Vue2 中使用 gmap - marker 和 GmapCluster 实现谷歌地图标点与聚合
一、安装与配置依赖
在项目开始前,请确保已安装vue2-google-maps
依赖。若尚未安装,在项目根目录下运行以下命令:
npm install vue2-google-maps
随后,在main.js
文件中进行基础配置,引入你的谷歌地图 API 密钥(可在谷歌云平台申请):
javascript
import Vue from 'vue';
import App from './App.vue';
import { VueGoogleMaps } from 'vue2-google-maps';
Vue.use(VueGoogleMaps, {
load: {
key: 'YOUR\_API\_KEY',
libraries: 'places' // 若需使用地点相关功能,可添加此选项
}
});
new Vue({
render: h => h(App)
}).$mount('#app');
二、创建地图组件
在src/components
目录下创建地图组件,例如GoogleMapWithCluster.vue
。
xml
<template>
<gmap-map
:center="{lat: 37.7749, lng: -122.4194}"
:zoom="12"
map-type-id="roadmap"
style="width: 100%; height: 500px;"
>
<gmap-cluster>
<gmap-marker
v-for="(marker, index) in markers"
:key="index"
:position="marker.position"
:title="marker.title"
/>
</gmap-cluster>
</gmap-map>
</template>
<script>
import { GmapMap, GmapMarker } from 'vue2-google-maps';
import GmapCluster from 'vue2-google-maps/src/components/cluster';
export default {
components: {
GmapMap,
GmapMarker,
GmapCluster
},
data() {
return {
markers: [
{
position: { lat: 37.7749, lng: -122.4194 },
title: 'Marker 1'
},
{
position: { lat: 37.7761, lng: -122.4185 },
title: 'Marker 2'
},
{
position: { lat: 37.7755, lng: -122.4178 },
title: 'Marker 3'
}
// 可按需添加更多标记数据
]
};
}
};
</script>
<style scoped>
/\* 地图样式可依需求调整 \*/
</style>
代码说明:
模板部分通过gmap-map
组件创建地图,设置了地图的中心坐标、缩放级别和地图类型。
将gmap-cluster
作为gmap-map
的子组件,在gmap-cluster
内部通过v-for
循环渲染gmap-marker
组件,每个gmap-marker
对应一组标记数据,以此实现标点和聚合的展示。
脚本部分引入所需的GmapMap
、GmapMarker
和GmapCluster
组件,并在components
选项中进行局部注册。同时,在data
中定义markers
数组,用于存储标记数据。
三、使用地图组件
在App.vue
文件中使用刚创建的GoogleMapWithCluster.vue
组件:
xml
<template>
<div id="app">
<GoogleMapWithCluster />
</div>
</template>
<script>
import GoogleMapWithCluster from './components/GoogleMapWithCluster.vue';
export default {
components: {
GoogleMapWithCluster
}
};
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
下图是效果(样式是经过修改):
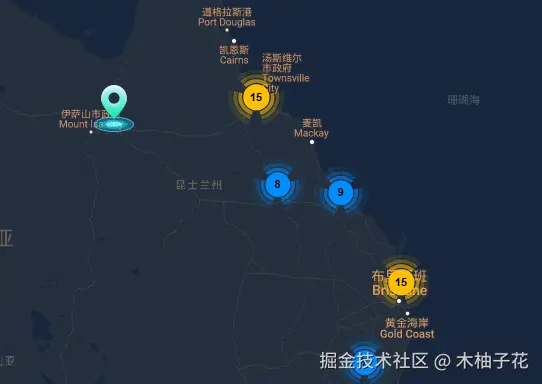