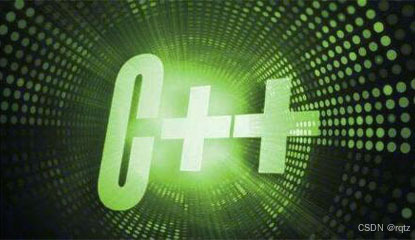

🔥个人主页 🔥
😈所属专栏😈
每文一诗 💪🏼
年年岁岁花相似,岁岁年年人不同 ------ 唐/刘希夷《代悲白头翁》
**译文:**年年岁岁繁花依旧,岁岁年年看花之人却不相同
目录
C++运算符重载概念
在 C++ 里,运算符重载属于多态的一种表现形式,它允许你为自定义的数据类型重新定义运算符的行为。
运算符重载其实就是对已有的运算符赋予新的功能,使它能够处理自定义类型的对象
C++运算符重载语法及作用
**语法:**返回类型 operator运算符(参数列表) {}
- 提高代码可读性 :在处理自定义类型时,使用重载后的运算符能让代码更直观、自然。例如,对于自定义的复数类,你可以重载
+
运算符,让两个复数相加的操作就像普通数字相加一样简单。 - 实现自定义类型的操作 :借助运算符重载,你能为自定义类型实现像内置类型那样的操作。比如,对于自定义的矩阵类,你可以重载
+
、-
、*
等运算符,实现矩阵的加减乘运算。 - 代码复用与一致性:运算符重载可以复用已有的运算符,使代码更具一致性。对于熟悉内置运算符的开发者来说,重载后的运算符也易于理解和使用。
加减乘除运算符重载
- 作类成员函数重载
cpp
#include <iostream>
class human
{
public:
human(){};
human(int a ,int b):m_a(a),m_b(b){
}
//加减乘除运算符重载(成员函数)
human operator*(const human& h){
human h2;
h2.m_a = this->m_a * h.m_a;
h2.m_b = this->m_b * h.m_b;
return h2;
}
int geta(){
return this->m_a;
}
int getb(){
return this->m_b;
}
private:
int m_a;
int m_b;
};
int main(int argc, char const *argv[])
{
human h(10,20);
human h1(2,10);
//相当于 h.operator*(h1)
human h3 = h * h1;
std::cout << h3.geta() <<" "<< h3.getb()<<std::endl;
return 0;
}
代码解读:
提供默认无参构造函数 和函数初始化列表
cpphuman(){}; human(int a ,int b):m_a(a),m_b(b){ }
加减乘除运算符重载(成员函数)这里指的是乘法
cpphuman operator*(const human& h){ human h2; h2.m_a = this->m_a * h.m_a; h2.m_b = this->m_b * h.m_b; return h2; }
- 这里的参数是指常量引用 :目的是不使用值传递的方式,防止对数据进行大量的拷贝,常量引用本质使用地址传递,地址通常占有4个字节,程序效率更高
- 这里的this指向调用该重载函数的哪个对象。
- 在栈区重新创建一个对象h,并将运算后的成员变量更新到该对象并返回。
cpphuman h3 = h * h1;
这个相当于 h.operator*(h1)
*
cppstd::cout << h3.geta() <<" "<< h3.getb()<<std::endl;
因为成员变量m_a和m_b时私有变量,不能在类外访问,所以在类内使用函数来访问。
输出
这样就实现了类型为human的变量使用运算符来进行运算,20 = 10 *2 ;200 = 20*10
其他加减除法以此类推。
2.作全局函数重载
cpp
#include <iostream>
class human
{
friend human operator*(const human& p2, int val);
public:
human(){};
human(int a ,int b):m_a(a),m_b(b){
}
//加减乘除运算符重载(成员函数)
human operator*(const human& h){
human h2;
h2.m_a = this->m_a * h.m_a;
h2.m_b = this->m_b * h.m_b;
return h2;
}
int geta(){
return this->m_a;
}
int getb(){
return this->m_b;
}
private:
int m_a;
int m_b;
};
// 加减乘除运算符重载(全局函数)访问私有成员需要加friend声明
human operator*(const human& p2, int val)
{
human temp;
temp.m_a = p2.m_a *val;
temp.m_b = p2.m_b * val;
return temp;
}
int main(int argc, char const *argv[])
{
human h(10,20);
human h1(2,10);
//相当与 operator*(h,90)
human h2 = h * 90;
//相当于 h.operator*(h1)
human h3 = h * h1;
std::cout << h2.geta() <<" "<< h2.getb()<<std::endl;
std::cout << h3.geta() <<" "<< h3.getb()<<std::endl;
return 0;
}
代码解读:
- 加减乘除运算符重载(全局函数)访问私有成员需要加friend声明
cpphuman operator*(const human& p2, int val) { human temp; temp.m_a = p2.m_a *val; temp.m_b = p2.m_b * val; return temp; }
该函数在类外定义,在类外定义的函数不能访问类内的私有变量,但是可以通过友元函数的方法来让类外定义的全局函数访问类内的私有变量。即
cppfriend human operator*(const human& p2, int val);
cpphuman h2 = h * 90;
相当与 operator*(h,90)调用的是全局函数。
- 输出
赋值运算符重载
返回值作为引用以实现链式赋值
cpp
#include <iostream>
class human
{
public:
human(){};
human(int a ,int b,int c):m_a(a),m_b(b),m_c(new int(c)){
}
//赋值运算符重载
human& operator=(const human& h){
if(this != &h){
if(m_c != nullptr){
delete m_c;
m_c = nullptr;
}
}
m_c = new int(*h.m_c);
return *this;
}
int geta(){
return this->m_a;
}
int getb(){
return this->m_b;
}
int getc(){
return *this->m_c;
}
private:
int m_a;
int m_b;
int* m_c;
};
int main(int argc, char const *argv[])
{
human h(10,20,12);
human h1(2,10,34);
human h2(22,10,4);
h = h1 = h2;
std::cout <<h.getc()<<std::endl;
return 0;
}
代码解读:
函数初始化列表来初始化成员变量
cpphuman(int a ,int b,int c):m_a(a),m_b(b),m_c(new int(c)){ }
赋值运算符重载
cpphuman& operator=(const human& h){ if(this != &h){ if(m_c != nullptr){ delete m_c; m_c = nullptr; } } m_c = new int(*h.m_c); return *this; }
首先使用this来判断调用该函数的对象是否和传入的对象是同一块内存,接着在判断调用该成员函数的对象中的成员变量m_c是否不为空,不为空则释放内存,再置为空。
两个对象不是同一块内存(this != &h)需要delete,再重新开辟内存。
**这样作的目的是用于:如果对一个成员变量是指针的对象,将该指针指向的值进行修改时,需要先释放调用该重载函数的对象中指针变量,再为空,然后在m_c = new int(*h.m_c);**重新开辟一块内存,内存的值是参数中对象的那个值。
原因: 若不释放这块内存,在赋值操作完成后,原来的内存就会变成无法访问的 "孤儿" 内存,程序无法再释放它,从而造成内存泄漏。执行
delete m_c;
就能正确释放这块内存,让系统可以重新使用它。两个对象是同一块内存**(this == &h)** 不需要delete。
- **原因:**当两个对象是统一块内存时,m_c这个指针变量指向的值是一样的,如果对其执行
delete m_c,
那么在后面
**m_c = new int(*h.m_c);**时使用*h.m_c时是非法的,因为这块内存已经被释放掉了,不能访问。为什么要m_c = new int(*h.m_c);
- 原因:编译器默认提供的是浅拷贝,对于一个含有指针变量的类中,浅拷贝会对这块内存多次释放,是不合法的,所以需要执行深拷贝来使得两个对象中的指针变量指向的值相同,但指针的值不同(地址不同)
- 有关【C++面向对象】封装(上):探寻构造函数的幽微之境-CSDN博客深拷贝,浅拷贝【C++面向对象】封装(上):探寻构造函数的幽微之境-CSDN博客
为什么return *this; 返回值类型是human& 即引用。
- 原因:this指向调用该成员函数的那个对象,而*this是指的该对象,而返回值是human&引用是为了实现链式调用,而返回值是human不能实现链式赋值,因为他返回的是该对象的拷贝。
关系运算符重载
大于号运算符重载
cpp
#include <iostream>
class human
{
friend bool operator>(const human& h1,int val);
public:
human(){};
human(int a ,int b,int c):m_a(a),m_b(b),m_c(new int(c)){
}
//关系运算符重载
bool operator>(const human& h1){
if(this->m_a > h1.m_a && this->m_b > h1.m_b)
return true;
else
return false;
}
int geta(){
return this->m_a;
}
int getb(){
return this->m_b;
}
int getc(){
return *this->m_c;
}
private:
int m_a;
int m_b;
int* m_c;
};
//关系运算符重载(全局函数)访问私有成员需要加friend声明
bool operator>(const human& h1,int val){
if(val< h1.m_a && val < h1.m_b)
return true;
else
return false;
}
int main(int argc, char const *argv[])
{
human h(10,20,12);
human h1(2,10,34);
//相当于 h.operator>(h1)
if(h > h1)
std::cout<<"h>h1"<<std::endl;
else
std::cout<<"h<h1"<<std::endl;
//相当与 operator>(h,12)
if(h > 12)
std::cout<<"h>12"<<std::endl;
else
std::cout<<"h<12"<<std::endl;
return 0;
}
代码解读:
上段代码分别用成员函数和全局函数的方法来实现对自定义类型的判断
- 对于
cpph > h1
相当于 h.operator>(h1)
- 对于
cpph > 12
相当与 operator>(h,12)
- 输出
相等运算符重载
cpp
#include <iostream>
class human
{
friend bool operator==(const human& h1,int val);
public:
human(){};
human(int a ,int b,int c):m_a(a),m_b(b),m_c(new int(c)){
}
//关系运算符重载
bool operator==(const human& h1){
if(this->m_a == h1.m_a && this->m_b == h1.m_b)
