一.新增分类
对员工操作完成后,我们要进行分类操作。分类就是对菜品进行集中管理,将菜品进行分类。分类总大类分为两类,一类是菜品分类,另一类是套餐分类。对于分类,我们定义实体类Category.class。DTO类CategoryDTO.class。
Category.class
java
package com.sky.entity;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.io.Serializable;
import java.time.LocalDateTime;
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class Category implements Serializable {
private static final long serialVersionUID = 1L;
private Long id;
//类型: 1菜品分类 2套餐分类
private Integer type;
//分类名称
private String name;
//顺序
private Integer sort;
//分类状态 0标识禁用 1表示启用
private Integer status;
//创建时间
private LocalDateTime createTime;
//更新时间
private LocalDateTime updateTime;
//创建人
private Long createUser;
//修改人
private Long updateUser;
}
CategoryDTO.class
java
package com.sky.dto;
import lombok.Data;
import java.io.Serializable;
@Data
public class CategoryDTO implements Serializable {
//主键
private Long id;
//类型 1 菜品分类 2 套餐分类
private Integer type;
//分类名称
private String name;
//排序
private Integer sort;
}
二.接口文档
针对接口文档我们开发新增分类功能,使用post请求。传递过来的参数以JSON格式被封装在employeeDTO对象中。不用给前端返回数据。
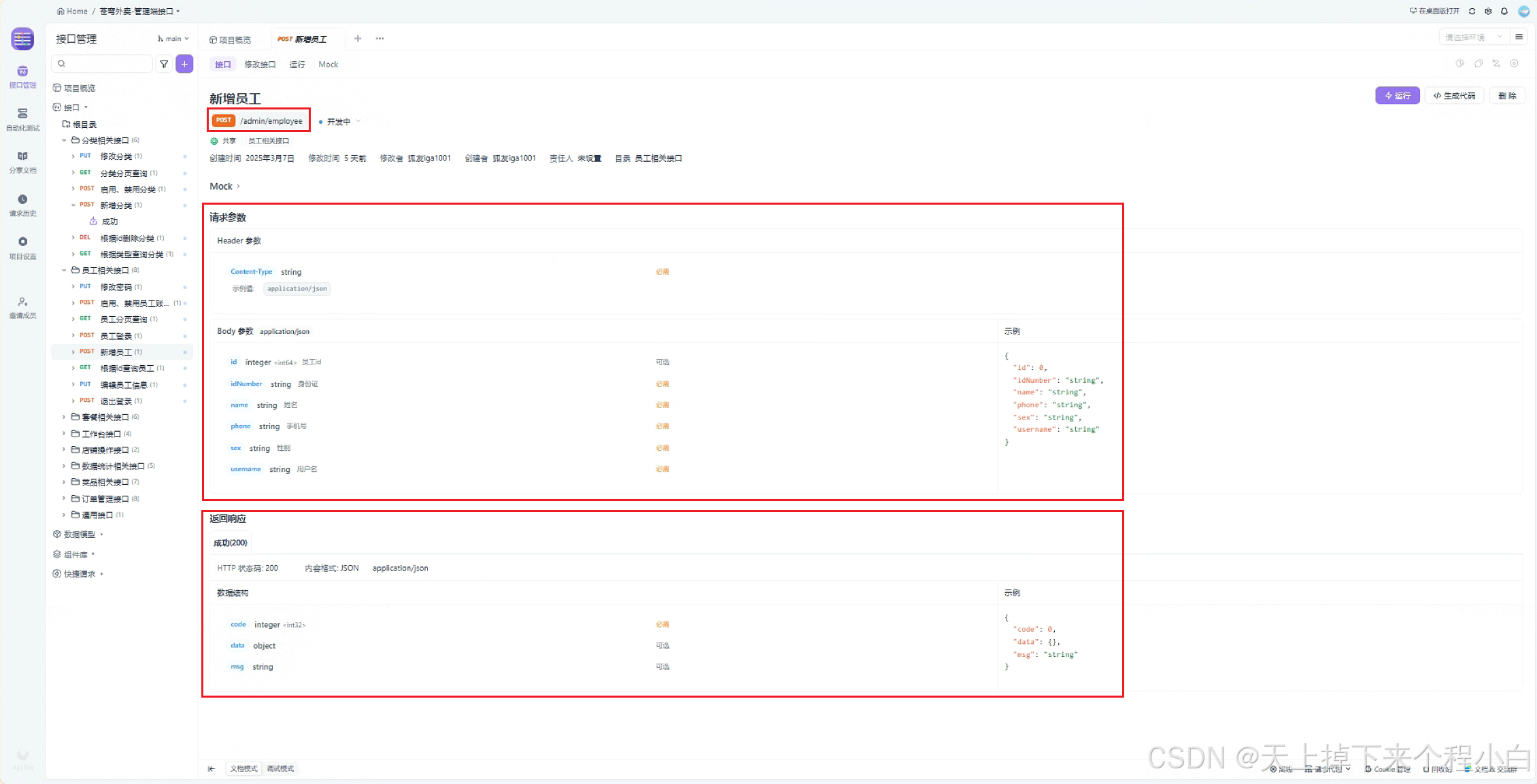
三.controller层
java
package com.sky.controller.admin;
import com.sky.dto.CategoryDTO;
import com.sky.entity.Category;
import com.sky.result.Result;
import com.sky.service.CategoryService;
import com.sky.service.impl.CategoryServiceImpl;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* 分类管理
*/
@RestController
@Api(tags = "分类相关接口")
@RequestMapping("/admin/category")
@Slf4j
public class CategoryController {
@Autowired
private CategoryService categoryService;
/**
* 新增分类
* @param categoryDTO
* @return
*/
@PostMapping
@ApiOperation("新增分类")
public Result save(@RequestBody CategoryDTO categoryDTO){
log.info("新增分类:{}",categoryDTO);
