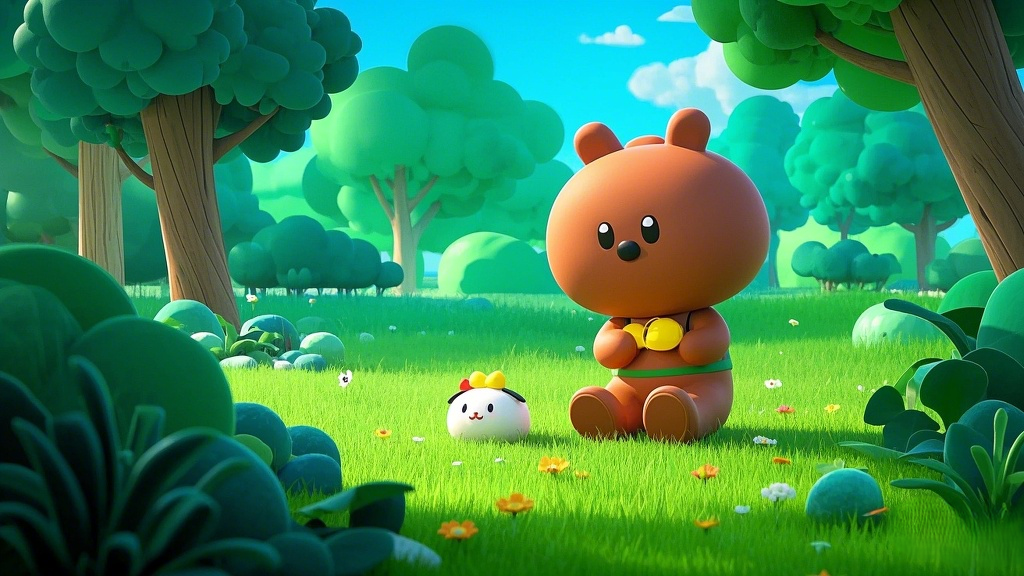
文章目录
在现代编程中,字符串操作是程序开发中不可或缺的一部分。C++20 标准的引入为字符串处理带来了诸多便捷功能,其中 std::string
和 std::string_view
的 starts_with
和 ends_with
方法尤为引人注目。这些方法不仅简化了代码,还提高了可读性和效率。本文将通过实际示例,深入探讨这些方法的使用场景和优势。
一、背景与动机
在 C++20 之前,判断一个字符串是否以某个特定的子串开头或结尾通常需要编写复杂的逻辑代码。例如,我们可能需要使用 std::string::substr
或 std::string::find
方法来实现类似功能,但这些方法不仅代码冗长,而且容易出错。C++20 的 starts_with
和 ends_with
方法正是为了解决这些问题而设计的。
二、string::starts_with 和 string::ends_with
(一)语法与功能
std::string::starts_with
和 std::string::ends_with
是 C++20 新增的成员函数,它们分别用于判断字符串是否以指定的字符或子串开头或结尾。其语法如下:
cpp
bool starts_with(char c) const noexcept;
bool starts_with(const char* s) const noexcept;
bool starts_with(const std::string& str) const noexcept;
bool ends_with(char c) const noexcept;
bool ends_with(const char* s) const noexcept;
bool ends_with(const std::string& str) const noexcept;
这些方法支持多种参数类型,包括单个字符、C 风格字符串和 std::string
对象,为开发者提供了极大的灵活性。
(二)使用示例
1. 判断字符串开头
cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
if (str.starts_with("Hello")) {
std::cout << "The string starts with 'Hello'" << std::endl;
}
if (str.starts_with('H')) {
std::cout << "The string starts with 'H'" << std::endl;
}
return 0;
}
输出结果为:
The string starts with 'Hello'
The string starts with 'H'
2. 判断字符串结尾
cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
if (str.ends_with("!")) {
std::cout << "The string ends with '!'" << std::endl;
}
if (str.ends_with("World!")) {
std::cout << "The string ends with 'World!'" << std::endl;
}
return 0;
}
输出结果为:
The string ends with '!'
The string ends with 'World!'
