后端实现步骤
- 添加Spring Boot WebSocket依赖
- 配置WebSocket端点和消息代理
- 创建控制器,使用SimpMessagingTemplate发送消息
前端实现步骤
- 安装sockjs-client和stompjs库
- 封装WebSocket连接工具类
- 在Vue组件中建立连接,订阅主题
详细实现步骤
后端(Spring Boot)实现步骤
1. 添加依赖
<!-- pom.xml -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
2. 配置WebSocket
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
// 注册WebSocket端点,前端连接此地址
registry.addEndpoint("/ws")
.setAllowedOriginPatterns("*") // 解决跨域问题
.withSockJS(); // 支持SockJS
}
@Override
public void configureMessageBroker(MessageBrokerRegistry registry) {
// 客户端订阅的主题前缀
registry.enableSimpleBroker("/topic");
}
}
3. 发送消息的Controller
@RestController
public class MessageController {
@Autowired
private SimpMessagingTemplate messagingTemplate;
// 发送消息到所有客户端
@GetMapping("/send")
public void sendToAll(String message) {
messagingTemplate.convertAndSend("/topic/messages", message);
}
}
前端(Vue)实现步骤
1. 安装依赖
npm install sockjs-client stompjs
2. 封装WebSocket工具类
// src/utils/websocket.js
import SockJS from 'sockjs-client';
import Stomp from 'stompjs';
let stompClient = null;
export function connect(url,callback) {
const socket = new SockJS(url);
stompClient = Stomp.over(socket);
stompClient.connect({}, () => {
stompClient.subscribe('/topic/messages', (message) => {
callback(message.body)
});
});
}
export function disconnect() {
if (stompClient) {
stompClient.disconnect();
}
}
3. Vue组件集成
<template>
<div>
<div>收到消息: {{ receivedMsg }}</div>
</div>
</template>
<script>
import { connect, disconnect } from '@/ws/websocket';
export default {
data() {
return {
inputMsg: '',
receivedMsg: ''
};
},
mounted() {
connect('http://localhost:8088/ws',(msg)=>{
this.receivedMsg = msg;
});
},
beforeDestroy() {
disconnect();
},
methods: {
}
};
</script>
测试
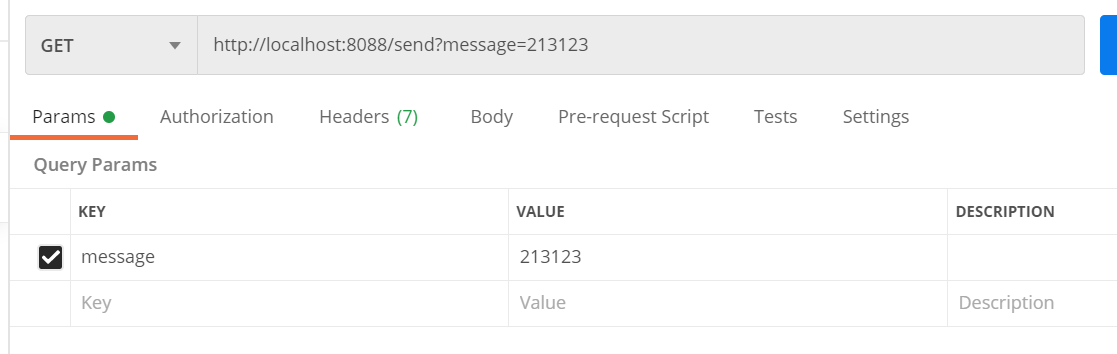
