这里写目录标题
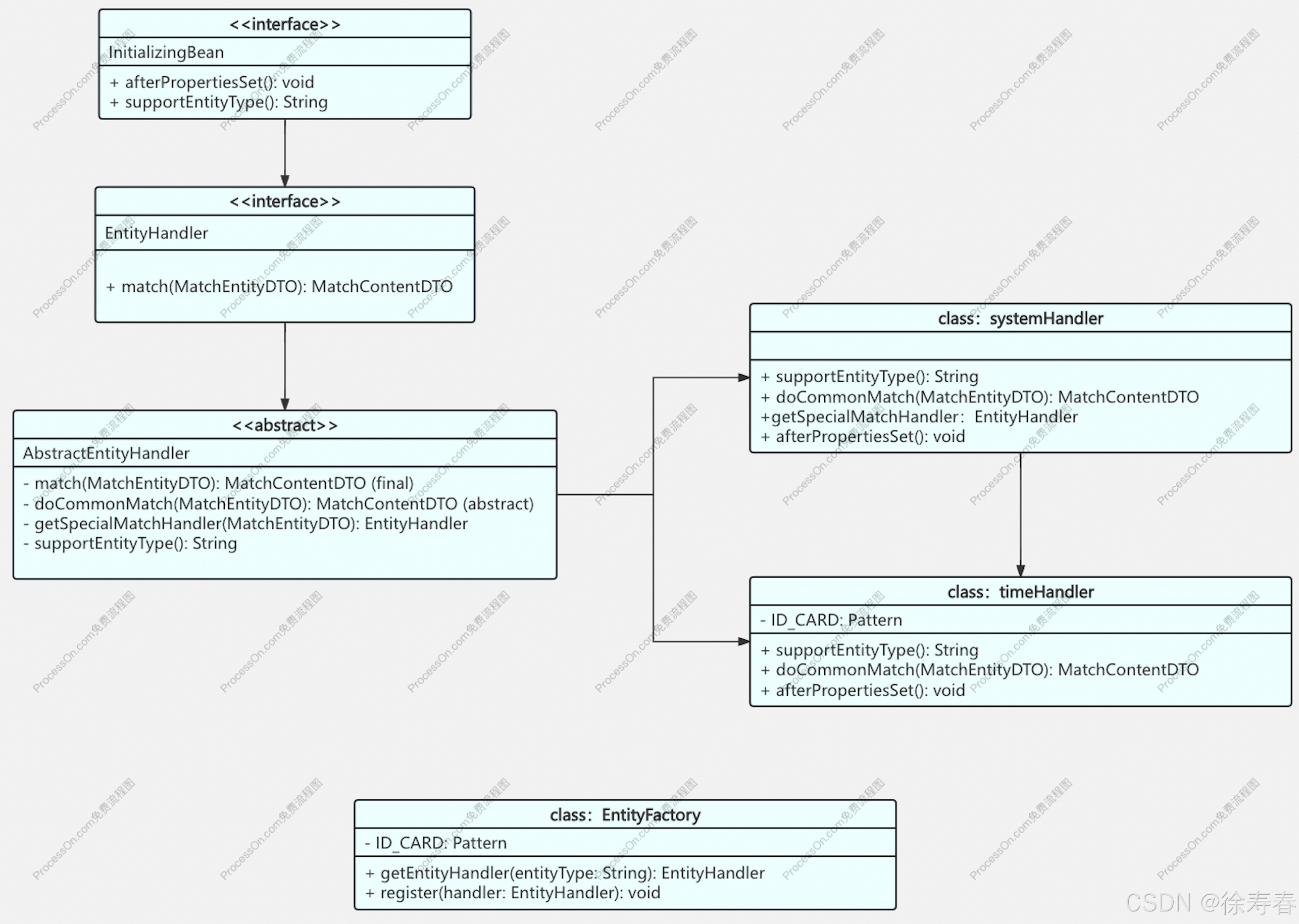
定义接口
java
public interface EntityHandler extends InitializingBean {
MatchContentDTO match(MatchEntityDTO matchEntityDTO);
String supportEntityType();
}
定义抽象类
java
public abstract class AbstractEntityHandler implements EntityHandler {
@Override
public final MatchContentDTO match(MatchEntityDTO matchEntityDTO) {
EntityHandler specialMatchHandler = getSpecialMatchHandler(matchEntityDTO);
if (specialMatchHandler != null) {
return specialMatchHandler.match(matchEntityDTO);
}
return doCommonMatch(matchEntityDTO);
}
public abstract MatchContentDTO doCommonMatch(MatchEntityDTO matchEntityDTO);
public EntityHandler getSpecialMatchHandler(MatchEntityDTO matchEntityDTO) {
return null;
}
}
定义主处理器
java
@Service
@Slf4j
public class EntitySystemHandler extends AbstractEntityHandler {
@Override
public MatchContentDTO doCommonMatch(MatchEntityDTO matchEntityDTO) {
log.info("EntitySystemHandler:{}", JSON.toJSONString(matchEntityDTO));
return new MatchContentDTO();
}
@Override
public String supportEntityType() {
return this.getClass().getName();
}
@Override
public void afterPropertiesSet() throws Exception {
EntityFactory.register(this);
}
@Override
public EntityHandler getSpecialMatchHandler(MatchEntityDTO matchEntityDTO) {