return true;
else
return false;
}
int geta(){
return this->m_a;
}
int getb(){
return this->m_b;
}
int getc(){
return *this->m_c;
}
private:
int m_a;
int m_b;
int* m_c;
};
//关系运算符重载(全局函数)访问私有成员需要加friend声明
bool operator==(const human& h1,int val){
if(val == h1.m_a)
return true;
else
return false;
}
int main(int argc, char const *argv[])
{
human h(10,20,12);
human h1(2,10,34);
//相当于 h.operator==(h1)
if(h == h1)
std::cout<<"二者相等"<<std::endl;
else
std::cout<<"二者不等"<<std::endl;
//相当与 operator==(h,23)
if(h == 23)
std::cout<<"二者相等"<<std::endl;
else
std::cout<<"二者不等"<<std::endl;
return 0;
}
代码解读:
上段代码分别用成员函数和全局函数的方法来实现对自定义类型的相等判断
- 对于
cpph == h1
相当于相当于 h.operator==(h1)
- 对于
cpph == 23
相当于 operator==(h,23)
- 输出
全部代码
cpp
#include <iostream>
class human
{
friend human operator*(const human& p2, int val);
friend bool operator==(const human& h1,int val);
friend bool operator>(const human& h1,int val);
public:
human(){};
human(int a ,int b,int c):m_a(a),m_b(b),m_c(new int(c)){
}
//加减乘除运算符重载(成员函数)
human operator*(const human& h){
human h2;
h2.m_a = this->m_a * h.m_a;
h2.m_b = this->m_b * h.m_b;
return h2;
}
//关系运算符重载
bool operator==(const human& h1){
if(this->m_a == h1.m_a && this->m_b == h1.m_b)
return true;
else
return false;
}
//关系运算符重载
bool operator>(const human& h1){
if(this->m_a > h1.m_a && this->m_b > h1.m_b)
return true;
else
return false;
}
//赋值运算符重载
human& operator=(const human& h){
if(this != &h){
if(m_c != nullptr){
delete m_c;
m_c = nullptr;
}
}
m_c = new int(*h.m_c);
return *this;
}
int geta(){
return this->m_a;
}
int getb(){
return this->m_b;
}
int getc(){
return *this->m_c;
}
private:
int m_a;
int m_b;
int* m_c;
};
// 加减乘除运算符重载(全局函数)访问私有成员需要加friend声明
human operator*(const human& p2, int val)
{
human temp;
temp.m_a = p2.m_a *val;
temp.m_b = p2.m_b * val;
return temp;
}
//关系运算符重载(全局函数)访问私有成员需要加friend声明
bool operator==(const human& h1,int val){
if(val == h1.m_a)
return true;
else
return false;
}
//关系运算符重载(全局函数)访问私有成员需要加friend声明
bool operator>(const human& h1,int val){
if(val< h1.m_a && val < h1.m_b)
return true;
else
return false;
}
int main(int argc, char const *argv[])
{
human h(10,20,12);
human h1(2,10,34);
//相当与 operator*(h,90)
human h2 = h * 90;
//相当于 h.operator*(h1)
human h3 = h * h1;
//相当于 h.operator==(h1)
if(h == h1)
std::cout<<"二者相等"<<std::endl;
else
std::cout<<"二者不等"<<std::endl;
//相当与 operator==(h,23)
if(h == 23)
std::cout<<"二者相等"<<std::endl;
else
std::cout<<"二者不等"<<std::endl;
//相当于 h.operator>(h1)
if(h > h1)
std::cout<<"h>h1"<<std::endl;
else
std::cout<<"h<h1"<<std::endl;
//相当与 operator>(h,12)
if(h > 12)
std::cout<<"h>12"<<std::endl;
else
std::cout<<"h<12"<<std::endl;
std::cout << h2.geta() <<" "<< h2.getb()<<std::endl;
std::cout << h3.geta() <<" "<< h3.getb()<<std::endl;
h = h1;
std::cout <<h.getc()<<std::endl;
return 0;
}
🔥个人主页 🔥
😈所属专栏😈
