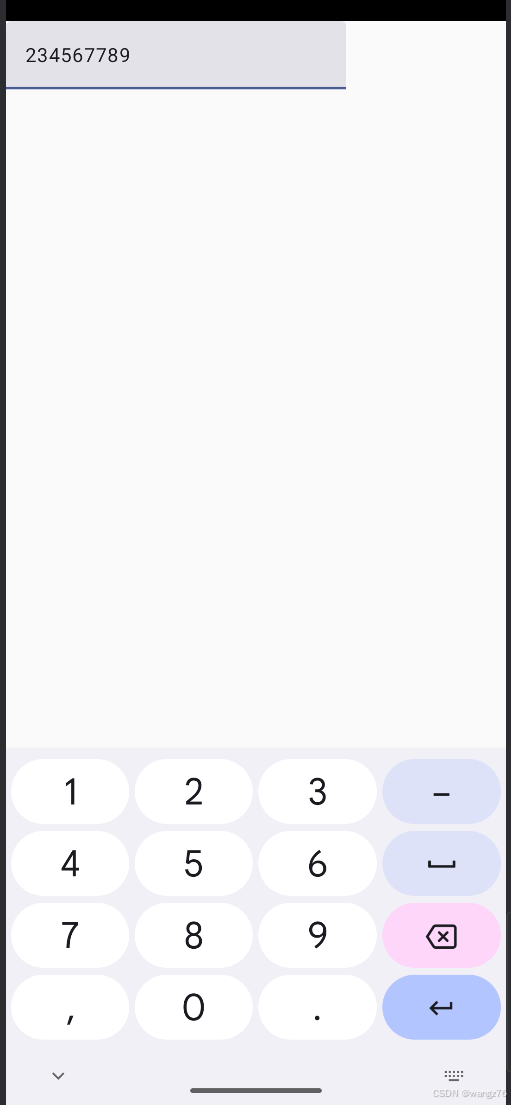
implementation ("androidx.datastore:datastore-preferences:1.0.0")
package com.example.mydatastore
import android.content.Context
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.activity.enableEdgeToEdge
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material3.TextField
import androidx.compose.runtime.*
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.text.input.KeyboardType
import androidx.datastore.core.DataStore
import androidx.datastore.preferences.core.Preferences
import androidx.datastore.preferences.core.edit
import androidx.datastore.preferences.core.intPreferencesKey
import androidx.datastore.preferences.preferencesDataStore
import com.example.mydatastore.ui.theme.MyDataStoreTheme
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.launch
// MainActivity 是应用的入口 Activity,继承自 ComponentActivity
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// 启用边缘到边缘的显示模式
enableEdgeToEdge()
// 设置 Compose 内容
setContent {
MyDataStoreTheme {
// 显示 NumberInputScreen 组件
NumberInputScreen()
}
}
}
}
// 定义 DataStore 的扩展属性,用于访问应用的设置数据存储
val Context.settingsDataStore: DataStore<Preferences> by preferencesDataStore(name = "my_settings")
// NumberInputScreen 是一个可组合函数,用于显示数字输入界面
@Composable
fun NumberInputScreen() {
// 获取当前上下文
val context = LocalContext.current
// 使用 remember 和 mutableStateOf 来保存输入的数字
var number by remember { mutableStateOf(0) }
// 使用 LaunchedEffect 在组合时执行一次,从 DataStore 读取初始值
LaunchedEffect(Unit) {
context.settingsDataStore.data.collect { preferences ->
number = preferences[PreferencesKeys.NUMBER] ?: 0
}
}
// 显示一个 TextField,用于输入数字
TextField(
value = number.toString(),
onValueChange = { newValue ->
// 将输入的值转换为整数,如果转换成功则更新状态并保存到 DataStore
newValue.toIntOrNull()?.let { num ->
number = num
// 在 IO 线程中异步保存到 DataStore
CoroutineScope(Dispatchers.IO).launch {
context.settingsDataStore.edit { settings ->
settings[PreferencesKeys.NUMBER] = num
}
}
}
},
// 设置键盘类型为数字键盘
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Number)
)
}
// PreferencesKeys 是一个单例对象,用于定义 DataStore 的键
private object PreferencesKeys {
val NUMBER = intPreferencesKey("saved_number")
}