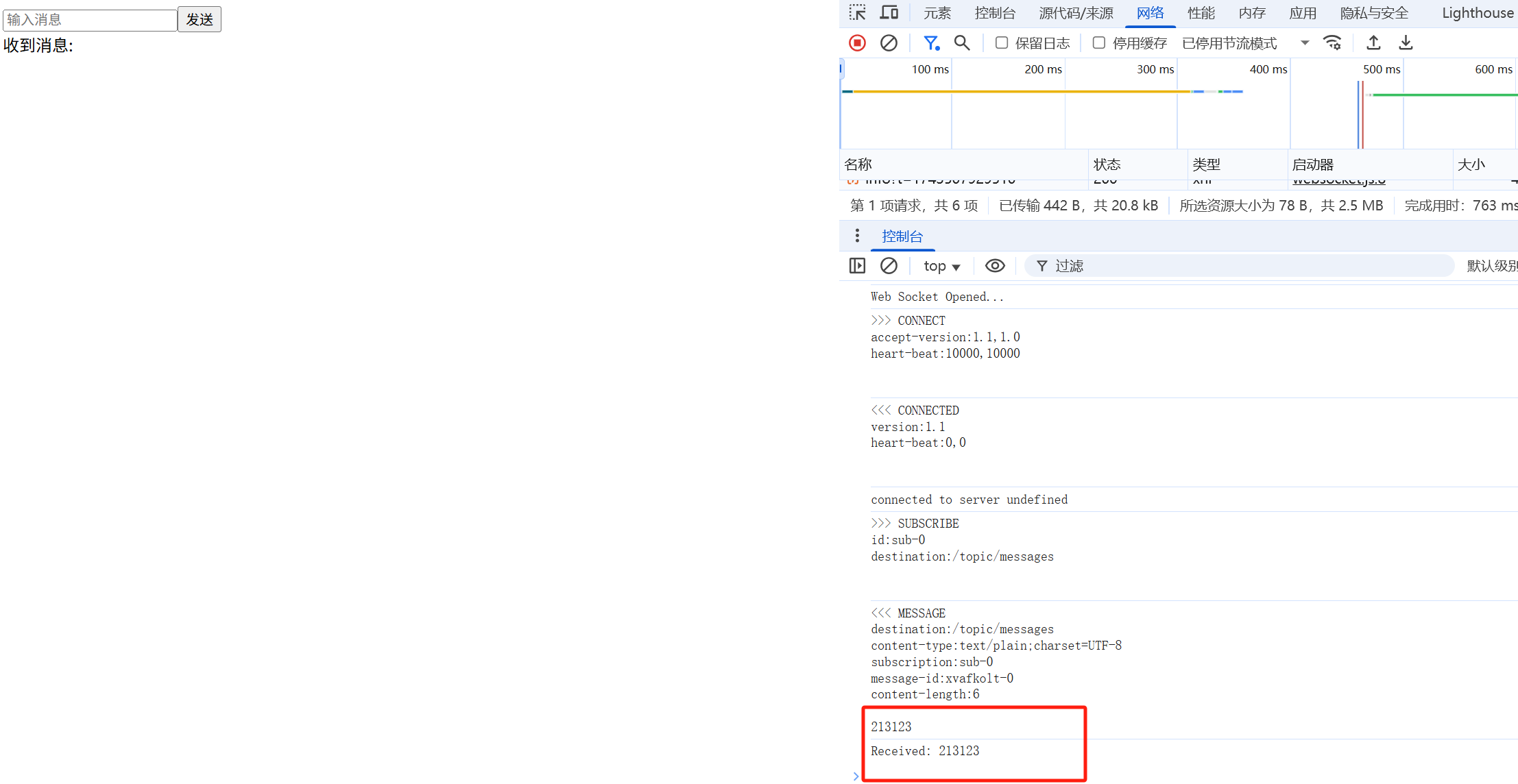
向指定客户端发送消息
后端
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
package com.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.messaging.simp.config.MessageBrokerRegistry;
import org.springframework.web.socket.config.annotation.EnableWebSocketMessageBroker;
import org.springframework.web.socket.config.annotation.StompEndpointRegistry;
import org.springframework.web.socket.config.annotation.WebSocketMessageBrokerConfigurer;
/**
* @author cyz
*/
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
// 客户端连接的端点(WebSocket URL)
registry.addEndpoint("/ws")
// 允许所有来源(根据需求调整)
.setAllowedOrigins("*")
// 支持 SockJS 降级(兼容不支持 WebSocket 的浏览器)
.withSockJS();
}
@Override
public void configureMessageBroker(MessageBrokerRegistry registry) {
// 客户端订阅的地址前缀(STOMP 主题)
registry.enableSimpleBroker("/portCheckProgress");
