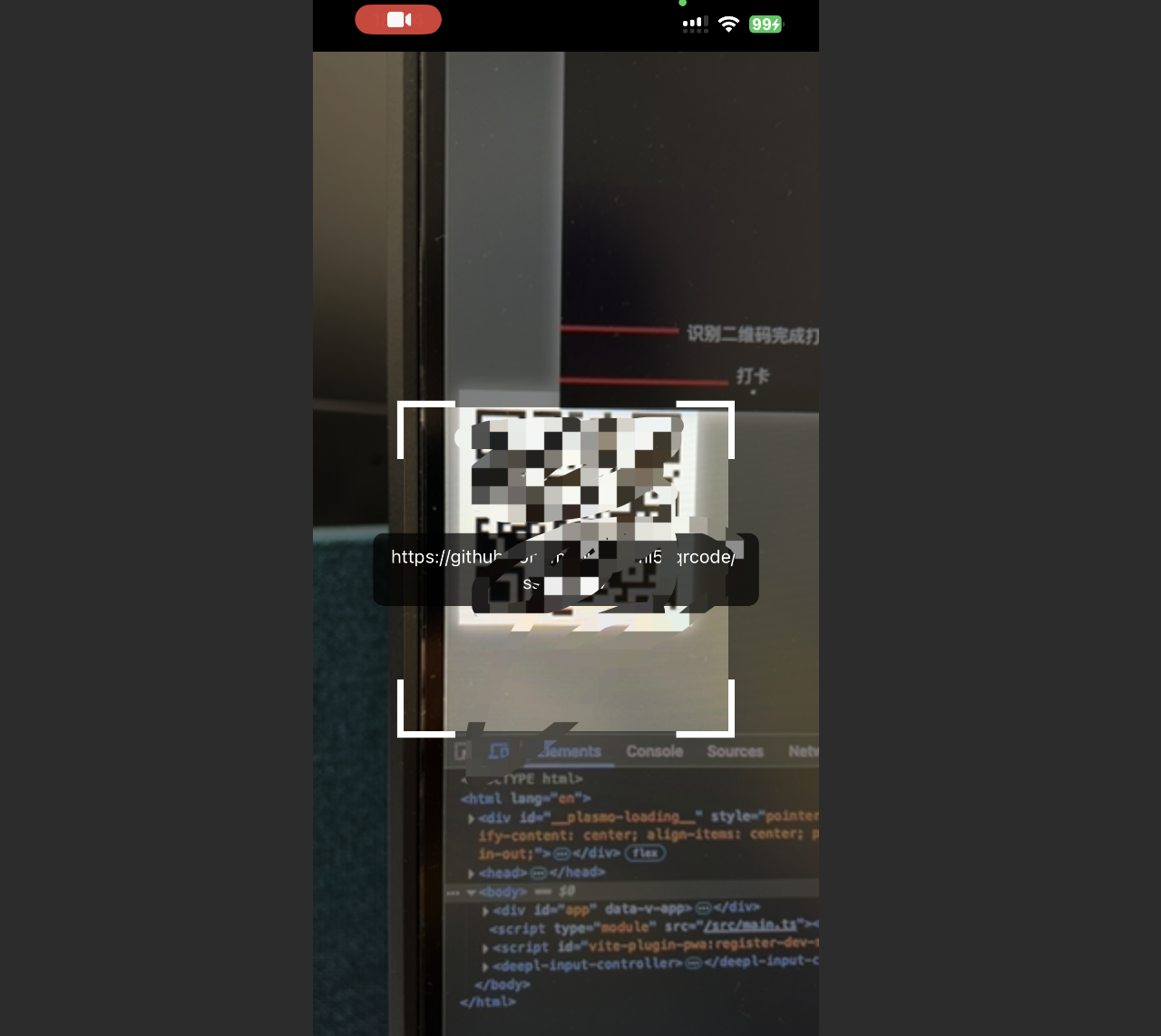
实现的效果如图所示,全屏打开并且扫描到二维码后弹窗提醒,主要就是使用html5-qrcode这个依赖库,html5-qrcode开源地址:GitHub - mebjas/html5-qrcode: A cross platform HTML5 QR code reader. See end to end implementation at: https://scanapp.org
使用文档:Getting started | ScanApp
安装依赖:
pnpm install --save-dev html5-qrcode
弹窗提示我用的vant这个ui库,开源地址:GitHub - youzan/vant: A lightweight, customizable Vue UI library for mobile web apps.
然后在项目中使用,我使用的是vue:
javascript
<template>
<div class="scanCode">
<div id="reader"></div>
</div>
</template>
<script setup lang="ts">
import { ref } from 'vue'
import { showToast } from 'vant'
import { Html5Qrcode } from 'html5-qrcode'
const codeContent = ref('1024')
// This method will trigger user permissions
Html5Qrcode.getCameras()
.then((devices) => {
// console.log(devices)
const html5QrCode = new Html5Qrcode('reader')
const width = window.innerWidth
const height = window.innerHeight
const aspectRatio = width / height
const reverseAspectRatio = height / width
const mobileAspectRatio =
reverseAspectRatio > 1.5
? reverseAspectRatio + (reverseAspectRatio * 12) / 100
: reverseAspectRatio
if (devices && devices.length) {
// .. use this to start scanning.
html5QrCode
.start(
{ facingMode: { exact: 'environment' } },
{
fps: 10, // Optional, frame per seconds for qr code scanning
aspectRatio: aspectRatio + 1,
qrbox: { width: 250, height: 250 }, // Optional, if you want bounded box UI
videoConstraints: {
facingMode: 'environment',
aspectRatio:
width < 600 ? mobileAspectRatio : aspectRatio,
},
},
(decodedText, decodedResult) => {
// do something when code is read
console.log('decodedText', decodedText)
// console.log('decodedResult', decodedResult)
codeContent.value = decodedText
showToast(decodedText)
},
(errorMessage) => {
// parse error, ignore it.
// console.log('parse error, ignore it.', errorMessage)
}
)
.catch((err) => {
// Start failed, handle it.
console.log('Start failed, handle it.')
})
}
})
.catch((err) => {
// handle err
console.log(err)
})
</script>
<style lang="scss" scoped>
.scanCode {
width: 100%;
height: 100vh;
display: flex;
flex-direction: column;
background: rgba(0, 0, 0);
#reader {
top: 50%;
left: 0;
transform: translateY(-50%);
}
}
</style>