return super.getSpecialMatchHandler(matchEntityDTO);
}
}
分支处理器
java
@Service
@Slf4j
public class TimeEntityHandler extends AbstractEntityHandler {
@Override
public String supportEntityType() {
return this.getClass().getName();
}
@Override
public MatchContentDTO doCommonMatch(MatchEntityDTO matchEntityDTO) {
log.info("TimeEntityHandler:{}", JSON.toJSONString(matchEntityDTO));
return new MatchContentDTO();
}
@Override
public void afterPropertiesSet() throws Exception {
EntityFactory.register(this);
}
}
定义工厂
java
public class EntityFactory{
private static final Map<String, EntityHandler> ENTITY_HANDLER_MAP = new HashMap<>();
public static EntityHandler getEntityHandler(String entityType){
return ENTITY_HANDLER_MAP.get(entityType);
}
public static void register(EntityHandler handler) {
if (handler == null) {
return;
}
ENTITY_HANDLER_MAP.put(handler.supportEntityType(), handler);
}
}
demo
java
@SpringBootTest
@RunWith(SpringRunner.class)
public class EntityTest {
@Test
public void getTest(){
EntityHandler entityHandler = EntityFactory.getEntityHandler("system");
MatchEntityDTO matchEntityDTO = new MatchEntityDTO();
entityHandler.match(matchEntityDTO);
}
}
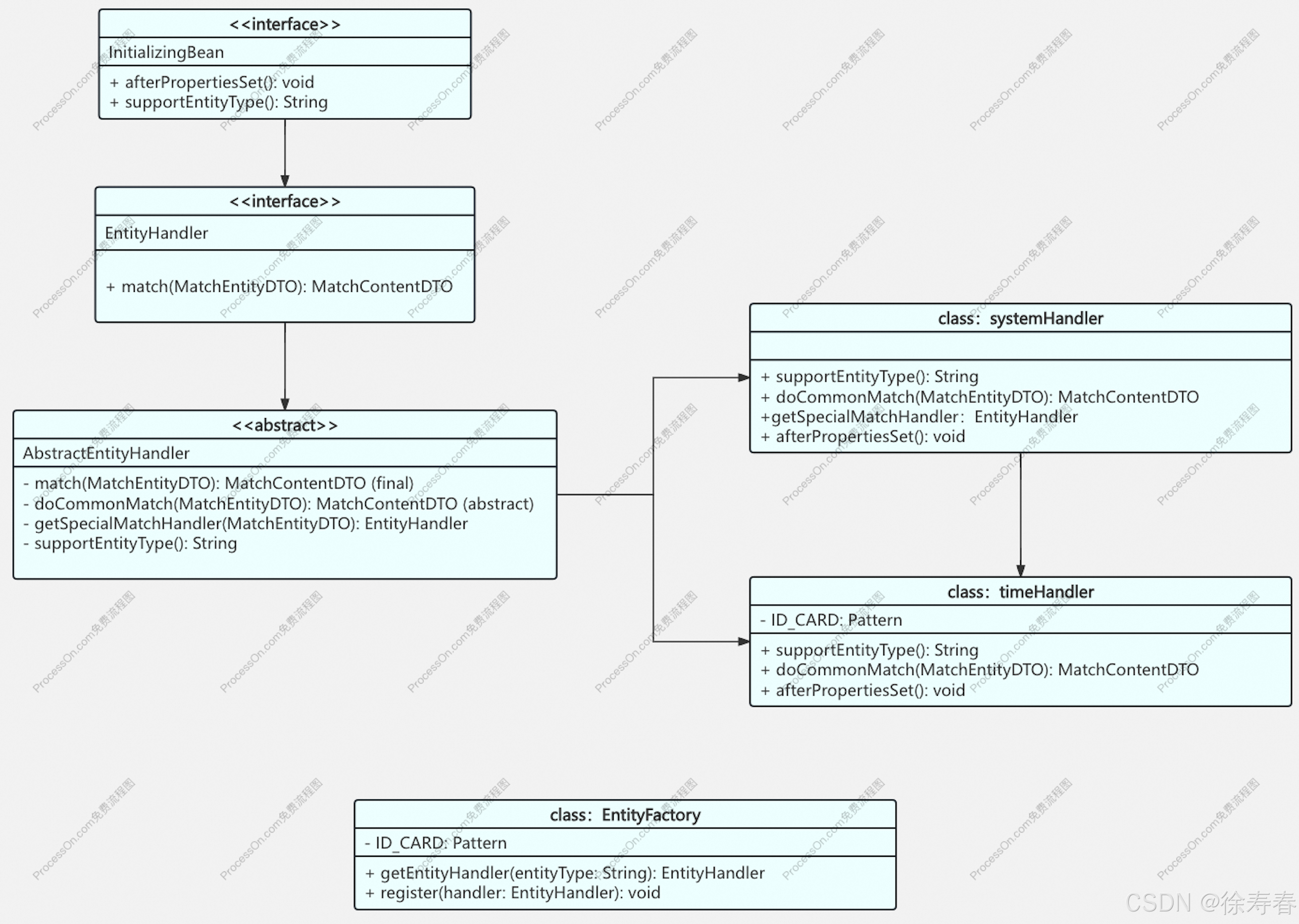