}
}
package com;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.messaging.simp.SimpMessagingTemplate;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
/**
* @author cyz
* @since 2025/4/1 下午5:15
*/
@RestController
public class MessageController {
@Autowired
private SimpMessagingTemplate messagingTemplate;
@GetMapping("/send-to-user")
public void sendToUser(@RequestParam String userCode, @RequestParam String message) {
String destination = "/portCheckProgress/info/"+userCode;
messagingTemplate.convertAndSend(destination, message);
}
}
package com;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
/**
* @author cyz
* @since 2025/4/1 下午5:12
*/
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}

前端
// src/utils/websocket.js
import SockJS from 'sockjs-client';
import Stomp from 'stompjs';
let stompClient = null;
export function connect(url,userCode,callback) {
const socket = new SockJS(url);
stompClient = Stomp.over(socket);
stompClient.connect({}, () => {
stompClient.subscribe('/portCheckProgress/info/'+userCode, (message) => {
callback(message.body)
});
});
}
export function disconnect() {
if (stompClient) {
stompClient.disconnect();
}
}
<template>
<div>
<div>收到消息: {{ receivedMsg }}</div>
</div>
</template>
<script>
import { connect, disconnect } from '@/ws/websocket';
export default {
data() {
return {
inputMsg: '',
receivedMsg: ''
};
},
mounted() {
var split = location.href.split("?userCode=");
var userCode = split[1]
connect('http://localhost:8088/ws',userCode,(msg)=>{
this.receivedMsg = msg;
});
},
beforeDestroy() {
disconnect();
},
methods: {
}
};
</script>
测试
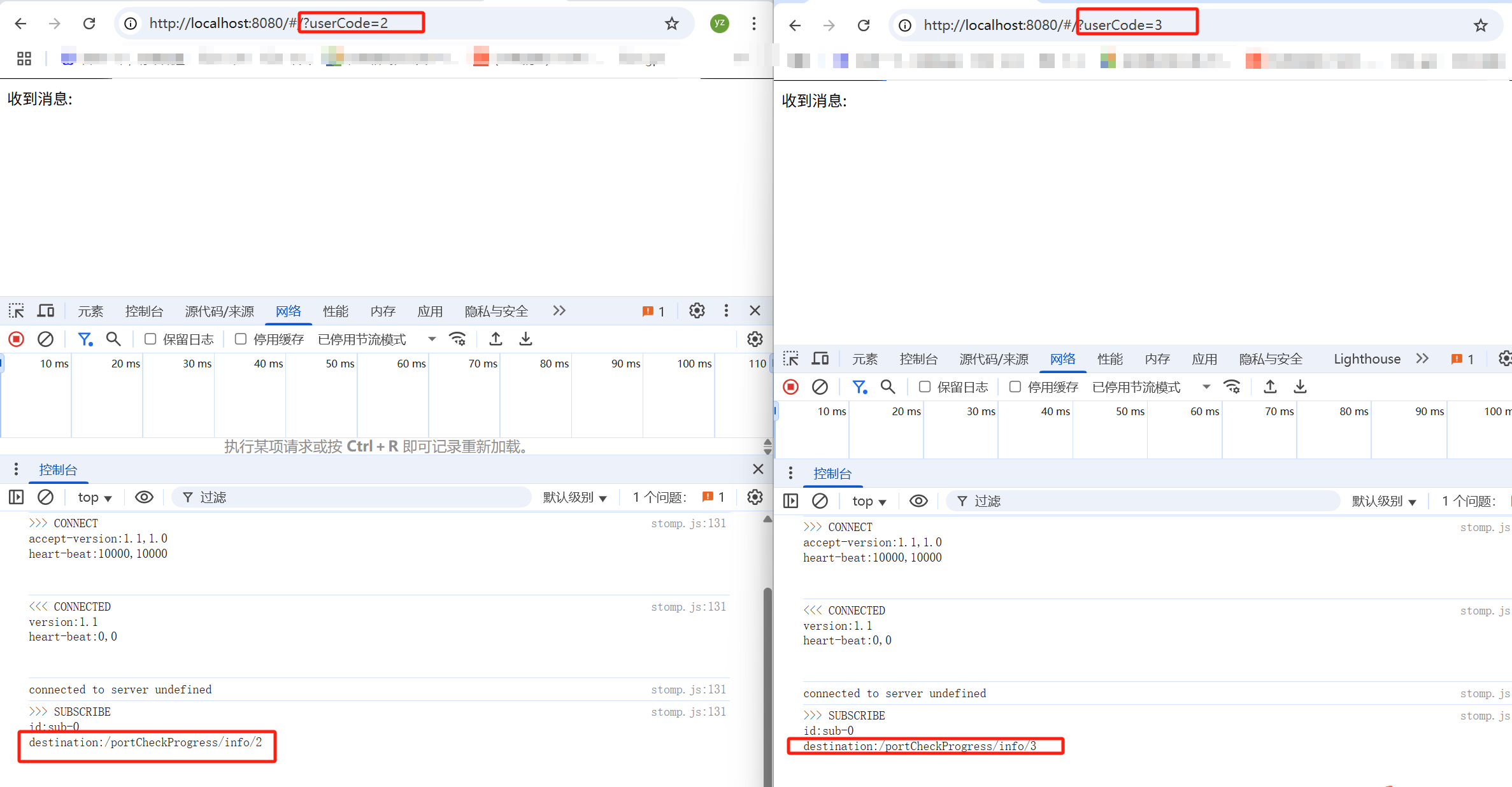
向2中发送消息
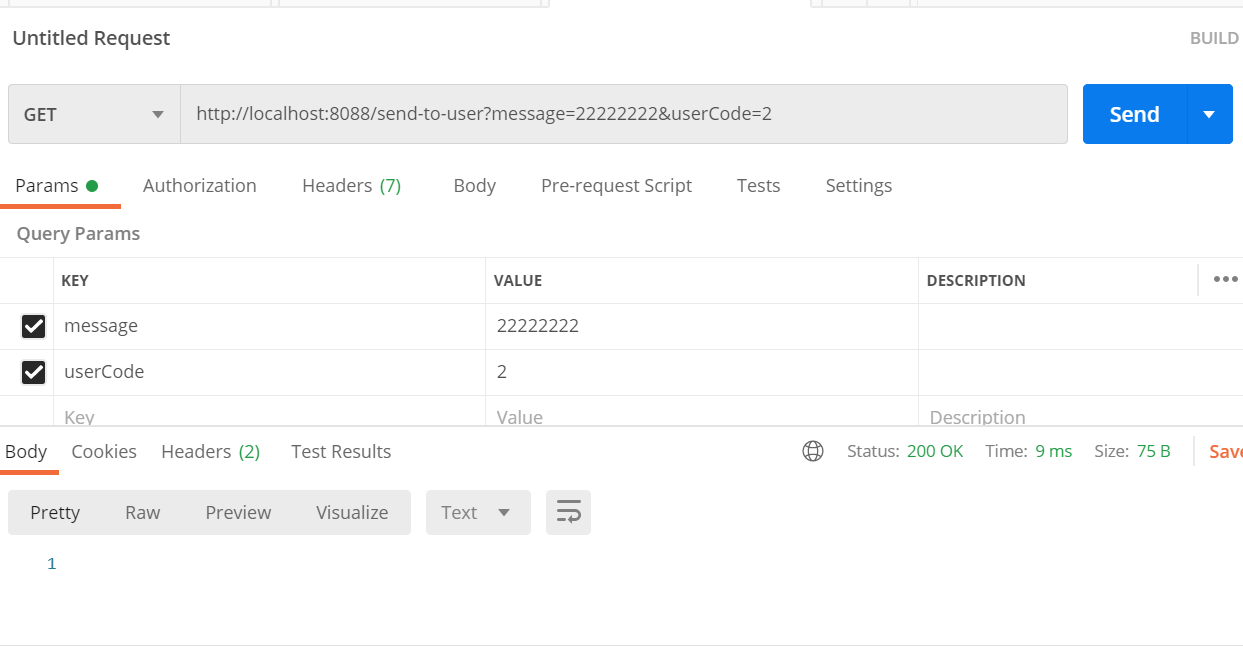
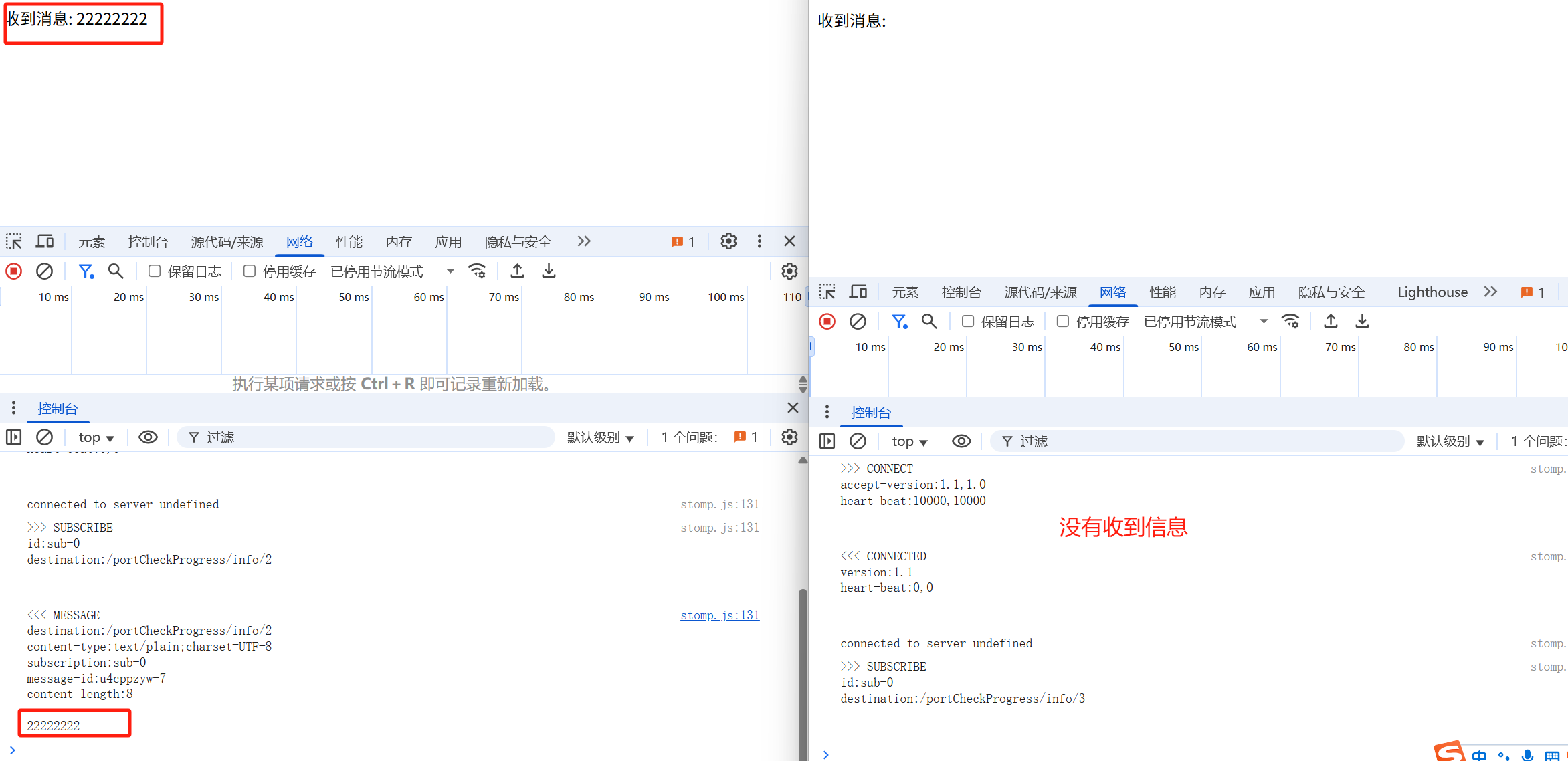
向3中发送消息