Redis简介:
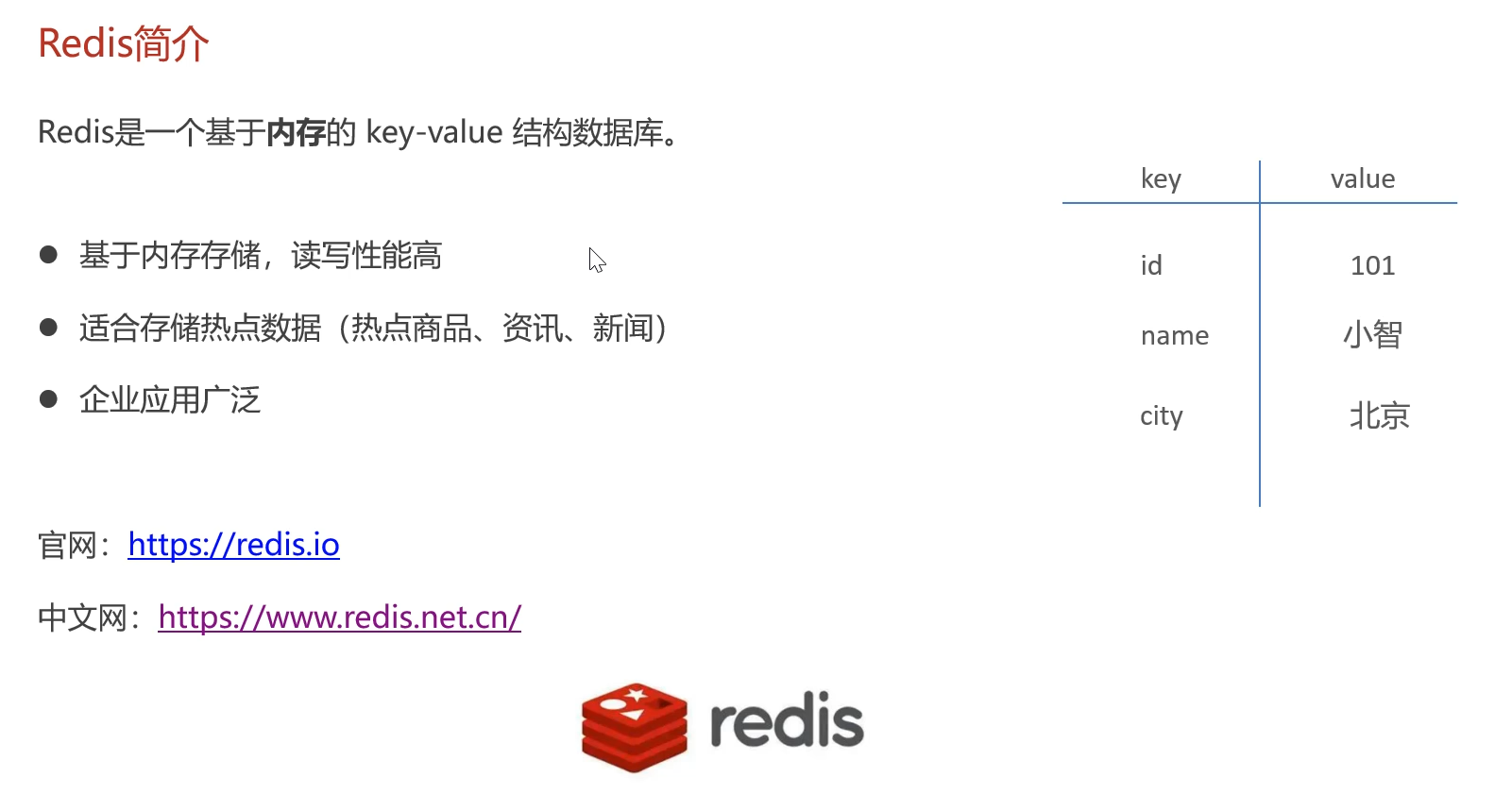
rRedis服务开启与停止:
服务开启:
在Redis配置文件中输入cmd进入命令行输入redis-server
redis-cli.exe -h -p:连接到redis服务
设置密码:在redis.windows.conf中找到requirepass 密码
服务停止:
在服务开启的界面按ctrl+c
Redis数据类型:
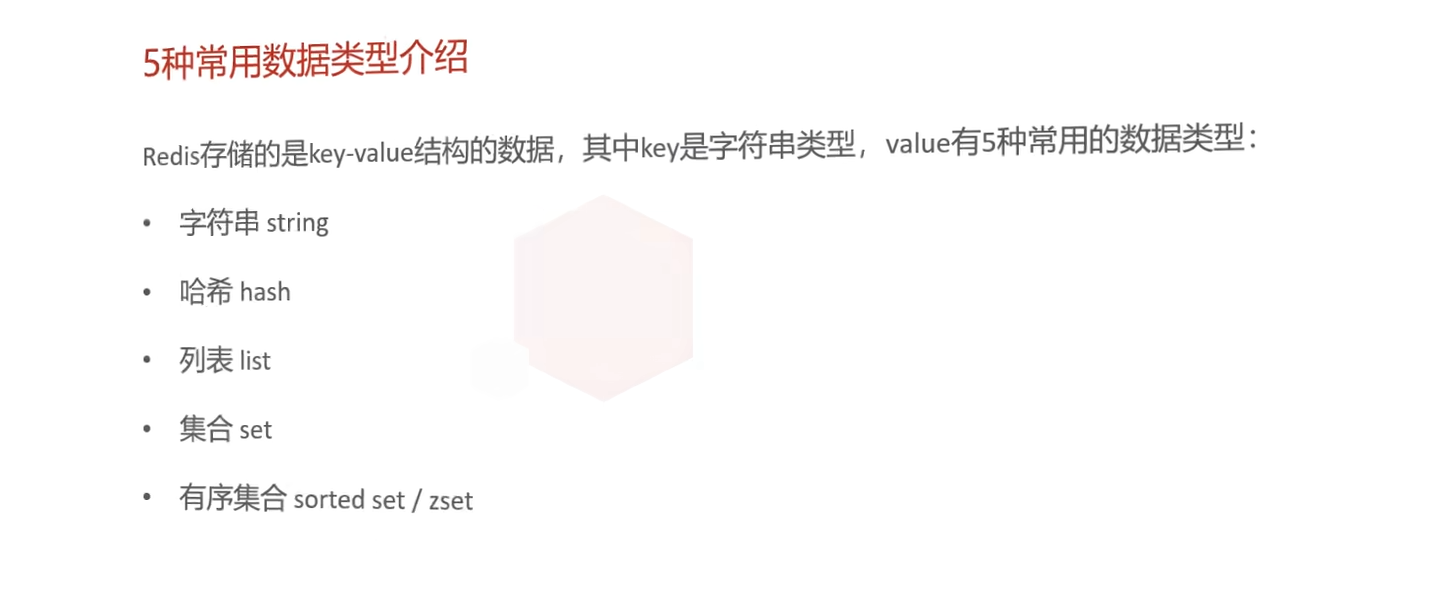
各种数据类型的特点:
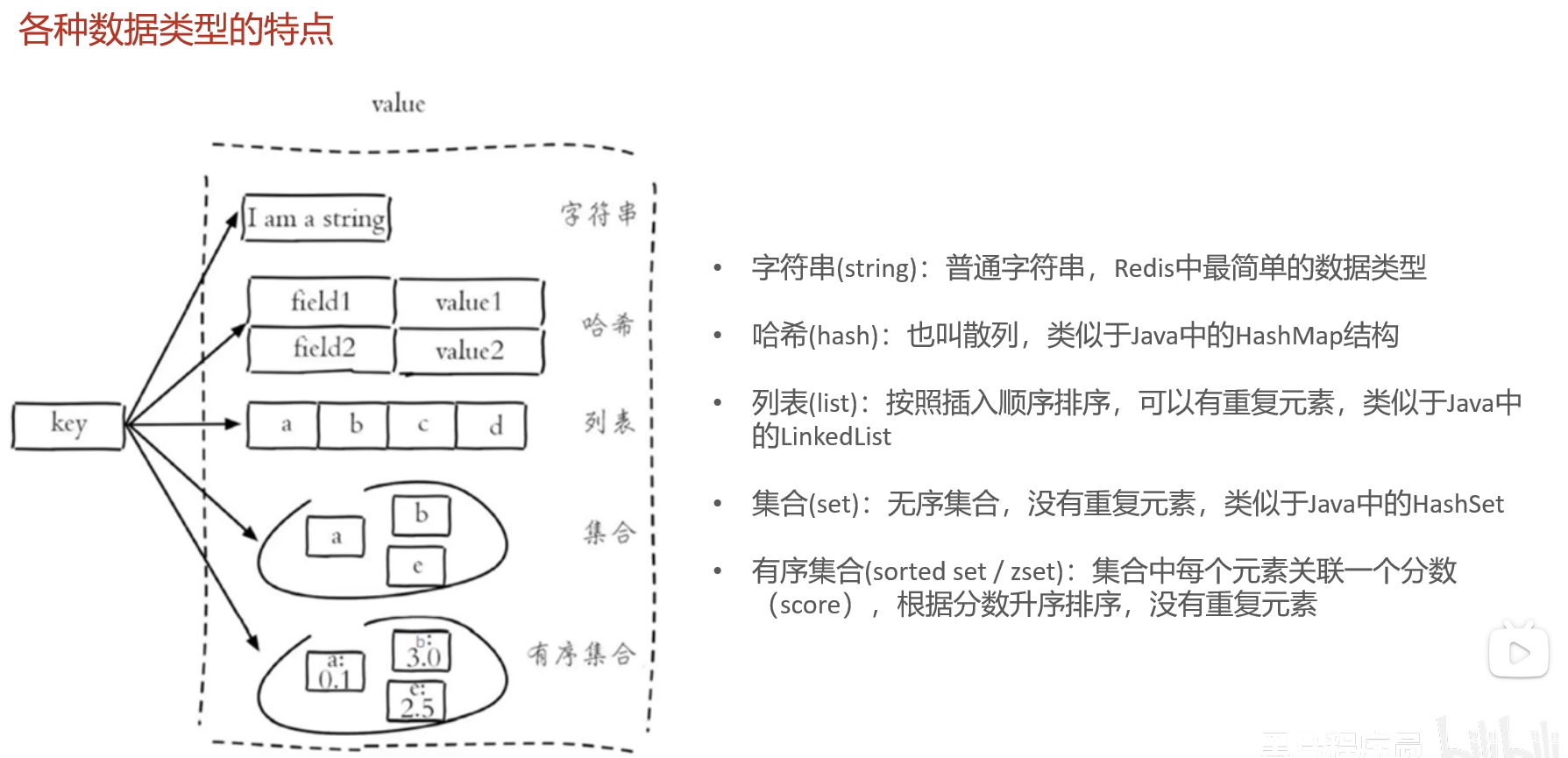
Redis常用命令:
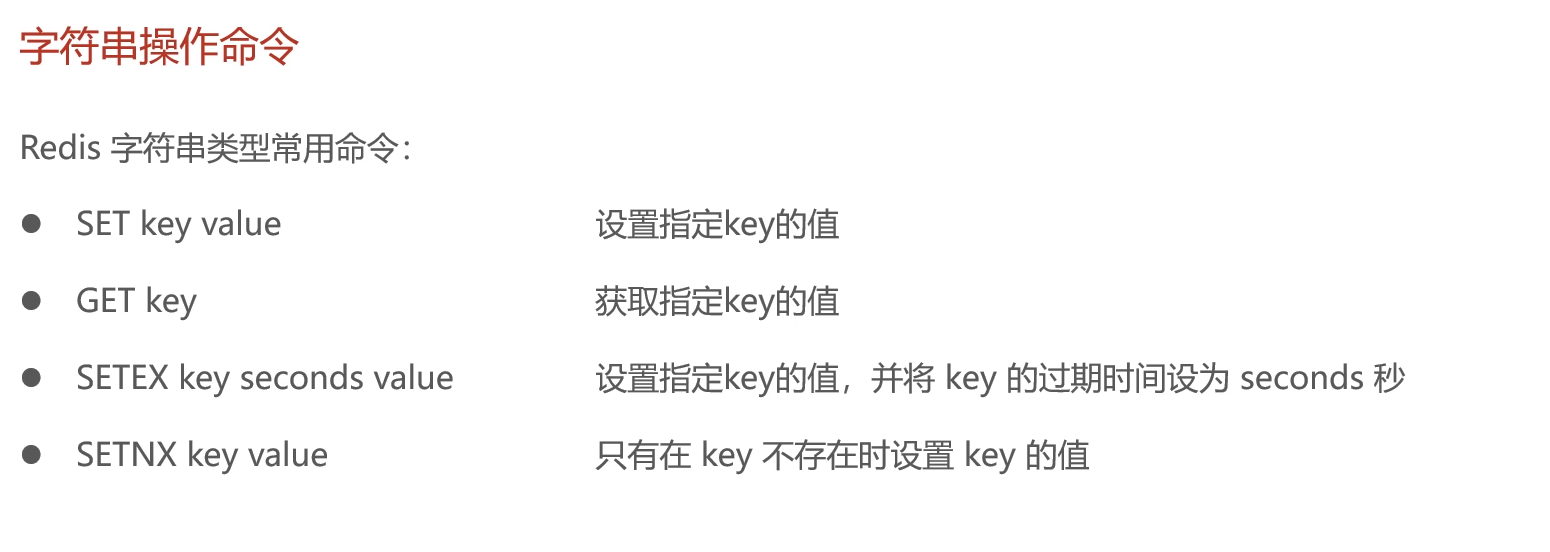
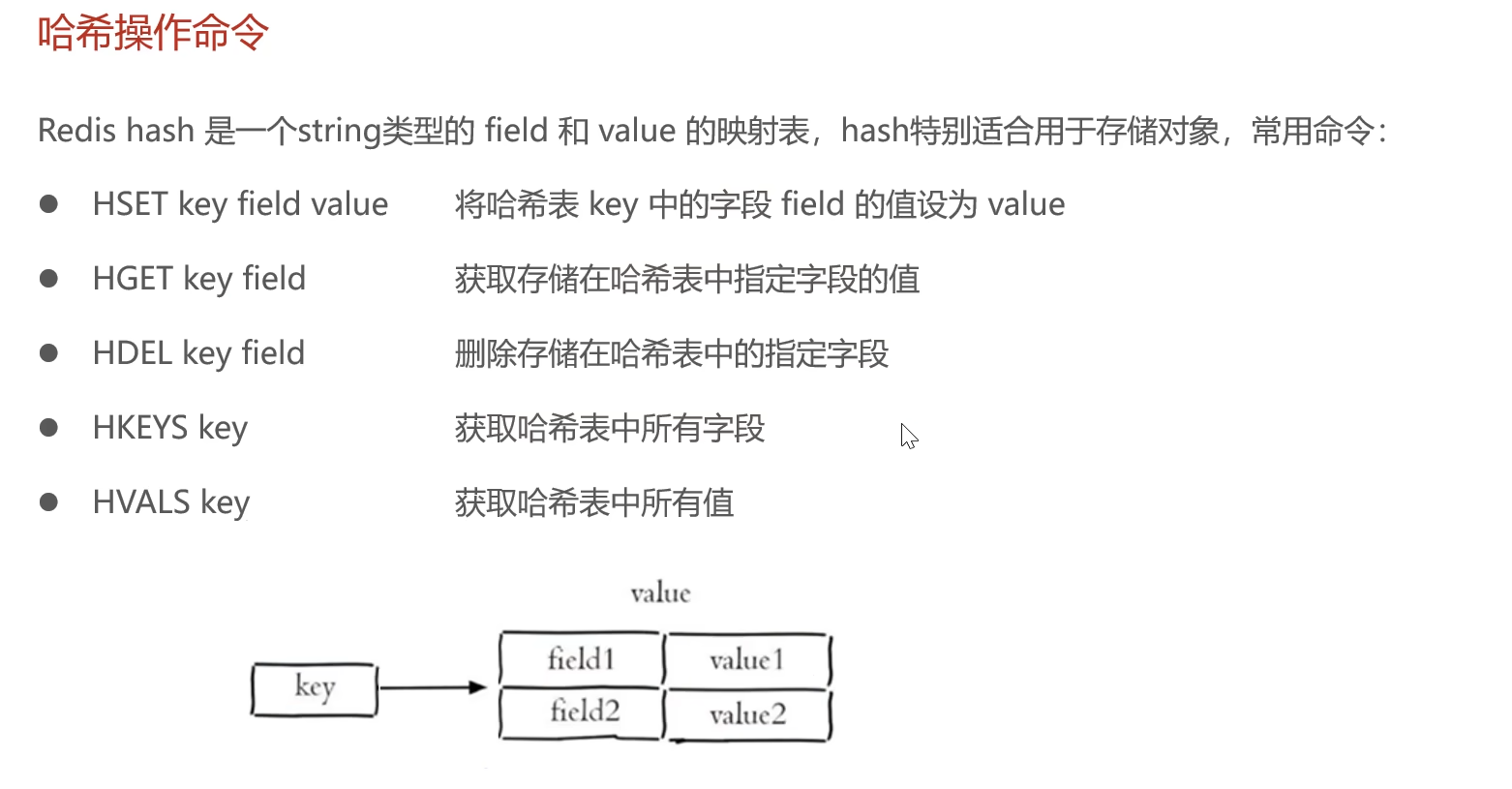
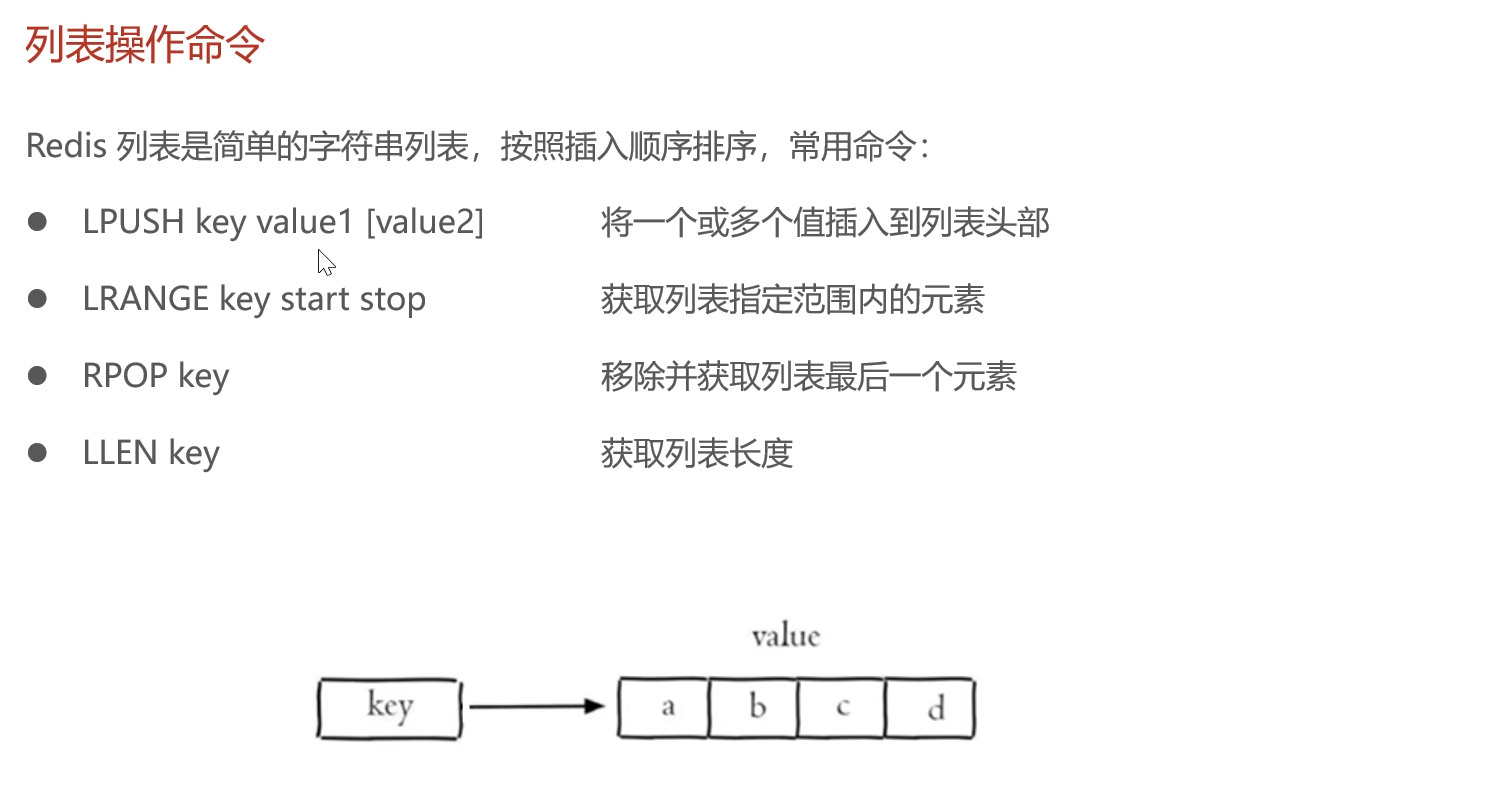
