一:图元
advance函数:
在 Qt 框架里,QGraphicsItem
是用于在 QGraphicsScene
中绘制图形项的基类。advance(int phase)
是 QGraphicsItem
类的一个虚函数,其主要用途是让图形项在场景的动画或更新过程里完成特定的逻辑操作。
phase
是一个整数参数,一般有两个阶段:
phase
为 0 时:代表预更新阶段,此阶段图形项能够为即将到来的更新做准备,像计算新的位置、状态等。phase
为 1 时:代表主更新阶段,在这个阶段图形项可以依据预更新阶段的计算结果来更新自身的状态和外观。
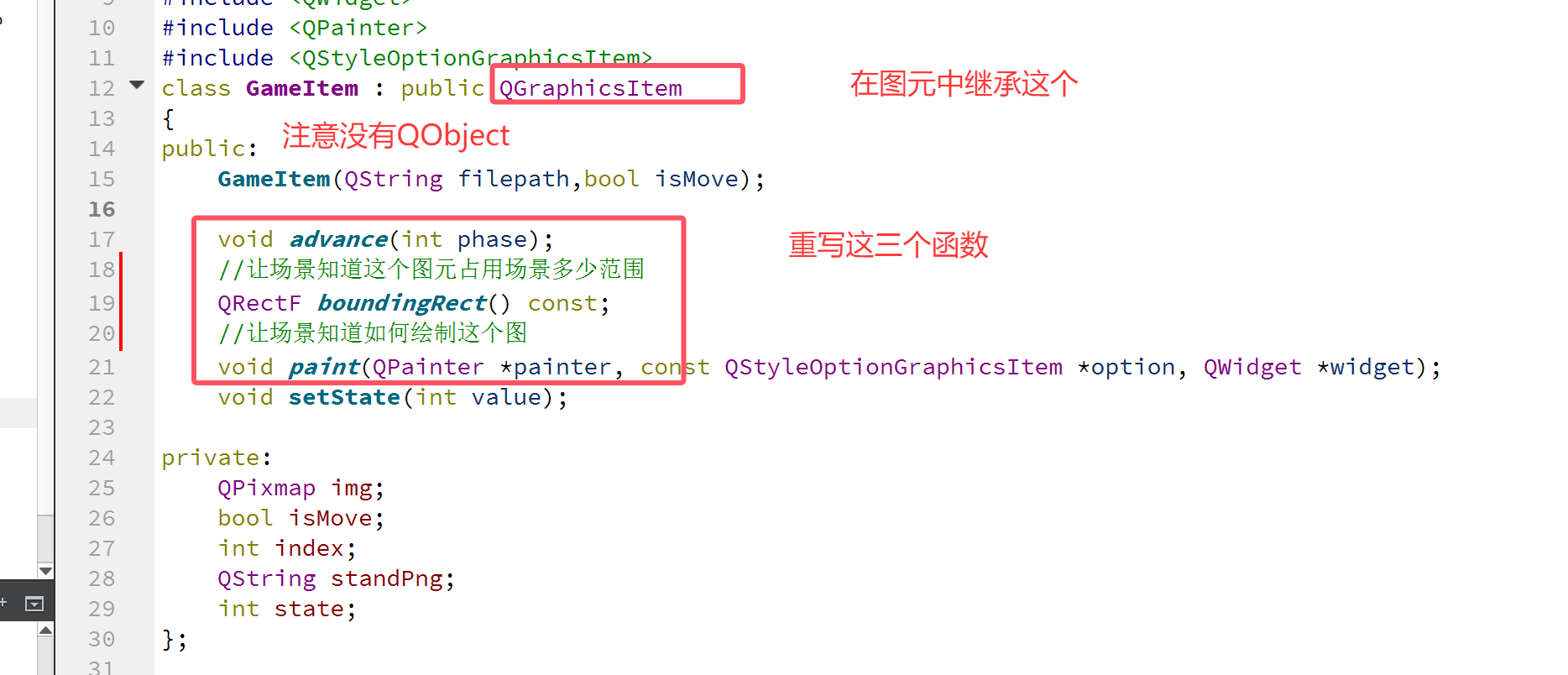
cpp
#include "gameitem.h"
GameItem::GameItem(QString filepath,bool isMove):QGraphicsItem()
{
this->state=0;
//图元读取一张图片才可以响应
this->img.load(filepath);
this->isMove=isMove;
this->index=0;
if( this->isMove==true)
{
this->standPng=filepath;
}
}
void GameItem::advance(int phase)
{
if(this->state==1)
{
this->img.load(this->standPng);
}
else {
if(this->isMove)
{
//替换
QString path=QString("img/mario/marioR/%1.png").arg(index);
this->img.load(path);
//mapToScene告诉场景要移动多少
this->setPos(mapToScene(3,0));
this->index=(this->index+1)%21;
//是否碰撞
if(collidingItems().count()>0)
{
this->img.load(this->standPng);
this->setPos(mapToScene(-3,0));
}
}
}
}
//让场景知道这个图元占用场景多少范围
QRectF GameItem::boundingRect() const
{
return QRectF(0,0,img.width(),img.height());
}
//让场景知道如何绘制这个图
void GameItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
painter->drawPixmap(0,0,img.width(),img.height(),img);
}
void GameItem::setState(int value)
{
state = value;
}
二、场景
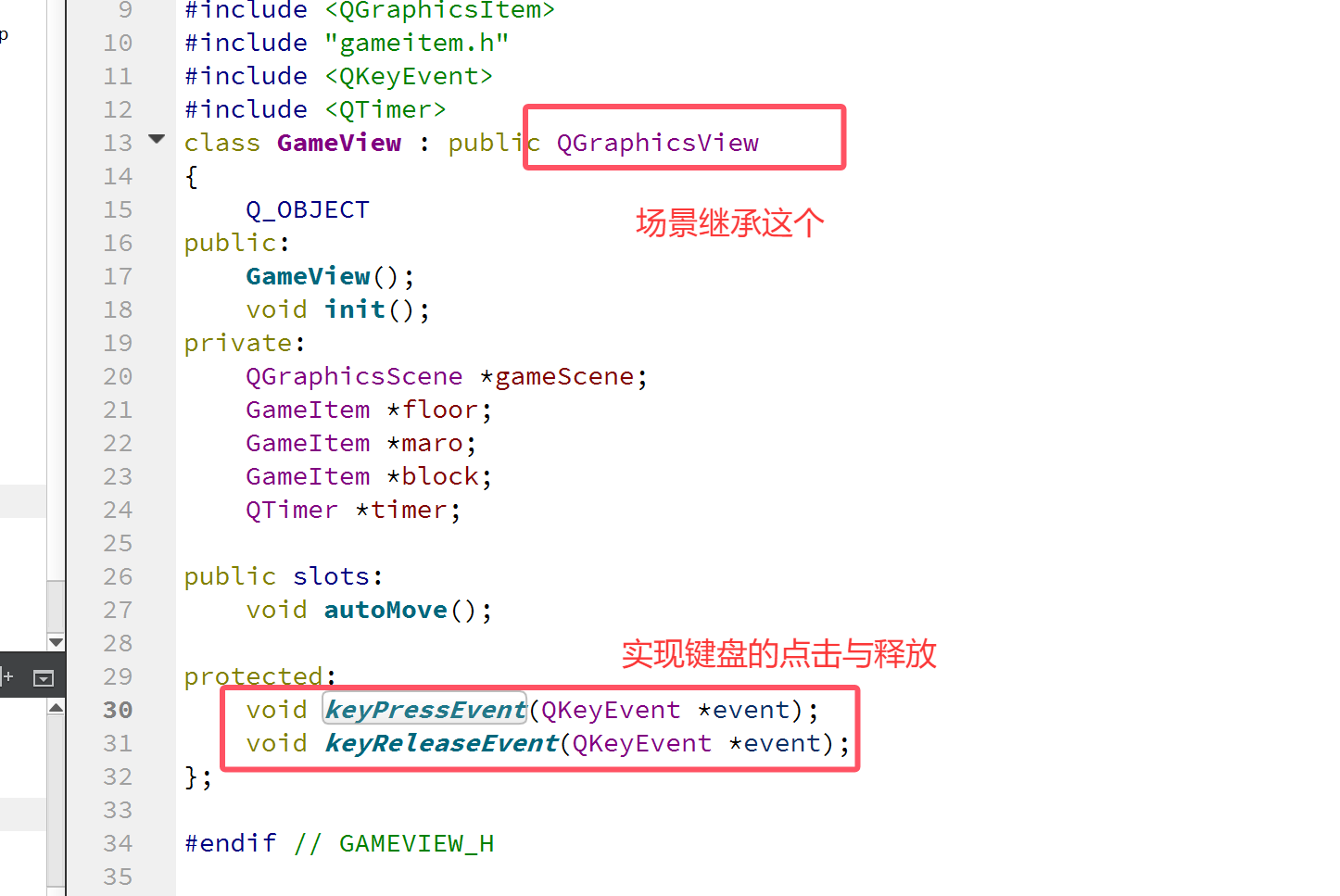
cpp
#include "gameview.h"
GameView::GameView():QGraphicsView()
{
this->setFixedSize(1333,364);
