Eclipse 插件开发 4 工具栏
- [1 增加工具(push)](#1 增加工具(push))
- [2 增加工具(toggle)](#2 增加工具(toggle))
- [3 增加工具(radio)](#3 增加工具(radio))
位置 | locationURI | 备注 |
---|---|---|
菜单栏 | menu:org.eclipse.ui.main.menu | 添加到传统菜单 |
工具栏 | toolbar:org.eclipse.ui.main.toolbar | 添加到工具栏 |
style 值 | 含义 | 显示效果 |
---|---|---|
push | 普通按钮(默认) | 普通的点击按钮,点一下执行一次 |
toggle | 切换按钮 | 有按下/弹起两种状态,比如"开关" |
radio | 单选按钮 | 多个按钮互斥选择,比如 "模式切换" |
1 增加工具(push)
java
package com.xu.learn.handlers;
import org.eclipse.core.commands.AbstractHandler;
import org.eclipse.core.commands.ExecutionEvent;
import org.eclipse.core.commands.ExecutionException;
import org.eclipse.ui.IWorkbenchWindow;
import org.eclipse.ui.handlers.HandlerUtil;
import org.eclipse.jface.dialogs.MessageDialog;
public class SampleHandler extends AbstractHandler {
@Override
public Object execute(ExecutionEvent event) throws ExecutionException {
IWorkbenchWindow window = HandlerUtil.getActiveWorkbenchWindowChecked(event);
MessageDialog.openInformation(
window.getShell(),
"Learn",
"点击菜单弹框");
return null;
}
}
xml
在<?xml version="1.0" encoding="UTF-8"?>
<?eclipse version="3.4"?>
<plugin>
<extension point="org.eclipse.ui.menus">
<menuContribution locationURI="toolbar:org.eclipse.ui.main.toolbar">
<toolbar id="com.example.toolbar">
<command commandId="com.example.commands.helloCommand" icon="icons/sample.png" tooltip="点我执行命令" label="按钮名称" style="push">
</command>
</toolbar>
</menuContribution>
</extension>
<extension point="org.eclipse.ui.handlers">
<handler class="com.xu.learn.handlers.SampleHandler" commandId="com.example.commands.helloCommand">
</handler>
</extension>
</plugin>
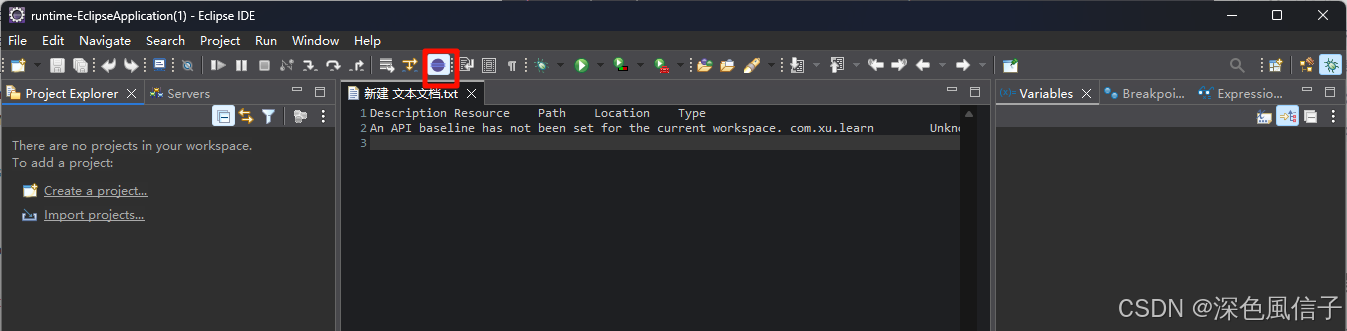
2 增加工具(toggle)
java
package com.xu.learn.handlers;
import org.eclipse.core.commands.AbstractHandler;
import org.eclipse.core.commands.Command;
import org.eclipse.core.commands.ExecutionEvent;
import org.eclipse.core.commands.ExecutionException;
import org.eclipse.jface.commands.ToggleState;
import org.eclipse.jface.preference.IPreferenceStore;
import org.eclipse.ui.handlers.HandlerUtil;
public class ToggleHandler extends AbstractHandler {
private static final String STATS = "org.eclipse.ui.commands.toggleState";
private static IPreferenceStore preferenceStore;
public static void setPreferenceStore(IPreferenceStore store) {
preferenceStore = store;
}
@Override
public Object execute(ExecutionEvent event) throws ExecutionException {
Command command = event.getCommand();
// 获取状态
ToggleState state = (ToggleState) command.getState(STATS);
if (state == null) {
state = new ToggleState();
command.addState(STATS, state);
}
// 打印状态
boolean currentState = HandlerUtil.toggleCommandState(command);