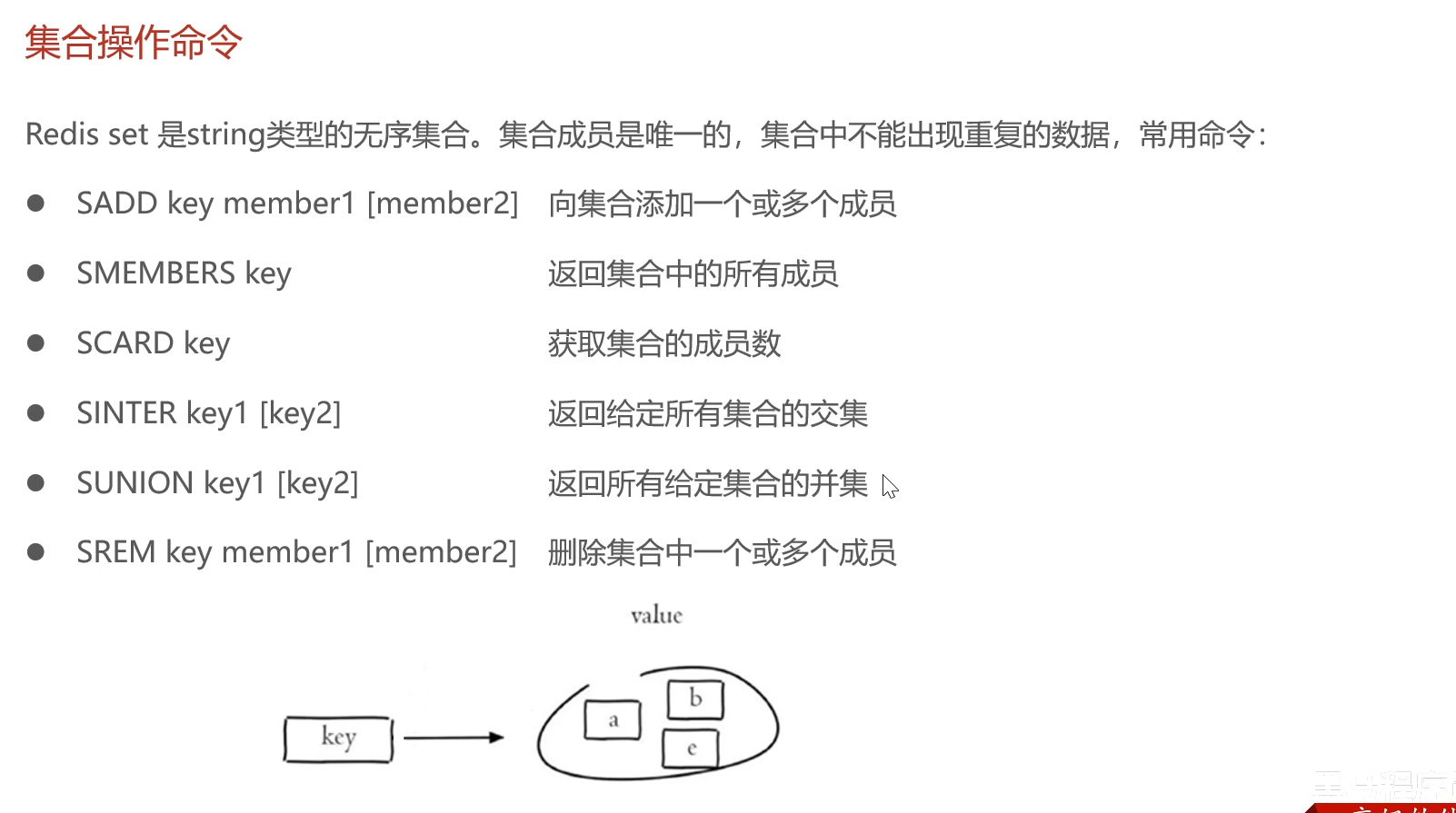
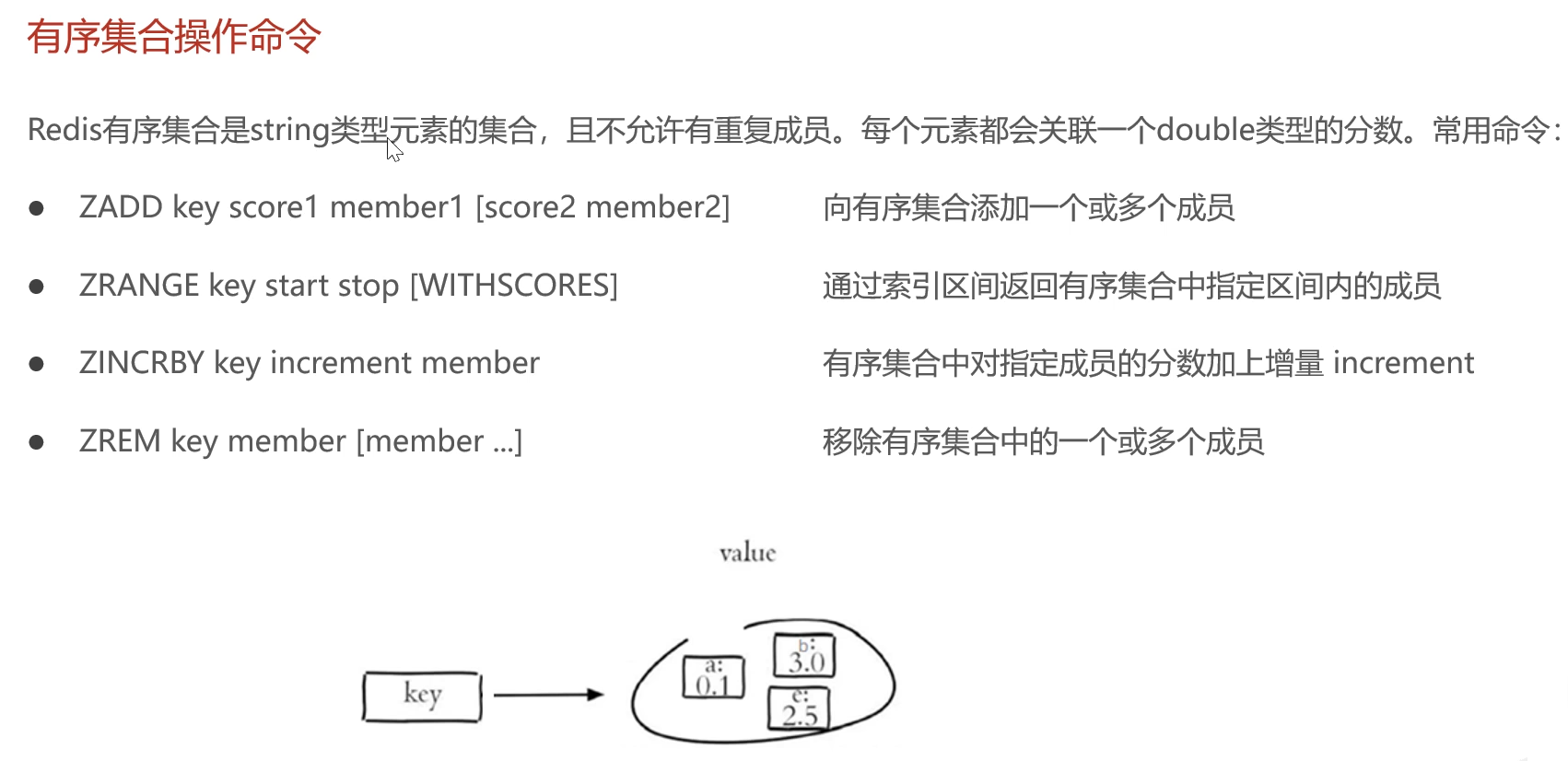
在java中操作Reids:
Redis的java客户端:
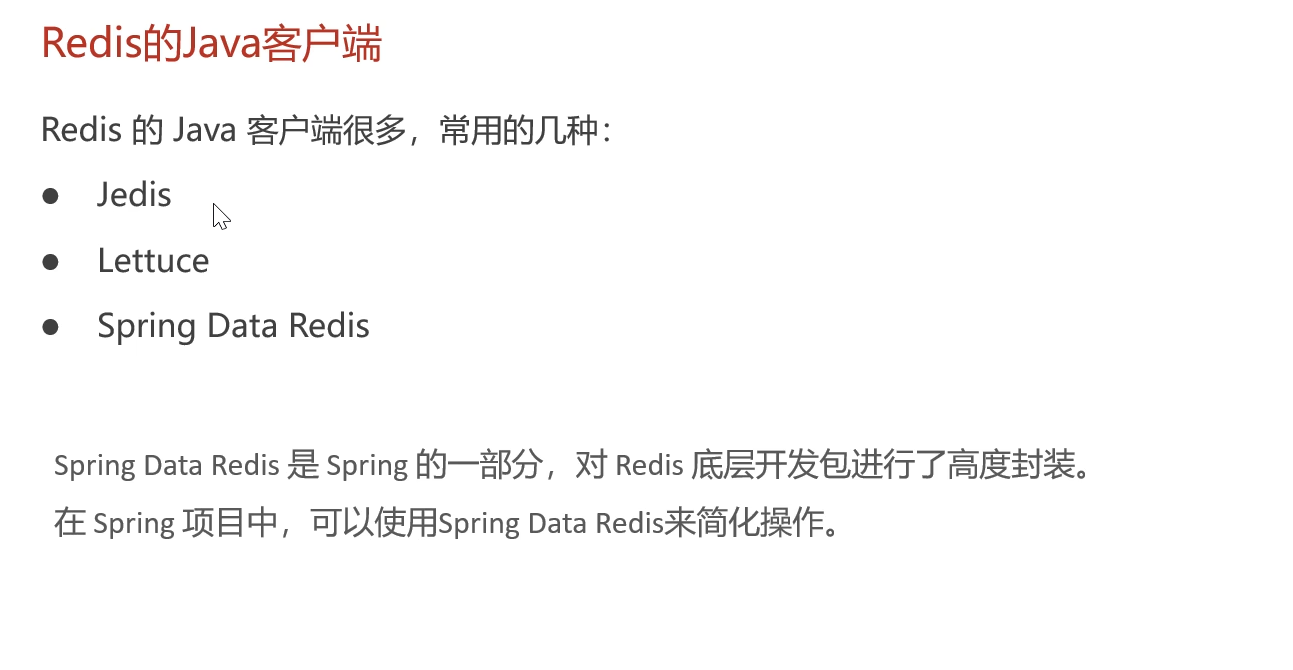
1.导入Spring Data Redis的maven坐标
XML
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2.配置Redis数据源
XML
sky:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
host: localhost
port: 3306
database: sky_take_out
username: root
password: CHC52099
maintainTimeStats: true
redis:
host: localhost
