1、安装jdk21 for mac(jdk-21_macos-aarch64_bin.dmg)
2、安装neo4j for mac(neo4j-community-5.26.0-unix.tar.gz)
3、使用默认neo4j/neo4j登录http://localhost:7474
修改登录密码,可以使用生成按钮生成密码,连接数据库,默认设置为neo4j://localhost:7687。
3、具体代码如下:
python
import networkx as nx
import json
import requests
import matplotlib.pyplot as plt
from matplotlib.font_manager import FontProperties
from py2neo import Graph, Node, Relationship
# 设置字体路径
font_path = "/System/Library/Fonts/STHeiti Light.ttc" # macOS系统自带的黑体字体路径
font_prop = FontProperties(fname=font_path)
# 连接到Neo4j数据库
def connect_to_neo4j(uri, user, password):
graph = Graph(uri, auth=(user, password))
return graph
# 将知识树保存到Neo4j数据库
def save_knowledge_tree_to_neo4j(graph, tree):
# 清空数据库
graph.delete_all()
# 添加节点和关系
for node in tree.nodes():
neo4j_node = Node("Concept", name=node)
graph.create(neo4j_node)
for u, v in tree.edges():
u_node = graph.nodes.match("Concept", name=u).first()
v_node = graph.nodes.match("Concept", name=v).first()
if u_node and v_node:
rel = Relationship(u_node, "ANSWERED_BY", v_node)
graph.create(rel)
# 可视化知识树
def visualize_tree(tree):
pos = nx.spring_layout(tree, k=0.5, iterations=20) # 节点布局
nx.draw(tree, pos, with_labels=True, node_size=500, node_color="skyblue", font_size=8, font_weight='bold', font_family=font_prop.get_name())
plt.title("知识树", fontproperties=font_prop)
plt.axis('off') # 关闭坐标轴
plt.show()
# 示例对话内容
dialogue = """
客户:你好,我想了解一下产品的保修政策。
坐席:您好!我们的产品保修期为一年,从购买之日起计算。
客户:如果产品在保修期内出现故障,我该怎么办?
坐席:您可以在保修期内将产品送到我们指定的维修点进行免费维修。
客户:维修点在哪里?
坐席:您可以在我们的官方网站上查询最近的维修点地址。
客户:好的,谢谢!
坐席:不客气,祝您生活愉快!
"""
# 手动解析对话内容
def parse_dialogue(dialogue):
lines = dialogue.strip().split("\n")
questions = []
answers = []
for i in range(0, len(lines), 2):
question = lines[i].replace("客户:", "").strip()
answer = lines[i + 1].replace("坐席:", "").strip()
questions.append(question)
answers.append(answer)
return questions, answers
questions, answers = parse_dialogue(dialogue)
print("问题:", questions)
print("答案:", answers)
def build_knowledge_tree(questions, answers):
tree = nx.DiGraph()
root = "根据对话初始化的知识树"
tree.add_node(root)
for q, a in zip(questions, answers):
tree.add_node(q)
tree.add_edge(root, q)
tree.add_node(a)
tree.add_edge(q, a)
return tree
knowledge_tree = build_knowledge_tree(questions, answers)
# 可视化知识树
visualize_tree(knowledge_tree)
# 生成需要补充的问题
def generate_supplement_questions(questions, answers):
prompt = "基于以上对话内容,您认为还需要补充哪些问题?请以问题的形式列出。\n"
for q, a in zip(questions, answers):
