目录
[1. 有效的括号](#1. 有效的括号)
[2. 最小栈](#2. 最小栈)
[3. 字符串解码](#3. 字符串解码)
[4. 每日温度](#4. 每日温度)
[5. 柱状图中最大的矩形](#5. 柱状图中最大的矩形)
[1. 数组中的第K个最大元素](#1. 数组中的第K个最大元素)
[2. 前K个高频元素](#2. 前K个高频元素)
[3. 数据流中的中位数](#3. 数据流中的中位数)
前言
一、栈:有效的括号,最小栈,字符串解码,每日温度,柱状图中最大的矩形。
二、堆:数组中的第K个最大元素,前K个高频元素,数据流中的中位数。
一、栈
1. 有效的括号
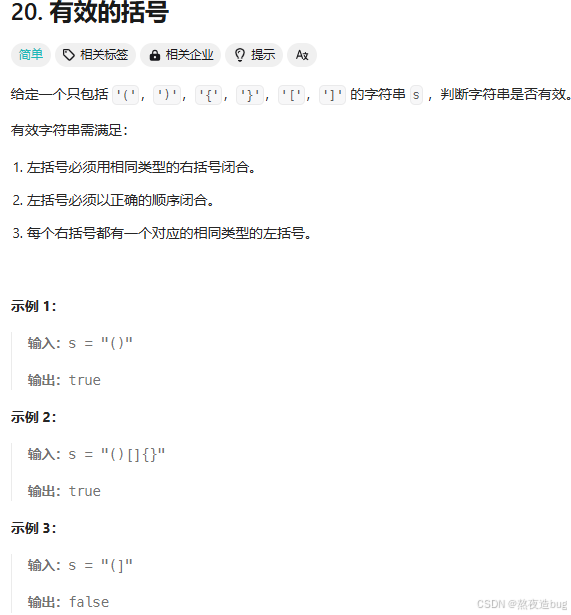
python
class Solution(object):
def isValid(self, s):
dicts = {
')':'(',
']': '[',
'}': '{'
}
stack = []
for s_ in s:
if stack and s_ in dicts:
if stack[-1] == dicts[s_]:
stack.pop()
else:
return False
else:
stack.append(s_)
return not stack
2. 最小栈
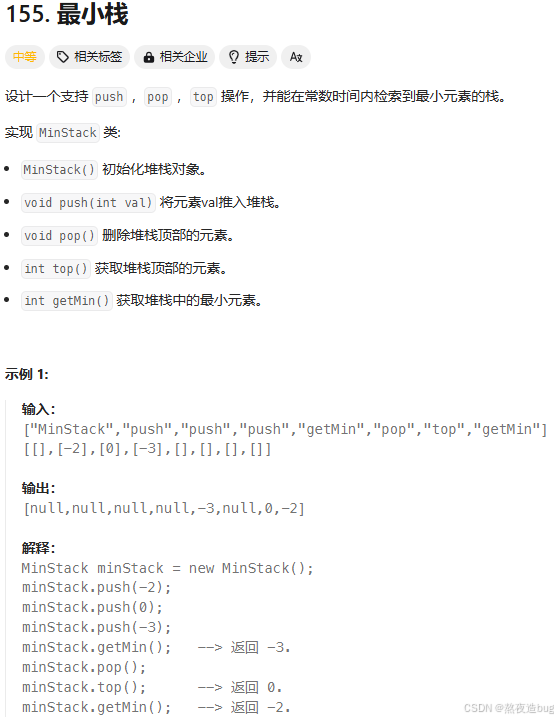
python
class MinStack(object):
def __init__(self):
self.lst = []
def push(self, val):
self.lst.append(val)
def pop(self):
self.lst.pop()
def top(self):
return self.lst[-1]
def getMin(self):
return min(self.lst)
# Your MinStack object will be instantiated and called as such:
# obj = MinStack()
# obj.push(val)
# obj.pop()
# param_3 = obj.top()
# param_4 = obj.getMin()
3. 字符串解码
原题链接:394. 字符串解码 - 力扣(LeetCode)
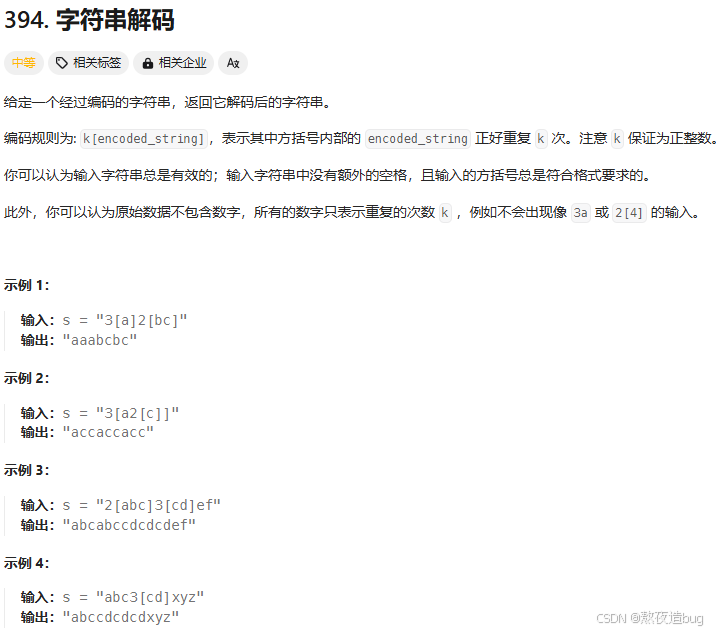
python
class Solution(object):