port: 6379
database: 10
3.编写配置类,创建RedisTemplate对象
java
package com.sky.config;
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
@Slf4j
public class RedisConfiguration {
@Bean
public RedisTemplate redisTemplate(RedisConnectionFactory redisConnectionFactory) {
log.info("开始创建redis模板对象...");
RedisTemplate redisTemplate = new RedisTemplate();
//设置redis的连接工厂对象
redisTemplate.setConnectionFactory(redisConnectionFactory);
//设置redis的key序列化器
redisTemplate.setKeySerializer(new StringRedisSerializer());
return redisTemplate;
}
}
4.通过RedisTemplate对象操作Redis
java
package com.sky.test;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.*;
import java.util.concurrent.TimeUnit;
@SpringBootTest
public class SpringDataRedis {
@Autowired
private RedisTemplate redisTemplate;
@Test
public void testRedisTemplate() {
System.out.println(redisTemplate);
ValueOperations valueOperations = redisTemplate.opsForValue();
HashOperations hashOperations = redisTemplate.opsForHash();
ListOperations listOperations = redisTemplate.opsForList();
SetOperations setOperations = redisTemplate.opsForSet();
ZSetOperations zSetOperations = redisTemplate.opsForZSet();
}
/**
* 操作字符串类型的数据
*/
@Test
public void testString() {
redisTemplate.opsForValue().set("hello", "world");
System.out.println(redisTemplate.opsForValue().get("hello"));