(三)优势
- 简洁性 :相比传统的字符串操作方法,
starts_with
和ends_with
提供了更简洁的语法,减少了代码量。 - 可读性:方法名称直观地表达了其功能,使得代码更易于理解和维护。
- 效率:这些方法经过优化,能够高效地处理字符串匹配操作。
三、string_view::starts_with 和 string_view::ends_with
(一)语法与功能
std::string_view
是 C++17 引入的轻量级字符串类,它提供了一种高效的方式处理字符串数据。C++20 为 std::string_view
添加了与 std::string
相同的 starts_with
和 ends_with
方法,其语法如下:
cpp
bool starts_with(char c) const noexcept;
bool starts_with(const char* s) const noexcept;
bool starts_with(const std::string_view& sv) const noexcept;
bool ends_with(char c) const noexcept;
bool ends_with(const char* s) const noexcept;
bool ends_with(const std::string_view& sv) const noexcept;
这些方法与 std::string
的版本类似,但它们专门针对 std::string_view
设计,进一步提升了性能。
(二)使用示例
1. 判断字符串开头
cpp
#include <iostream>
#include <string_view>
int main() {
std::string_view sv = "Hello, World!";
if (sv.starts_with("Hello")) {
std::cout << "The string view starts with 'Hello'" << std::endl;
}
if (sv.starts_with('H')) {
std::cout << "The string view starts with 'H'" << std::endl;
}
return 0;
}
输出结果为:
The string view starts with 'Hello'
The string view starts with 'H'
2. 判断字符串结尾
cpp
#include <iostream>
#include <string_view>
int main() {
std::string_view sv = "Hello, World!";
if (sv.ends_with("!")) {
std::cout << "The string view ends with '!'" << std::endl;
}
if (sv.ends_with("World!")) {
std::cout << "The string view ends with 'World!'" << std::endl;
}
return 0;
}
输出结果为:
The string view ends with '!'
The string view ends with 'World!'
(三)优势
- 性能优势 :
std::string_view
不会复制字符串数据,而是直接引用原始字符串。因此,在使用starts_with
和ends_with
方法时,不会产生额外的内存分配和复制开销。 - 灵活性 :
std::string_view
可以与std::string
无缝配合,使得代码更加灵活。
四、实践案例
(一)文件名处理
假设我们正在开发一个文件处理程序,需要判断文件名是否以特定的扩展名结尾。使用 ends_with
方法可以轻松实现这一功能:
cpp
#include <iostream>
#include <string>
bool isImageFile(const std::string& filename) {
return filename.ends_with(".jpg") || filename.ends_with(".png") || filename.ends_with(".gif");
}
int main() {
std::string filename = "example.jpg";
if (isImageFile(filename)) {
std::cout << filename << " is an image file." << std::endl;
} else {
std::cout << filename << " is not an image file." << std::endl;
}
return 0;
}
输出结果为:
example.jpg is an image file.
(二)HTTP 请求解析
在解析 HTTP 请求时,我们可能需要判断请求路径是否以特定的前缀开头。使用 starts_with
方法可以方便地实现这一功能:
cpp
#include <iostream>
#include <string>
bool isApiRequest(const std::string& path) {
return path.starts_with("/api/");
}
int main() {
std::string path = "/api/users";
if (isApiRequest(path)) {
std::cout << path << " is an API request." << std::endl;
} else {
std::cout << path << " is not an API request." << std::endl;
}
return 0;
}
输出结果为:
/api/users is an API request.
五、注意事项
- 空字符串处理 :如果目标字符串为空,
starts_with
和ends_with
方法会返回false
。 - 参数类型匹配 :在使用
starts_with
和ends_with
方法时,需要注意参数类型与目标字符串类型的一致性。例如,不要将std::string
对象传递给std::string_view
的方法。 - 性能优化 :虽然
std::string_view
提供了更高的性能,但在某些场景下,可能需要将std::string_view
转换为std::string
。此时需要注意内存分配和复制的开销。
六、总结
C++20 的 std::string::starts_with
、std::string::ends_with
、std::string_view::starts_with
和 std::string_view::ends_with
方法为字符串处理带来了极大的便利。它们不仅简化了代码,提高了可读性,还提升了性能。在实际开发中,合理使用这些方法可以显著提高开发效率和代码质量。希望本文的介绍和实践案例能够帮助你更好地理解和应用这些功能。