prompt += f"问题:{q}\n答案:{a}\n"
prompt += "需要补充的问题:"
print('user:', prompt)
# 调用 Ollama API 生成补充问题
data = {
"model": "qwen2.5:14b",
"prompt": prompt,
"stream": False,
"temperature": 0.7,
"max_tokens": 200
}
try:
response = requests.post("http://127.0.0.1:11434/api/generate", json=data)
if response.status_code == 200:
return response.json().get("response", "")
else:
return f"API 请求失败,状态码:{response.status_code}"
except Exception as e:
return f"API 请求失败,错误信息:{e}"
# 生成需要补充的问题
supplement_questions = generate_supplement_questions(questions, answers)
print("assistant:需要补充的问题:", supplement_questions)
# 专家回答
def expert_answers(supplement_questions):
print("专家,请回答以下问题:")
print(supplement_questions)
answers = []
for q in supplement_questions.split("\n"):
if q.strip():
print(f"问题:{q}")
answer = input("专家回答输入:")
answers.append(answer)
return answers
# 专家回答
expert_answers_list = expert_answers(supplement_questions)
print("专家的回答:", expert_answers_list)
# 构建知识树
def build_knowledge_tree(questions, answers, supplement_questions, expert_answers):
tree = nx.DiGraph()
root = "专家补充回答后的知识树"
tree.add_node(root)
for q, a in zip(questions, answers):
tree.add_node(q)
tree.add_edge(root, q)
tree.add_node(a)
tree.add_edge(q, a)
for q, a in zip(supplement_questions.split("\n"), expert_answers):
if q.strip():
tree.add_node(q)
tree.add_edge(root, q)
tree.add_node(a)
tree.add_edge(q, a)
return tree
# 构建知识树
knowledge_tree = build_knowledge_tree(questions, answers, supplement_questions, expert_answers_list)
# 连接到Neo4j数据库
neo4j_uri = "neo4j://localhost:7687"
neo4j_user = "neo4j"
neo4j_password = "密码" # 替换为你的Neo4j密码(生成或设置的密码)
graph = connect_to_neo4j(neo4j_uri, neo4j_user, neo4j_password)
# 将知识树保存到Neo4j数据库
save_knowledge_tree_to_neo4j(graph, knowledge_tree)
# 查询功能
def query_knowledge_tree(graph, question):
query = """
MATCH (q:Concept {name: $question})-[:ANSWERED_BY]->(a:Concept)
RETURN a.name AS answer
"""
result = graph.run(query, question=question)
return [record["answer"] for record in result]
# 测试查询功能
for question in questions:
result = query_knowledge_tree(graph, question)
print("原始问题:", question)
if result:
print(f"查询结果:")
for node in result:
print(node)
else:
print("未找到相关问题的答案。")
for question in supplement_questions.split("\n"):
result = query_knowledge_tree(graph, question)
print("补充问题:", question)
if result:
print(f"查询结果:")
for node in result:
print(node)
else:
print("未找到相关问题的答案。")
4、显示效果:
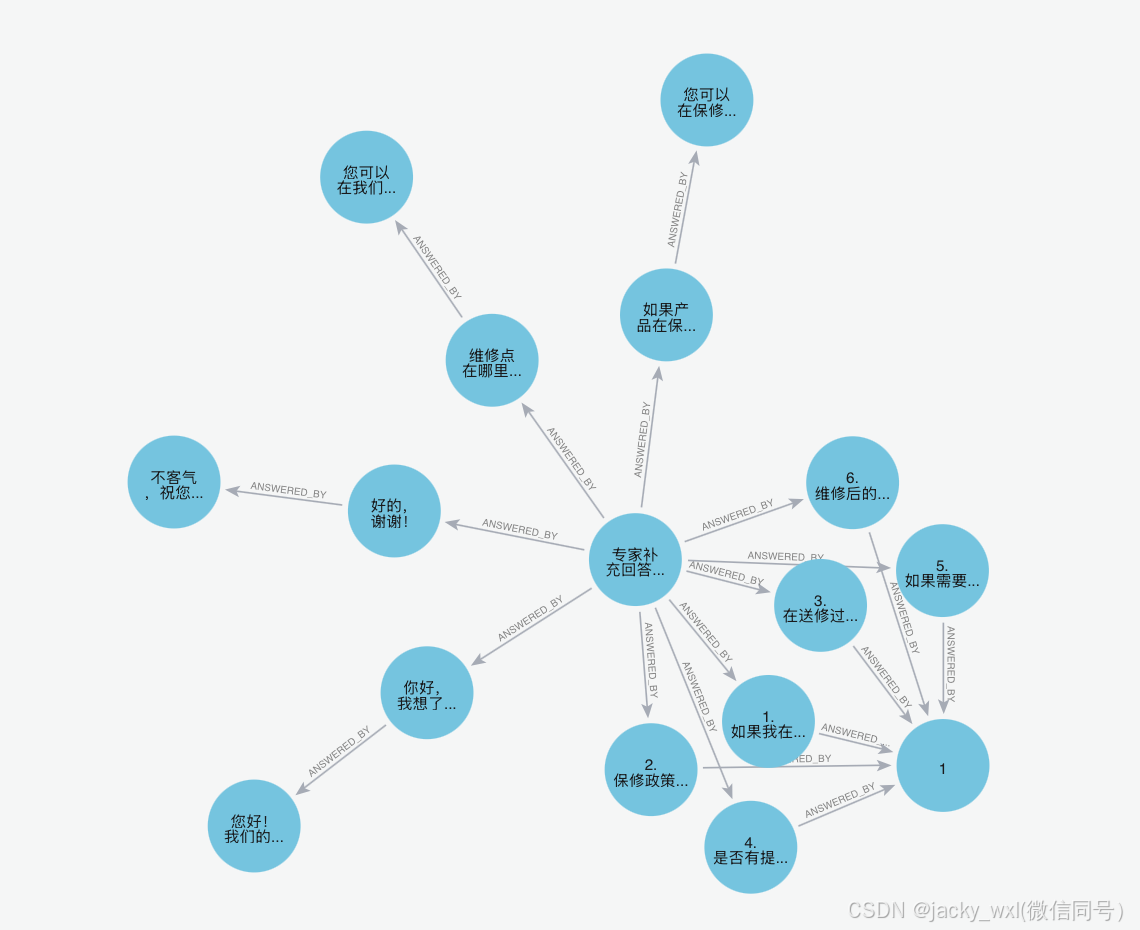