1.AvFormatContext结构体
是ffmpeg中用于处理多媒体文件的核心结构体之一,属于libavformat模块,主要负责描述一个多媒体文件或流的封装格式,用来打开,读取,写入,操作媒体文件(如.MP4 .mkv .flv等)
2.AvFormatContext常用字段
字段名 | 类型 | 说明 |
---|---|---|
url |
char[1024] |
媒体文件路径 |
nb_streams |
unsigned int |
媒体流数量(音频、视频、字幕等) |
streams |
AVStream ** |
所有媒体流的数组 |
iformat |
AVInputFormat * |
输入格式(读取文件时使用) |
oformat |
AVOutputFormat * |
输出格式(写文件时使用) |
duration |
int64_t |
媒体总时长,单位为 AV_TIME_BASE |
bit_rate |
int64_t |
平均比特率(bps) |
start_time |
int64_t |
媒体的起始时间戳 |
metadata |
AVDictionary * |
全局元数据(如标题、作者等) |
pb |
AVIOContext * |
底层的 I/O 上下文(读写数据流用) |
3.AvFormatContext的常用相关方法
函数名 | 参数列表 | 返回类型 | 功能说明 |
---|---|---|---|
avformat_alloc_context |
无 | AVFormatContext* |
分配并初始化 AVFormatContext(需手动释放) |
avformat_open_input |
AVFormatContext **ps , const char *url , AVInputFormat *fmt , AVDictionary **options |
int |
打开输入文件或流,并分配填充 AVFormatContext |
avformat_find_stream_info |
AVFormatContext *ic , AVDictionary **options |
int |
读取并分析媒体流信息(如分辨率、采样率等) |
av_read_frame |
AVFormatContext *s , AVPacket *pkt |
int |
读取一帧压缩的音频或视频数据(存入 AVPacket) |
av_seek_frame |
AVFormatContext *s , int stream_index , int64_t timestamp , int flags |
int |
按时间戳跳转媒体位置 |
avformat_close_input |
AVFormatContext **s |
void |
关闭输入文件并释放资源 |
av_dump_format |
AVFormatContext *ic , int index , const char *url , int is_output |
void |
打印文件的封装信息(流、格式、时长、码率等) |
avformat_alloc_output_context2 |
AVFormatContext **ctx , AVOutputFormat *oformat , const char *format_name , const char *filename |
int |
创建输出文件的 AVFormatContext(推流或录制时使用) |
av_interleaved_write_frame |
AVFormatContext *s , AVPacket *pkt |
int |
将数据包写入输出媒体文件(带交错处理) |
av_write_trailer |
AVFormatContext *s |
int |
写入尾部信息(完成文件封装) |
avformat_free_context |
AVFormatContext *s |
void |
释放 AVFormatContext 所占用内存 |
4.常用字段的程序示例
cpp
#ifndef LIBAVFORMAT_CASE_H
#define LIBAVFORMAT_CASE_H
#include <libavformat/avformat.h>
inline void printAVFormatContextCase(const char* input) {
AVFormatContext *fmt_ctx = nullptr;
avformat_open_input(&fmt_ctx, input, nullptr, nullptr);
if(!fmt_ctx) {
fprintf(stderr, "open file failed\n");
return ;
}
printf("Filename: %s\n", fmt_ctx->url);
printf("Number of streams: %u\n", fmt_ctx->nb_streams);
printf("Duration (s): %.2f\n", fmt_ctx->duration / (double)AV_TIME_BASE);
printf("Bitrate: %lld\n", fmt_ctx->bit_rate);
printf("Start time: %lld\n", fmt_ctx->start_time);
if (fmt_ctx->iformat)
printf("Input format: %s\n", fmt_ctx->iformat->name);
// 打印元数据
AVDictionaryEntry *tag = NULL;
while ((tag = av_dict_get(fmt_ctx->metadata, "", tag, AV_DICT_IGNORE_SUFFIX))) {
printf("Metadata: %s = %s\n", tag->key, tag->value);
}
avformat_close_input(&fmt_ctx);
}
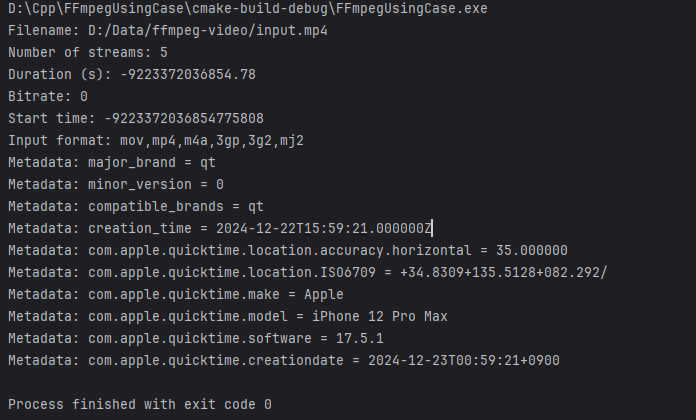