this->setWindowTitle("Super Mario");
this->setWindowIcon(QIcon("img/wm.png"));
this->init();
}
void GameView::init()
{
//背景图片
this->setBackgroundBrush(QBrush(QPixmap("img/background.png").scaled(1333,364)));
//舞台
this->gameScene=new QGraphicsScene;
this->setScene(this->gameScene);
//设置场景与视图坐标相同
this->setSceneRect(0,0,this->width(),this->height());
//隐藏滚动条
this->setHorizontalScrollBarPolicy(Qt::ScrollBarAlwaysOff);
this->setVerticalScrollBarPolicy(Qt::ScrollBarAlwaysOff);
//创建图元(演员)
this->floor=new GameItem("img/ground.png",false);
this->gameScene->addItem(this->floor);
this->floor->setPos(0,291);//位置
this->maro=new GameItem("img/mario/marioR/stand.png",true);
this->gameScene->addItem(this->maro);
this->maro->setPos(0,223);
this->block=new GameItem("img/normalwall.png",false);
this->gameScene->addItem(this->block);
this->block->setPos(500,191);
//计时器对象
this->timer=new QTimer;
this->timer->start(100);//启动计时器
//计时相应槽函数
connect(this->timer,SIGNAL(timeout()),this,SLOT(autoMove()));
}
void GameView::autoMove()
{
this->gameScene->advance();
}
//当前键盘事件
void GameView::keyPressEvent(QKeyEvent *event)
{
switch (event->key())
{
case Qt::Key_Right:
this->maro->setState(0);
this->gameScene->advance();
break;
}
}
void GameView::keyReleaseEvent(QKeyEvent *event)
{
// switch (event->key())
// {
// case Qt::Key_Right:
// this->maro->setState(1);
// this->gameScene->advance();
// break;
// }
}