redisTemplate.opsForValue().setIfAbsent("hello", "sky");
redisTemplate.opsForValue().setIfPresent("hello", "sky",3, TimeUnit.MINUTES);
}
@Test
public void testHash(){
redisTemplate.opsForHash().put("sky","name","sky");
redisTemplate.opsForHash().put("sky","age",18);
System.out.println(redisTemplate.opsForHash().get("sky","name"));
System.out.println(redisTemplate.opsForHash().get("sky","age"));
}
}
设置营业状态:
需求说明:
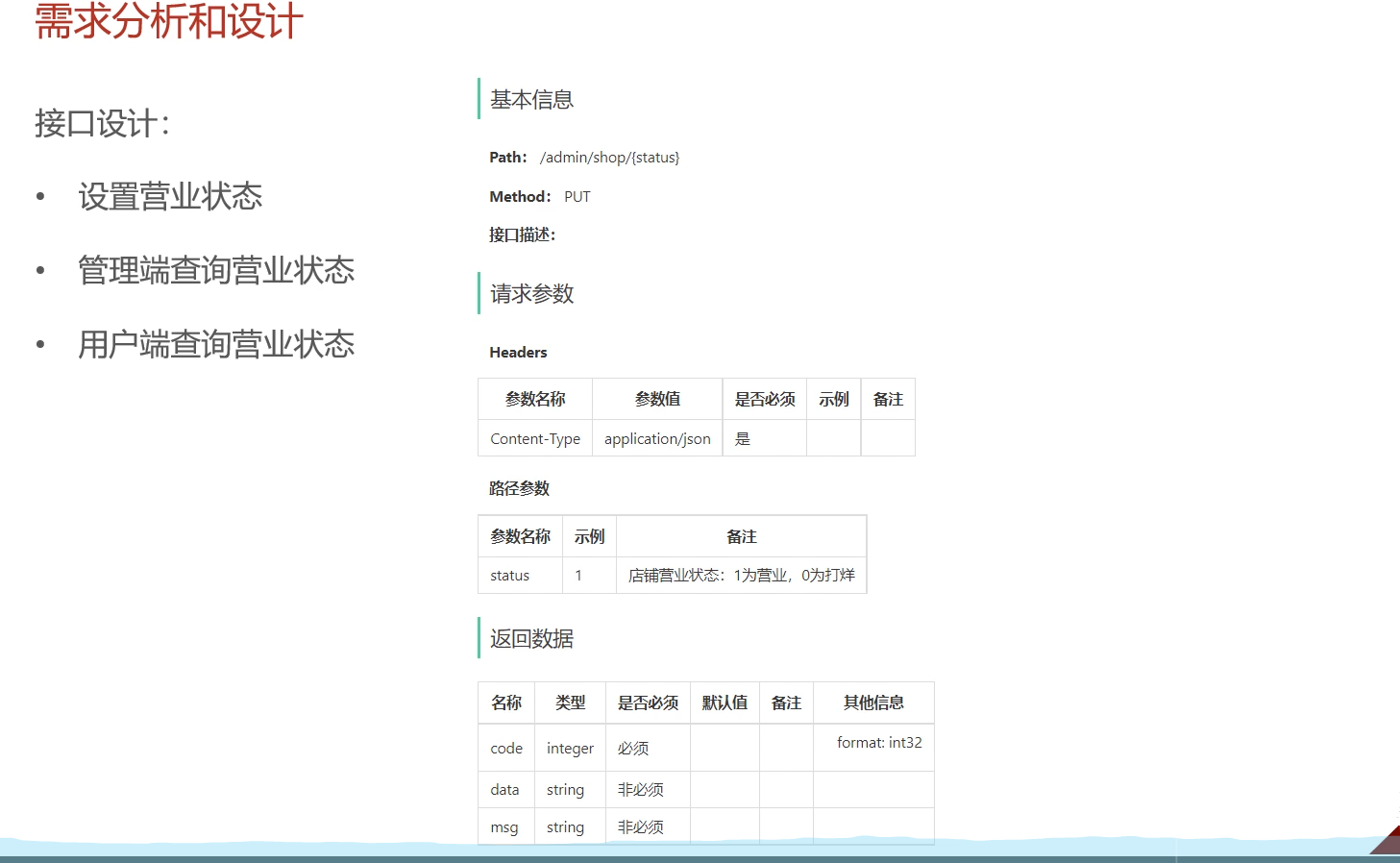
controller层:
java
package com.sky.controller.admin;
import com.sky.result.Result;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController("adminShopController")
@RequestMapping("/admin/shop")
@Api(tags = "店铺相关接口")
@Slf4j
public class ShopController {
@Autowired
private RedisTemplate redisTemplate;
/**
* 设置店铺营业状态
*
* @param status
* @return
*/
@RequestMapping("/{status}")
@ApiOperation("设置店铺状态")
public Result setStatus(@PathVariable Integer status) {
log.info("设置店铺状态为:{}", status == 1 ? "营业中" : "打烊了");
redisTemplate.opsForValue().set("SHOP_STATUS", status);
return Result.success();
}
@GetMapping("/status")
@ApiOperation("获取店铺营业状态")
public Result<Integer> getStatus() {
Integer status = (Integer) redisTemplate.opsForValue().get("SHOP_STATUS");
log.info("获取店铺营业状态为:{}", status == 1 ? "营业中" : "打烊了");
return Result.success(status);
}
}
然后拿接口文档测试没有问题