System.out.println("当前按钮状态:" + currentState);
// 保存状态
if (preferenceStore != null) {
preferenceStore.setValue(STATS, currentState);
}
return null;
}
}
xml
<?xml version="1.0" encoding="UTF-8"?>
<?eclipse version="3.4"?>
<plugin>
<extension point="org.eclipse.ui.commands">
<command id="com.example.commands.toggleCommand" name="切换按钮命令"/>
</extension>
<extension point="org.eclipse.ui.handlers">
<handler class="com.xu.learn.handlers.ToggleHandler" commandId="com.example.commands.toggleCommand"/>
</extension>
<extension point="org.eclipse.ui.menus">
<menuContribution locationURI="toolbar:org.eclipse.ui.main.toolbar">
<toolbar id="com.example.toolbar">
<command commandId="com.example.commands.toggleCommand" icon="icons/sample.png" style="toggle" tooltip="开关按钮示例"/>
</toolbar>
</menuContribution>
</extension>
</plugin>
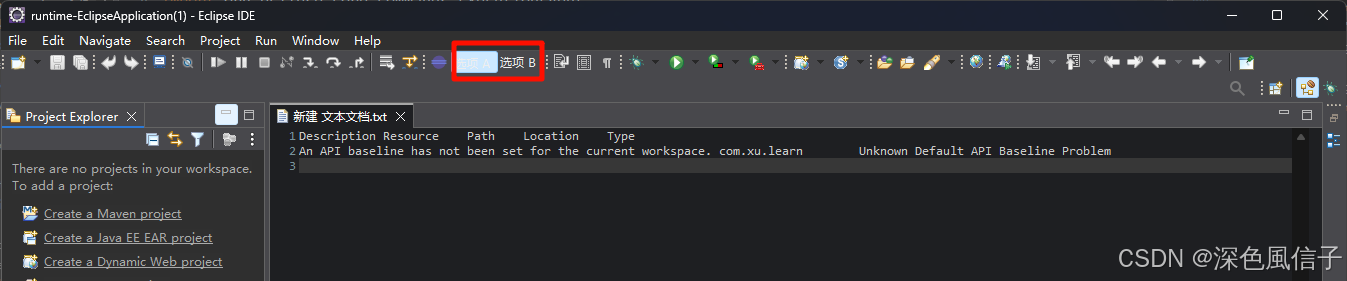
3 增加工具(radio)
java
package com.xu.learn.handlers;
import org.eclipse.core.commands.AbstractHandler;
import org.eclipse.core.commands.ExecutionEvent;
import org.eclipse.core.commands.ExecutionException;
public class RadioHandler extends AbstractHandler {
@Override
public Object execute(ExecutionEvent event) throws ExecutionException {
// 拿到参数
String option = event.getParameter("option");
System.out.println("当前选中的Radio按钮是:选项 " + option);
// 这里可以根据 option 做不同处理
if ("1".equals(option)) {
// 选中了第一个
} else if ("2".equals(option)) {
// 选中了第二个
} else if ("3".equals(option)) {
// 选中了第三个
}
return null;
}
}
xml
<?xml version="1.0" encoding="UTF-8"?>
<?eclipse version="3.4"?>
<plugin>
<extension point="org.eclipse.ui.commands">
<command id="com.example.commands.radioCommand" name="单选按钮命令">
<commandParameter id="option" name="选项" optional="true"/>
</command>
</extension>
<extension point="org.eclipse.ui.handlers">
<handler class="com.xu.learn.handlers.RadioHandler" commandId="com.example.commands.radioCommand"/>
</extension>
<extension point="org.eclipse.ui.menus">
<menuContribution locationURI="toolbar:org.eclipse.ui.main.toolbar">
<toolbar id="com.example.toolbar">
<command commandId="com.example.commands.radioCommand" icon="icons/sample.png" label="选项1" style="radio">
<parameter name="option" value="1"/>
</command>
<command commandId="com.example.commands.radioCommand" icon="icons/sample.png" label="选项2" style="radio">
<parameter name="option" value="2"/>
</command>
<command commandId="com.example.commands.radioCommand" icon="icons/sample.png" label="选项3" style="radio">
<parameter name="option" value="3"/>
</command>
</toolbar>
</menuContribution>
</extension>
</plugin>
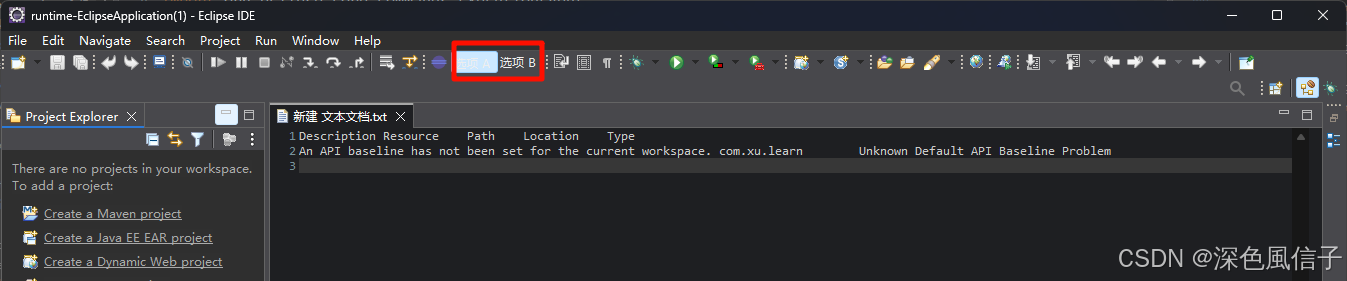