categoryService.save(categoryDTO);
return Result.success();
}
}
四.service层
接口
java
package com.sky.service;
import com.sky.dto.CategoryDTO;
public interface CategoryService {
/**
* 新增分类
* @param categoryDTO
*/
void save(CategoryDTO categoryDTO);
}
实现类
java
package com.sky.service.impl;
import com.sky.context.BaseContext;
import com.sky.dto.CategoryDTO;
import com.sky.entity.Category;
import com.sky.mapper.CategoryMapper;
import com.sky.service.CategoryService;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.time.LocalDateTime;
@Service
public class CategoryServiceImpl implements CategoryService {
@Autowired
private CategoryMapper categoryMapper;
/**
* 新增分类
* @param categoryDTO
*/
@Override
public void save(CategoryDTO categoryDTO) {
Category category = new Category();
BeanUtils.copyProperties(category,categoryDTO);
category.setStatus(StatusConstant.DISABLE);
category.setCreateTime(LocalDateTime.now());
category.setUpdateTime(LocalDateTime.now());
category.setCreateUser(BaseContext.getCurrentId());
category.setUpdateUser(BaseContext.getCurrentId());
categoryMapper.insert(category);
}
}
因为我们在mapper接口层和数据库进行连接时要新增一个category类的对象,因此我们在service层要创建一个category的实现类对象,然后将categoryDTO对象中的属性赋值给他,然后设置category中独有的属性。这其中status表示启用或者禁用该分类,我们要注意这里我们默认将其设置为0,这是因为我们当前的分类中还没有添加菜品,因此先不展示给用户。这里的setUpdateUser仍然使用ThreadLocal来获取。
五.mapper层
java
package com.sky.mapper;
import com.sky.entity.Category;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface CategoryMapper {
/**
* 新增分类接口
* @param category
*/
@Insert("insert into category(type, name, sort, status, create_time, update_time, create_user, update_user) " +
"values " +
"(#{type},#{name},#{sort},#{status},#{createTime},#{updateTime},#{createUser},#{updateUser})")
void insert(Category category);
}