分片与大表优化实战 | cmdragon's Blog
- 查询优化:提升数据库性能的实用技巧 | cmdragon's Blog
- 性能优化与调优:全面解析数据库索引 | cmdragon's Blog
- 存储过程与触发器:提高数据库性能与安全性的利器 | cmdragon's Blog
- 数据操作与事务:确保数据一致性的关键 | cmdragon's Blog
- 深入掌握 SQL 深度应用:复杂查询的艺术与技巧 | cmdragon's Blog
- 彻底理解数据库设计原则:生命周期、约束与反范式的应用 | cmdragon's Blog
- 深入剖析实体-关系模型(ER 图):理论与实践全解析 | cmdragon's Blog
- 数据库范式详解:从第一范式到第五范式 | cmdragon's Blog
- PostgreSQL:数据库迁移与版本控制 | cmdragon's Blog
- Node.js 与 PostgreSQL 集成:深入 pg 模块的应用与实践 | cmdragon's Blog
- Python 与 PostgreSQL 集成:深入 psycopg2 的应用与实践 | cmdragon's Blog
- 应用中的 PostgreSQL项目案例 | cmdragon's Blog