本文介绍了管理 Go Context 的检验法则和最佳实践,从而有助于创建更健壮的 Go 应用程序。原文:Go Context Best Practices: A Better Way to Store Values
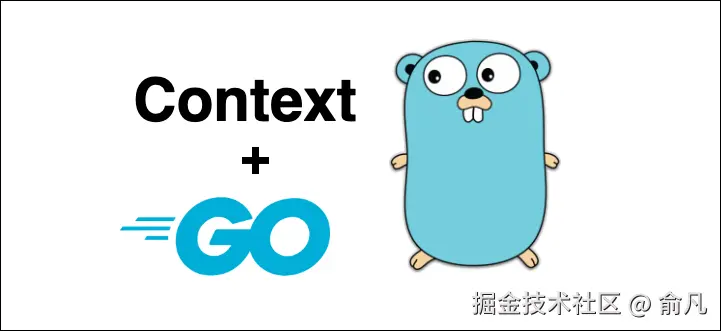
上下文(Context)管理是编写简洁、可维护 Go 代码的重要方面,对于服务端应用尤为重要。本文将和大家分享一些关于在上下文中存储值的见解,并告诉大家如何从常见的做法发展为更强大的解决方案。
通用方法:全局字符串键
许多开发人员从最简单的方法开始 -- 使用全局字符串键:
golang
package main
// 常见(但并非理想)的方法
const (
KeyUserID = "user_id"
KeyEmail = "email"
KeyRole = "role"
)
func storeUserInfo(ctx context.Context, userID, email, role string) context.Context {
ctx = context.WithValue(ctx, KeyUserID, userID)
ctx = context.WithValue(ctx, KeyEmail, email)
ctx = context.WithValue(ctx, KeyRole, role)
return ctx
}
func getUserInfo(ctx context.Context) (string, string, string) {
userID := ctx.Value(KeyUserID).(string)
email := ctx.Value(KeyEmail).(string)
role := ctx.Value(KeyRole).(string)
return userID, email, role
}
这种方法的问题:
- 类型安全:类型断言会在运行时引起 panic
- 名称冲突:字符串键可能会在软件包之间发生冲突
- 多重查询:每个值都需要单独的上下文查找
- 缺乏 IDE 支持:没有可用上下文值的自动完成功能
- 无封装:单独存储值,无逻辑分组
更好的方法:结构化上下文值
下面是一种更稳妥的方法,使用结构化类型和私有上下文键:
golang
package userctx
// 用私有类型作为上下文键以避免冲突
type contextKey struct{}
// ContextValue 保存所有用户相关的上下文值
type ContextValue struct {
UserID string
Name string
Email string
Role string
}
// NewContext 基于用户值创建新的上下文
func NewContext(ctx context.Context, val *ContextValue) context.Context {
return context.WithValue(ctx, contextKey{}, val)
}
// FromContext 从上下文中获取用户值
func FromContext(ctx context.Context) *ContextValue {
v, ok := ctx.Value(contextKey{}).(*ContextValue)
if !ok {
return nil
}
return v
}
使用示例:
golang
func HandleRequest(w http.ResponseWriter, r *http.Request) {
// 保存值
ctx := userctx.NewContext(r.Context(), &userctx.ContextValue{
UserID: "123",
Name: "John Doe",
Email: "john@example.com",
Role: "admin",
})
// 获取值
if userInfo := userctx.FromContext(ctx); userInfo != nil {
log.Printf("User %s (%s) accessing the system",
userInfo.Name, userInfo.Role)
}
// 将上下文传递给其他函数
processRequest(ctx)
}
这种方法的好处:
- 类型安全
- 有类型的结构字段
- 无需运行时类型断言
- 编译时类型检查
- 封装
- 私有上下文键可防止被外部修改
- 清晰的封装边界
- 自洽实现
- 更好的开发体验
- IDE 支持结构化字段的自动完成功能
- 轻松添加新字段
- 通过结构标签提供清晰的文档
- 单一上下文查询
- 一次操作检索所有值
- 更好的性能
- 更简单的错误处理
- 可维护性
- 结构易于修改
- 明确的依赖管理
- 必要时可进行集中验证
最佳实现方法
- 经常检查是否为 Nil
golang
func ProcessUserData(ctx context.Context) error {
userData := userctx.FromContext(ctx)
if userData == nil {
return errors.New("user data not found in context")
}
// 处理数据...
}
- 使用软件包级范围
golang
// userctx/context.go
package userctx
// 将实现细节封装在包内
// 只导出必要接口
- 考虑不变性
golang
type ContextValue struct {
userID string // 私有字段
// ... 其他字段
// 公开取值函数
UserID() string { return cv.userID }
}
结论
虽然全局字符串键的方法乍看起来可能更简单,但使用结构化上下文值在类型安全性、可维护性和开发体验方面有很多好处,可以更好的支撑不断增长的代码库,并有助于防止出现常见的运行时错误。
请记住:上下文值应用于传输 API 请求生命周期内的数据,而不是用于向函数传递可选参数。请将上下文值的重点放在用户身份验证、请求跟踪和截止日期等横向问题上。
通过遵循这些实践,将会有助于创建更健壮、更易维护的 Go 应用程序,而且更容易调试和扩展。
你好,我是俞凡,在Motorola做过研发,现在在Mavenir做技术工作,对通信、网络、后端架构、云原生、DevOps、CICD、区块链、AI等技术始终保持着浓厚的兴趣,平时喜欢阅读、思考,相信持续学习、终身成长,欢迎一起交流学习。为了方便大家以后能第一时间看到文章,请朋友们关注公众号"DeepNoMind",并设个星标吧,如果能一键三连(转发、点赞、在看),则能给我带来更多的支持和动力,激励我持续写下去,和大家共同成长进步!