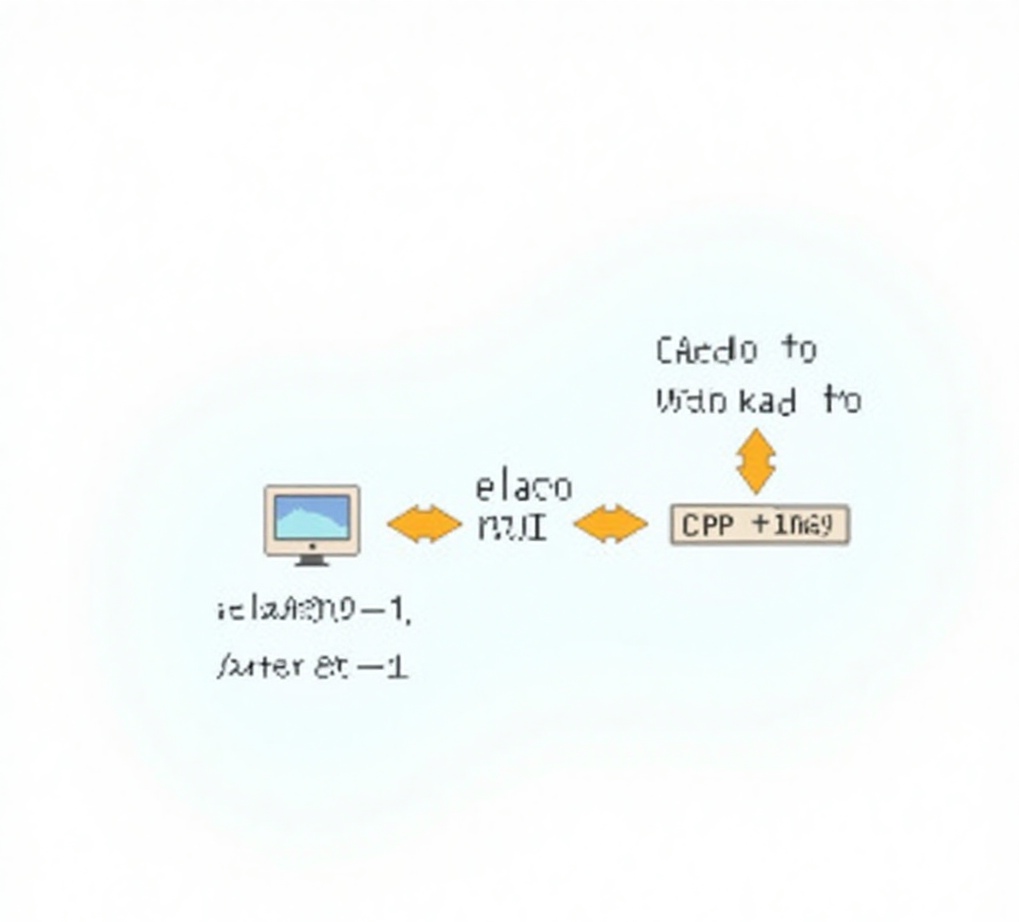
引言
在现代Web开发中,异步编程已成为提高性能的关键技术。Rust语言凭借其高性能、内存安全和强大的异步生态(如tokio
、async-std
、hyper
等),成为构建高效网络服务的理想选择。
本文将介绍如何使用 Rust + Hyper + Tokio 实现 异步HTTP请求 并 高效读取响应体(Response Body),涵盖:
- 异步HTTP请求的基本概念
- Hyper库的使用方法
- 如何异步读取HTTP响应数据
- 完整代码实现及优化建议
1. 异步HTTP请求的基本概念
1.1 同步 vs. 异步HTTP请求
- 同步请求:客户端发送请求后,必须等待服务器响应才能继续执行后续代码。
- 异步请求:客户端发送请求后,可以继续执行其他任务,待响应到达后再处理数据。
异步的优势:
✅ 更高的吞吐量:单线程可处理多个并发请求
✅ 更低的延迟:避免阻塞,提高IO密集型任务效率
✅ 适合高并发场景:如爬虫、API调用、微服务通信
1.2 Rust的异步生态
Rust的异步编程主要依赖:
Future
:表示一个尚未完成的计算async/await
:简化异步代码编写Tokio
:最流行的异步运行时(Runtime)Hyper
:高性能HTTP客户端/服务器库
2.实现异步读取HTTP响应体
1. 环境准备
在开始之前,确保你的Rust环境已经安装完成
安装完成后,创建一个新的Rust项目:
2. 添加依赖
在Cargo.toml
文件中,添加hyper
和futures
库的依赖:
toml
[dependencies]
hyper = "0.14"
futures = "0.3"
tokio = { version = "1", features = ["full"] }
这里我们还添加了tokio
,它是一个异步运行时,用于执行异步任务。
3. 编写代码
在src/main.rs
文件中,编写以下代码:
rust
use hyper::{Client, Uri};
use hyper::body;
use futures::TryFutureExt;
use tokio;
#[tokio::main]
async fn main() {
// 创建一个HTTP客户端
let client = Client::new();
// 定义目标URL
let uri: Uri = "http://www.renren.com".parse().unwrap();
// 发送GET请求
let response = client.get(uri).await.unwrap();
// 异步读取响应体
let body = body::to_bytes(response.into_body()).await.unwrap();
// 将响应体转换为字符串并打印
let html = String::from_utf8(body.to_vec()).unwrap();
println!("{}", html);
}
4. 代码解析
- 创建HTTP客户端:
Client::new()
用于创建一个HTTP客户端实例。 - 定义目标URL:我们将目标网站的URL解析为
Uri
类型。 - 发送GET请求:使用
client.get(uri)
发送GET请求,await
关键字用于等待异步操作完成。 - 异步读取响应体:
body::to_bytes(response.into_body())
将响应体转换为字节数组,await
等待操作完成。 - 转换为字符串并打印:将字节数组转换为字符串,并打印出来。
3.扩展功能
1. 添加代理服务器
在实际应用中,我们可能需要通过代理服务器发送请求。hyper
支持配置代理服务器,以下是修改后的代码:
rust
use hyper::{Client, Uri};
use hyper::body;
use hyper::client::HttpConnector;
use hyper_proxy::{Intercept, Proxy, ProxyConnector};
use hyper_proxy::ProxyConnectorBuilder;
use hyper::header::HeaderValue;
use futures::TryFutureExt;
use tokio;
#[tokio::main]
async fn main() {
// 定义代理服务器信息
let proxy_host = "www.16yun.cn";
let proxy_port = 5445; // 端口需要是整数类型
let proxy_user = "16QMSOML";
let proxy_pass = "280651";
// 构造代理认证的Basic认证头