def decodeString(self, s):
stack = []
res, dig = '', 0
for s_ in s:
if s_.isdigit():
dig = dig*10 + int(s_) # 处理300, 21等数字
elif 'a'<=s_<='z':
res = res + s_
elif s_ == '[':
stack.append((res, dig))
res, dig = '', 0
else:
res_top, dig_top = stack.pop()
res = res_top + res * dig_top # [('', 3), ('a', 0)] --> res = 'a' --> res = ''+ 'a'*3 = 'aaa'
return res
4. 每日温度
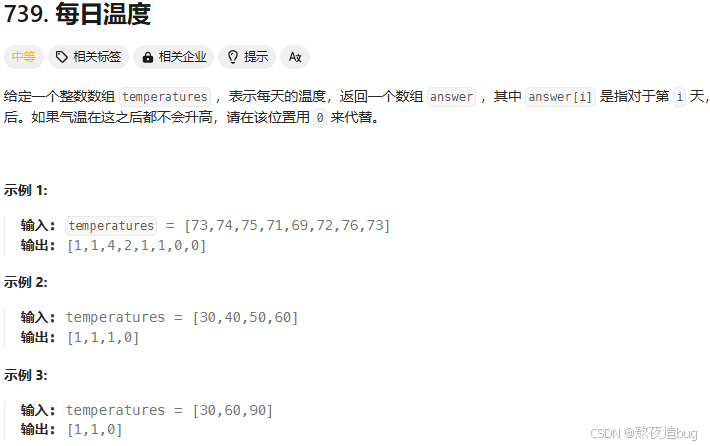
python
class Solution(object):
def dailyTemperatures(self, temperatures):
stack = []
T = temperatures
dicts = {i:0 for i in range(len(T))}
for i in range(len(T)):
while stack and T[stack[-1]] < T[i]:
dicts[stack[-1]] = i - stack[-1]
stack.pop()
stack.append(i)
return list(dicts.values())
5. 柱状图中最大的矩形
原题链接:84. 柱状图中最大的矩形 - 力扣(LeetCode)
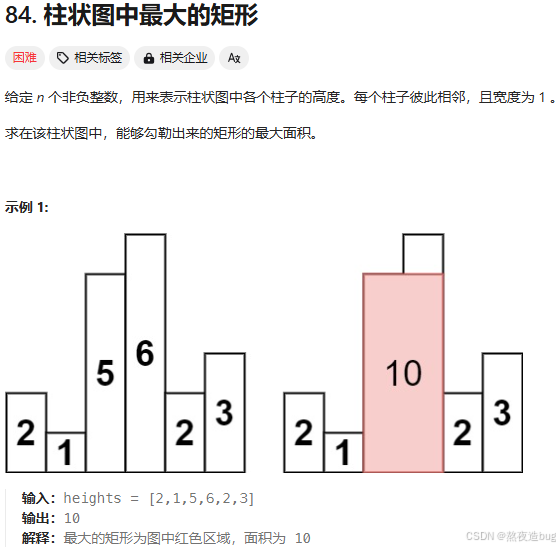
python
class Solution(object):
def largestRectangleArea(self, heights):
stack = []
heights = [0] + heights + [0]
res = 0
n = len(heights)
for i in range(n):
while stack and heights[stack[-1]] > heights[i]:
h=heights[stack.pop()]
w = i-1-stack[-1]
res = max(res, h*w) # h递减,w递增
stack.append(i)
return res
二、堆
1. 数组中的第K个最大元素