let proxy_auth = format!("Basic {}", base64::encode(format!("{}:{}", proxy_user, proxy_pass)));
let proxy_auth_header = HeaderValue::from_str(&proxy_auth).unwrap();
// 创建代理对象
let proxy = Proxy::new(Intercept::All, format!("http://{}:{}", proxy_host, proxy_port).parse().unwrap());
// 创建支持代理的HTTP客户端
let proxy_connector = ProxyConnectorBuilder::new(HttpConnector::new(), proxy)
.set_authorization(proxy_auth_header)
.build()
.unwrap();
let client = Client::builder().build::<_, hyper::Body>(proxy_connector);
// 定义目标URL
let uri: Uri = "http://www.renren.com".parse().unwrap();
// 发送GET请求
let response = client.get(uri).await.unwrap();
// 异步读取响应体
let body = body::to_bytes(response.into_body()).await.unwrap();
// 将响应体转换为字符串并打印
let html = String::from_utf8(body.to_vec()).unwrap();
println!("{}", html);
}
2. 错误处理
在实际应用中,我们需要对可能出现的错误进行处理。以下是添加错误处理后的代码:
rust
use hyper::{Client, Uri};
use hyper::body;
use hyper::client::HttpConnector;
use hyper_proxy::{Intercept, Proxy, ProxyConnector};
use futures::TryFutureExt;
use tokio;
#[tokio::main]
async fn main() {
// 定义代理服务器
let proxy = Proxy::new(Intercept::All, "http://ip.16yun.cn:31111".parse().unwrap());
// 创建一个支持代理的HTTP客户端
let proxy_connector = ProxyConnector::from_proxy(HttpConnector::new(), proxy).unwrap();
let client = Client::builder().build::<_, hyper::Body>(proxy_connector);
// 定义目标URL
let uri: Uri = "http://www.renren.com".parse().unwrap();
// 发送GET请求并处理错误
match client.get(uri).await {
Ok(response) => {
// 异步读取响应体
match body::to_bytes(response.into_body()).await {
Ok(body) => {
// 将响应体转换为字符串并打印
match String::from_utf8(body.to_vec()) {
Ok(html) => println!("{}", html),
Err(e) => eprintln!("Failed to convert body to string: {}", e),
}
}
Err(e) => eprintln!("Failed to read response body: {}", e),
}
}
Err(e) => eprintln!("Failed to send request: {}", e),
}
}
六、总结
本文详细介绍了如何在Rust中使用hyper
和futures
库实现异步读取HTTP响应体的过程。我们从环境准备、代码编写到扩展功能,逐步展示了如何发送HTTP请求、异步处理响应,并读取响应体中的内容。通过添加代理服务器和错误处理,我们使程序更加健壮和实用。
Rust的异步编程模型不仅提供了高性能的I/O操作,还通过async/await
语法简化了异步代码的编写。hyper
和futures
库的结合使用,使得异步网络请求的处理变得简单而高效。希望本文能够帮助你更好地理解和应用Rust的异步编程技术。