原题链接:215. 数组中的第K个最大元素 - 力扣(LeetCode)
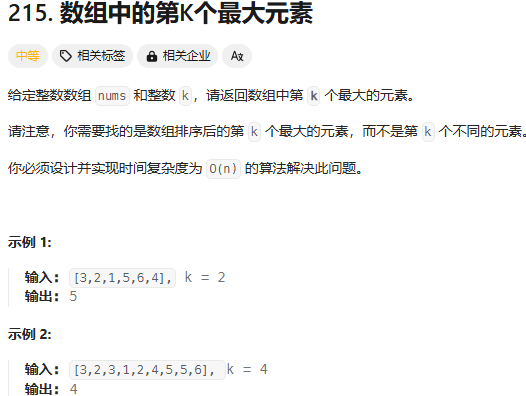
python
class Solution(object):
def findKthLargest(self, nums, k):
nums.sort()
nums.reverse()
return nums[k-1]
2. 前K个高频元素
原题链接:347. 前 K 个高频元素 - 力扣(LeetCode)
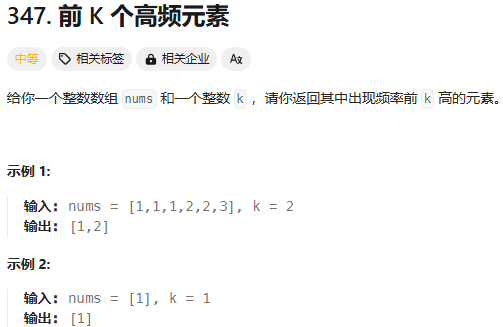
python
# 解法(1)
class Solution(object):
def topKFrequent(self, nums, k):
from collections import Counter
lst = []
for k, v in Counter(nums).most_common(k):
lst.append(k)
return lst
# 解法(2)
class Solution:
def topKFrequent(self, nums: List[int], k: int) -> List[int]:
snums = set(nums)
dicts = {k:0 for k in snums}
for n in nums:
if n in dicts:
dicts[n]+=1
dicts_sort = sorted(dicts.items(), key=lambda x: x[1], reverse=True)
lst = []
for k,v in dicts_sort[:k]:
lst.append(k)
return lst
3. 数据流中的中位数
原题链接:295. 数据流的中位数 - 力扣(LeetCode)
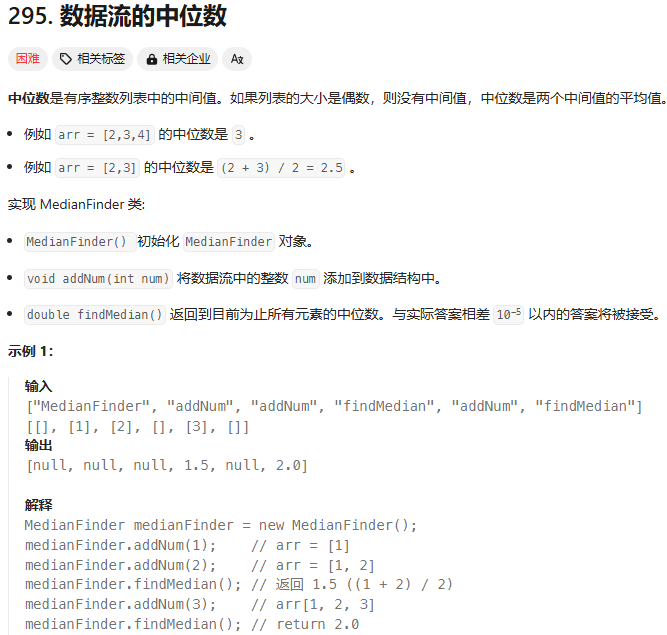
python
class MedianFinder(object):
def __init__(self):
self.lst = []
def addNum(self, num):
self.lst.append(num)
def findMedian(self):
self.lst.sort()
ln = len(self.lst)
if ln % 2 == 0:
mid = (self.lst[ln//2-1] + self.lst[ln//2]) / 2.0
else:
mid = self.lst[ln//2]
return mid